Vue Js Generate Random Id
Vue Js Generate Random Id: In Vue.js, generating a random ID can be achieved by using a combination of built-in methods and JavaScript functions. One common approach is to use the "Math.random()" method to generate a random number and then convert it to a base 36 string using the "toString()" method. This string can then be sliced to a desired length to generate the ID. Another approach is to use the "Date.now()" method to get a unique timestamp and append a random number using the "Math.floor()" and "Math.random()" functions. Either method can be used to generate a unique ID that can be used for various purposes in Vue.js applications.
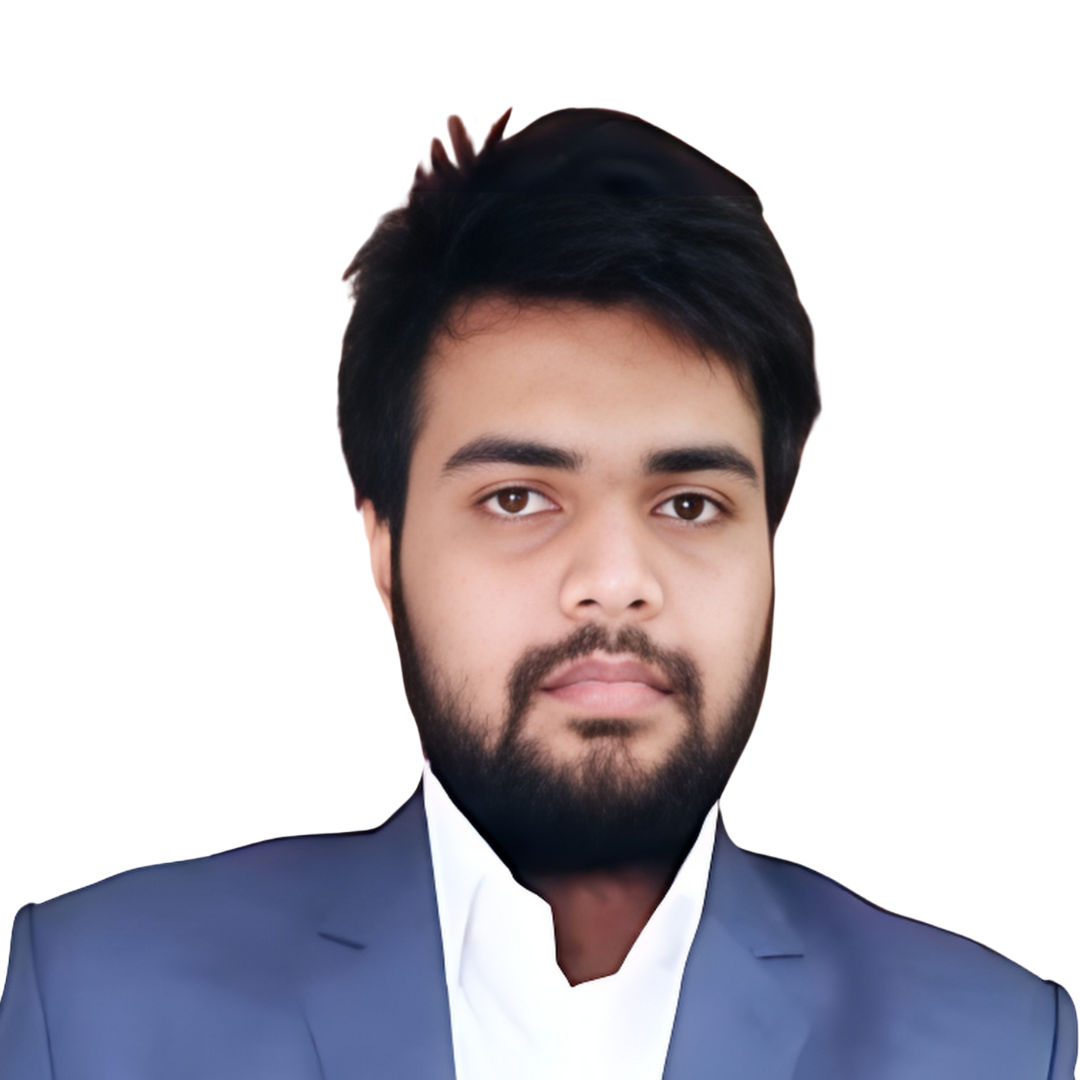
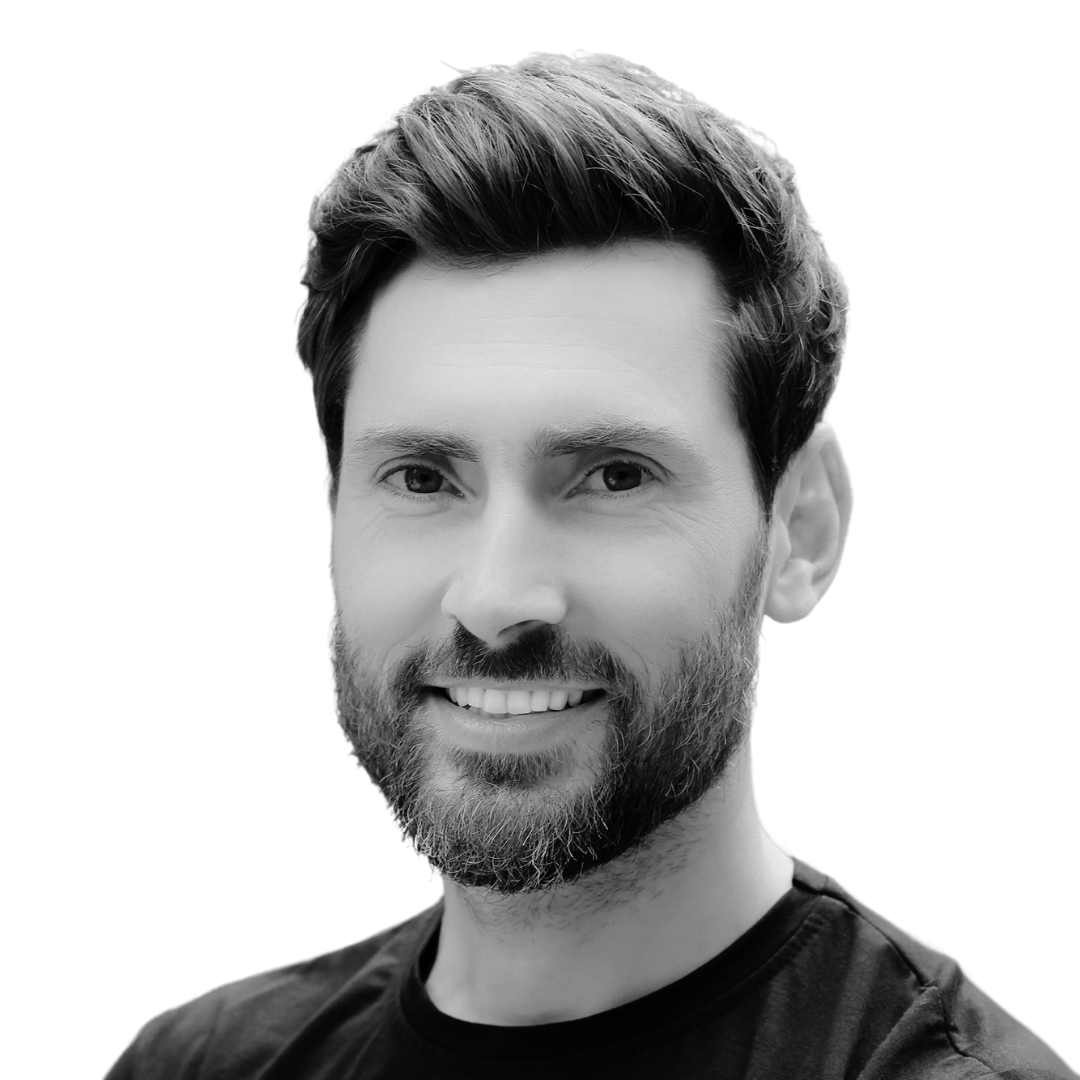
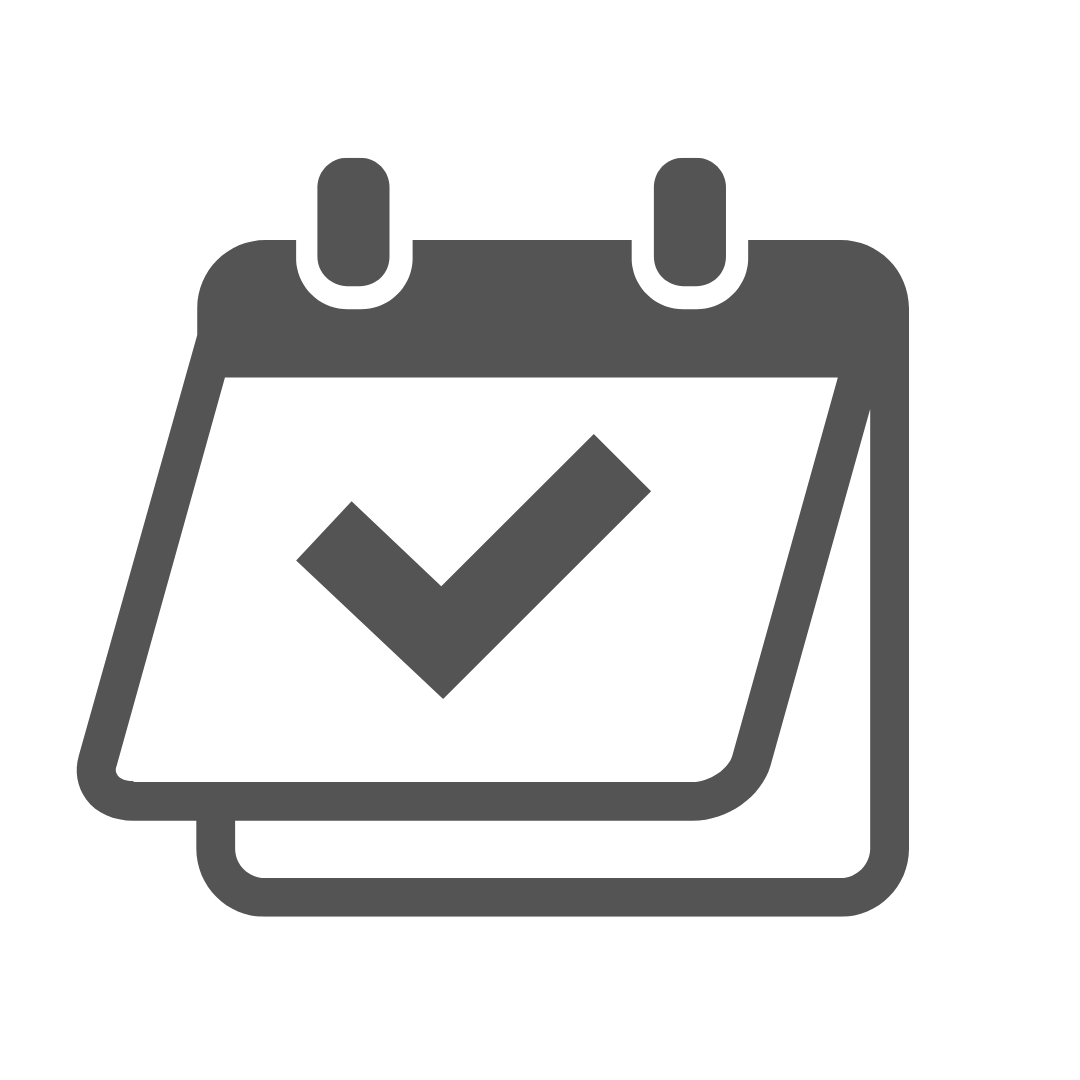
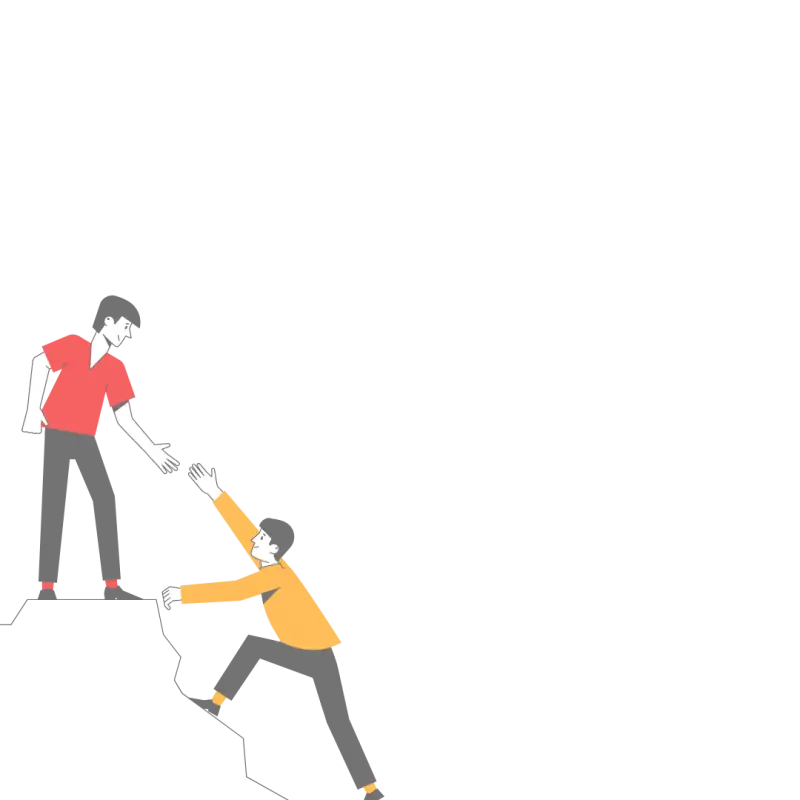
Thanks for your feedback!
Your contributions will help us to improve service.
How can Vue Js be used to generate a random ID for elements in a web application?
This is a Vue.js script that generates a random ID when a button is clicked. The generated ID is displayed in a heading element. The code uses the Vue.js framework to bind the message variable to the heading element, and the generateId method to update the message variable with a randomly generated ID. The random ID is created by concatenating a prefix, a random string, and the current timestamp. The code is written in JavaScript and uses Vue.js syntax to create a reactive UI that updates dynamically.