React copy to clipboard onclick | React Js Paste from Clipboard Example
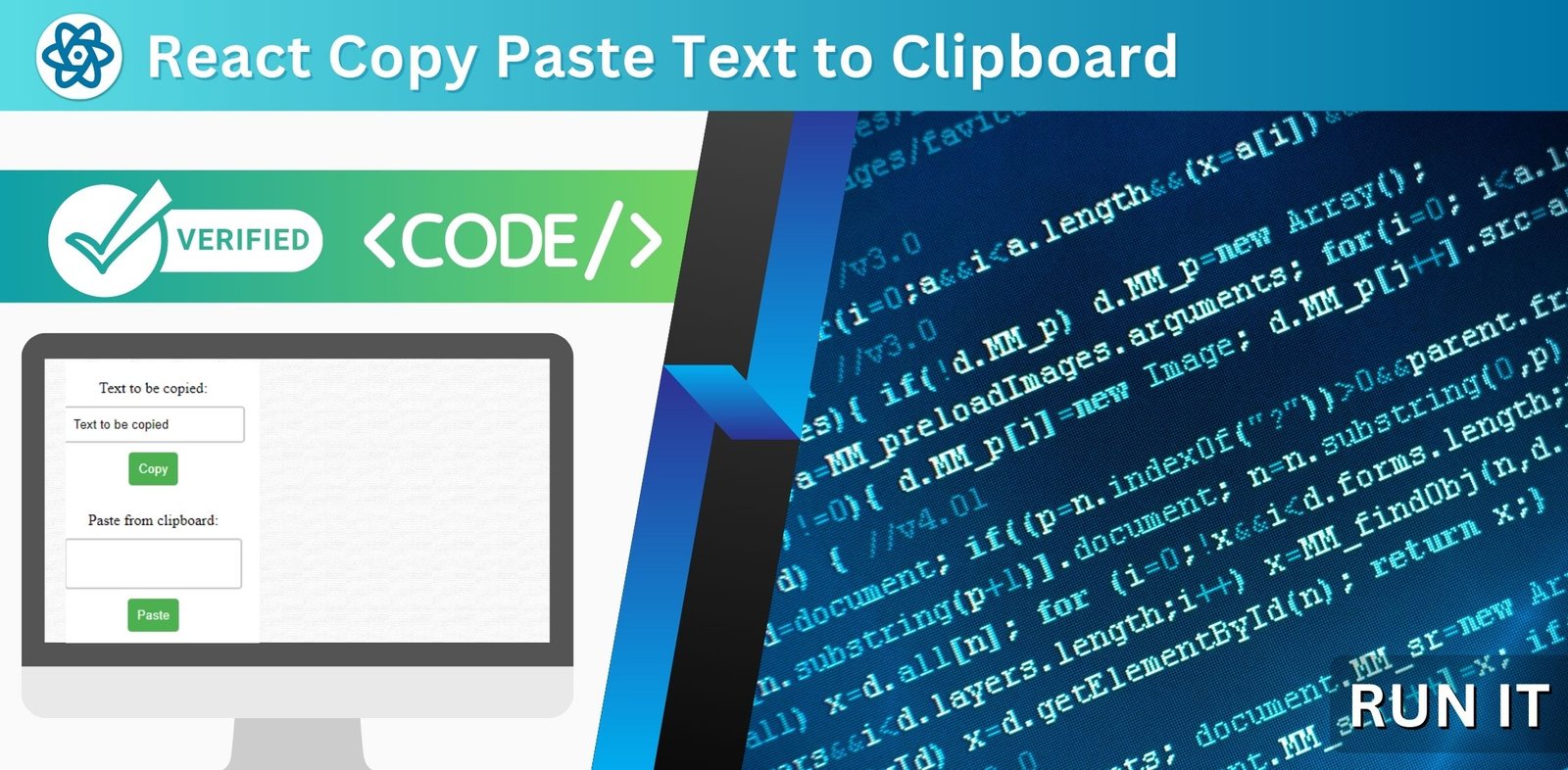
React copy to clipboard onclick | React Js Paste from Clipboard Example: In Reactjs, you can enable the copy and paste functionality using the clipboard API. The process involves three main steps. First, you need to access the clipboard using the navigator.clipboard object. Second, you can use the clipboard.writeText() method to write the desired text to the clipboard. Finally, you can retrieve the copied text by calling clipboard.readText(). By utilizing these methods, you can implement copy and paste functionality within your React.js application, allowing users to easily copy and paste text content.
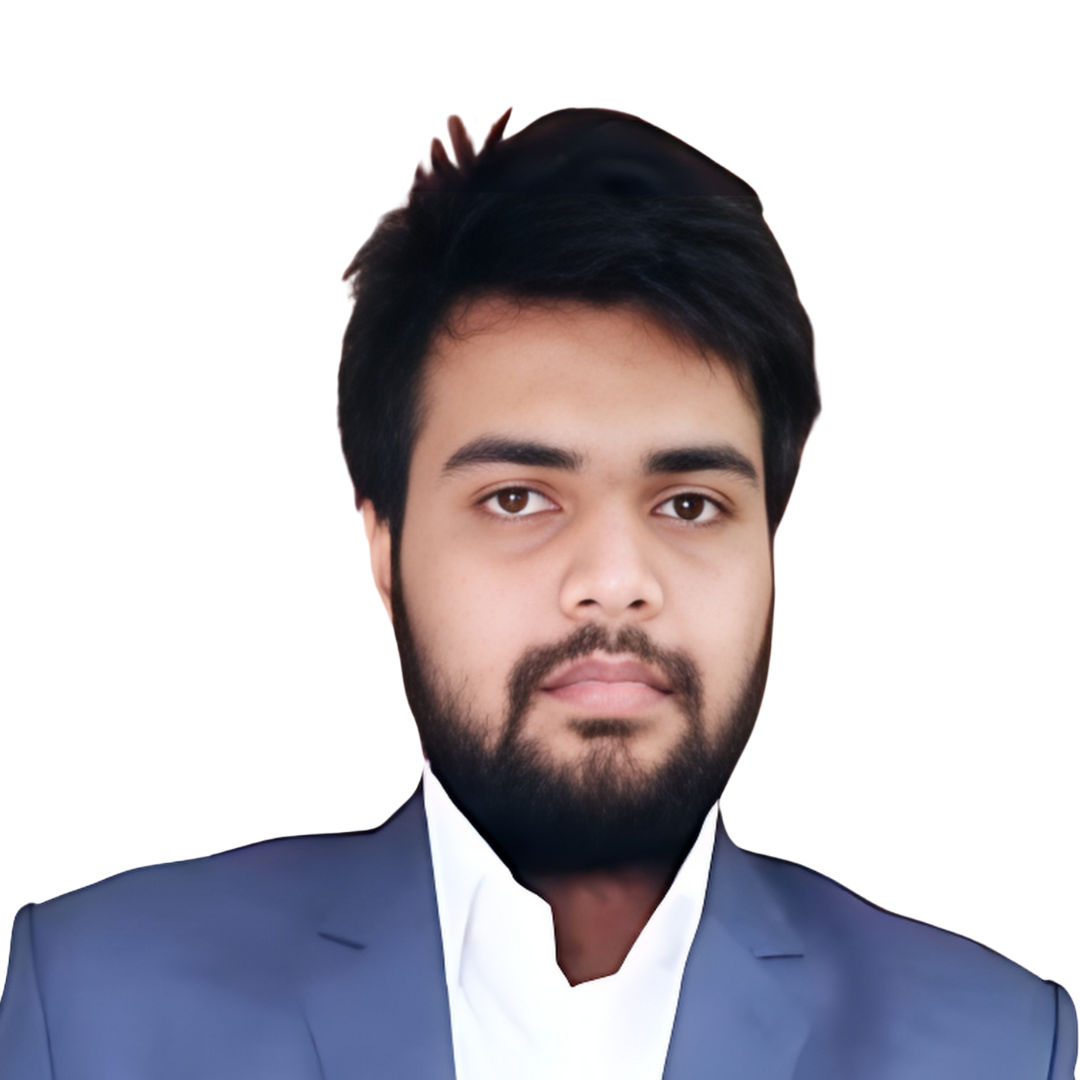
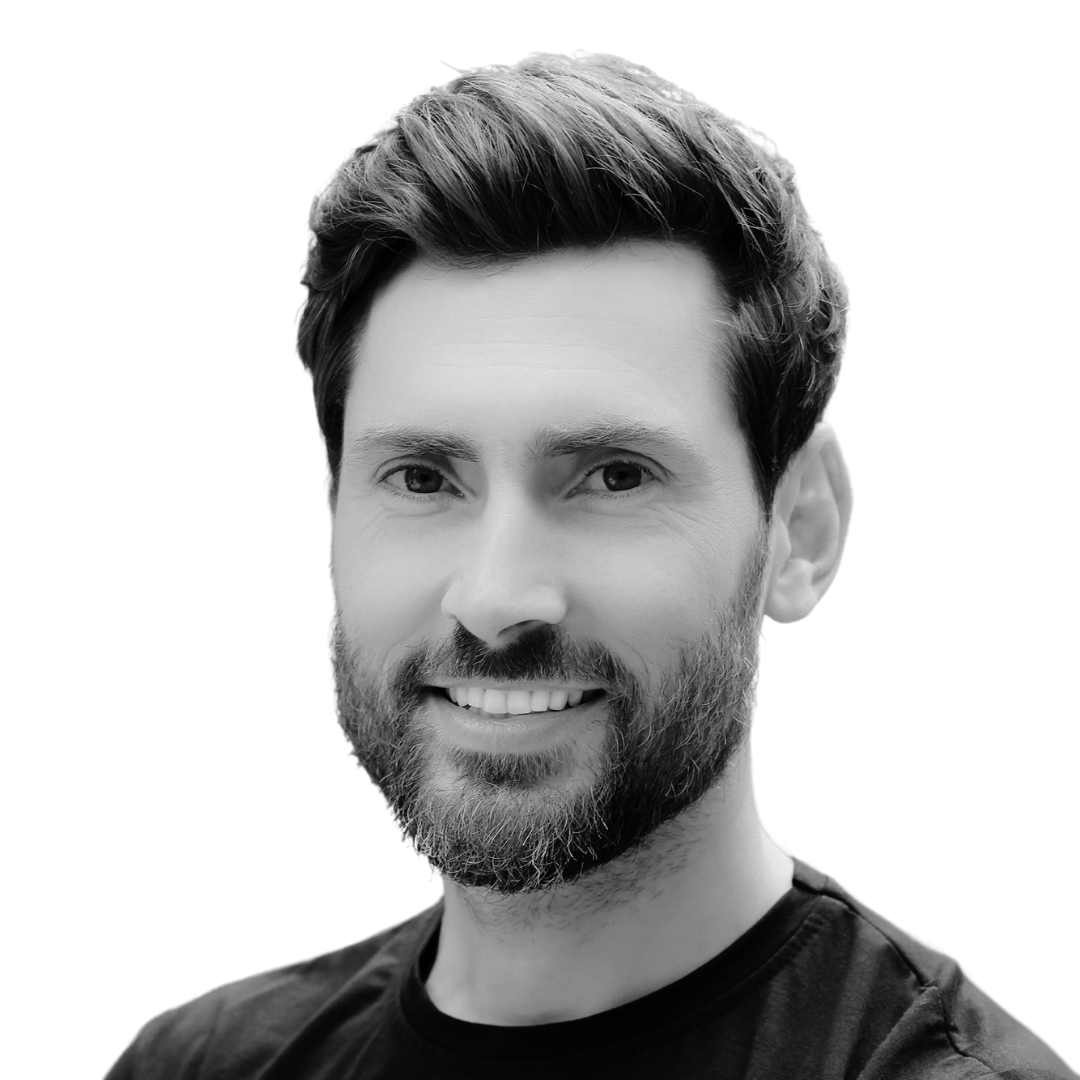
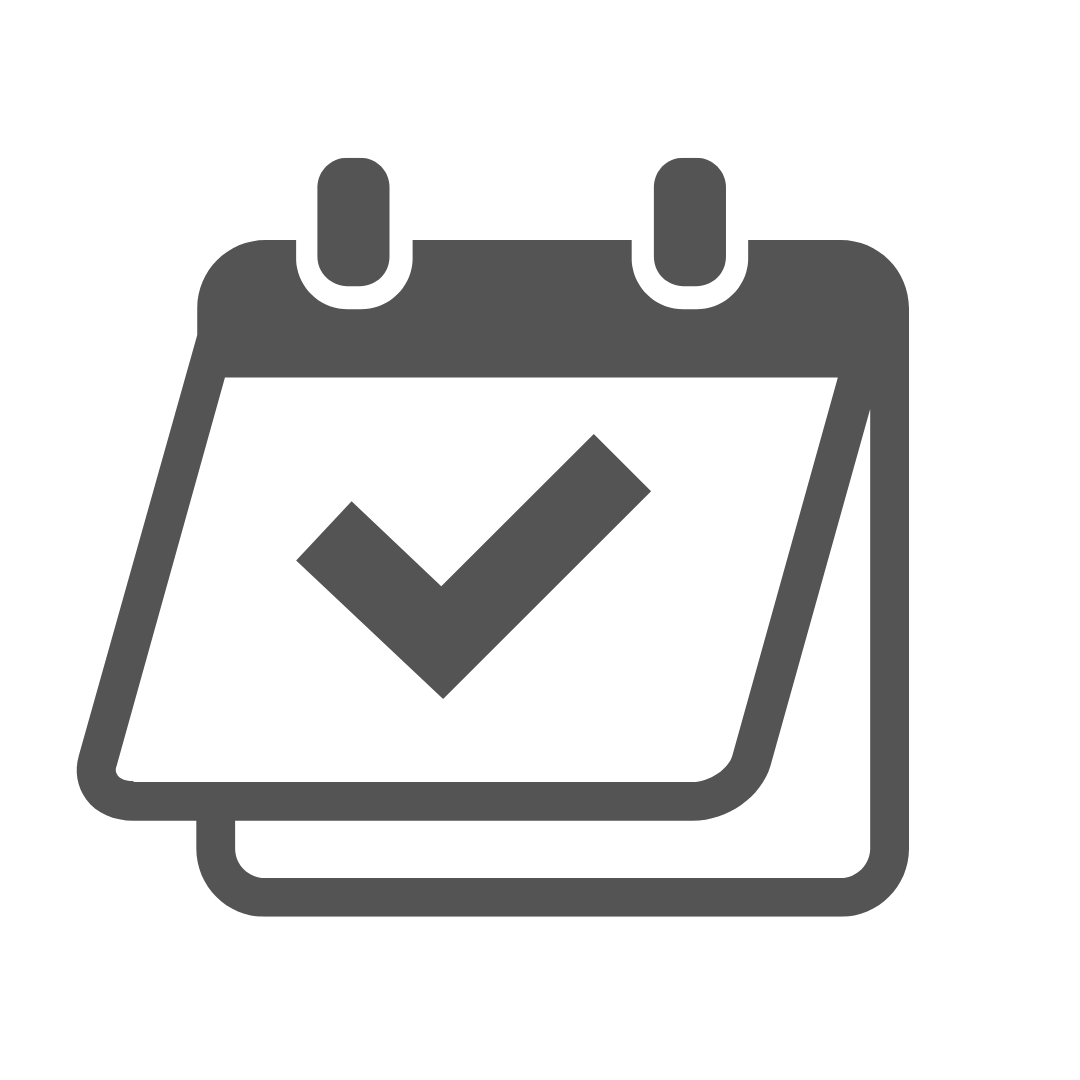
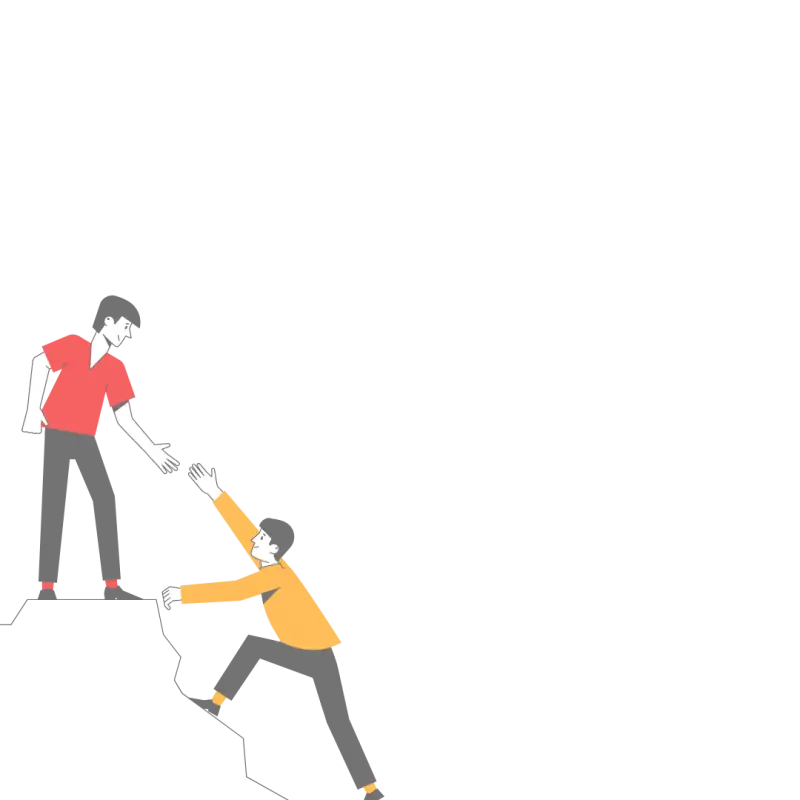
Thanks for your feedback!
Your contributions will help us to improve service.
How can I implement React js copy clipboard?
This code snippet demonstrates how to implement text copy and paste functionality using React.js. The code uses the `navigator.clipboard` API to interact with the system clipboard.
The component `App` sets up three states: `copyText` for the text to be copied, `pasteText` for the pasted text, and `result` to display the result message.
The `copyToClipboard` function uses `navigator.clipboard.writeText` to write the `copyText` to the clipboard. It updates the `result` state with a success message if the operation is successful or logs an error if it fails.
The `pasteFromClipboard` function uses `navigator.clipboard.readText` to read the text from the clipboard. It sets the `pasteText` state with the retrieved text and updates the `result` state with a success message or logs an error if the operation fails.
The render method sets up the HTML structure with input fields for copy and paste, along with buttons to trigger the copy and paste functions. The `result` state is displayed in a `<pre>` element if it has a value.
Output of React Copy to Clipboard
How can I implement a ReactJS example to enable users to paste content from their clipboard ?
This React JS example demonstrates how to enable pasting text from the clipboard using the navigator.clipboard.readText()
method. When the "Paste from Clipboard" button is clicked, it reads the clipboard's text and populates the textarea with the copied content. The useRef
hook is used to access and modify the textarea's value.