Vue Js URL Encode/Decode
Vue Js URL Encode/Decode Method: The encodeURIComponent() function in Vue.js is used to encode URLs as a URI component by replacing each instance of certain characters by one, two, three, or four escape sequences representing the UTF-8 encoding of the character, whether The decodeURIComponent() method in Vue.js is a built-in function that is used to Decode URLs. It takes an encoded URI component and returns the decoded value. Here in this tutorial, we will learn how to encode and decode URLs by using native JavaScript and Vue.js.
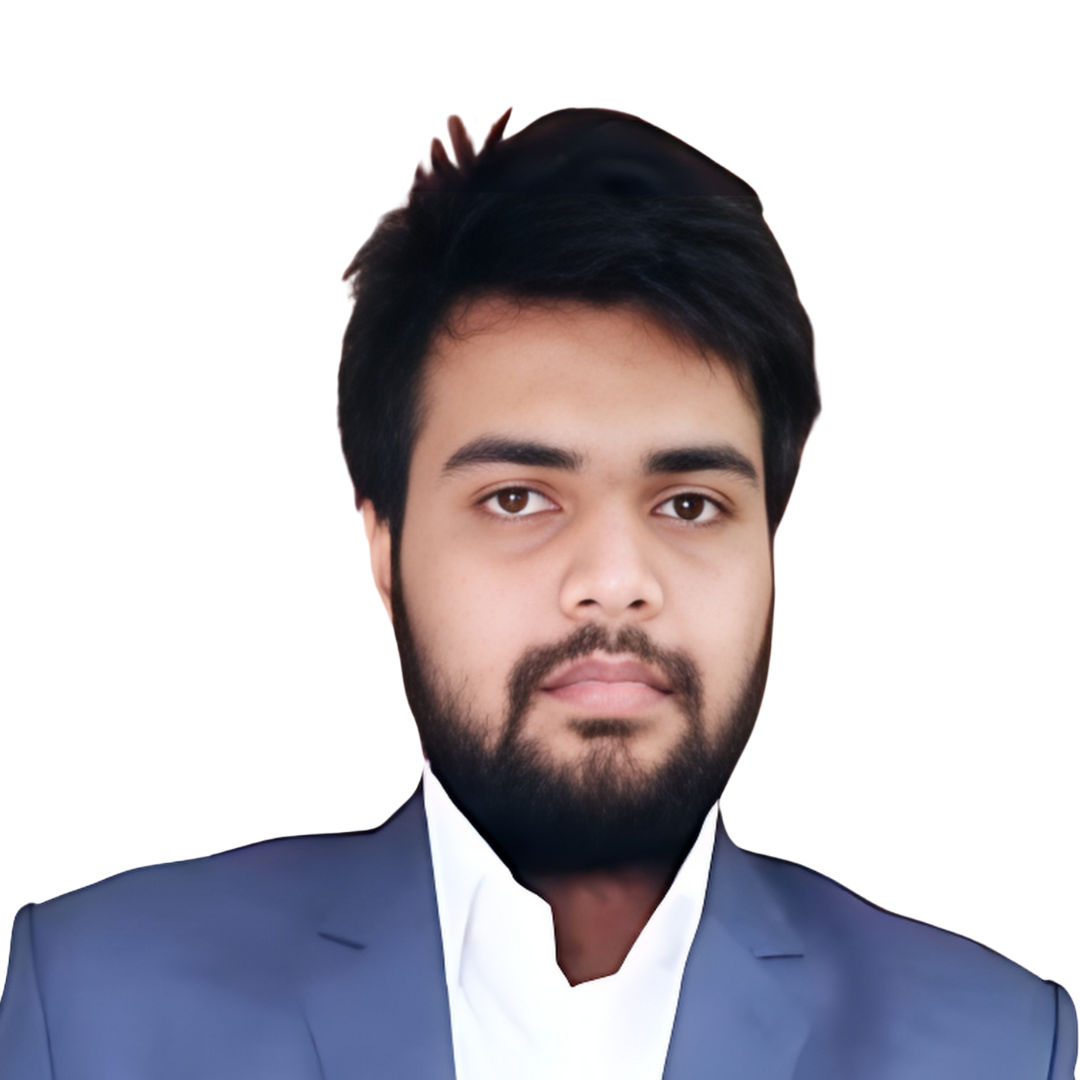
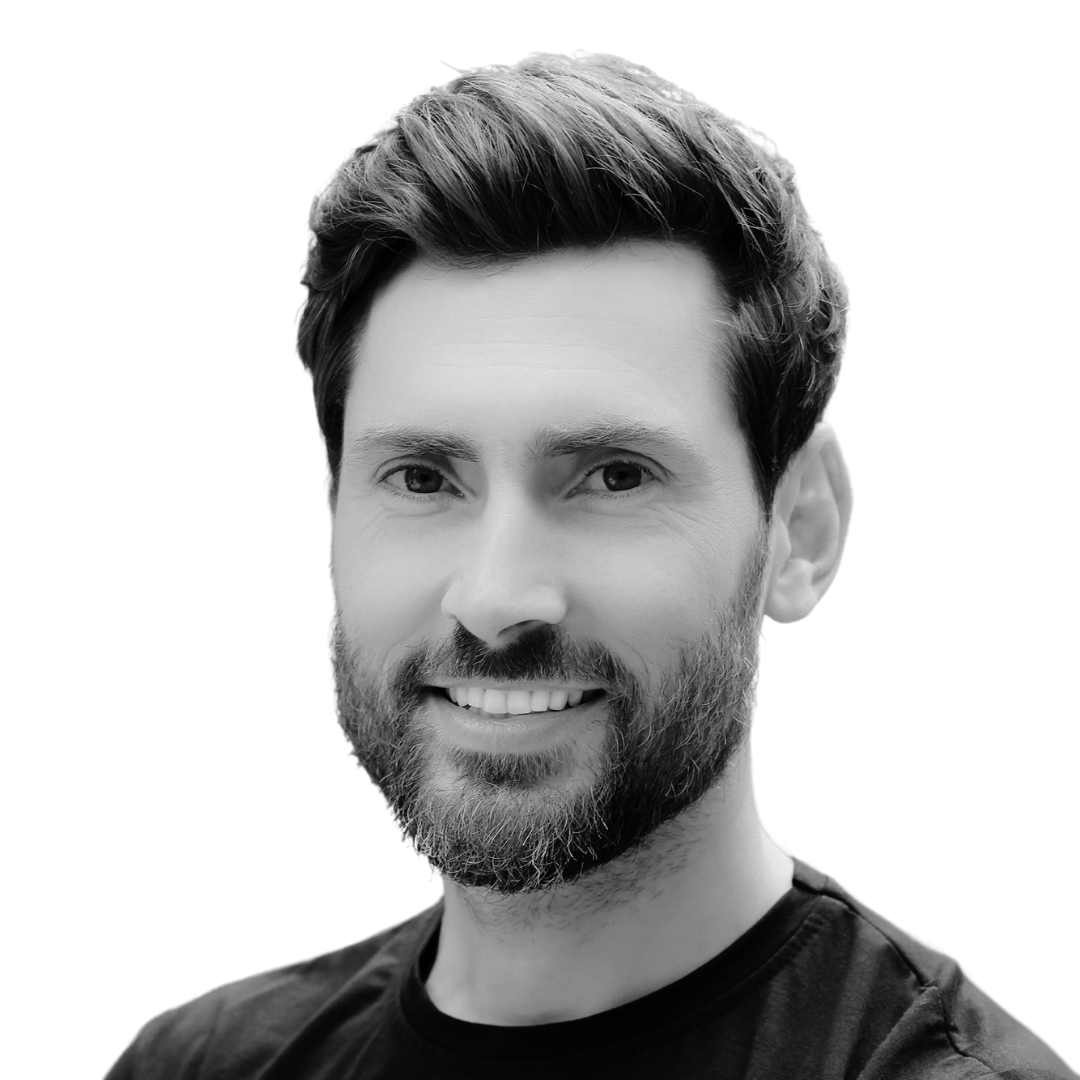
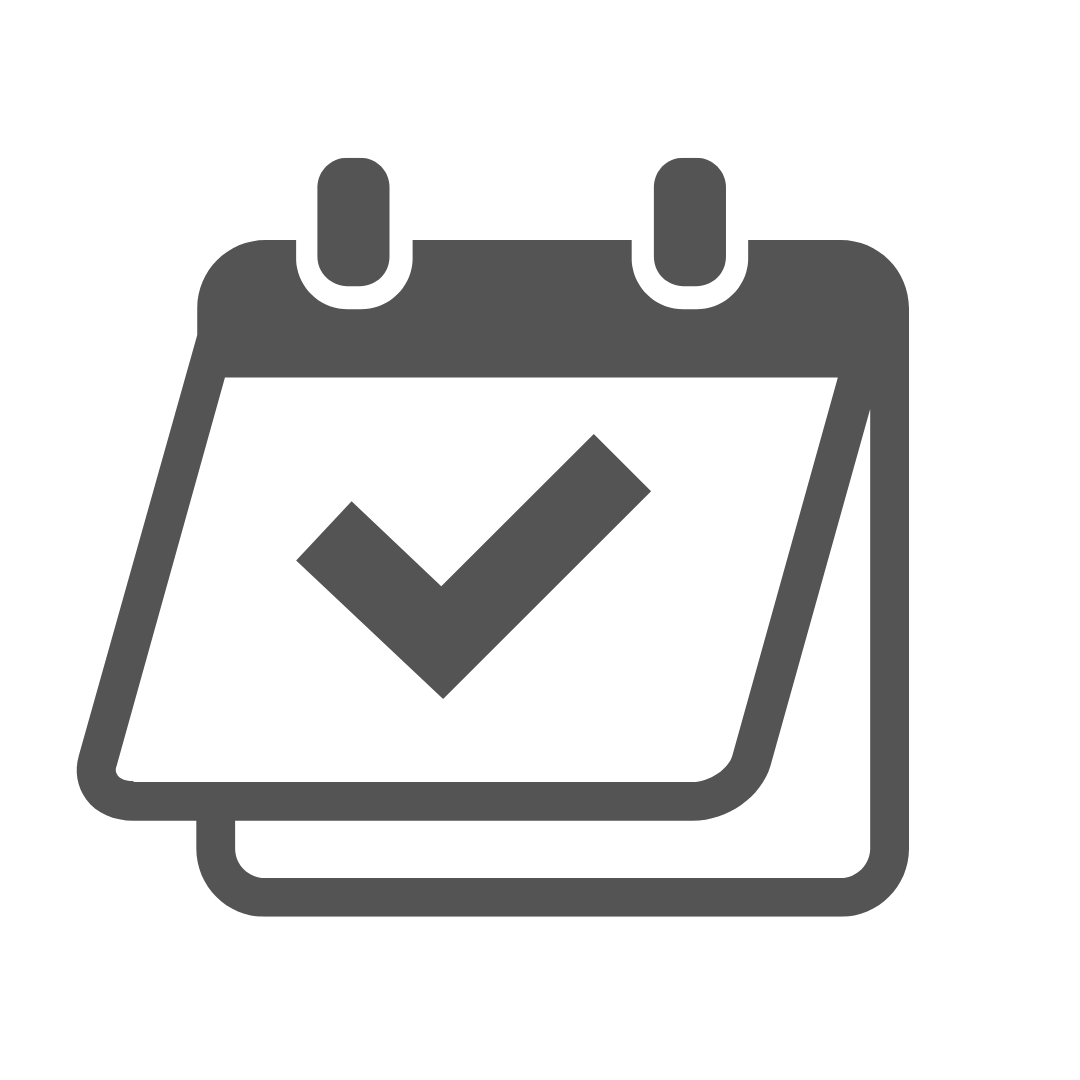
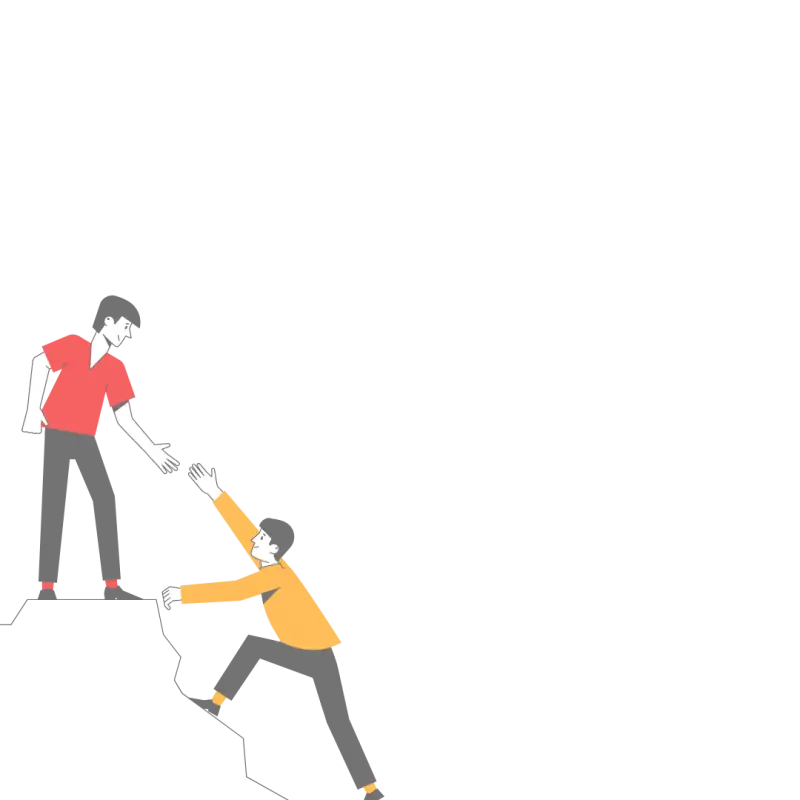
Thanks for your feedback!
Your contributions will help us to improve service.
How to URL Encode in Vue Js?
To encode URLs in Vue.js is a simple Method, we can use the built-in JavaScript function ‘encodeURIComponent()’ which is used to encode a URI component
Vue Js Encode URL | Example
xxxxxxxxxx
<div id="app">
<button @click="myFunction">Click me</button>
<p>Url: {{url}}</p>
<p>{{result}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
url:'https://fontawesomeicons.com/fa/vue-js-url-encode-decode?preview=1',
result:'',
}
},
methods:{
myFunction(){
this.result = 'Encoded URLs: ' + encodeURIComponent(this.url)
}
}
}).mount('#app')
</script>
Output of above example
How to Decode URLs in Vue.js ?
Decoding URLs in Vue.js is a fairly straightforward process, and can be accomplished using the decodeURIComponent() methods available in JavaScript
Vue Js Decode URL | Example
xxxxxxxxxx
<div id="app">
<button @click="myFunction">Click me</button>
<p>Encoded Url: {{url}}</p>
<p>{{result}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
url:'https%3A%2F%2Ffontawesomeicons.com%2Ffa%2Fvue-js-url-encode-decode%3Fpreview%3D1',
result:'',
}
},
methods:{
myFunction(){
this.result = 'Decoded URLs: ' + decodeURIComponent(this.url)
}
}
}).mount('#app')
</script>
Output of above example