Vue Detect Viewport Changes
Vue Detect Viewport Chnages:Vue is a frontend JavaScript framework that helps developers create interactive web applications. To make these applications responsive and optimize their performance, it's crucial to detect changes in the viewport. One effective method for doing so is by using the window.addEventListener method to listen for resize events. This method allows you to trigger certain functions or updates when the user changes the size of their browser window, such as adjusting the layout of your application or reloading data. By implementing this method in your Vue application, you can ensure that your website is responsive and user-friendly across all devices and screen sizes.
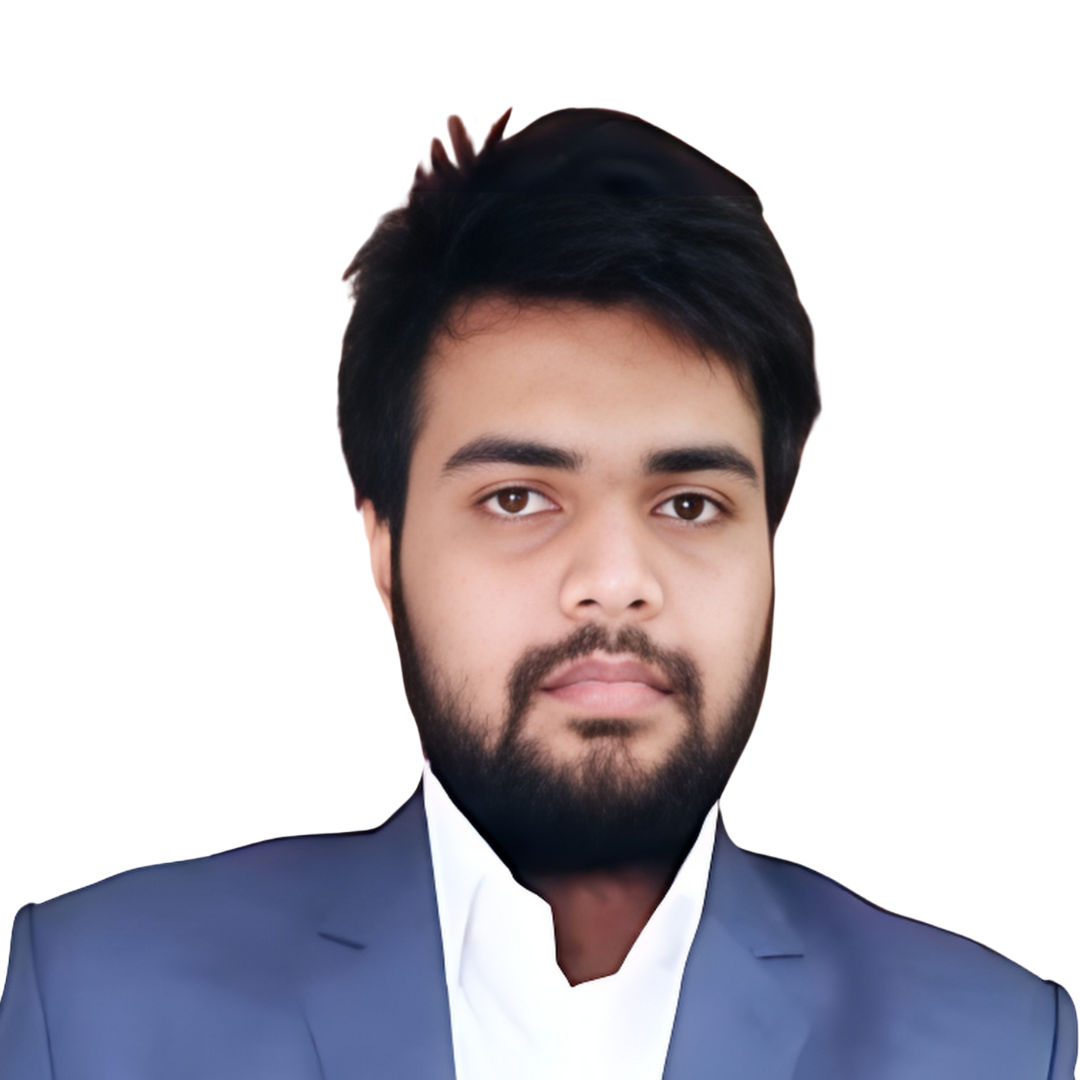
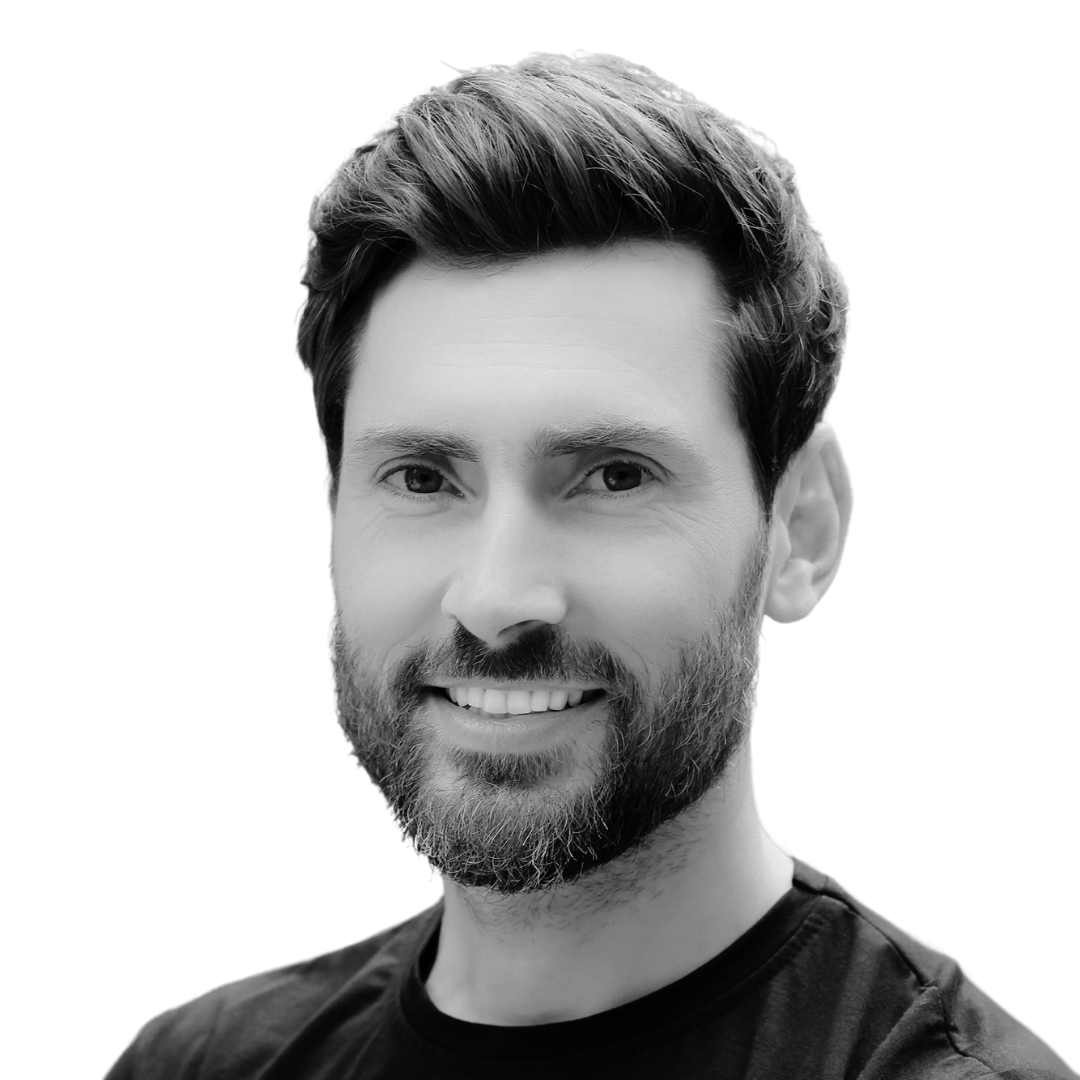
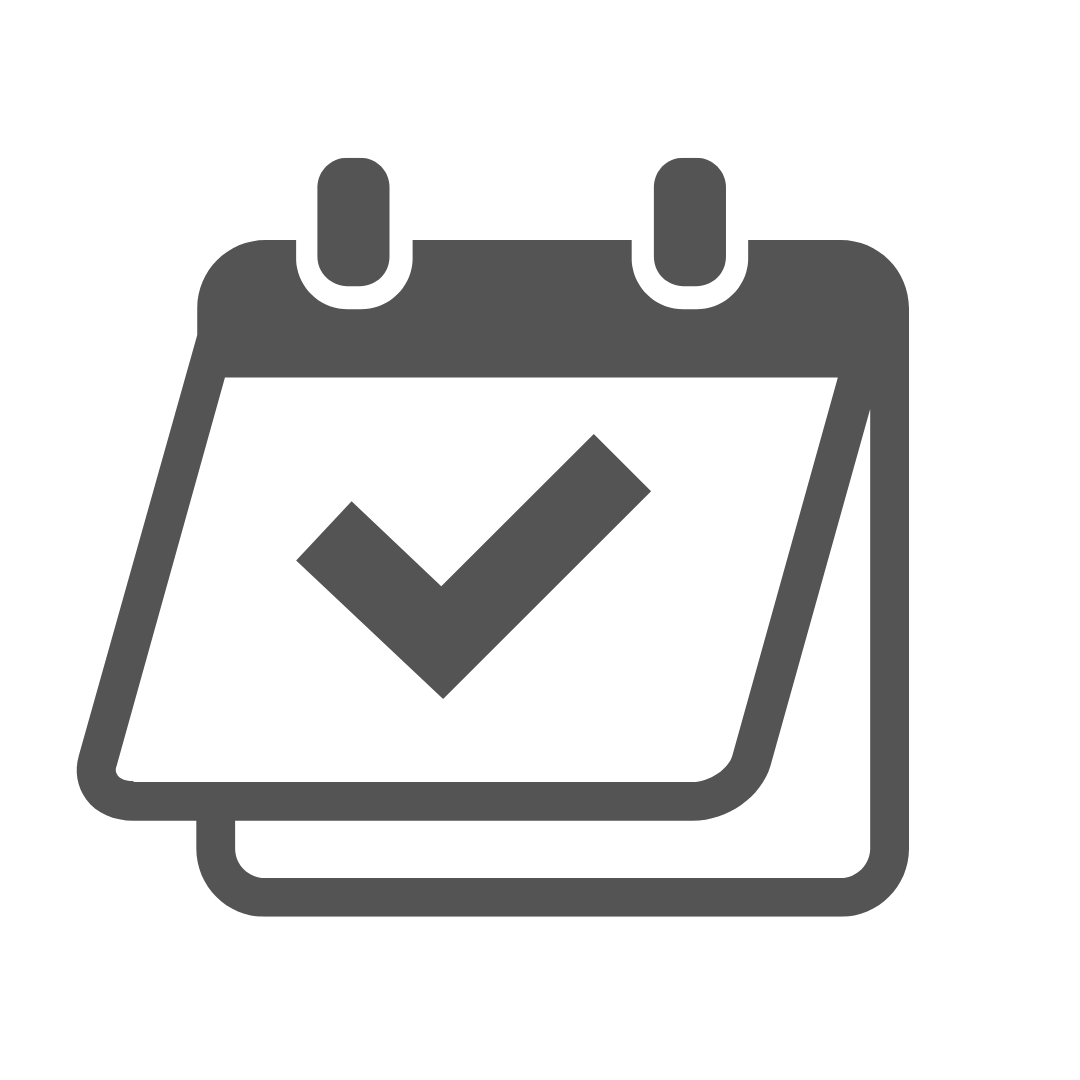
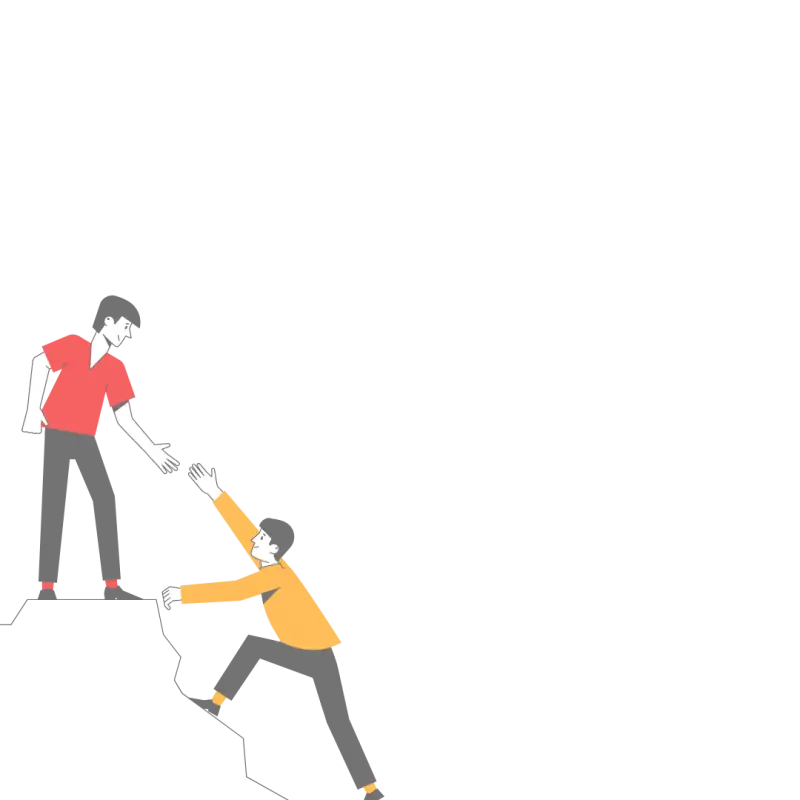
Thanks for your feedback!
Your contributions will help us to improve service.
How can Vue detect changes to the viewport size and adjust the rendering of components accordingly?
This Vue code creates a new app instance that has two data properties: viewportWidth
and viewportHeight
. These properties are initialized with the current window's width and height.
The mounted
lifecycle hook adds an event listener for the resize
event, which will trigger the handleResize
method when the window size changes. The beforeDestroy
lifecycle hook removes the event listener to avoid memory leaks. The handleResize
method updates the data properties with the new window size values.
The template displays the viewportWidth
and viewportHeight
values. When the window size changes, Vue will detect the change and automatically update the displayed values.
Vue Detect Viewport Changes Example
<div id="app">
<p>Viewport width: {{ viewportWidth }}</p>
<p>Viewport height: {{ viewportHeight }}</p>
</div>
<script>
const app = Vue.createApp({
data() {
return {
viewportWidth: window.innerWidth,
viewportHeight: window.innerHeight
};
},
mounted() {
window.addEventListener('resize', this.handleResize);
},
beforeDestroy() {
window.removeEventListener('resize', this.handleResize);
},
methods: {
handleResize() {
this.viewportWidth = window.innerWidth;
this.viewportHeight = window.innerHeight;
}
}
});
app.mount("#app");
</script>