Vue Js Convert Degree into Radian
Vue.js is a JavaScript framework that can be used to create dynamic and responsive user interfaces. It is commonly used for web development.
To convert a decimal value to radians, you can use the formula: radians = degrees * (Math.PI/180)
. In Vue.js, you can implement this formula in a method or computed property to convert decimal values to radians.
To convert radians to decimal, you can use the formula: degrees = radians * (180/Math.PI)
. This can also be implemented in a method or computed property in Vue.js.
In summary, Vue.js can be used to convert decimal values to radians and radians to decimal using simple mathematical formulas.
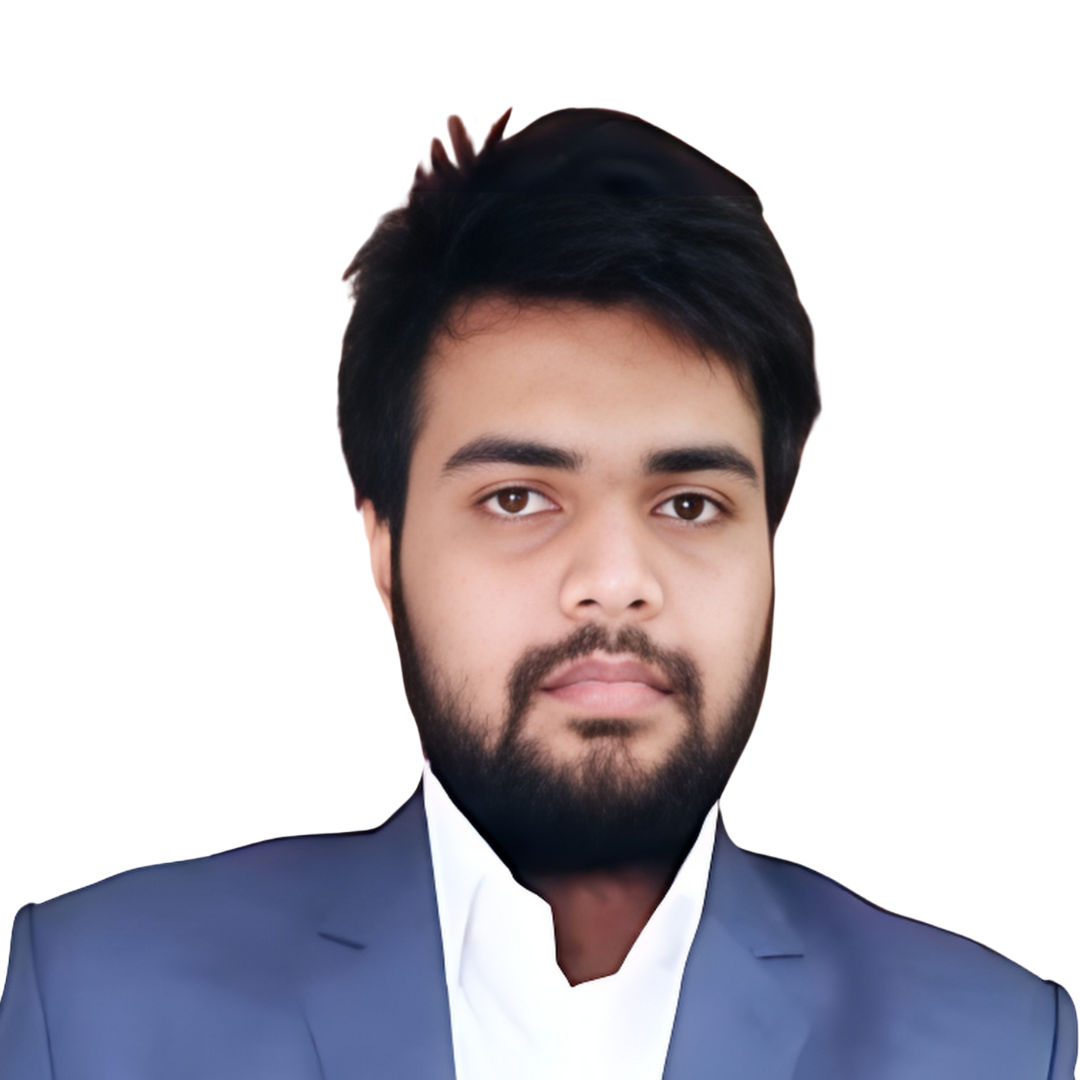
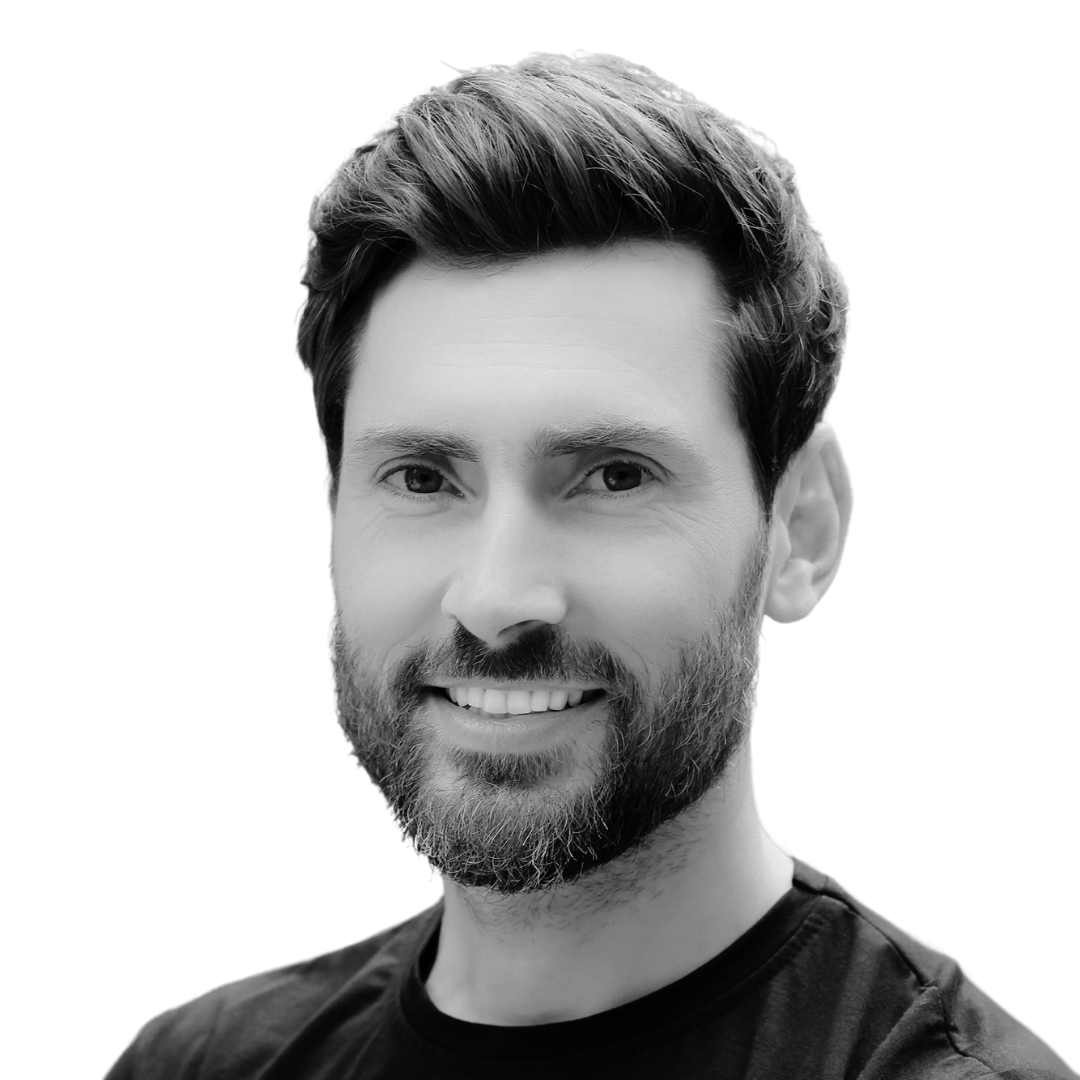
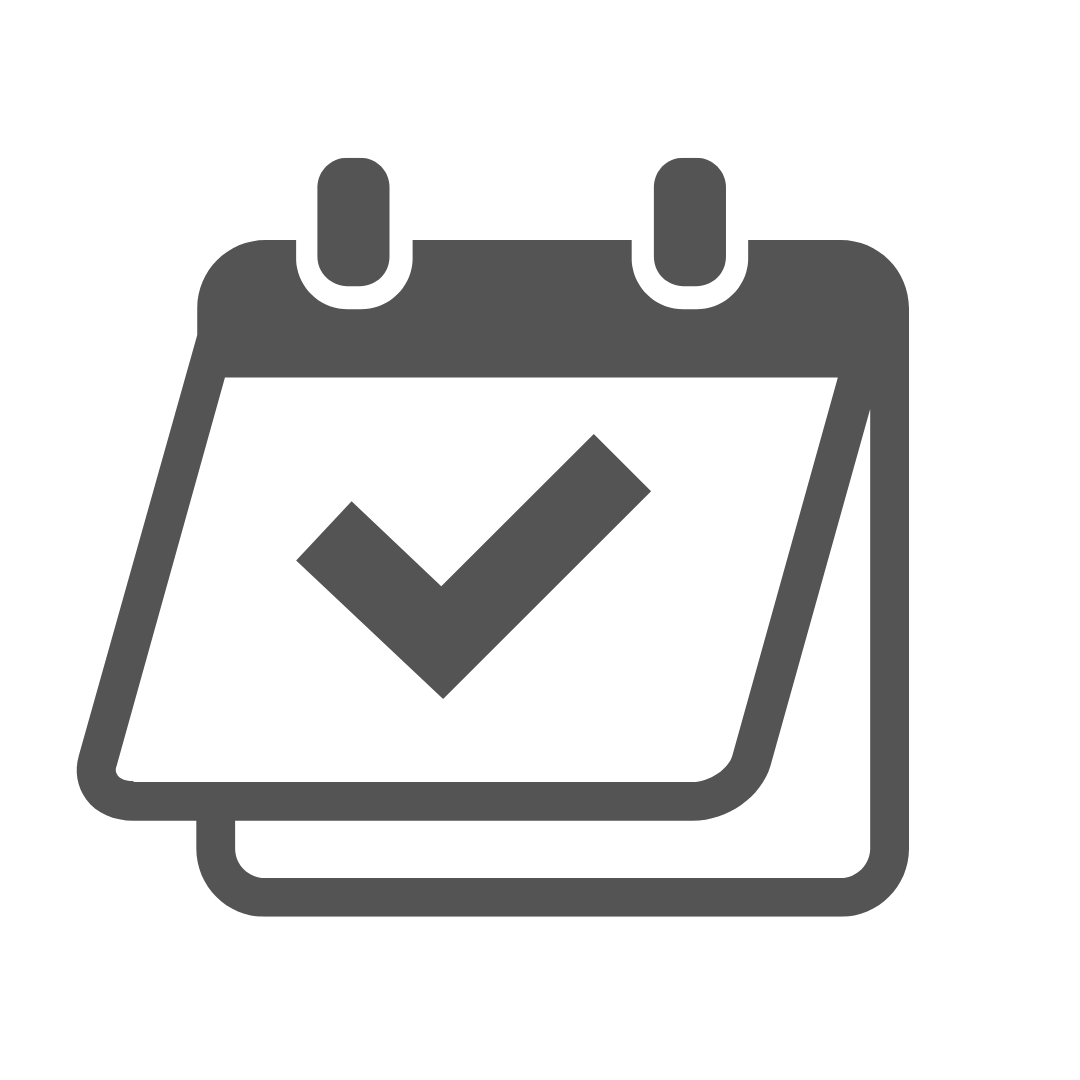
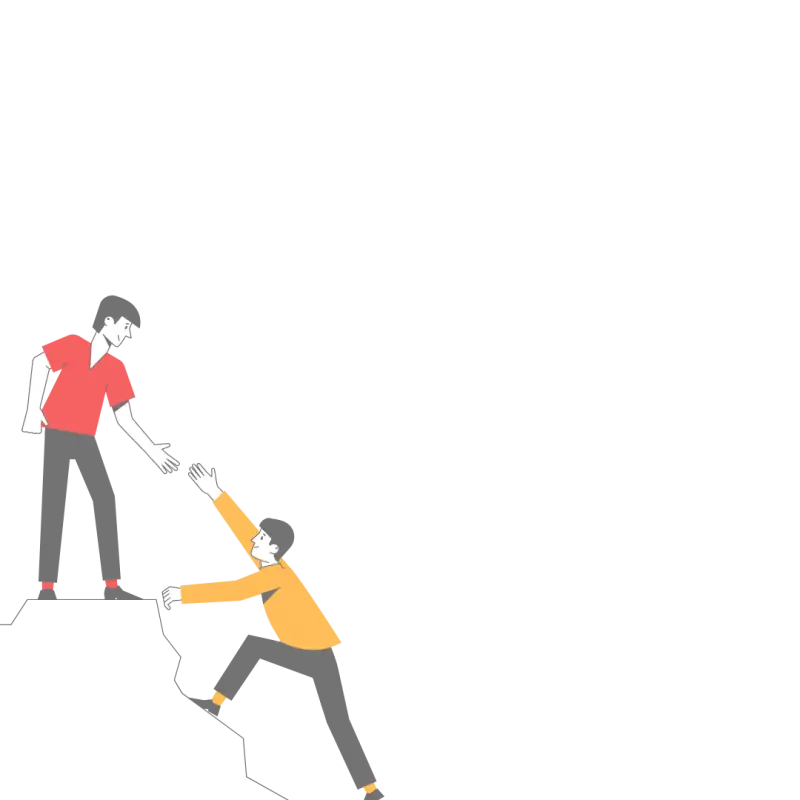
Thanks for your feedback!
Your contributions will help us to improve service.
How to Convert Degree into Radian in Vue Js
-
The HTML code defines a form with an input field and a label. The label is associated with the input field using the "for" attribute.
-
The input field has an ID of "degrees" and a type of "number". The v-model directive is used to bind the value of the input field to the "degrees" property of the Vue instance.
-
The Vue instance has a "data" object that contains the initial state of the application. In this case, it sets the initial value of the "degrees" property to 45.
-
The Vue instance has a "computed" property that calculates the value of the "radians" property based on the value of the "degrees" property. The "computed" property is a function that returns the result of multiplying the "degrees" property by (Math.PI / 180), which converts degrees to radians.
- When the user enters a value in the input field, it updates the value of the "degrees" property of the Vue instance.
-
The "radians" property is automatically updated based on the new value of the "degrees" property, thanks to the "computed" property. The value of the "radians" property is displayed in the paragraph element using double curly braces and the "radians" property name.
Vue Js output of above example
How to Convert Radian into Degree in Vue Js
This code is written in JavaScript and uses the Vue.js library to create a web application that converts radians to degrees.
The Vue app is created using the Vue.createApp()
method, which takes an object with configuration options as an argument. The object has a data()
method which returns an object containing the initial data for the app. In this case, the radians
property is set to 1.
The app also has a computed
property called degrees
which uses the value of radians
and the conversion formula (180 / Math.PI)
to calculate the equivalent value in degrees. The computed property is used to update the value of degrees whenever the value of radians changes.