Javascript Convert Array to Set - React Js | Vue Js
Javascript Convert Array to Set - React Js | Vue Js:In JavaScript, converting an array to a Set can be done using the Set object. In frameworks like React.js or Vue.js, you can achieve this by creating a new Set and passing the array as an argument. By doing so, duplicate elements will be automatically removed, and only the unique values will be stored in the Set. This can be useful when you want to eliminate duplicates from an array and work with a collection of distinct values.
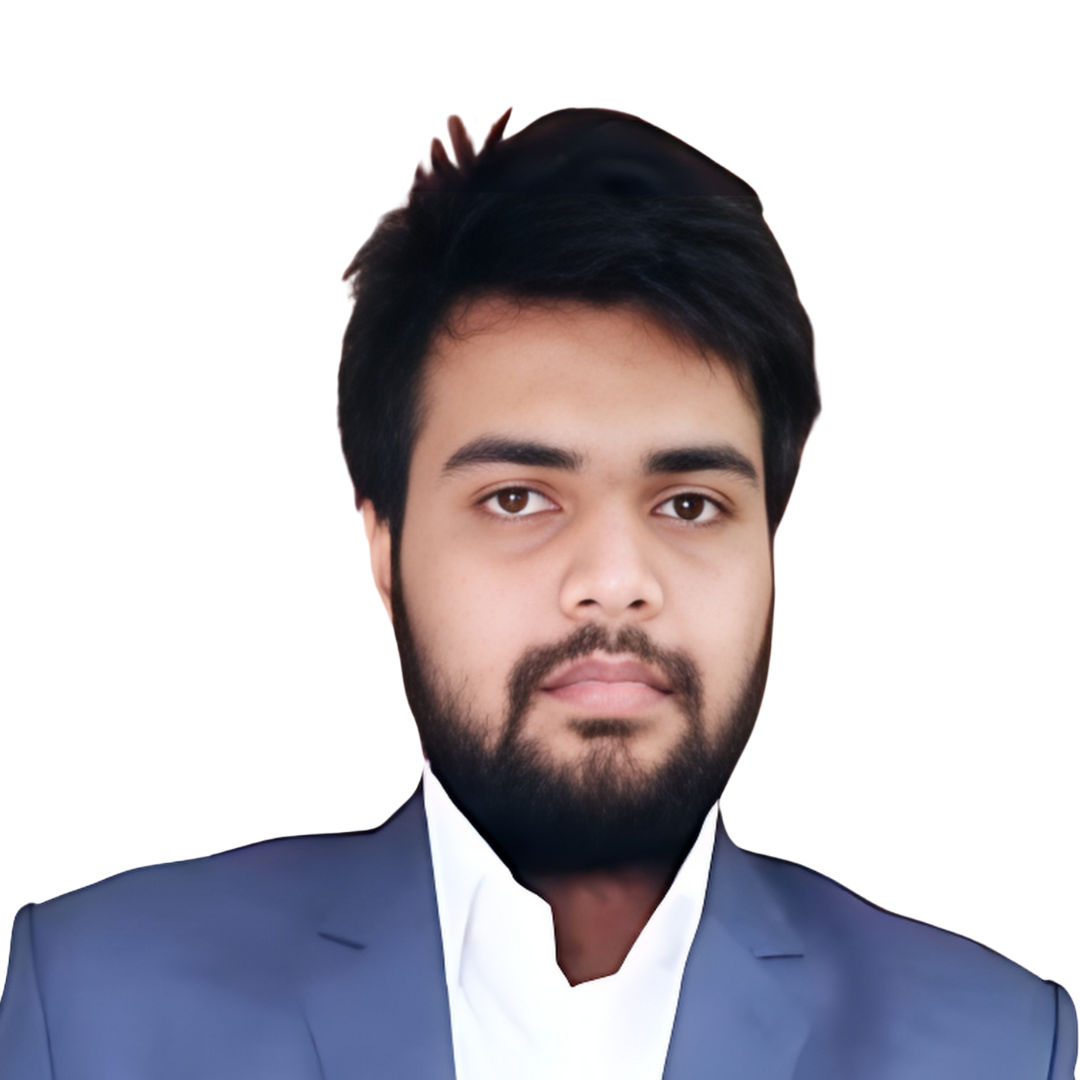
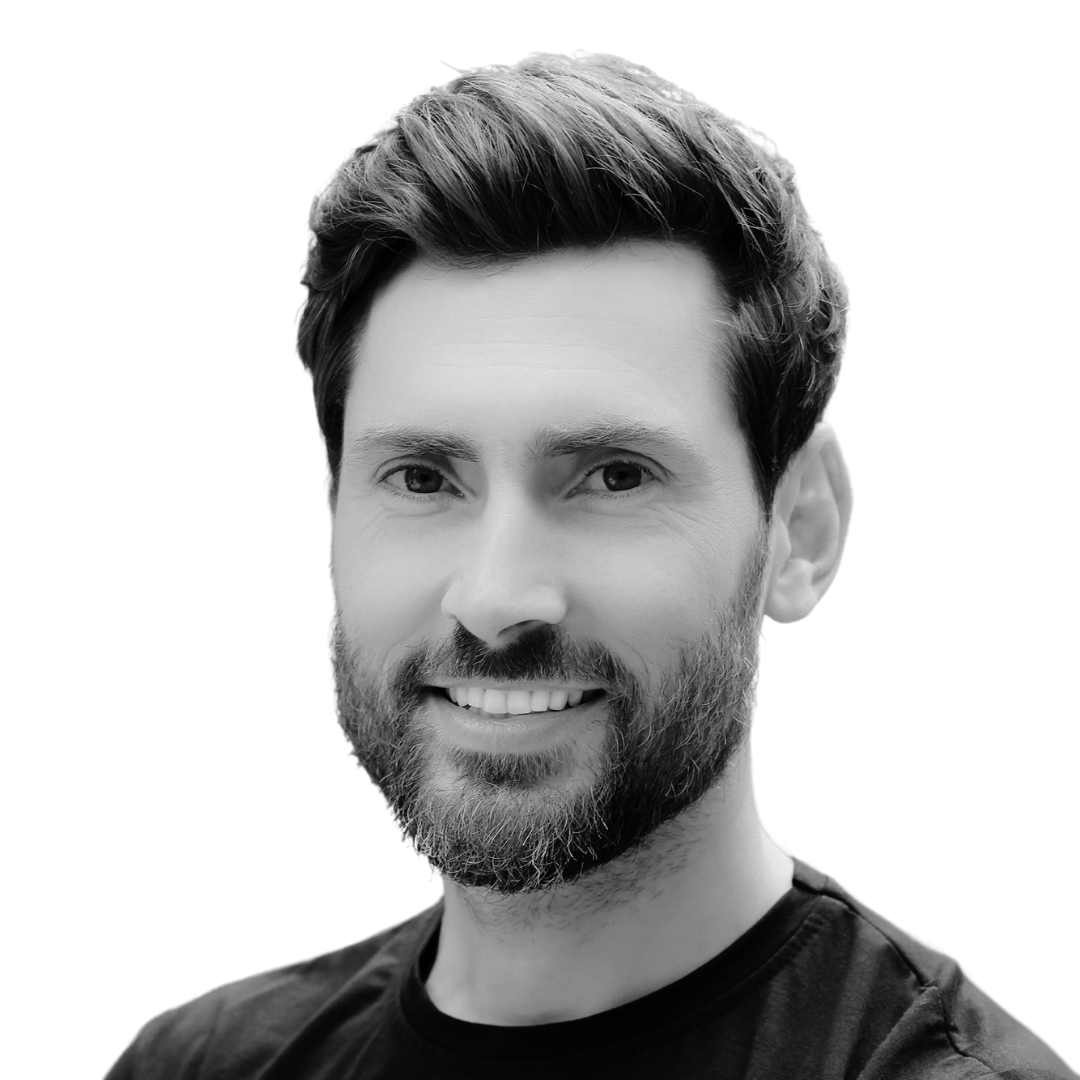
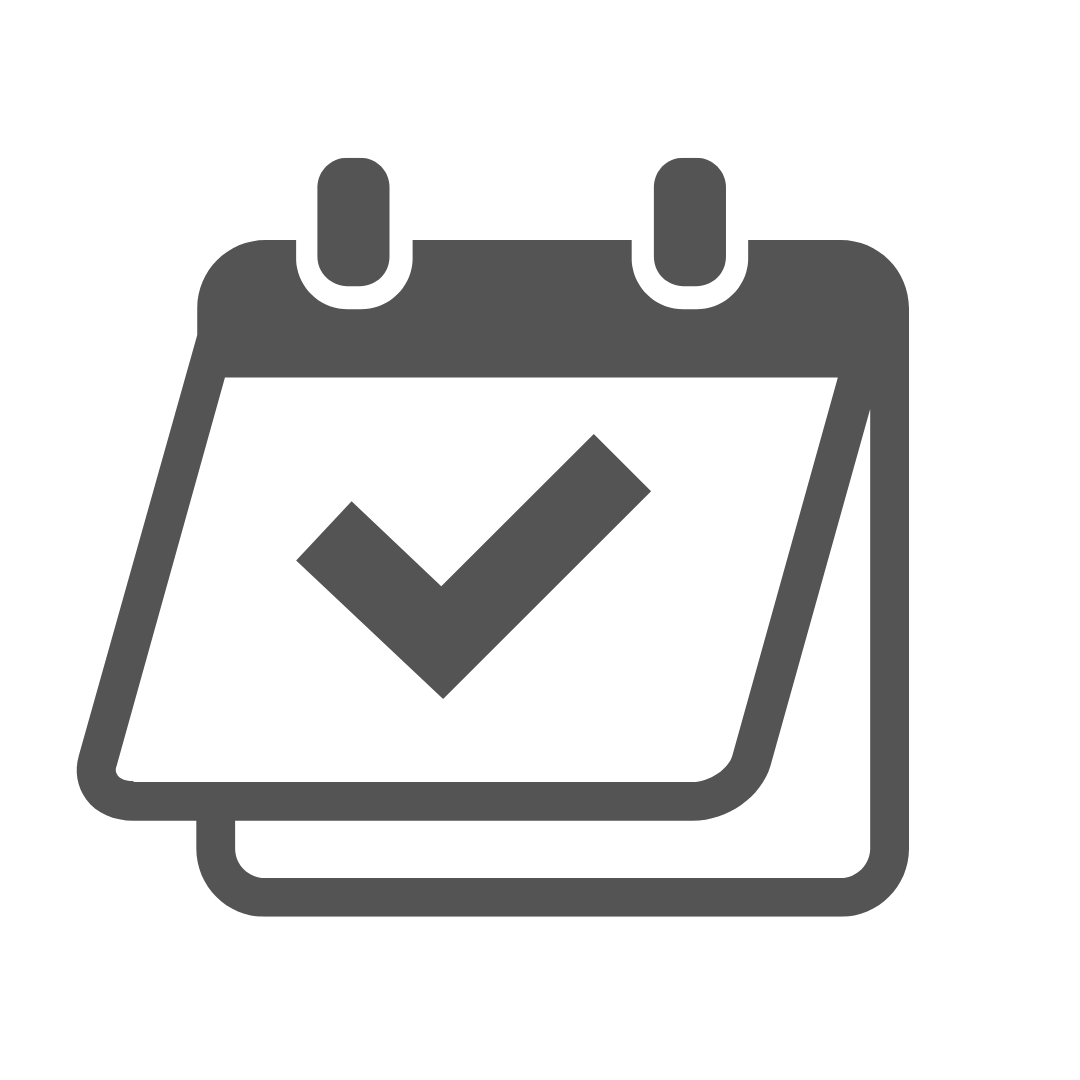
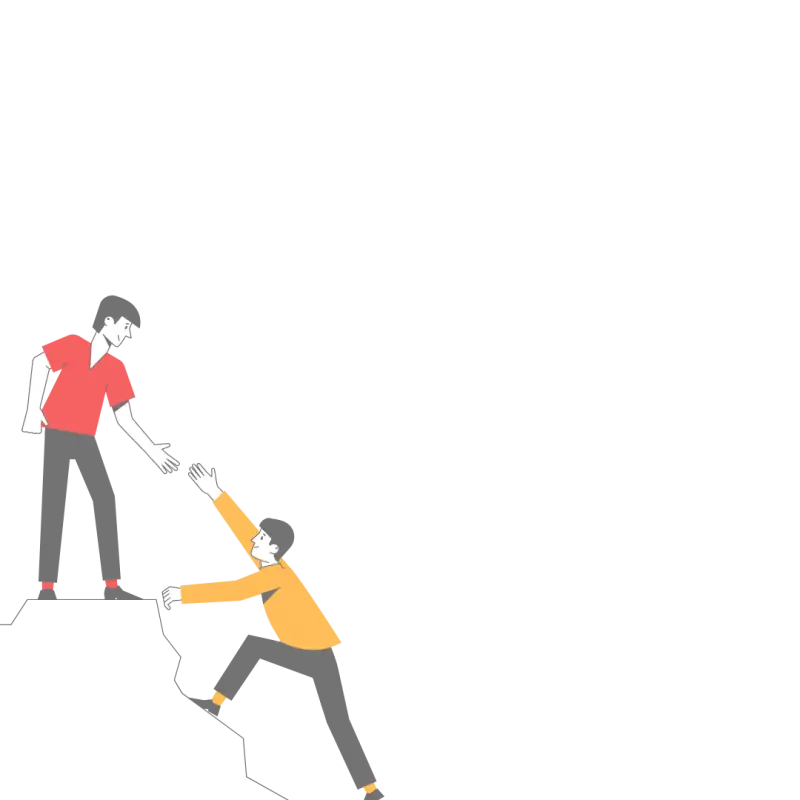
Thanks for your feedback!
Your contributions will help us to improve service.
How can you convert an array to a set in JavaScript?
This JavaScript code converts an array to a set. It defines a function called convertArrayToSet()
that is triggered when the page loads. Inside the function, an array with duplicate elements is created. The original array is displayed on the webpage. Then, a new Set is created from the array, eliminating duplicates. The Set is then converted back to an array using Array.from()
. The converted array is displayed on the webpage.
Output of Javascript Convert Array to Set
How can you convert an array to a set in Reactjs?
This React.js code snippet converts an array to a set using the useState
and useEffect
hooks. Initially, the array [1, 2, 3, 3, 4, 5, 5, "a", "b", "c", "c"]
is stored in the array
state variable. The setSet
function from the useState
hook is used to initialize an empty set.
Inside the useEffect
hook, the array
is converted to a set using new Set(array)
, and then the setSet
function is called to update the set
state variable with the converted set. The converted set is displayed in the rendered JSX using Array.from(set)
.
Output of React Js Convert Array to Set
How can I convert an array to a set using Vuejs?
This Vue.js code snippet demonstrates how to convert an array into a set using Vue.js. The initial array is defined in the data
section, and the converted set is stored in the setArray
variable. The mounted
hook calls the convertArrayToSet
method, which converts the array to a set using Array.from(new Set(this.array))
. The resulting set is assigned to setArray
and can be displayed in the HTML template using the double curly brace syntax.