Vue search text in Google on click
Vue search text in Google on click:To implement the "Vue search text in Google on click" functionality, you would create a search box and button using Vue.js template syntax. You would then create a method that would be triggered when the button is clicked, which would extract the text entered by the user and construct a Google search URL using JavaScript. This URL can be opened in a new browser tab using the window.open() method, which will perform the Google search with the entered text. Overall, this functionality involves creating a user interface with Vue.js and using JavaScript to handle user input and perform actions based on that input.
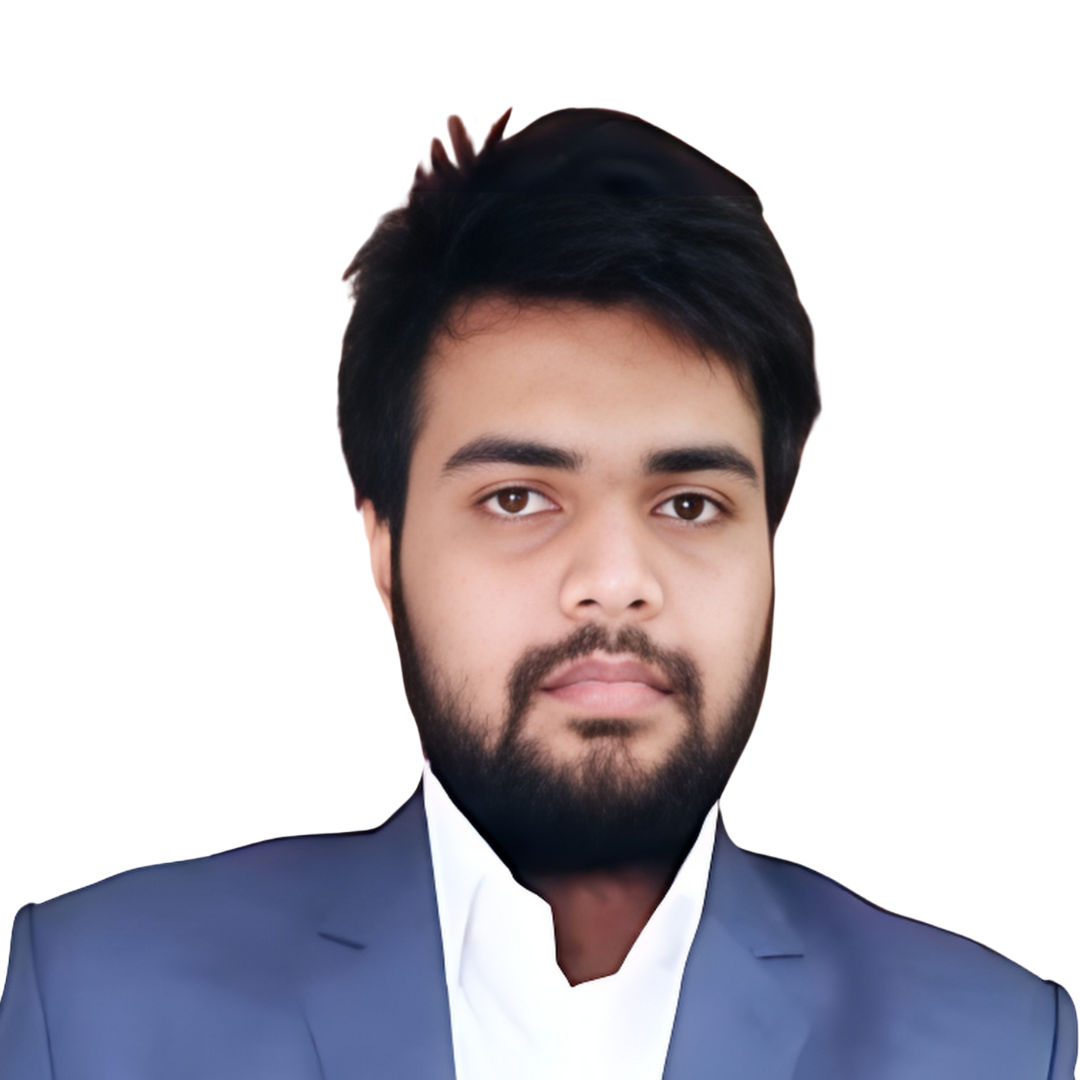
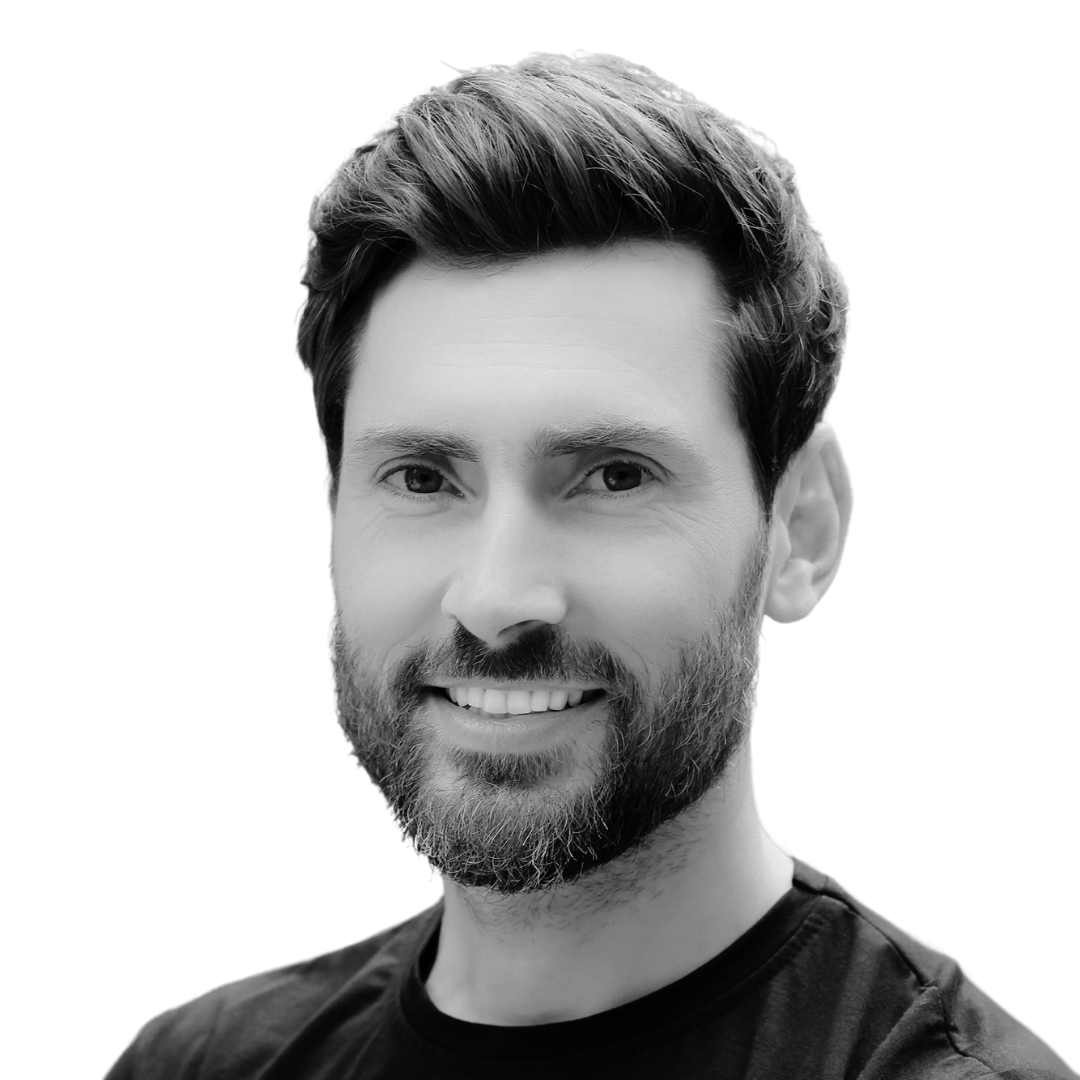
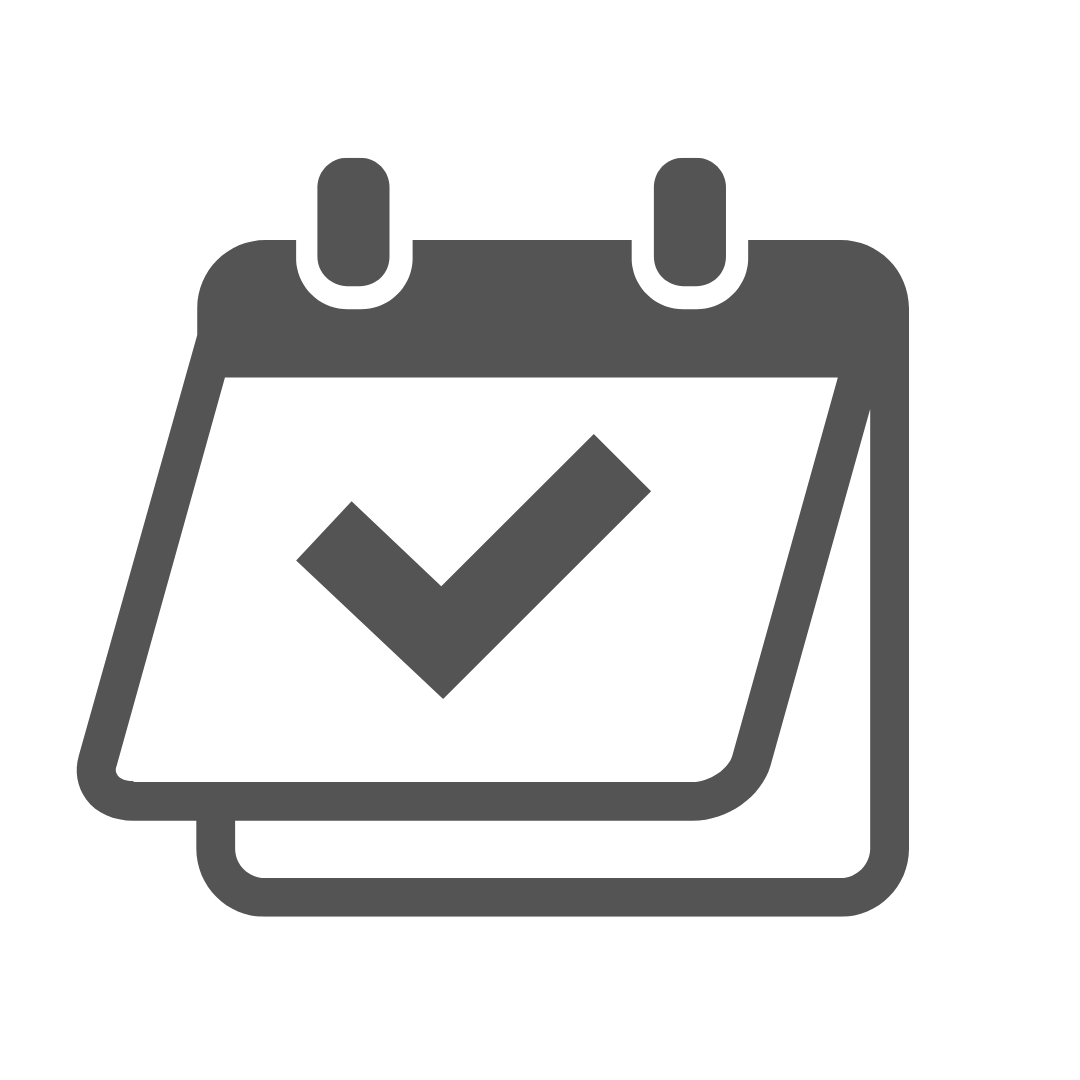
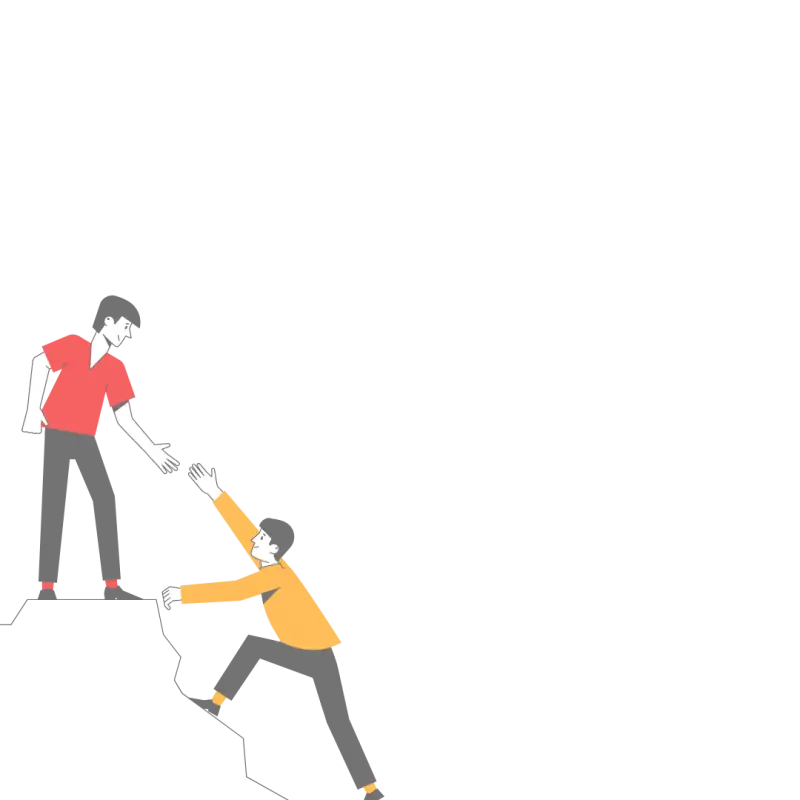
Thanks for your feedback!
Your contributions will help us to improve service.
How can I create a Vue component that allows the user to search a given text in Google when a button is clicked?
This code creates a Vue component with a form that allows the user to search Google when a button is clicked.
The input field is bound to a data property called "searchTerm" using v-model. When the form is submitted, the searchGoogle() method is called.
This method constructs a URL based on the user's search term and opens it in a new browser tab using the window.open() method