Bootstrap Scroll to Top Button
A Bootstrap Scroll To Top button, created using jQuery or JavaScript, allows users to smoothly navigate to the page's top. With jQuery, we bind a click event to a button, animating the scroll. JavaScript achieves the same by detecting the scroll position, showing the button when scrolled down, and smoothly scrolling up on click. Both methods enhance user experience and are vital for long web pages.
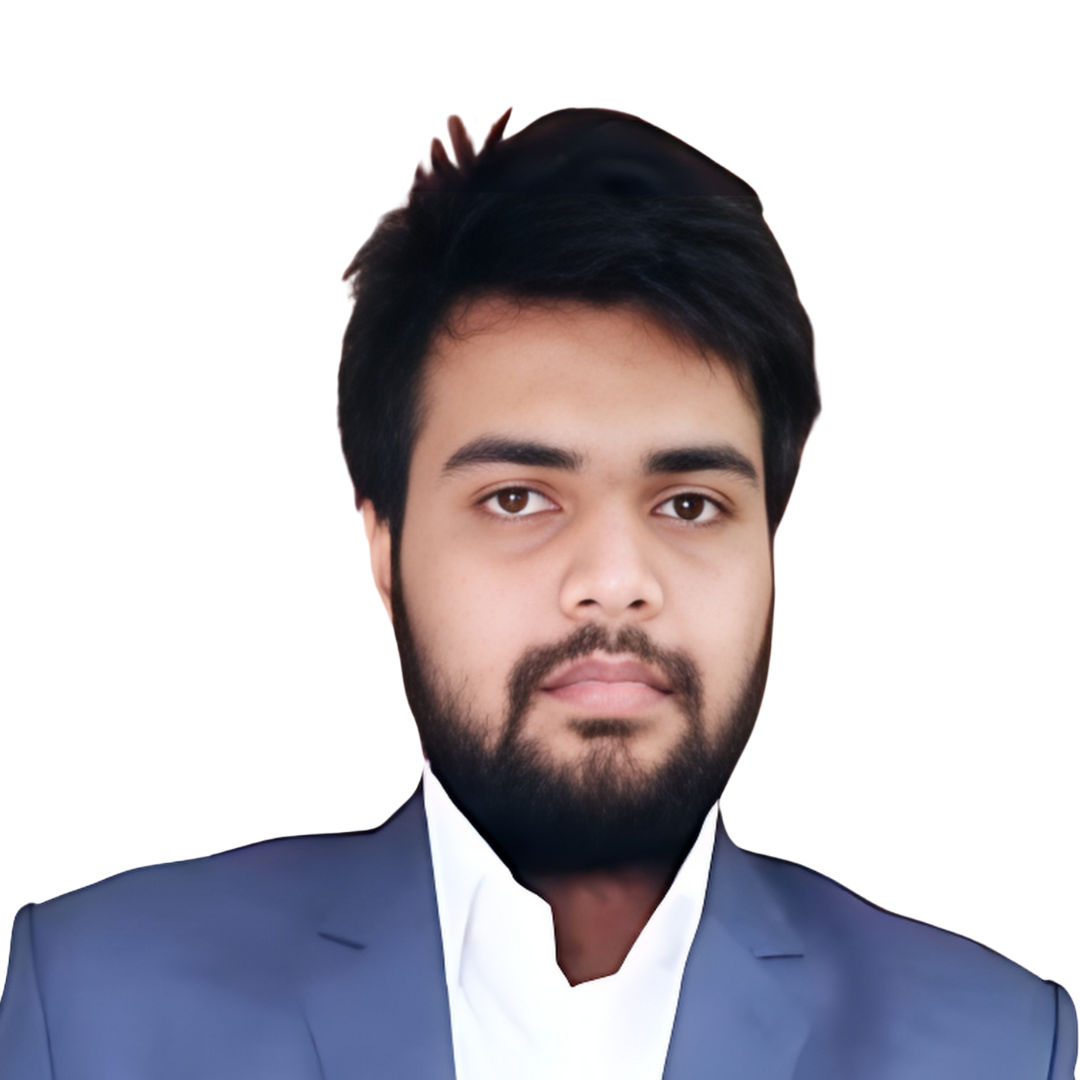
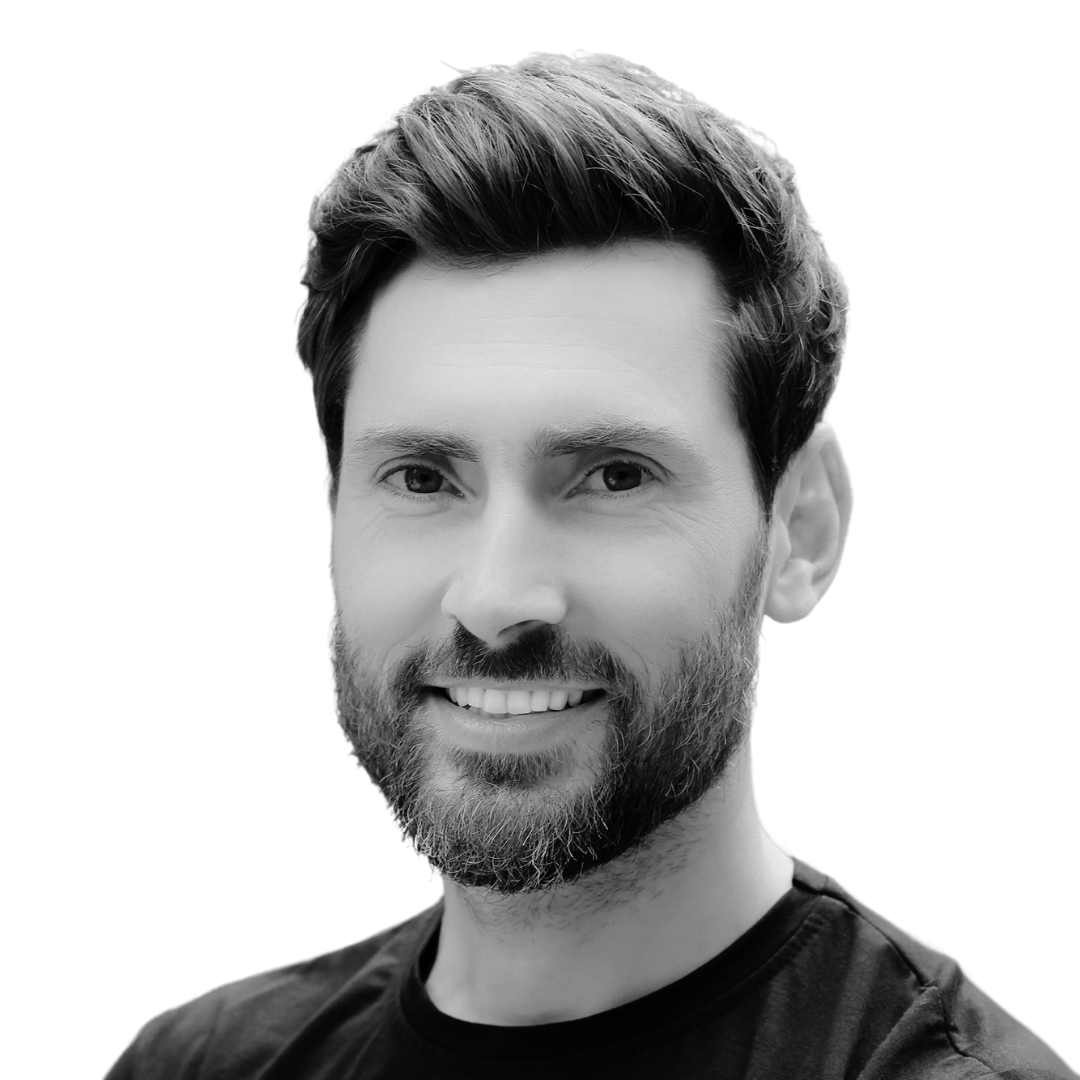
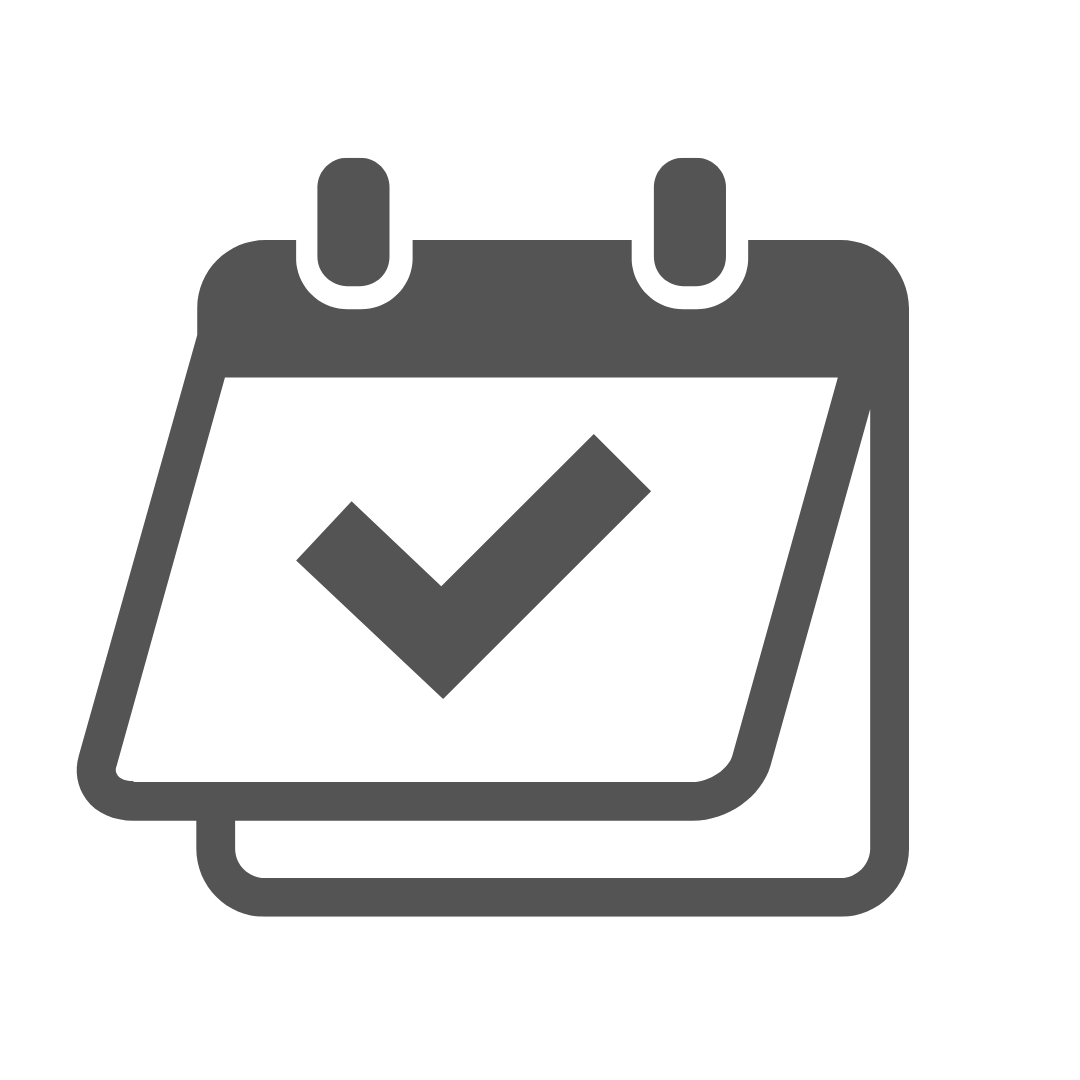
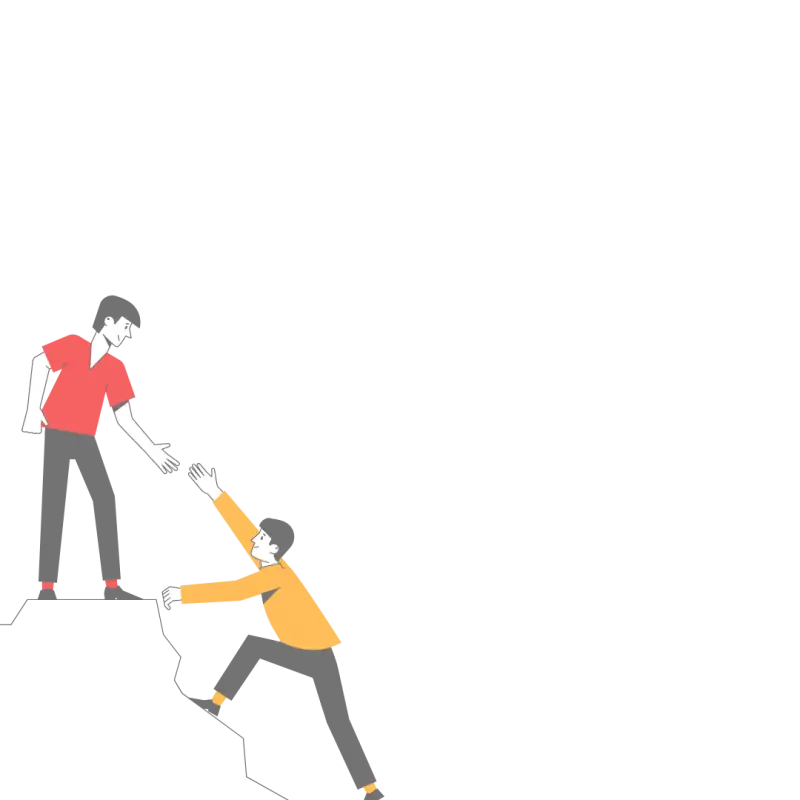
Thanks for your feedback!
Your contributions will help us to improve service.
How can I implement a Bootstrap Scroll To Top button using jQuery in a web page?
This code snippet creates a Bootstrap "Scroll to Top" button using jQuery. Initially hidden, the button appears as you scroll down the page. When clicked, it smoothly scrolls the page to the top. A CSS style defines the button's fixed position at the bottom-right of the screen. In the JavaScript section, an event listener monitors the scroll position. When the user scrolls past 100 pixels, the button fades in; otherwise, it fades out. The click event triggers an animation that smoothly scrolls the page's HTML and body elements to the top, creating a user-friendly "back to top" functionality for long pages.
How can I create a Bootstrap Scroll To Top Button using JavaScript?
This code snippet creates a Bootstrap-style "Scroll to Top" button using JavaScript. Initially hidden, the button becomes visible when the user scrolls 20 pixels down the page. The button is placed at the bottom right corner of the page. When clicked, it smoothly scrolls the page back to the top. This is achieved by detecting the scroll position with window.onscroll
and toggling the button's display accordingly. The button's click event triggers document.body.scrollTop
and document.documentElement.scrollTop
adjustments to ensure compatibility with various browsers. It enhances user experience by providing an easy way to navigate to the page's top without manually scrolling.