Vue Js Array copyWithin Function
Vue Js Array copyWithin Function : The copyWithin() method in Vue.js allows you to copy elements within an array and replace the previous values with the copied elements. It does not change the length of the array. This method can be used to efficiently manipulate arrays by copying and rearranging elements without the need for additional memory allocation or loop iteration. The tutorial provides step-by-step instructions on how to use this method in Vue.js and also offers an online editor for users to edit and run the code directly.
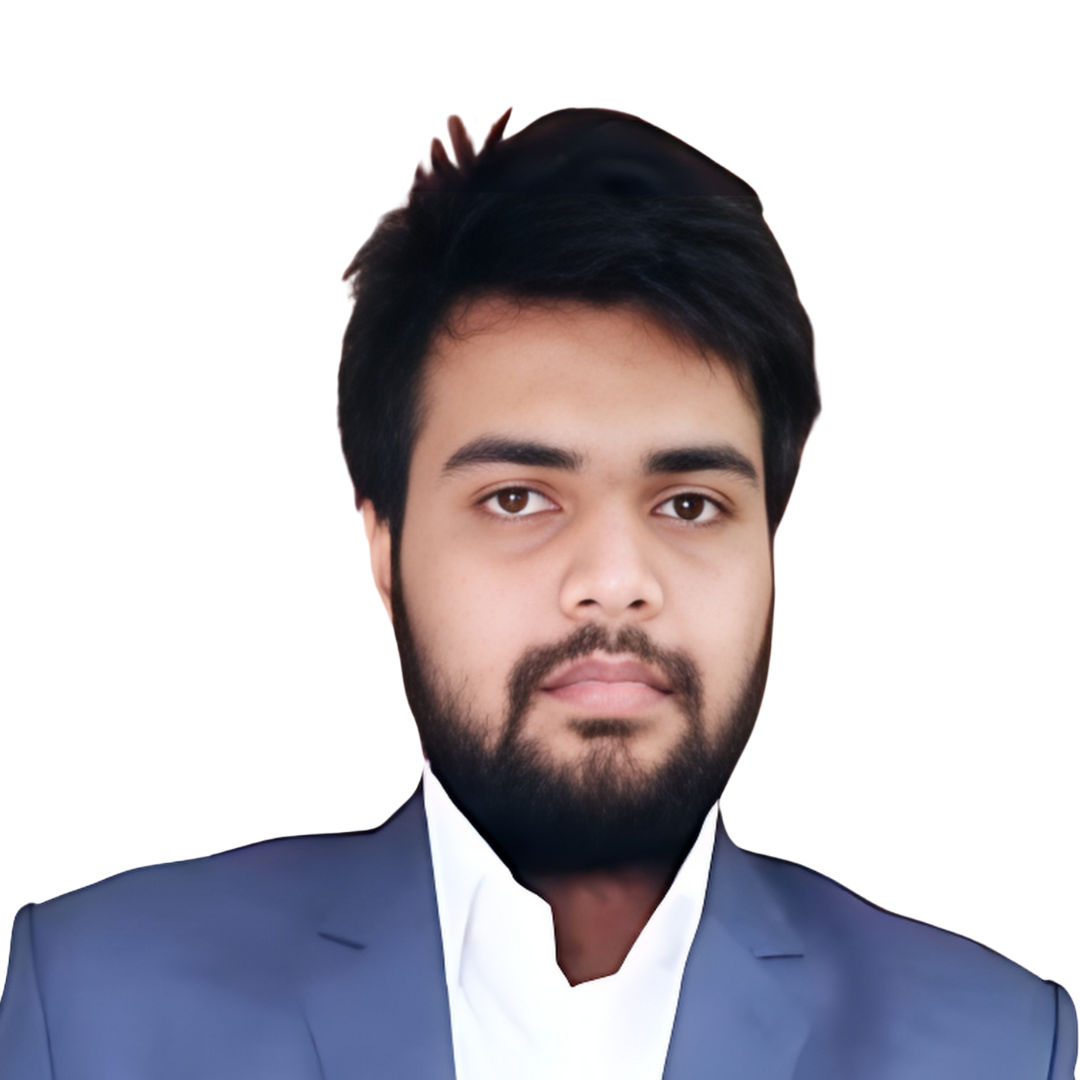
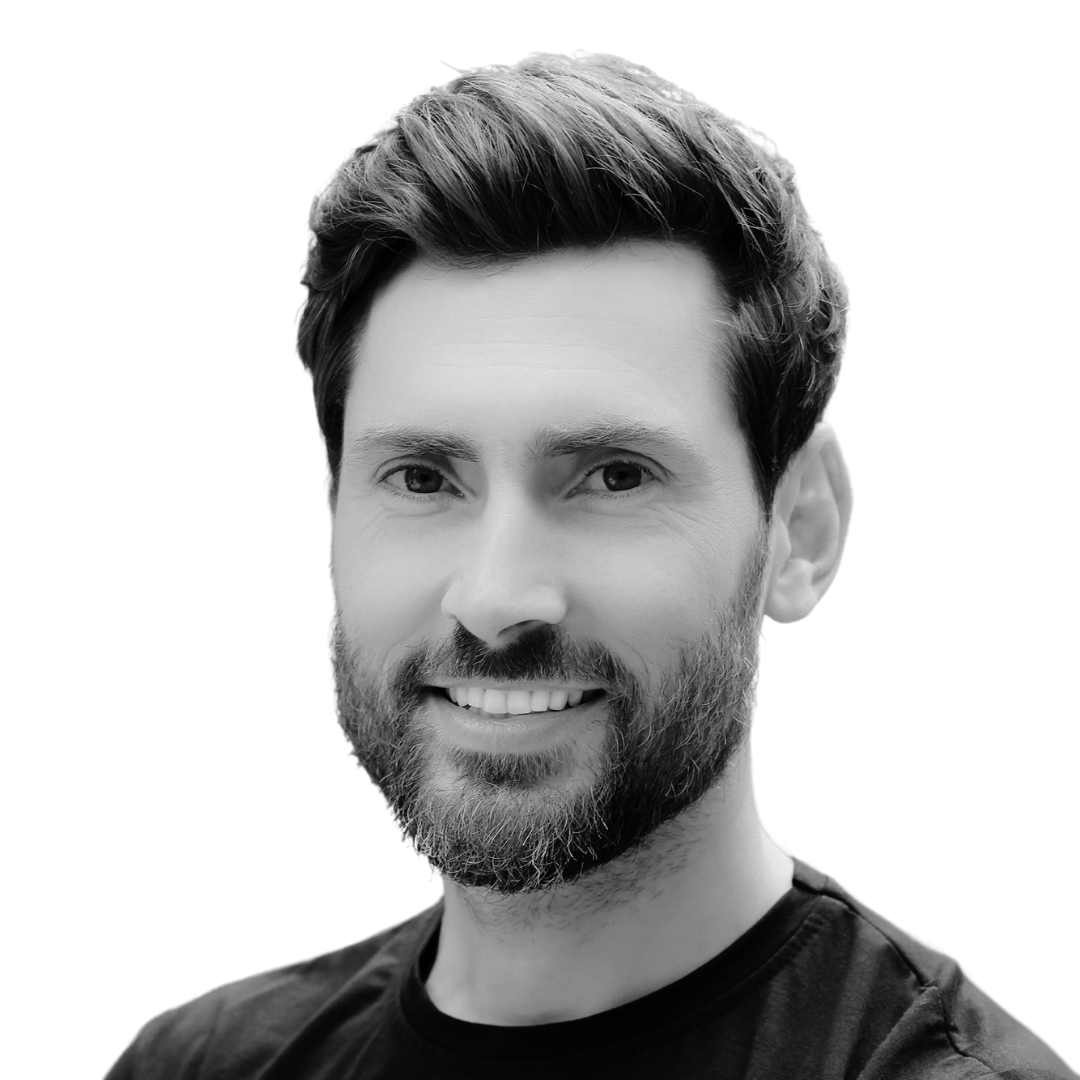
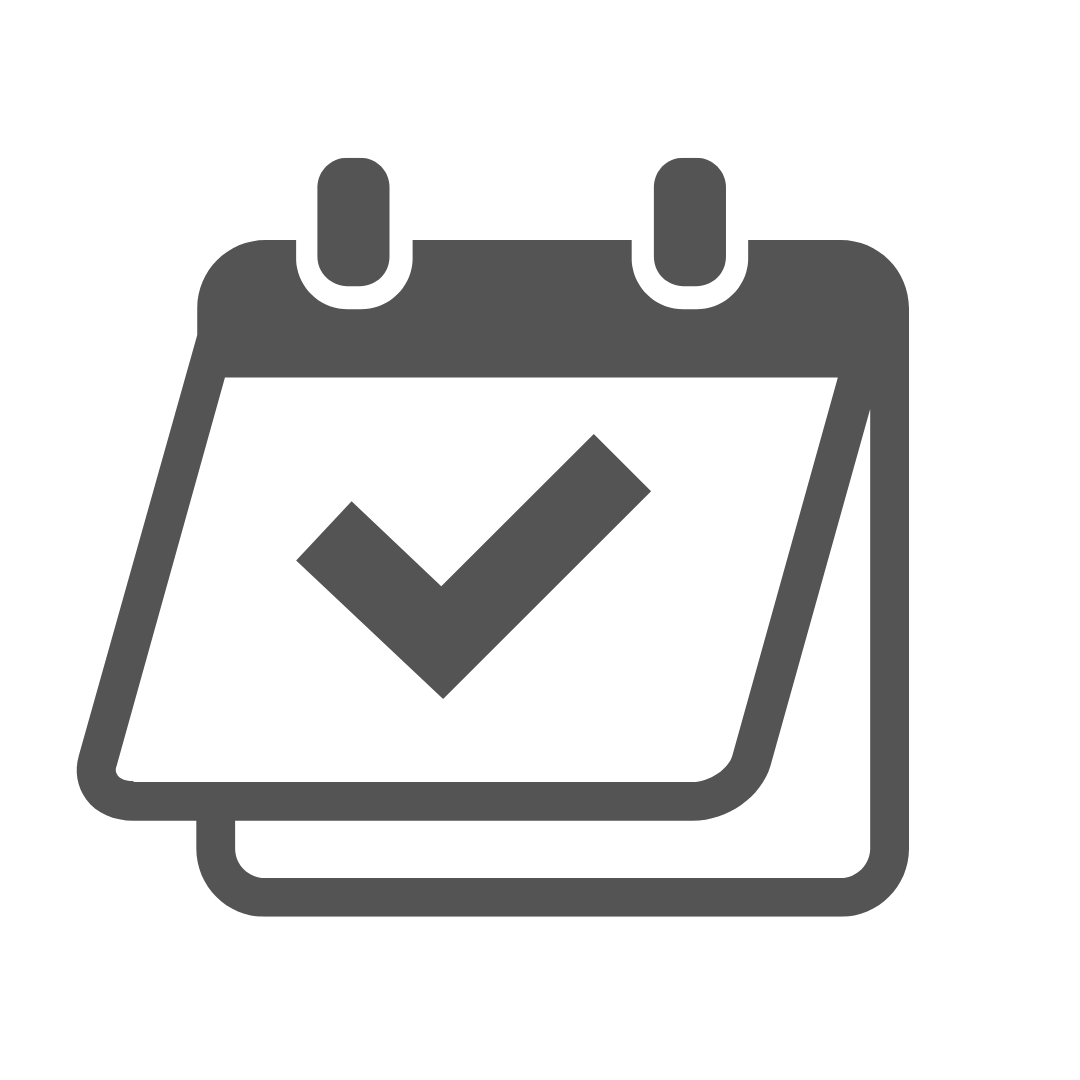
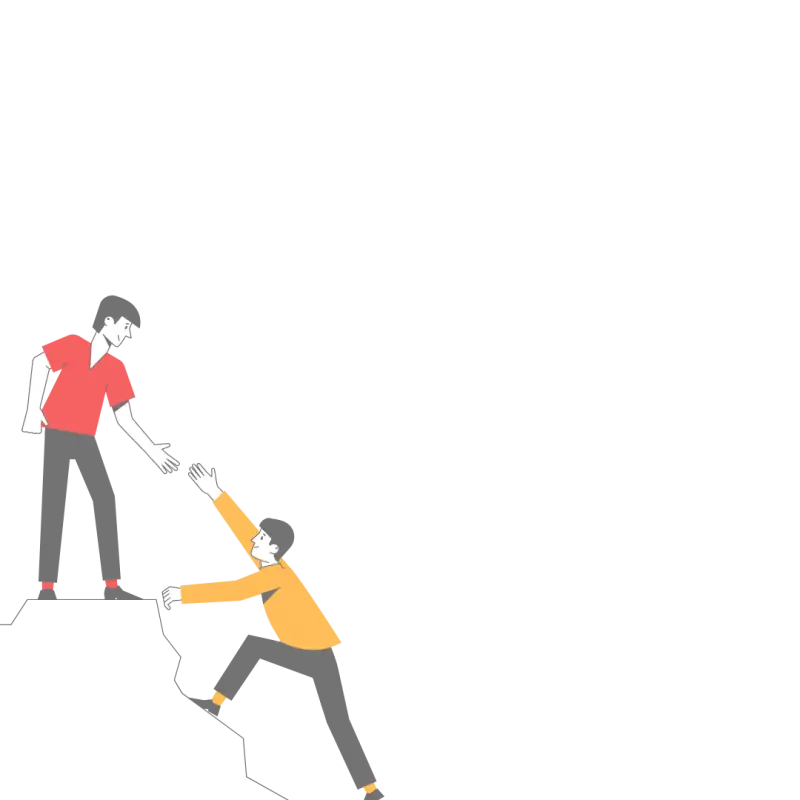
Thanks for your feedback!
Your contributions will help us to improve service.
Copying Final Two Array Elements to First Two Elements in Vue Js
This code block is written in JavaScript and uses the Vue.js library to create a reactive application. The application defines a data property called number
which is an array of numbers from 1 to 6.
The copyWithin()
method is called on the number
array within a method called copywithin()
. The copyWithin()
method is used to copy a sequence of array elements within the same array, and returns the modified array.
The copyWithin()
method takes three arguments:
- The index at which to start copying elements to. In this case, it is 0.
- The index at which to start copying elements from. In this case, it is 4.
- The index at which to stop copying elements from. In this case, it is 6.
The result of the copyWithin()
method is to replace the elements at indices 0, 1, and 2 in the number
array with the elements at indices 4 and 5, resulting in the array [5, 6, 3, 4, 5, 6].
The createApp()
method is used to create a new Vue application instance and pass in an object with two properties:
data()
, which returns an object containing thenumber
array.methods
, which contains thecopywithin()
method.
The mount()
method is then used to mount the Vue instance to a DOM element with the ID app
. This will make the application reactive, allowing it to update the DOM whenever the number
array is modified.
Using the Array copyWithin Method in Vue.js: A Practical Example
<div id="app" class="container">
<P>Number : {{number}}</P>
<button @click='copywithin' class='btn btn-primary'>Copy Array</button>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
number : ['1','2','3','4','5','6'],
}
},
methods:{
copywithin(){
this.number.copyWithin(0,4,6);
}
}
}).mount('#app')
</script>
Output of above example will look something like this -
How can you transfer the first two elements of an array to positions 4 and 5 in Vue Js?
This is a Vue.js app that displays a list of cities and has a button to copy an element within the array. The cities are stored in an array called "city" within the app's data object.
When the button is clicked, the "copywithin" method is called, which uses the built-in "copyWithin" method of arrays to copy the first two elements of the array to the third position.
This updates the "city" array, and because it's reactive, Vue.js automatically updates the displayed list of cities.
Vue js coywithin method | Example 2
<div id="app" class="container">
<P>City : {{city}}</P>
<button @click='copywithin' class='btn btn-primary'>Copy Array</button>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
city : ['Delhi','Mumbai','Kolkata','Pune','Noida','Bihar'],
}
},
methods:{
copywithin(){
this.city.copyWithin(3,0,2);
}
}
}).mount('#app')
</script>
Output of above example will look something like this -