React Js Remove leading Zero from Number
React Js Remove leading Zero from Number:To remove a leading zero from a number in React.js, you can utilize one of the following methods:
-
Using the parseInt function: Convert the number to a string, apply parseInt with a radix of 10 (to ensure decimal interpretation), and store the result. This will remove any leading zeros. Example:
const parsedNumber = parseInt(number.toString(), 10);
-
Using the Number constructor: Create a new Number object by passing the number as an argument, which automatically removes leading zeros. Example:
const newNumber = new Number(number);
-
Using the parseFloat function: Similar to parseInt, convert the number to a string, apply parseFloat, and store the result. This will remove leading zeros and any trailing decimal places if present. Example:
const parsedFloat = parseFloat(number.toString());
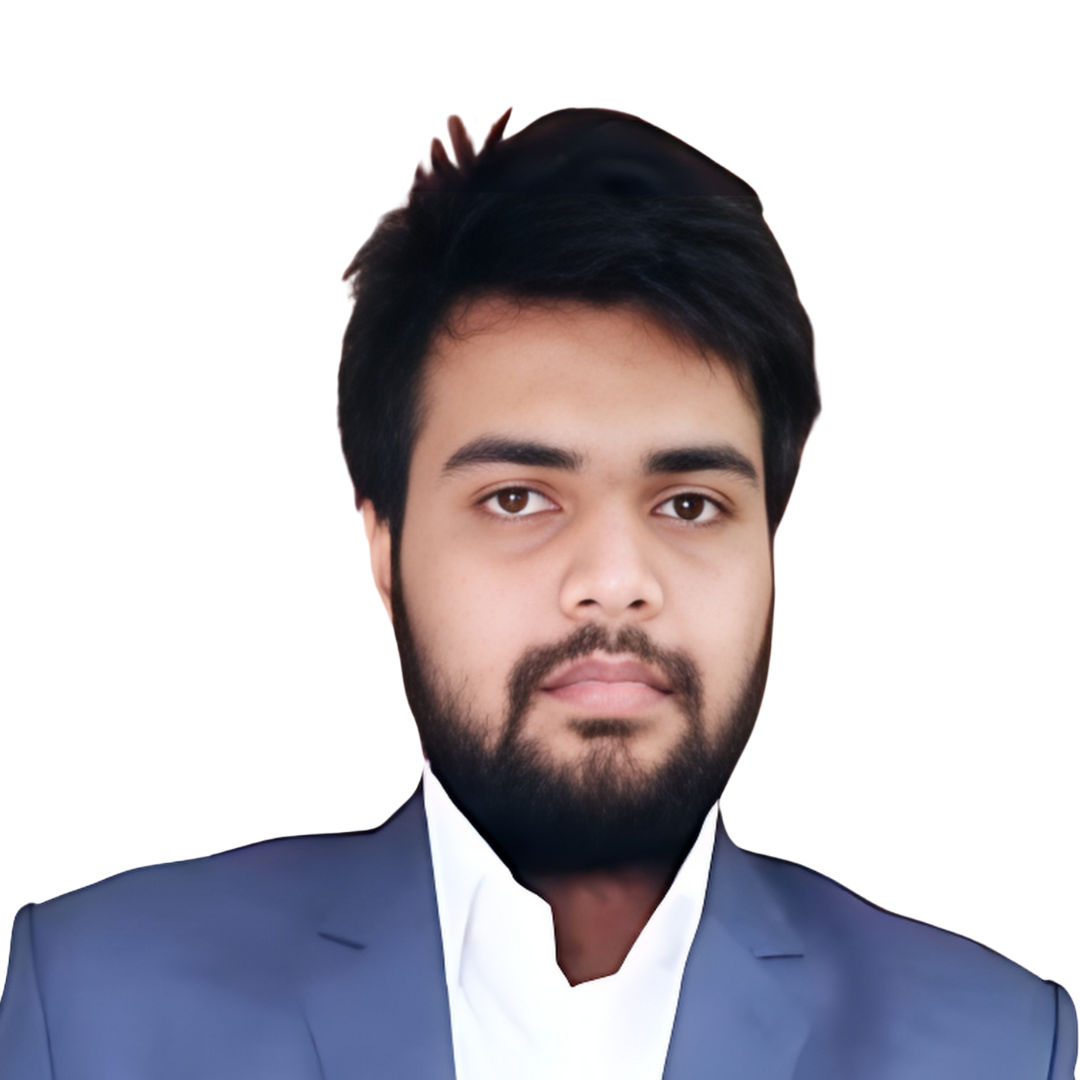
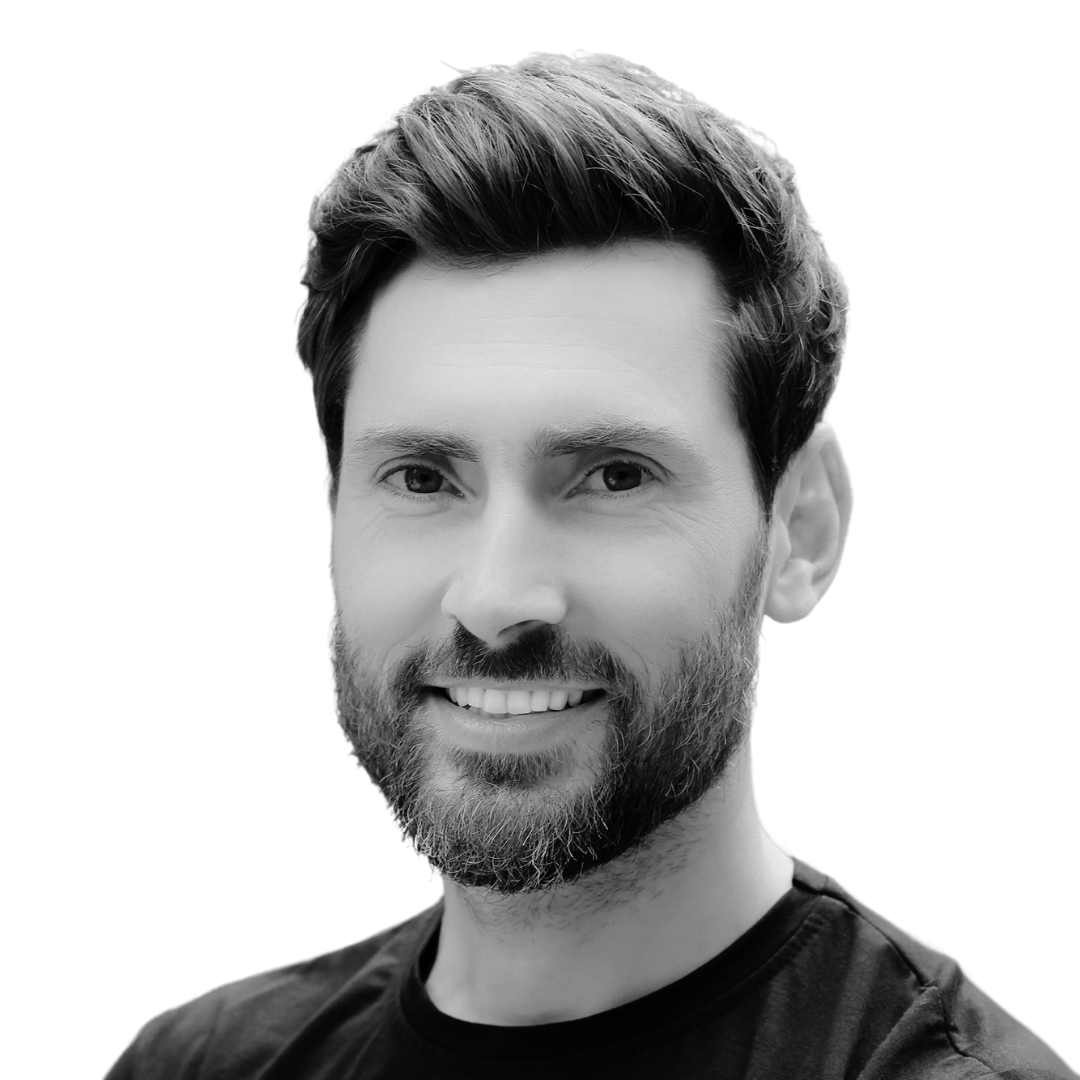
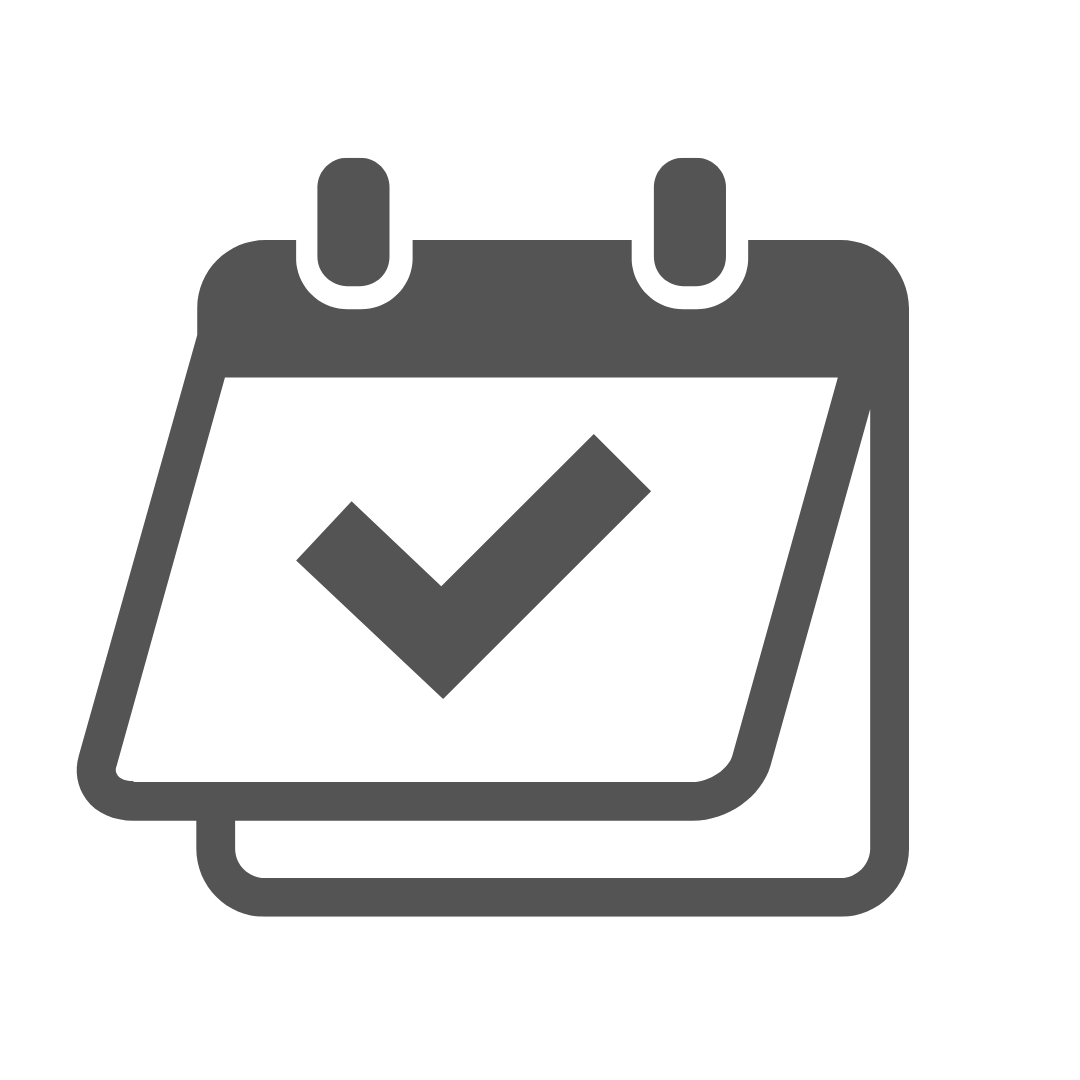
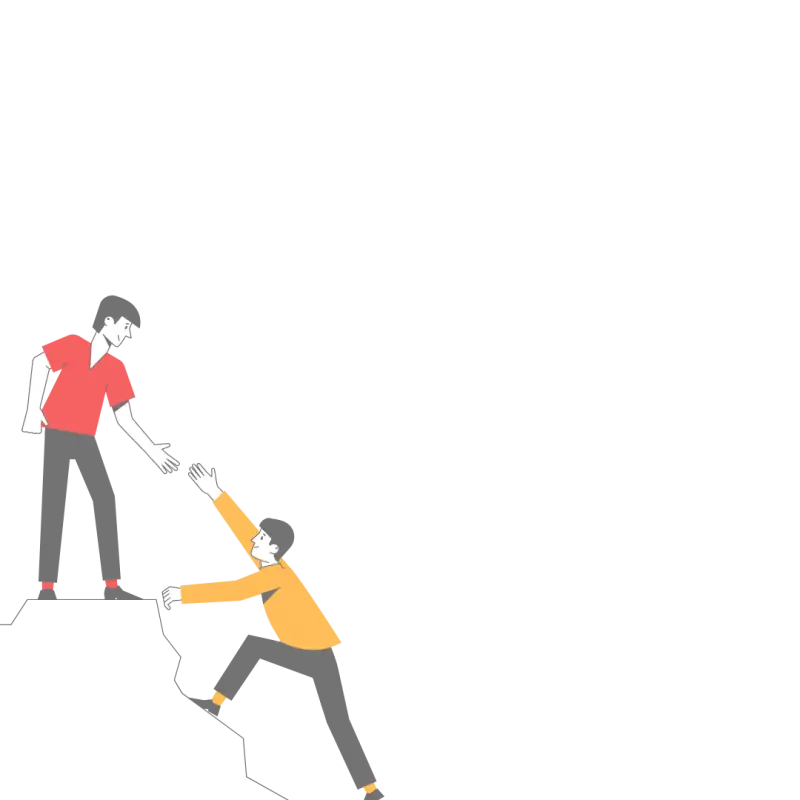
Thanks for your feedback!
Your contributions will help us to improve service.
How can the leading zero be removed from a number in React.js using the parseInt
function?
In the given React.js code, a number "0123" is assigned to the variable number
. The code then uses parseInt()
function to convert the number to an integer, removing the leading zero. The parsed number is stored in the variable parsedNumber
.
Output of React Js Remove Leading Zero from Number
How can you remove leading zeros from a number in React.js using the Number constructor?
In the given React.js code, a number with leading zeros ("0004063") is converted to a numeric value using the Number()
function. The converted number is then displayed on the webpage. The purpose of this code is to demonstrate how React.js can remove leading zeros from a number. The original number ("0004063") is displayed along with the parsed number (4063) on the webpage.
How can you use the parseFloat
function in React.js to remove leading zeros from a number?
The React.js code snippet removes the leading zero from a number. The variable "number" is assigned the value "0123". The parseFloat() function is then used to parse the number, which automatically removes the leading zero. The original number and the parsed number are displayed in the HTML output using JSX syntax.