Vue Js Convert Decimal to Binary
Vue Js Convert Decimal to Binary: you can convert a decimal number into binary using the built-in toString()
method in Vue.js. The toString()
method is used to convert a number into a string, and it takes an optional parameter called radix
that specifies the base of the number system to use for the conversion.
To convert a decimal number into binary, you can call the toString()
method on the decimal number and pass the radix
parameter with a value of 2. This tells the method to use the binary system for the conversion.
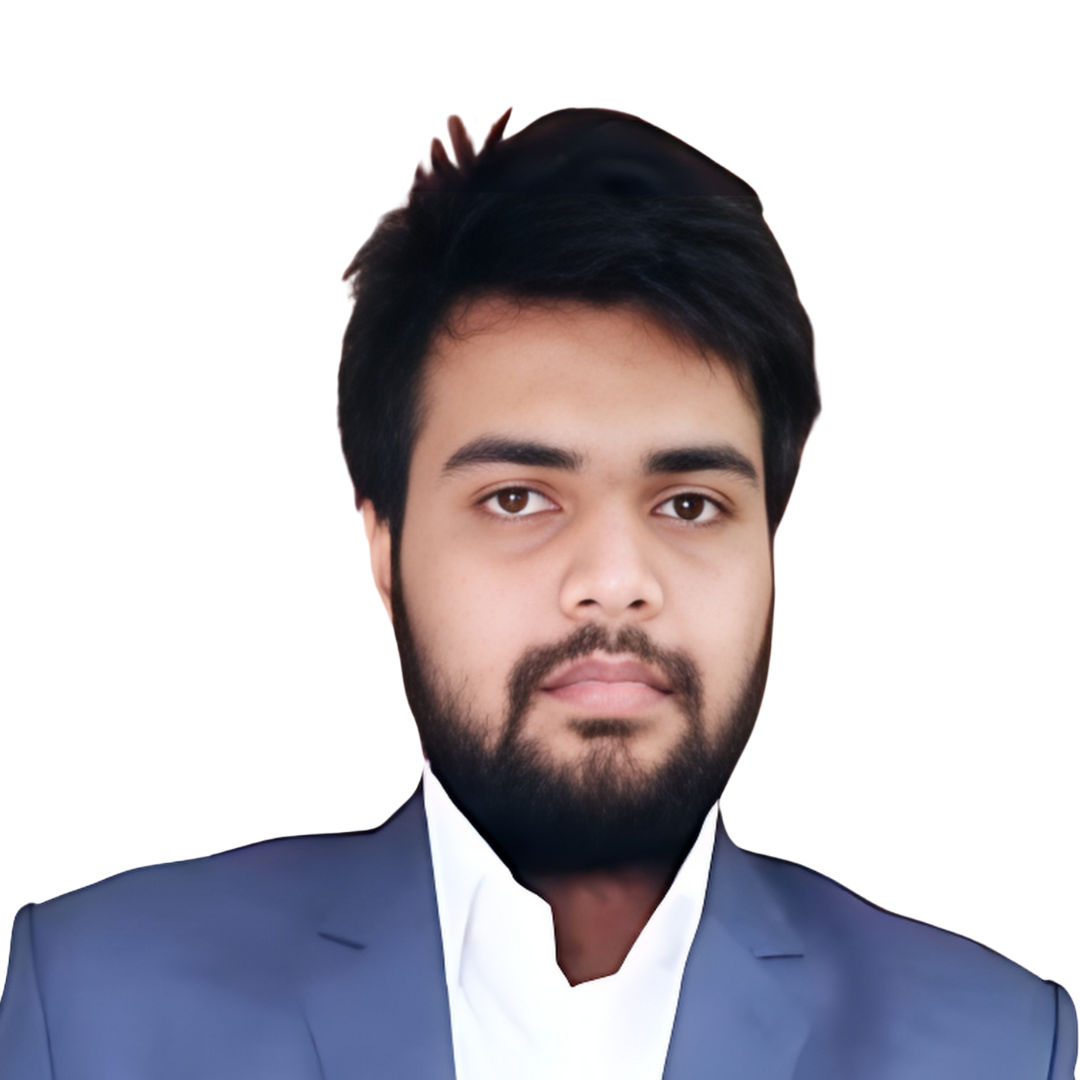
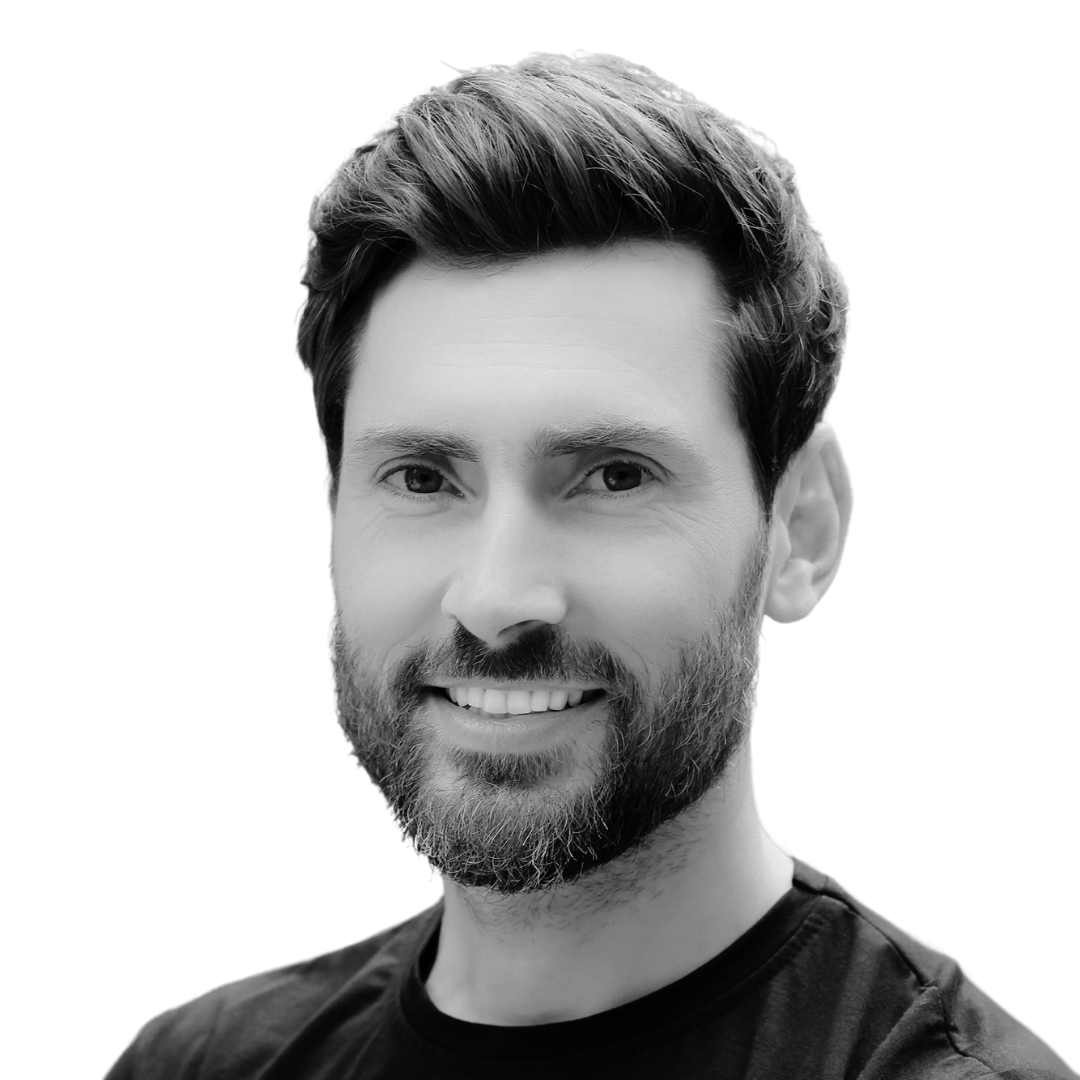
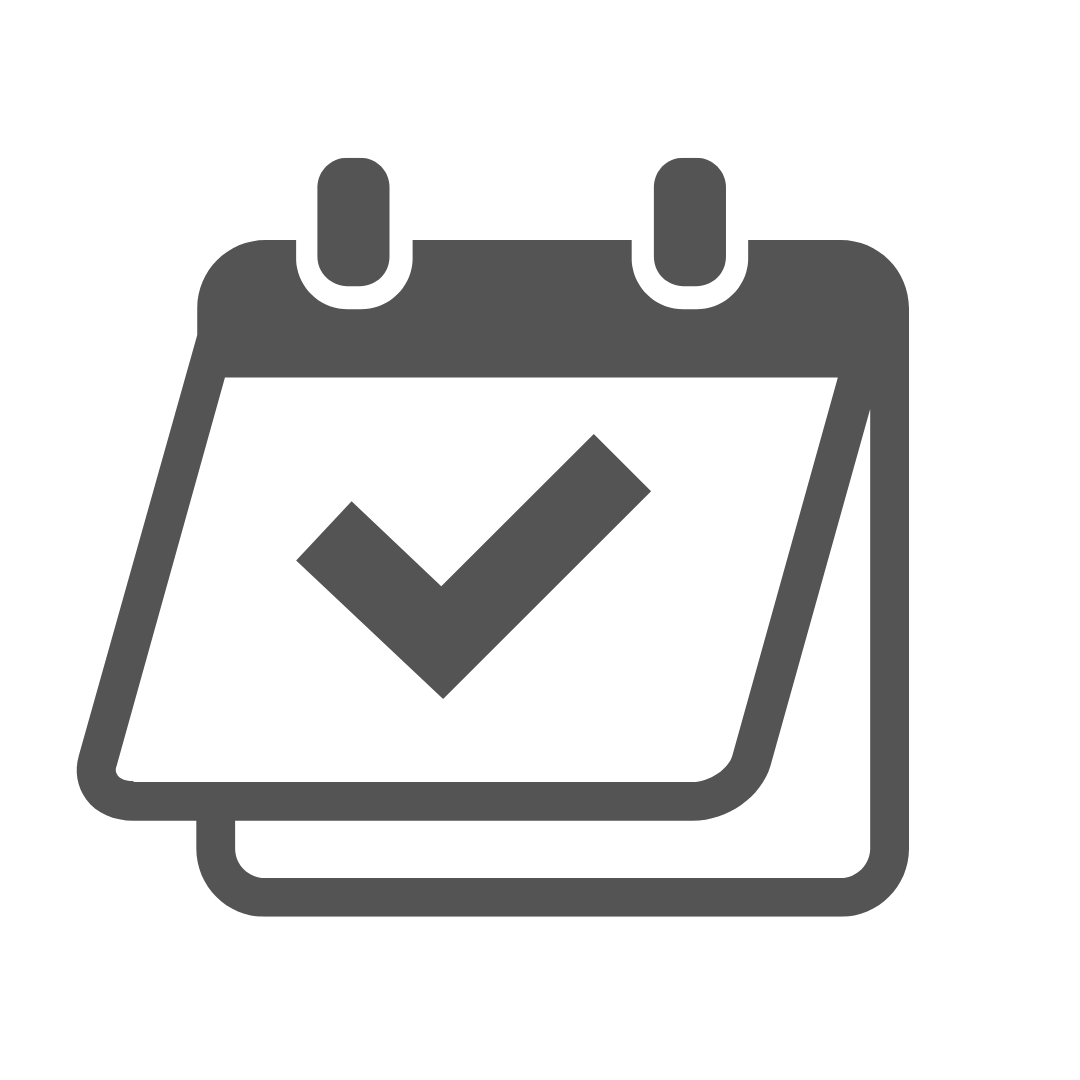
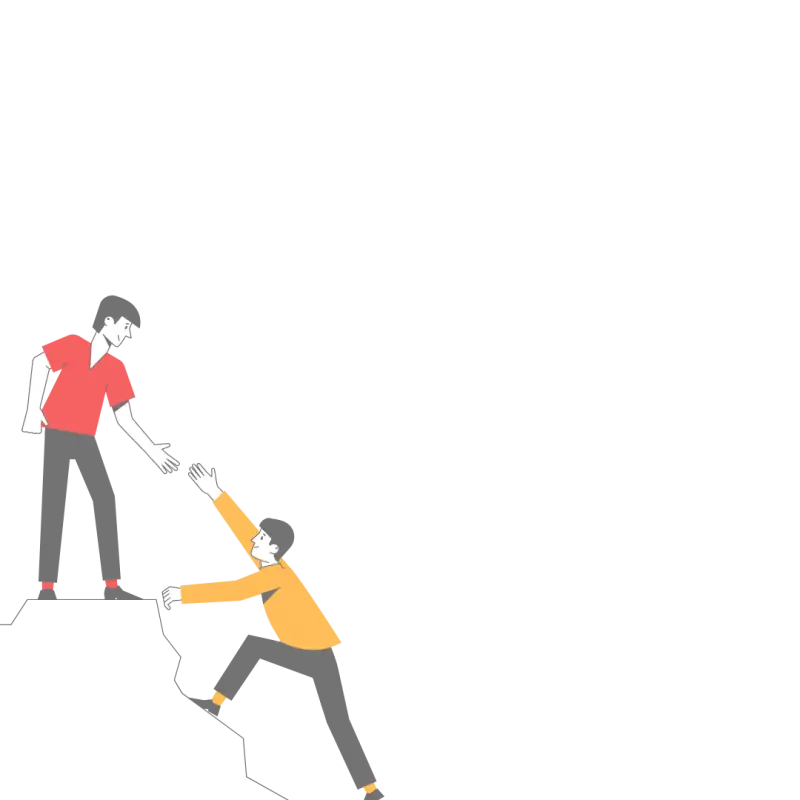
Thanks for your feedback!
Your contributions will help us to improve service.
How to Convert Deciaml to Binary in Vue Js
-
The
data
property in the Vue application defines two properties:decimalNumber
andbinaryNumber
. ThedecimalNumber
property is initialized with the value of11
and thebinaryNumber
property is left empty. -
The
computed
property defines a method calledconvertToBinary
, which is used to calculate the binary equivalent ofdecimalNumber
using thetoString()
method with a parameter of2
, which returns the binary representation ofdecimalNumber
. The computed property is used instead of a method so that it can be called as a property, and it will update automatically wheneverdecimalNumber
changes. -
Inside the
convertToBinary
method, the binary value is assigned tobinaryNumber
, which is then bound to theinput
element in the Vue template.
Overall, this code sets up a simple Vue application that allows the user to enter a decimal number and displays the binary equivalent of that number in real-time using Vue's reactivity system.