Firebase Setting with Vue Js
Firebase Setting With Vue Js: Firebase is a Backend-as-a-Service (BaaS) platform that provides a variety of services such as real-time database, authentication, storage, and hosting. In order to use Firebase with Vue.js
-
Create a Firebase project and configure the necessary services that you want to use, such as Authentication, Realtime Database, and Cloud Storage. You can do this by going to the Firebase console and following the prompts to create a new project.
-
Install the Firebase SDK and Vue.js Firebase bindings using npm. The Firebase SDK contains the client-side code necessary to interact with Firebase services, while the Vue.js Firebase bindings provide an easy-to-use API for integrating Firebase with your Vue.js application. You can install both of these dependencies by running the following command:
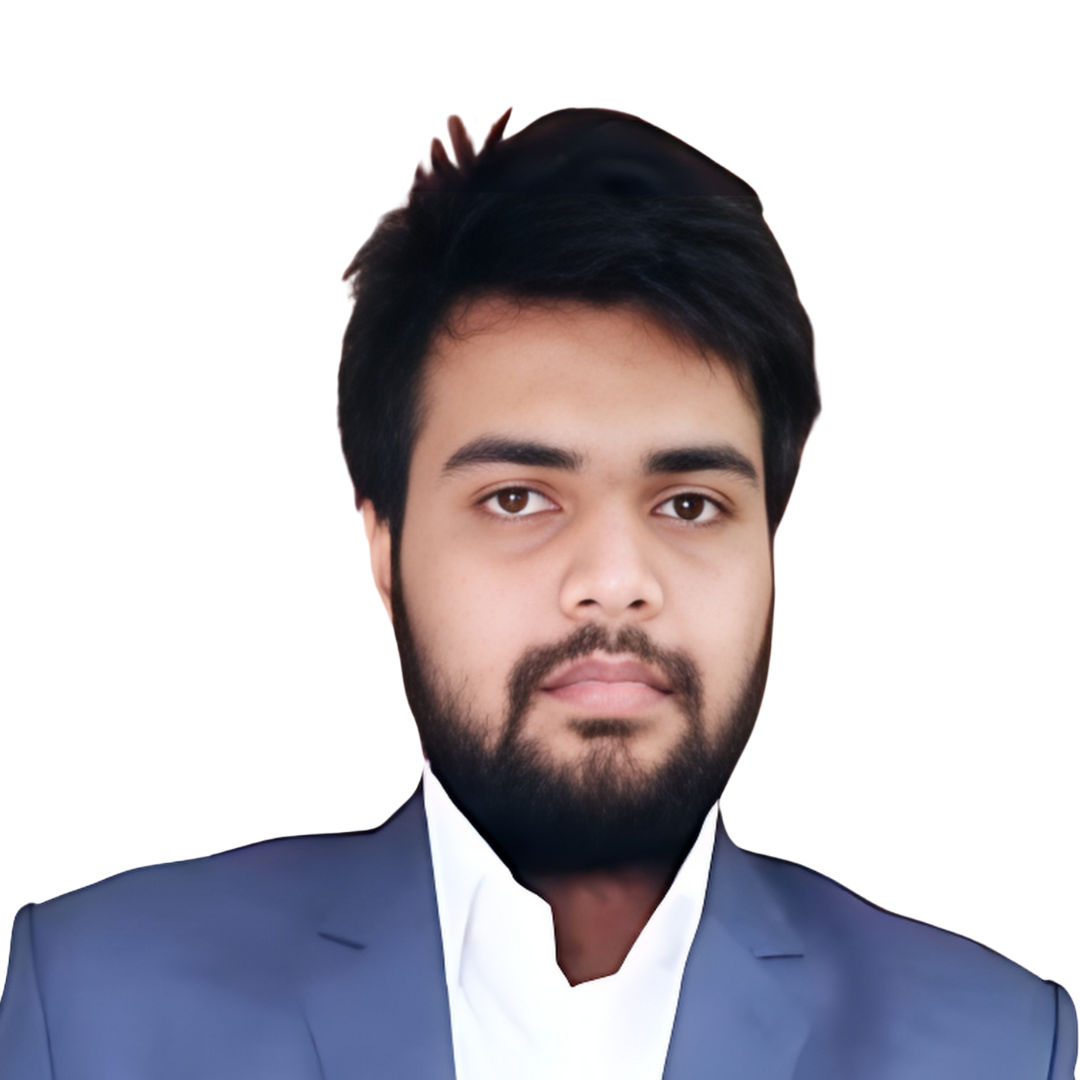
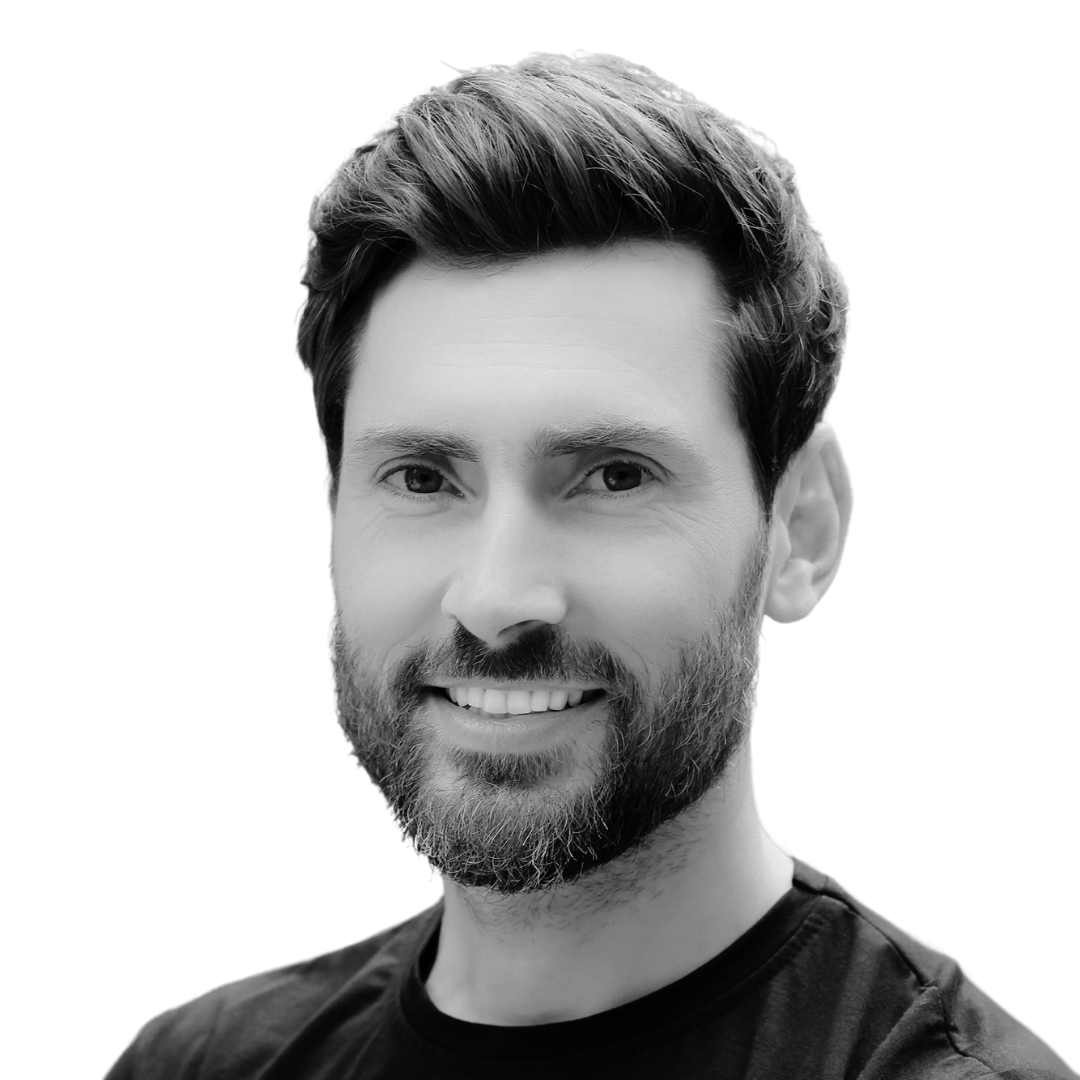
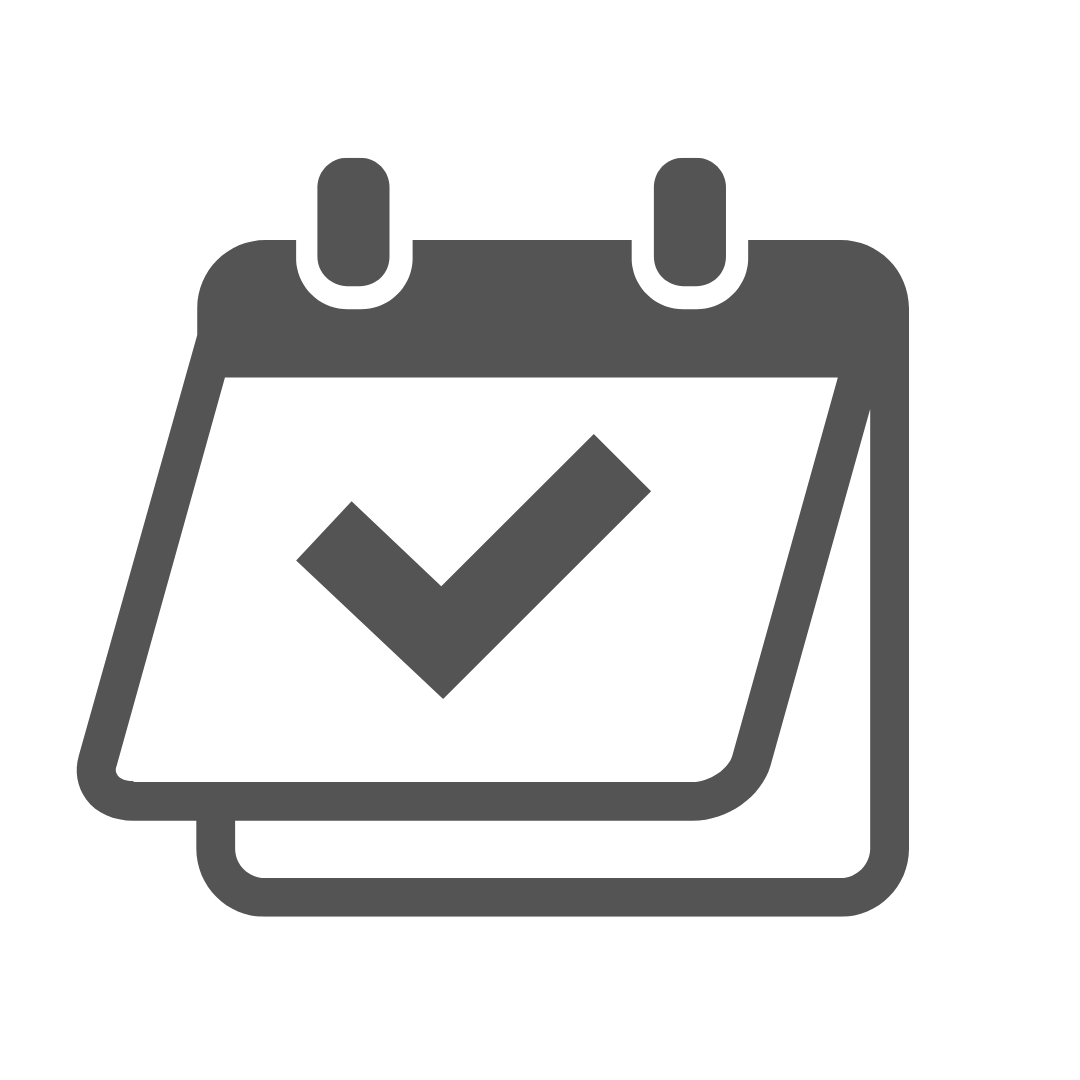
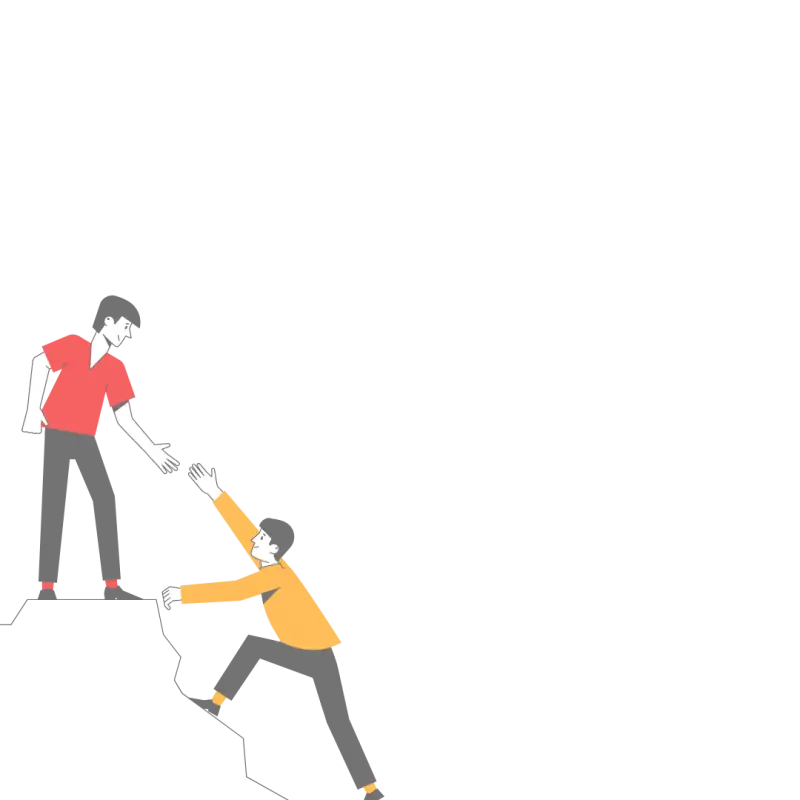
Thanks for your feedback!
Your contributions will help us to improve service.
Setting up Firebase with Vue.js involves the following steps:
-
Create a Firebase project: Go to the Firebase Console, sign in with your Google account, and create a new project.
-
Set up Firebase in Vue.js: Install the Firebase SDK in your Vue.js project using npm or yarn.
Import Firebase and initialize it in your main Vue.js file. You can do this by creating a new file called firebase.js
in your project's src
folder and adding the following code:
import firebase from 'firebase/app'
This line imports the firebase
module from the NPM package firebase
. The firebase
module is the core module that provides access to all the other Firebase services and functionality.
import 'firebase/auth'
This line imports the auth
submodule of the firebase
module. The auth
submodule provides authentication functionality, such as user sign-up, sign-in, and authentication state management.
import 'firebase/firestore'
This line imports the firestore
submodule of the firebase
module. The firestore
submodule provides access to the Firestore database, which is a NoSQL document-based database provided by Firebase.
Firebase Setting with Vue Js Code
This above code defines a JavaScript object called firebaseConfig
that contains the necessary configuration information for connecting to a Firebase project. The values for apiKey
, authDomain
, databaseURL
, projectId
, storageBucket
, messagingSenderId
, and appId
should be replaced with the actual values for your Firebase project.
This above code initializes the Firebase app with the configuration information provided in firebaseConfig
. It should be called before using any Firebase services.
These above lines define and export two constants: auth
and db
. auth
is initialized as firebase.auth()
, which provides authentication functionality. db
is initialized as firebase.firestore()
, which provides access to the Firestore database. These constants can be imported and used in other parts of the code to access the corresponding Firebase services.