Vue Js Tutorial

1. Introduction to Vue js
What is Vue Js?
Vue js, often referred to simply as Vue, is an open-source JavaScript framework for building user interfaces (UIs) and single-page applications. It was created by Evan You and was first released in 2014. Vue.js is designed to be a progressive framework, meaning that you can use as much or as little of it as you need in your web projects. It is commonly used for building dynamic, interactive web applications.
Why use Vue js?
Vue js is a popular JavaScript framework for building user interfaces and single-page applications. There are several reasons why developers choose to use Vue.js:
-
Progressive Framework: Vue is often referred to as a "progressive framework" because you can use as much or as little of it as you need. You can integrate it into an existing project or use it to build an entire application from scratch.
-
Declarative Rendering: Vue.js uses a template-based syntax that allows you to declaratively render data to the DOM. This makes it easy to understand how your application's UI corresponds to the underlying data.
-
Reactive Data Binding: Vue.js uses a reactive data-binding system. When your data changes, the DOM updates automatically. This eliminates the need for manual manipulation of the DOM, making your code more concise and less error-prone.
-
Component-Based Architecture: Vue encourages you to build your application as a collection of reusable and composable components. This modularity makes it easier to manage and maintain your code, as well as collaborate with other developers.
-
Vue CLI: Vue comes with a command-line tool (Vue CLI) that simplifies project setup, development, and testing. It offers a variety of project templates and a plugin system for extending functionality.
-
Community and Ecosystem: Vue.js has a strong and growing community, which means there are a wealth of resources, plugins, and third-party libraries available to extend Vue's capabilities. The ecosystem includes tools for state management (Vuex), routing (Vue Router), and server-side rendering (Nuxt.js).
-
Performance: Vue is known for its good performance. It has a virtual DOM that efficiently updates the real DOM when data changes, and it provides various optimization techniques to help with faster rendering.
-
Detailed Documentation: Vue.js has well-documented and easy-to-understand documentation, making it accessible to developers of all skill levels.
-
Versatility: Vue can be used for a wide range of projects, from simple interactive web pages to complex single-page applications. Its flexibility makes it a good choice for both small and large projects.
-
Integration: Vue is designed to be easy to integrate with other technologies and libraries. It can work with jQuery, other JavaScript frameworks, and even be used in combination with server-side technologies like Node.js.
-
Active Development: The Vue.js core team is actively developing the framework, which means it keeps up with modern web development trends and best practices
Key features and benefits of Vue Js
- Directives: Vue offers a set of built-in directives (e.g., v-if, v-for, v-bind) that simplify common tasks like conditional rendering, iterating over lists, and binding values to HTML attributes.
-
Two-Way Data Binding: Vue.js supports two-way data binding, which means changes to the model (data) automatically update the view and vice versa, making it easy to keep data and UI in sync.
-
Virtual DOM: Vue uses a virtual DOM to optimize rendering performance. It updates only the parts of the real DOM that have changed, reducing the overall rendering overhead.
-
Templates: Vue allows you to define your templates in HTML with Vue-specific syntax. This separation of concerns makes it easier to understand and maintain your code.
-
Vuex: Vuex is a state management library for Vue.js that helps manage application-wide state in a predictable and consistent way.
Setting up Vue.js involves several steps, including installation and setup, creating your first Vue.js project, and understanding the project structure and files. Here's a guide on how to do this:
Installation and Setup:
a. CDN (Content Delivery Network):
- You can include Vue.js directly in your HTML file using a CDN. Add the following script tag in your HTML file's
<head>
section:
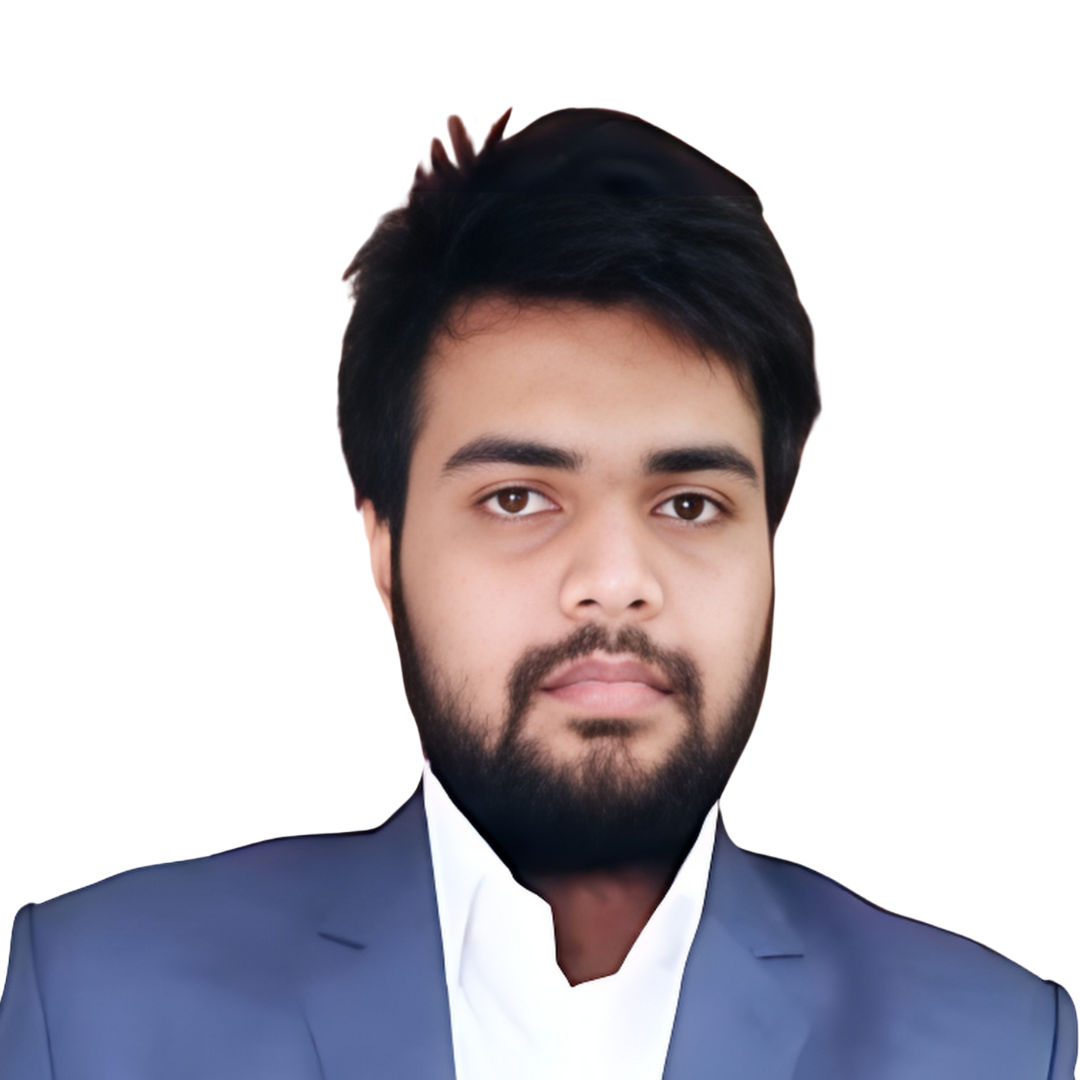
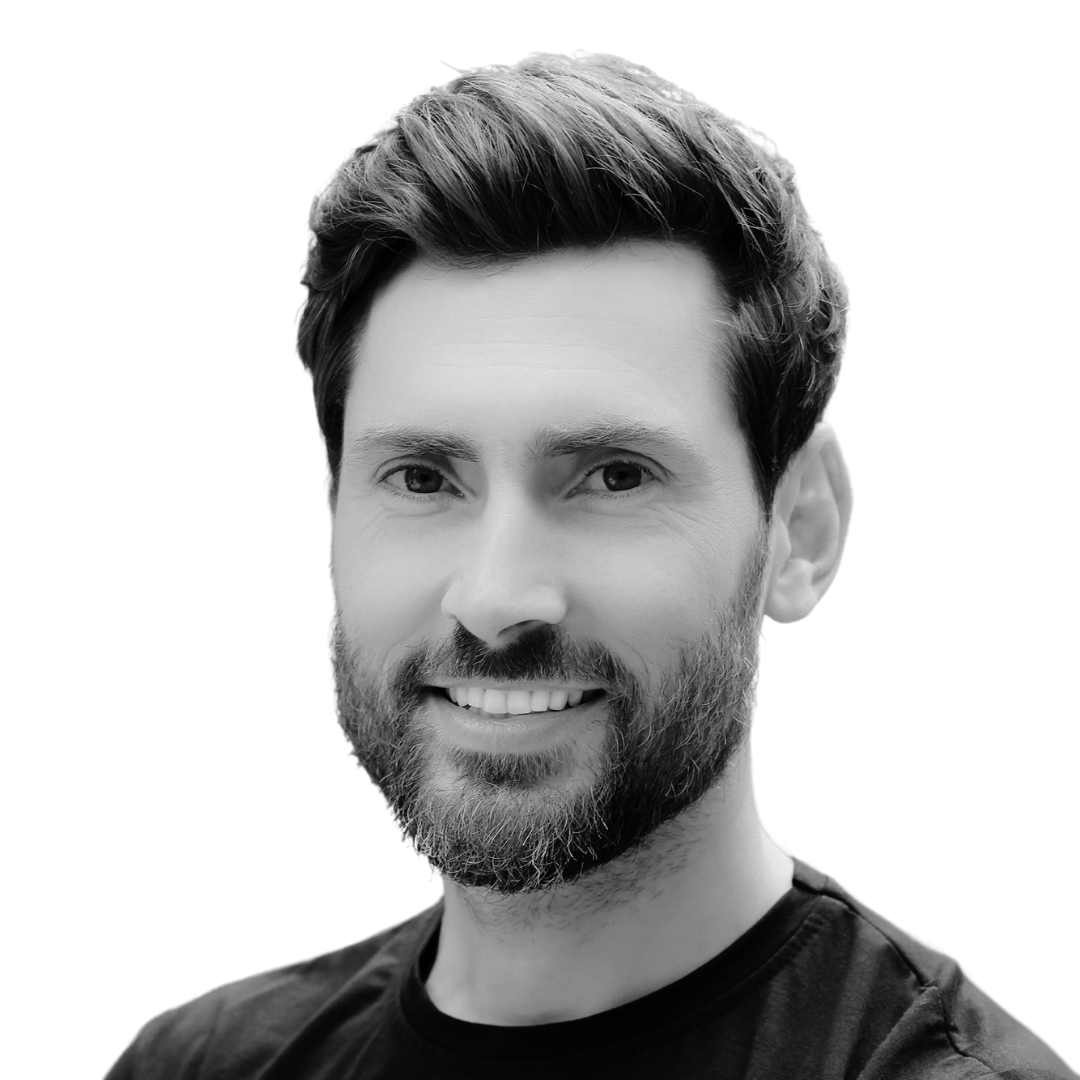
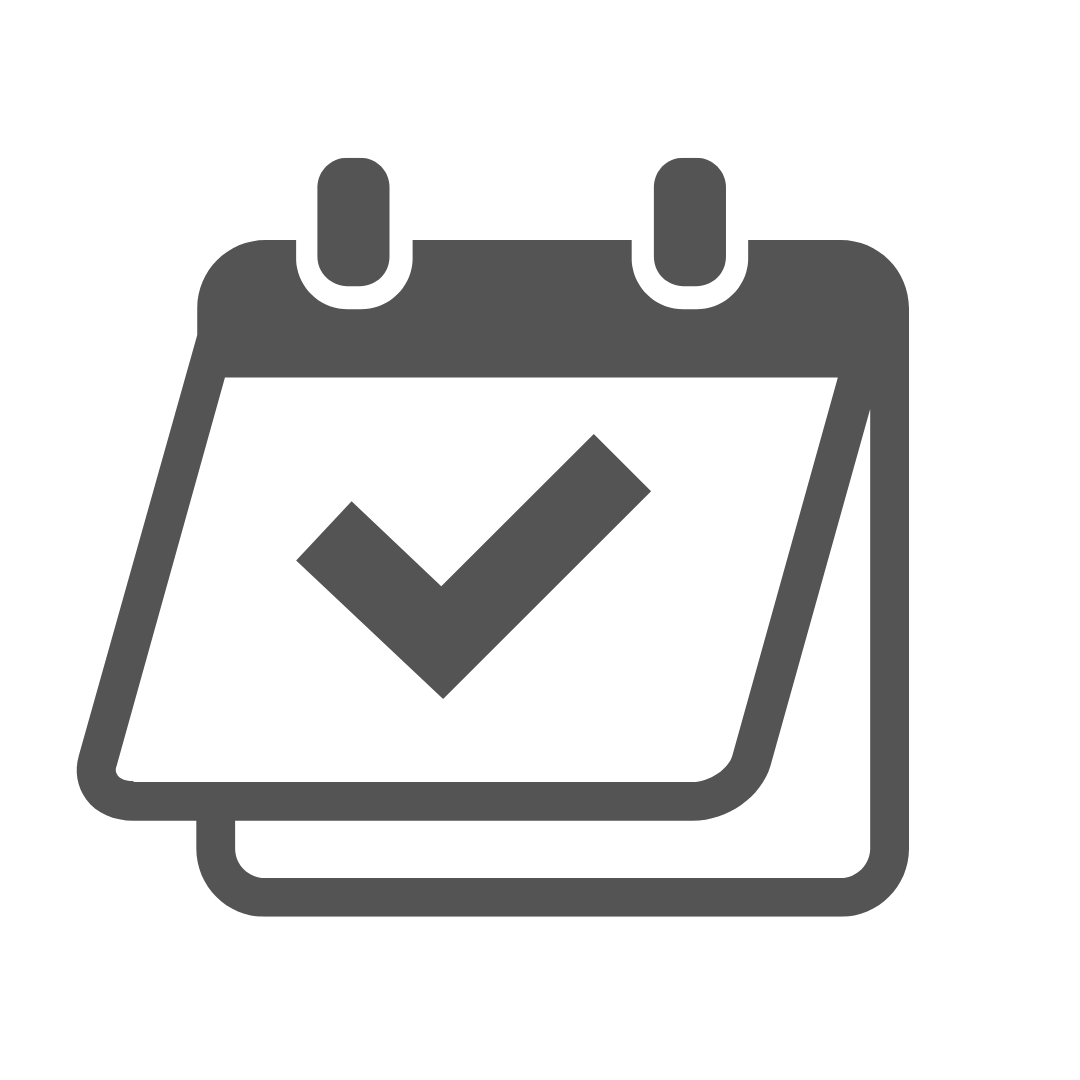
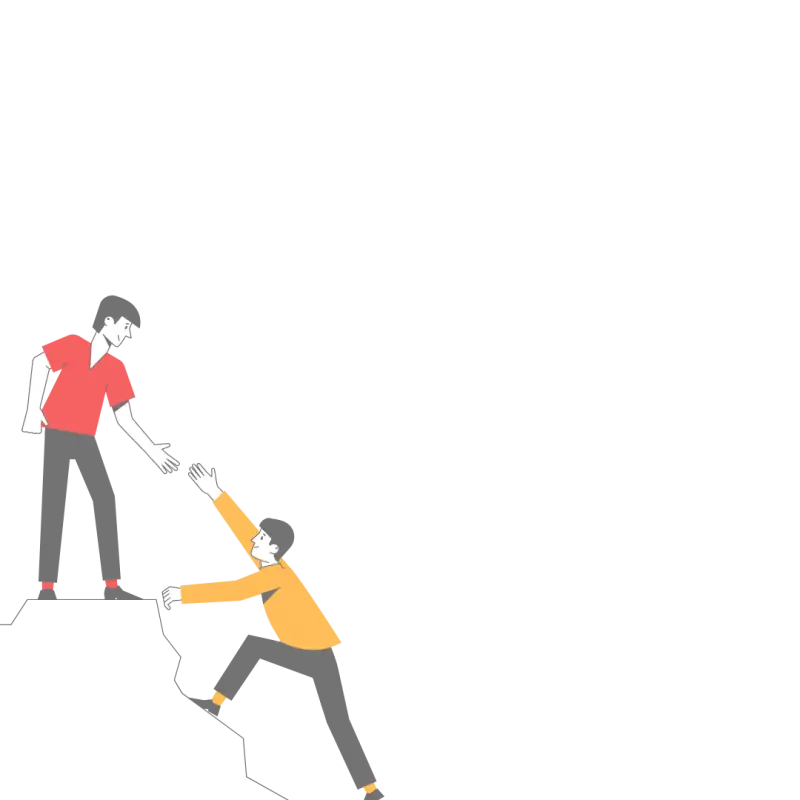
Thanks for your feedback!
Your contributions will help us to improve service.
Vue Js CDN
b. Vue CLI (Command Line Interface):
-
Vue CLI is the official tool for scaffolding and managing Vue.js projects. To use Vue CLI, you need to have Node.js and npm (Node Package Manager) installed on your system.
-
Install Vue CLI globally via npm:
The provided command initiates the installation and execution of create-vue
, Vue's official project scaffolding tool. This utility guides users through an interactive setup process, offering choices for optional functionalities like TypeScript and testing support. By running this command, you can easily set up a Vue project tailored to your preferences and requirements
When uncertain about an option, press Enter and select "No" for the time being. After creating the project, proceed with installing required dependencies and launching the development server following the provided instructions
Congratulations! Your inaugural Vue project is up and running. This achievement signifies that you've successfully created and executed your Vue.js application. You're now equipped to develop web applications using Vue's powerful framework. It's an exciting milestone, opening the door to building dynamic and interactive user interfaces with ease. From here, you can begin coding, designing, and customizing your project to suit your specific needs and goals. Enjoy your Vue journey!
-
Project Structure and Files:
a. If you used Vue CLI to create your project, it will have a predefined directory structure. Here are some of the important files and directories:
src/
: This directory contains your project's source code.public/
: Contains static files that are not processed by Webpack.node_modules/
: Where your project dependencies are stored.package.json
: Defines project metadata and dependencies.babel.config.js
: Babel configuration for transpiling code.webpack.config.js
: Webpack configuration (hidden by default in Vue CLI 3).
b. In the
src/
directory, you'll typically find the following important files:main.js
: The entry point for your application, where you create the Vue instance.App.vue
: The root Vue component, which can be composed of other components.components/
: Directory for your Vue components.
Understanding the project structure is essential for organizing and developing your Vue.js application efficiently.
That's a basic overview of how to set up Vue.js, create a project, and understand the project structure. You can now start building your Vue.js applications with this foundation
The command "npm run build" is a crucial step in the web development process. It's used in projects managed with npm (Node Package Manager) to create a production-ready build of the application. When executed, it triggers a series of tasks defined in the project's package.json file, typically involving tasks like code minification, asset optimization, and bundling. The end result is a compressed and optimized version of your web application, ideal for deployment. This command ensures that the code is efficient and small in size, improving website performance and reducing load times when users access your site.
b. Manually (Without Vue CLI):
- You can also create a Vue.js project manually by adding Vue.js to an HTML file. Here's a basic example:
Here are a few examples of Vue.js tutorials.
☞ Vue Js Get Element Height and Width
☞ Vue Js Automatic Refresh Page
☞ Vue Js Format Date Example | DD/MM/YYYY
☞ Vue Js Allow Only Number in Input Field
☞ Vue Js Convert String to HTMl | v-html
☞ Vue Js Refresh Page onclick Button | Reload
☞ Vue Js Modifiy Url without Reloading Page
☞ Vue Js Print Page | window.print