Vue Js Get Access DOM Element
Vue Js Get Access DOM Element:In Vue.js, to access a DOM element, you can use the ref
attribute along with the $refs
object. First, assign a ref
to the desired element in your Vue template. Then, in the corresponding Vue component, you can access the DOM element using this.$refs
followed by the assigned ref
name. For example, if you have <div ref="myElement">...</div>
, you can access it using this.$refs.myElement
. This allows you to manipulate or retrieve properties of the DOM element programmatically within the Vue component's methods or lifecycle hooks
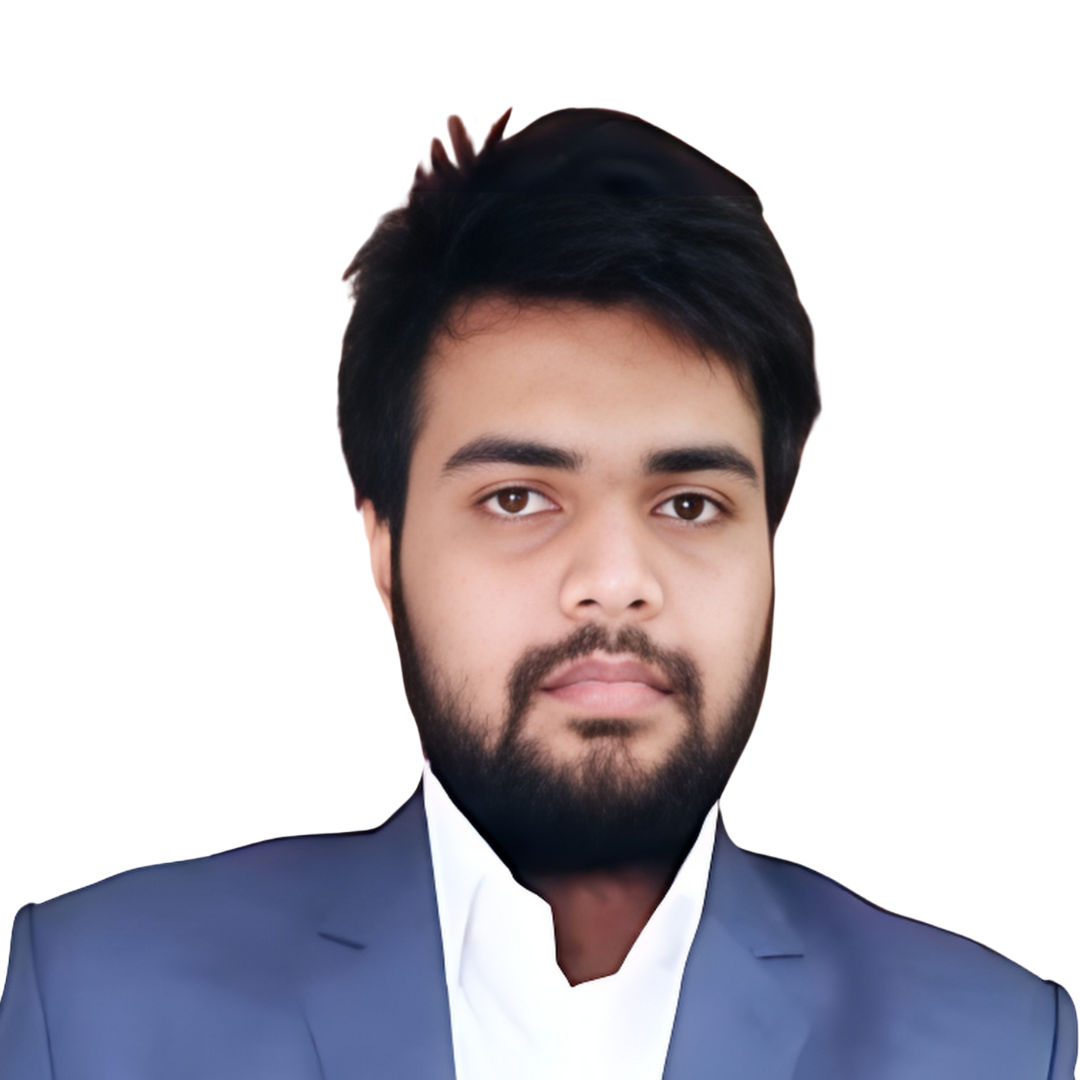
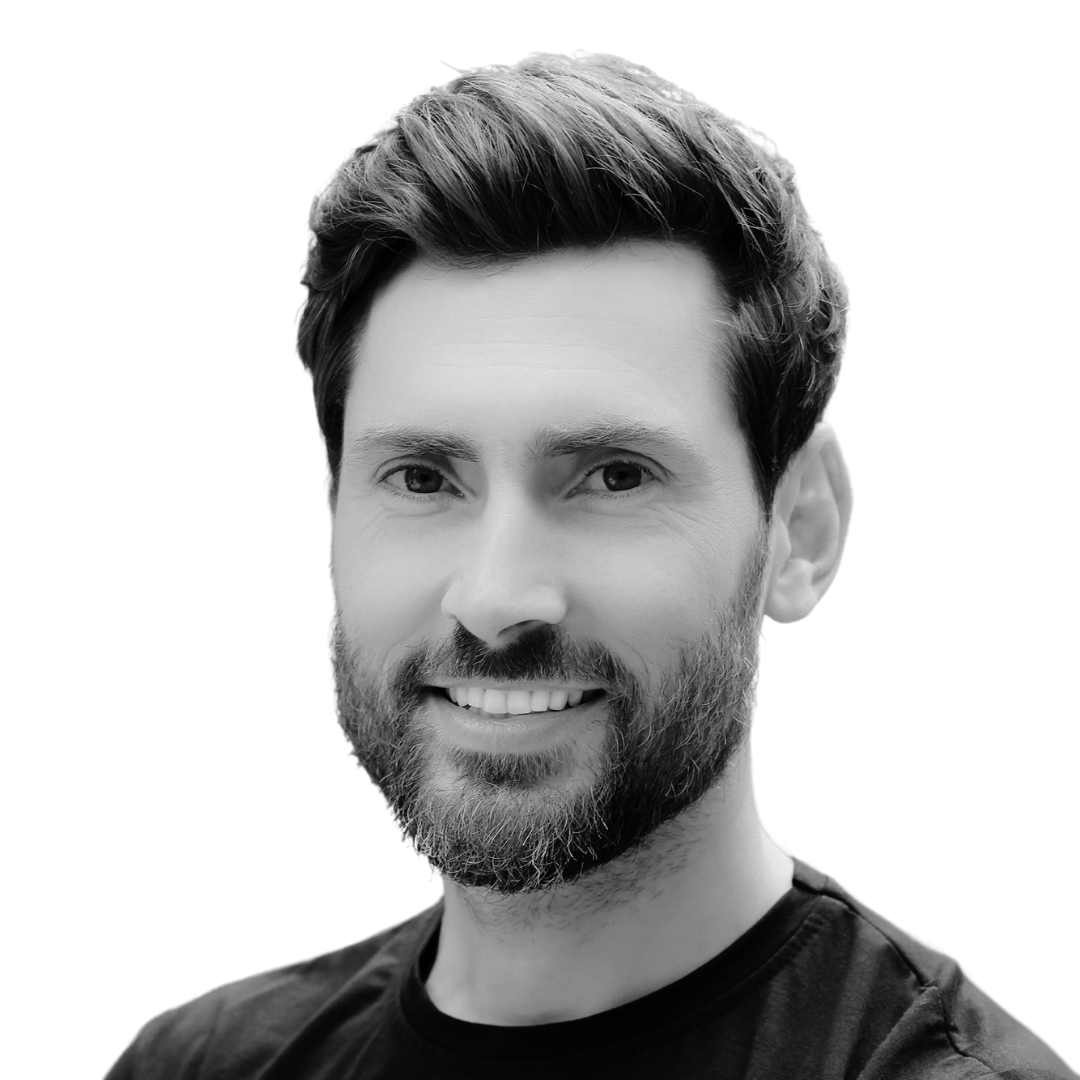
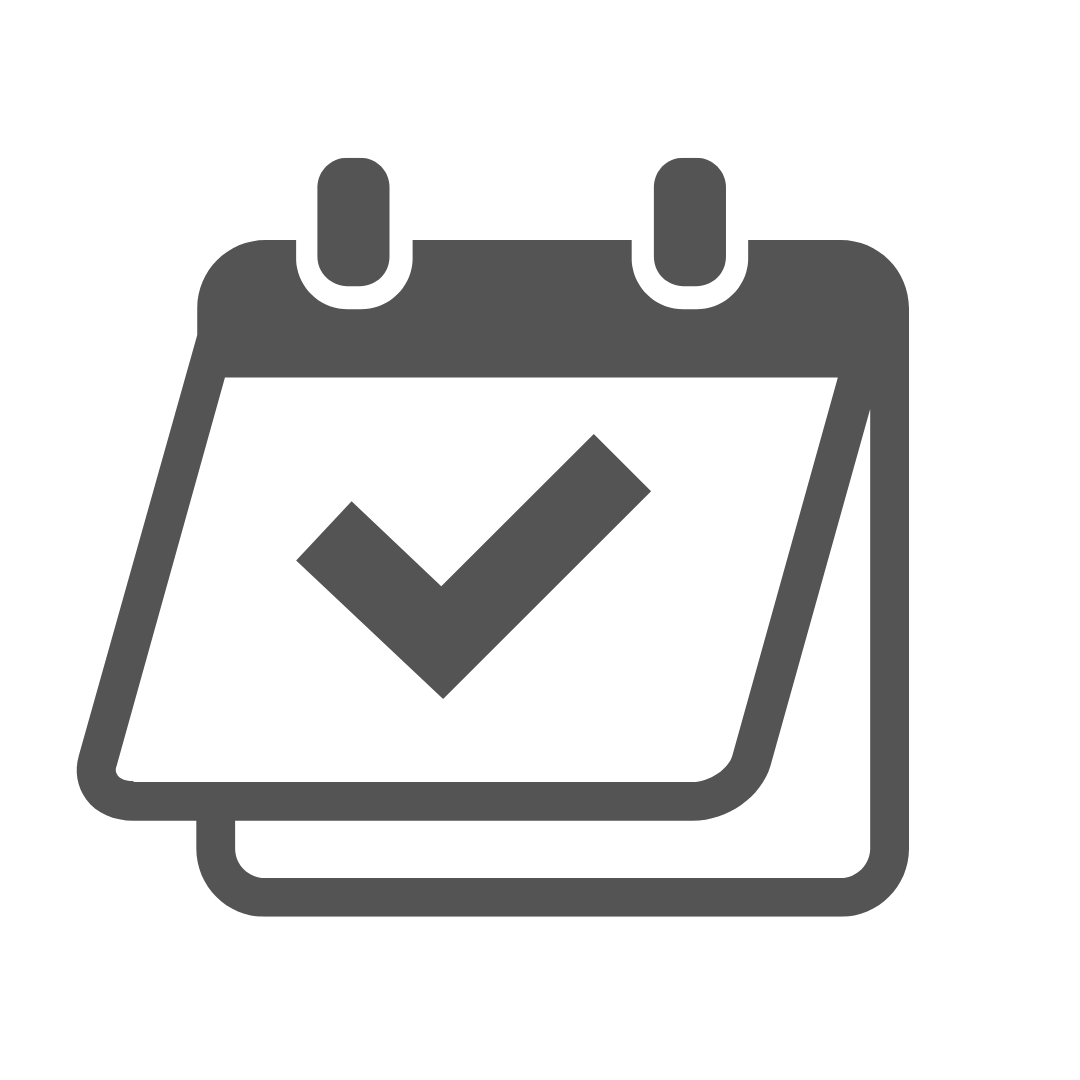
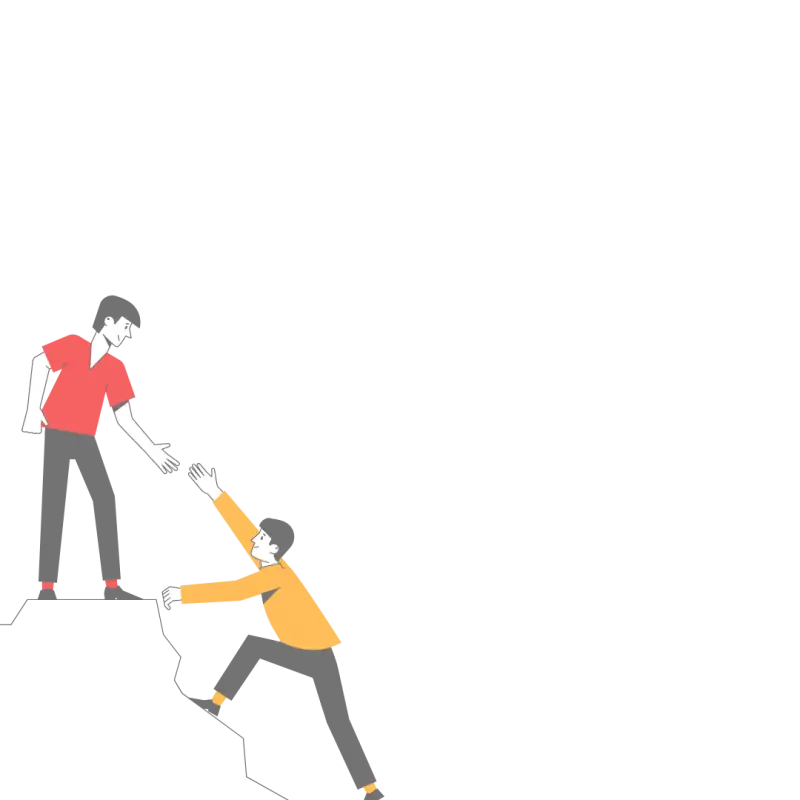
Thanks for your feedback!
Your contributions will help us to improve service.
How can I access a DOM element in Vue js?
In the Below code snippet, the Vue.js component is using the $refs
property to access a DOM element. The ref
attribute is assigned to the button element as ref="myButton"
. Here's an explanation of how it works:
- The
ref
attribute is used to give a reference name to a particular element within the component template. In this case, the reference name is "myButton". - Inside the Vue instance's
methods
object, there is a method calledhandleClick()
. This method is triggered when the button is clicked, as indicated by the@click="handleClick"
event listener on the button element. - Inside the
handleClick()
method,this.$refs.myButton
is used to access the DOM element with the reference name "myButton". It retrieves the DOM element associated with that reference. - Once the DOM element is obtained, you can perform operations on it. In this example,
buttonElement.innerText = 'Clicked!'
is used to change the text content of the button to "Clicked!".
By using the $refs
property, you can access and manipulate DOM elements within a Vue.js componen
Vue Js Get Access DOM Element Example
xxxxxxxxxx
<div id="app">
<button ref="myButton" @click="handleClick">Click me</button>
</div>
<script type="module">
const app = new Vue({
el: "#app",
methods: {
handleClick() {
// Access the DOM element using $refs
const buttonElement = this.$refs.myButton;
// Perform operations on the DOM element
buttonElement.innerText = 'Clicked!';
}
}
});
</script>