Vue js Select On Change Update Value
Vue Js Select On Change Update Value -: In this tutorial, we will learn how to use Vuejs to create a select dropdown element and update its value when the user makes a selection. We will implement an event handler that listens for changes to the select element and updates a data property in the Vue instance accordingly. This way, we can reactively update other parts of our application based on the user's selection in the dropdown.
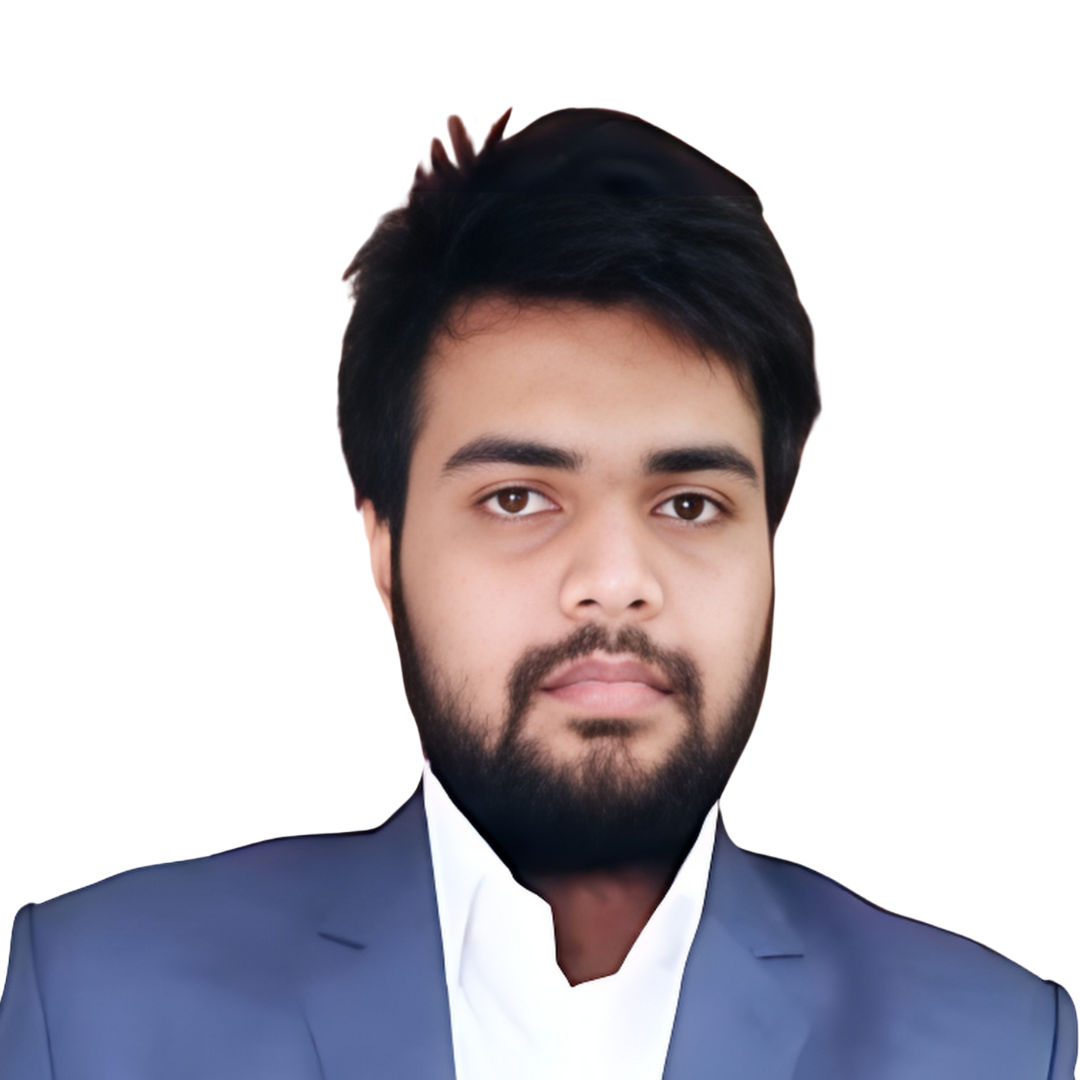
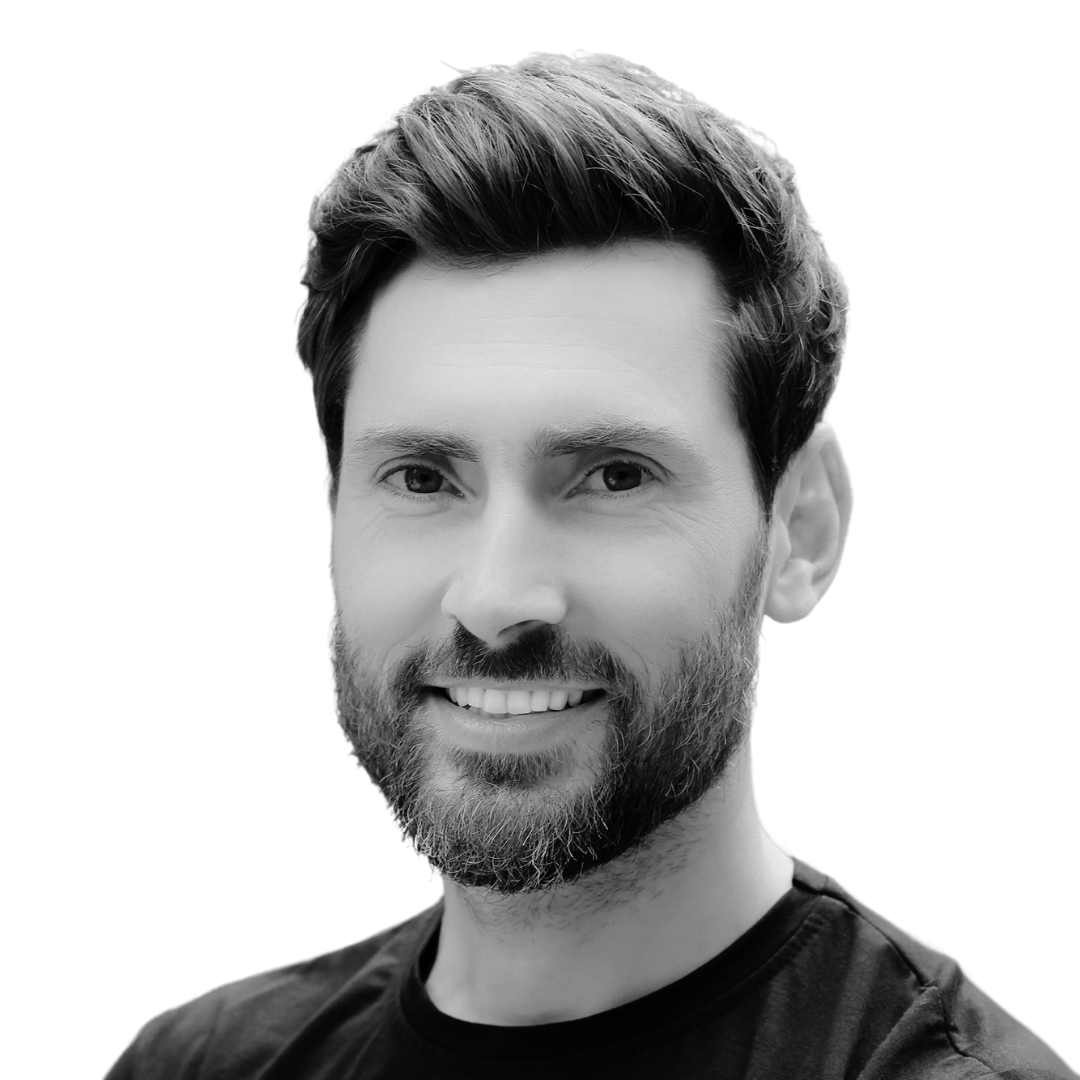
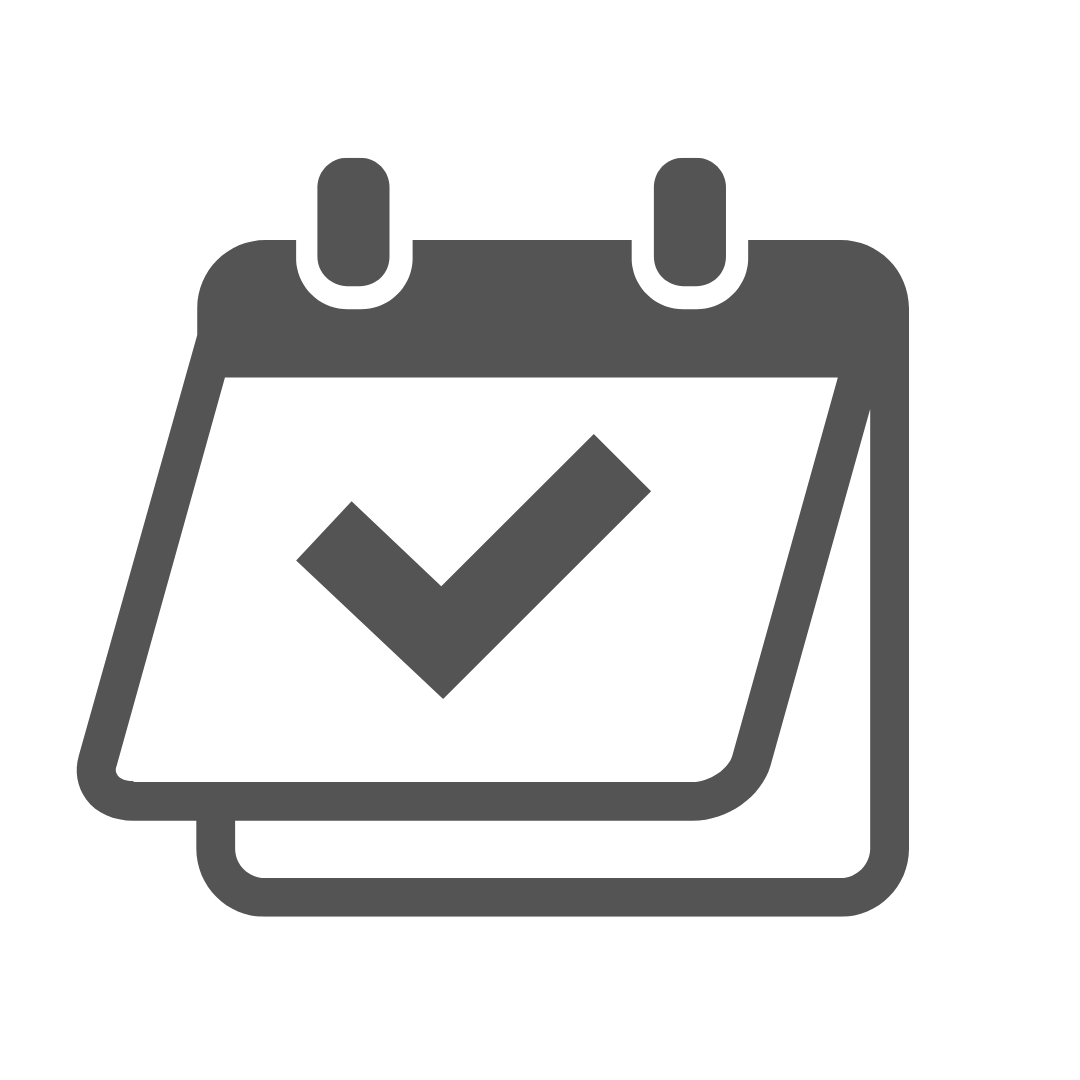
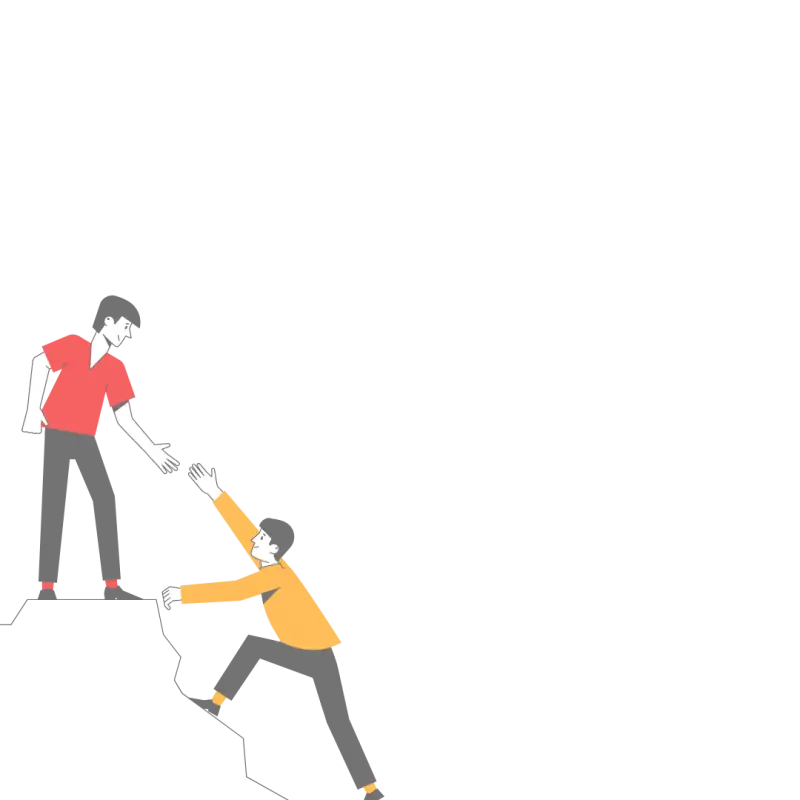
Thanks for your feedback!
Your contributions will help us to improve service.
How can I implement a Vuejs select element that updates a value when its option is changed
This Vuejs code creates a custom select element with options for different fruits. When the user selects a fruit, the "Selected Fruit" paragraph dynamically updates to show the chosen fruit. The v-model
directive binds the selected fruit to the selectedFruit
data property. A watch function is used to track changes in the selectedFruit
property, allowing you to perform additional logic when the selection changes. In this example, a message is logged to the console with the newly selected fruit's value.