Vue Js Set Cookie
Vue Js Set Cookie:Vue itself does not have a built-in way to set cookies, but you can use JavaScript's document.cookie
property to set cookies in your Vue application. Cookies are small pieces of data stored on a user's browser that can be used to remember user preferences, login information, and other stateful data. To set a cookie in Vue, you can create a function that takes a cookie name, value, and optional expiration date, and then use document.cookie
to set the cookie with those values.
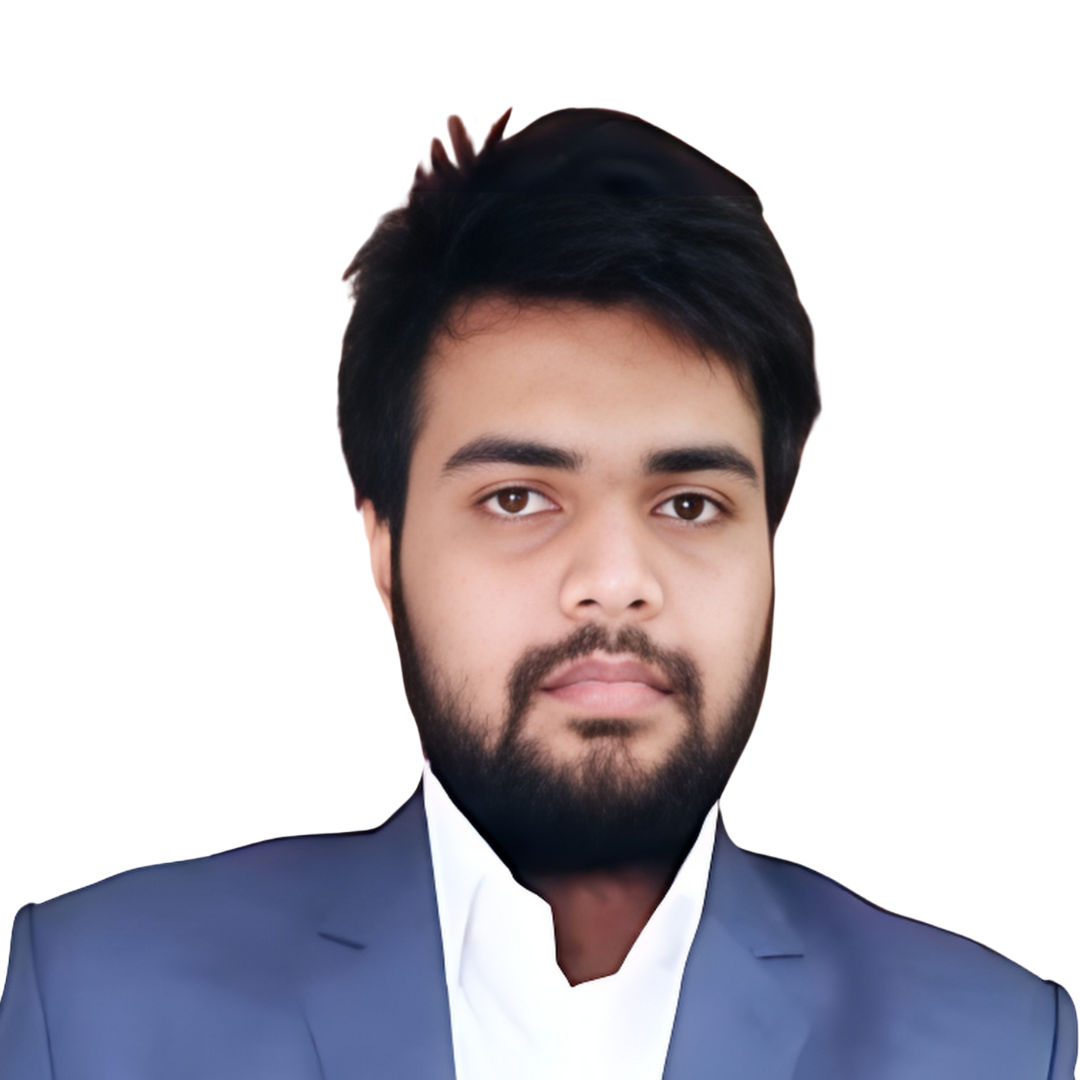
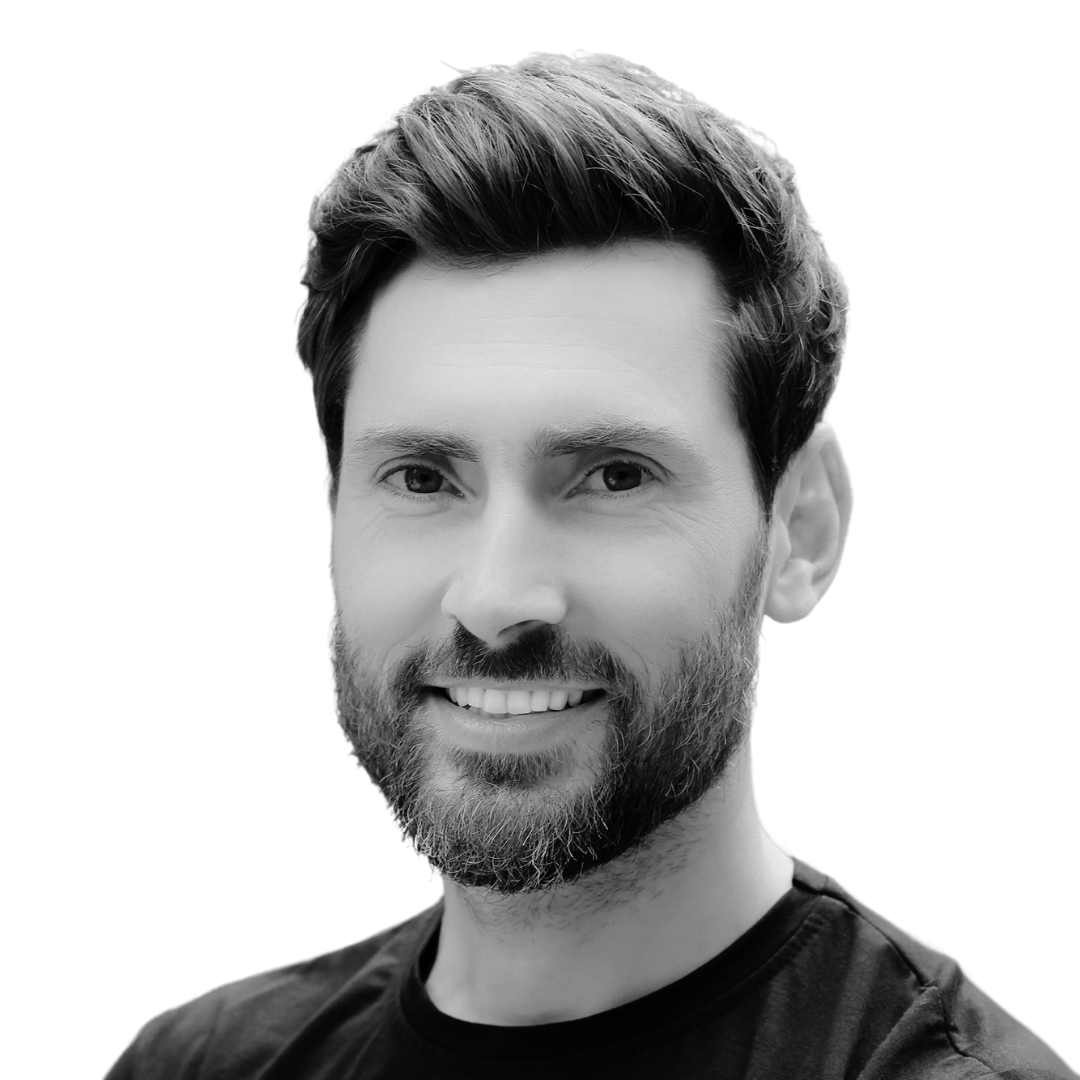
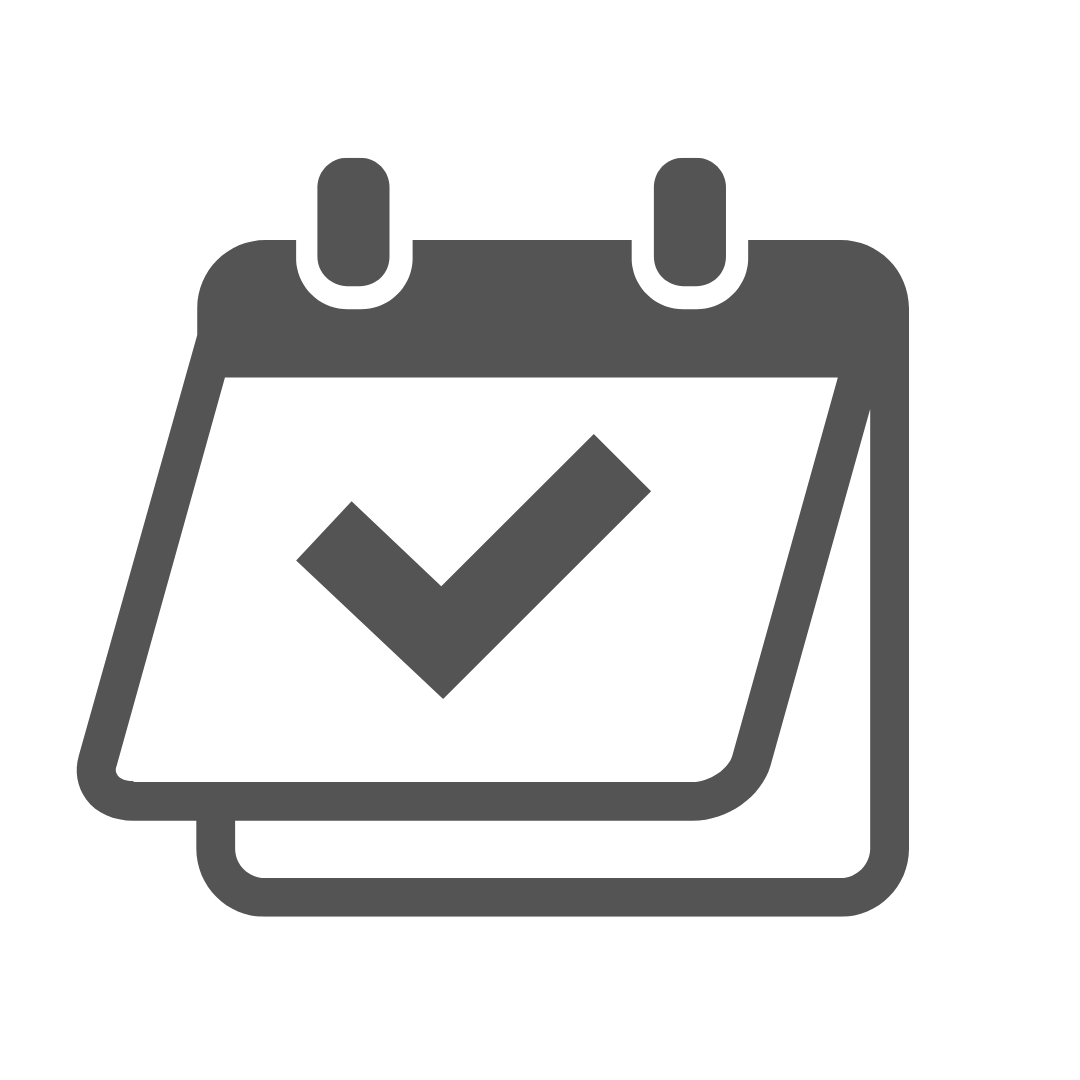
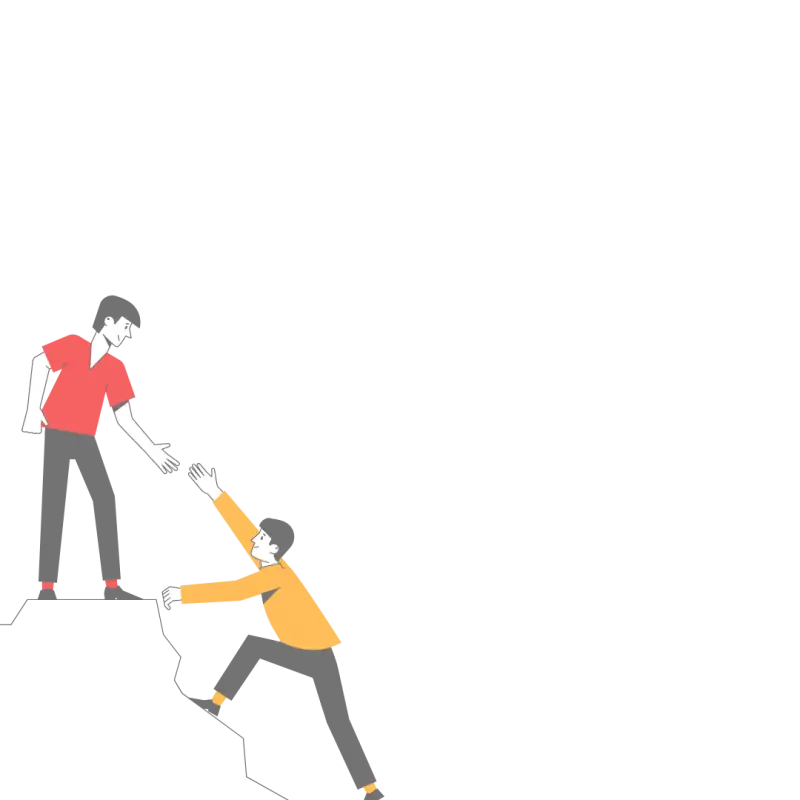
Thanks for your feedback!
Your contributions will help us to improve service.
How can cookies be set in Vue js?
This is a Vue.js code snippet that creates a button with an onclick event that triggers a method called "setMyData". When the button is clicked, the method prompts the user for a key, value, and an optional number of days to keep the cookie.
The "setCookie" method is called with the provided key, value, and days arguments. It sets a cookie with the given key, value, and expiration time using the document.cookie property. If an expiration time is provided, the method converts it to a UTC string and adds it to the cookie string with a path of "/".
Overall, this code allows the user to set a cookie with a key, value, and an optional expiration time in days using a button click event and Vue.js methods.