React Js setInterval Method | SetTimeOut
React Js setInterval Method: The setInterval method in ReactJS is a built-in JavaScript function that allows developers to execute a specific code repeatedly at a defined interval. It takes two arguments: a callback function and a time interval in milliseconds. The callback function is executed every time the interval elapses until the clearInterval method is called. Developers commonly use setInterval to perform periodic updates, and animations, or to fetch new data from an API.
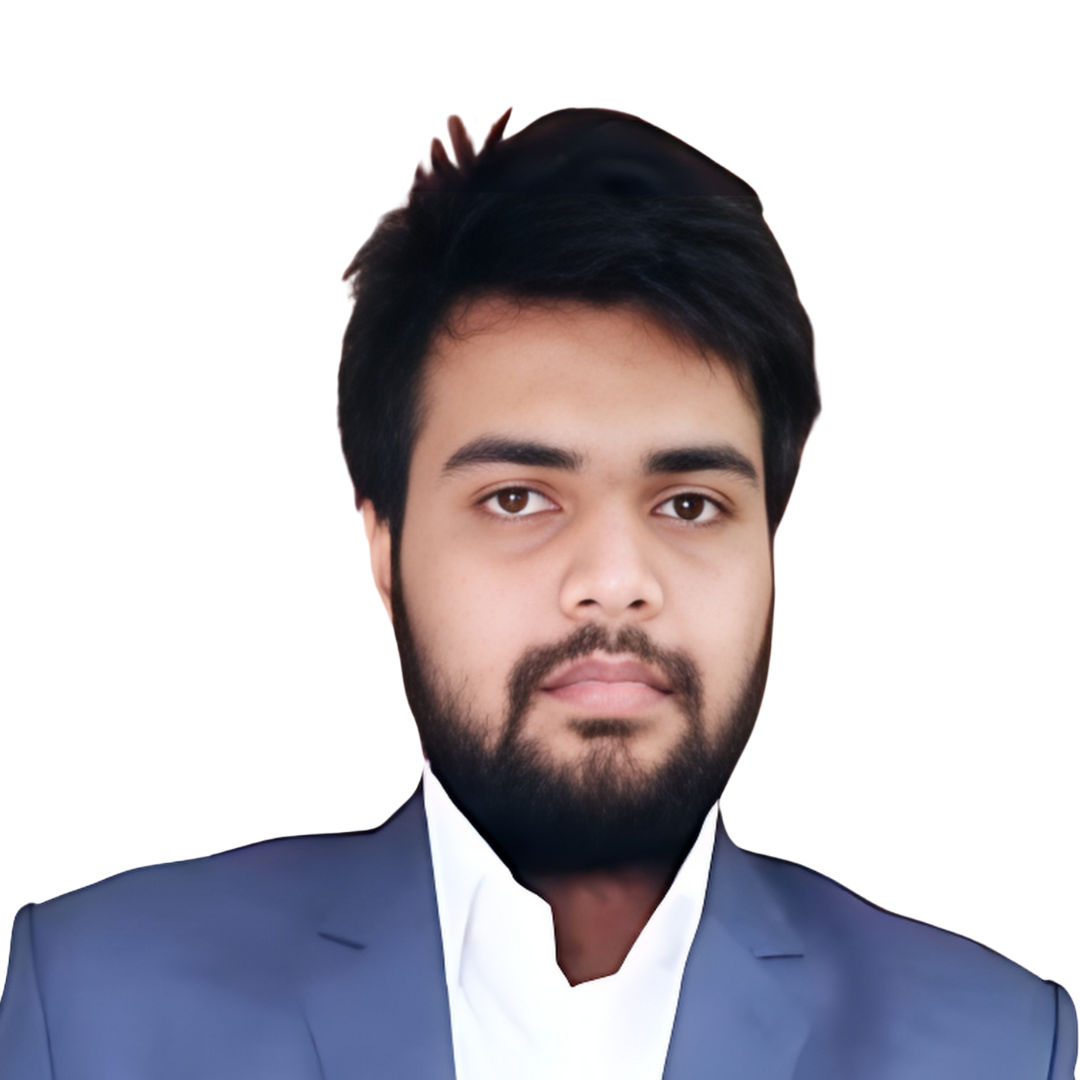
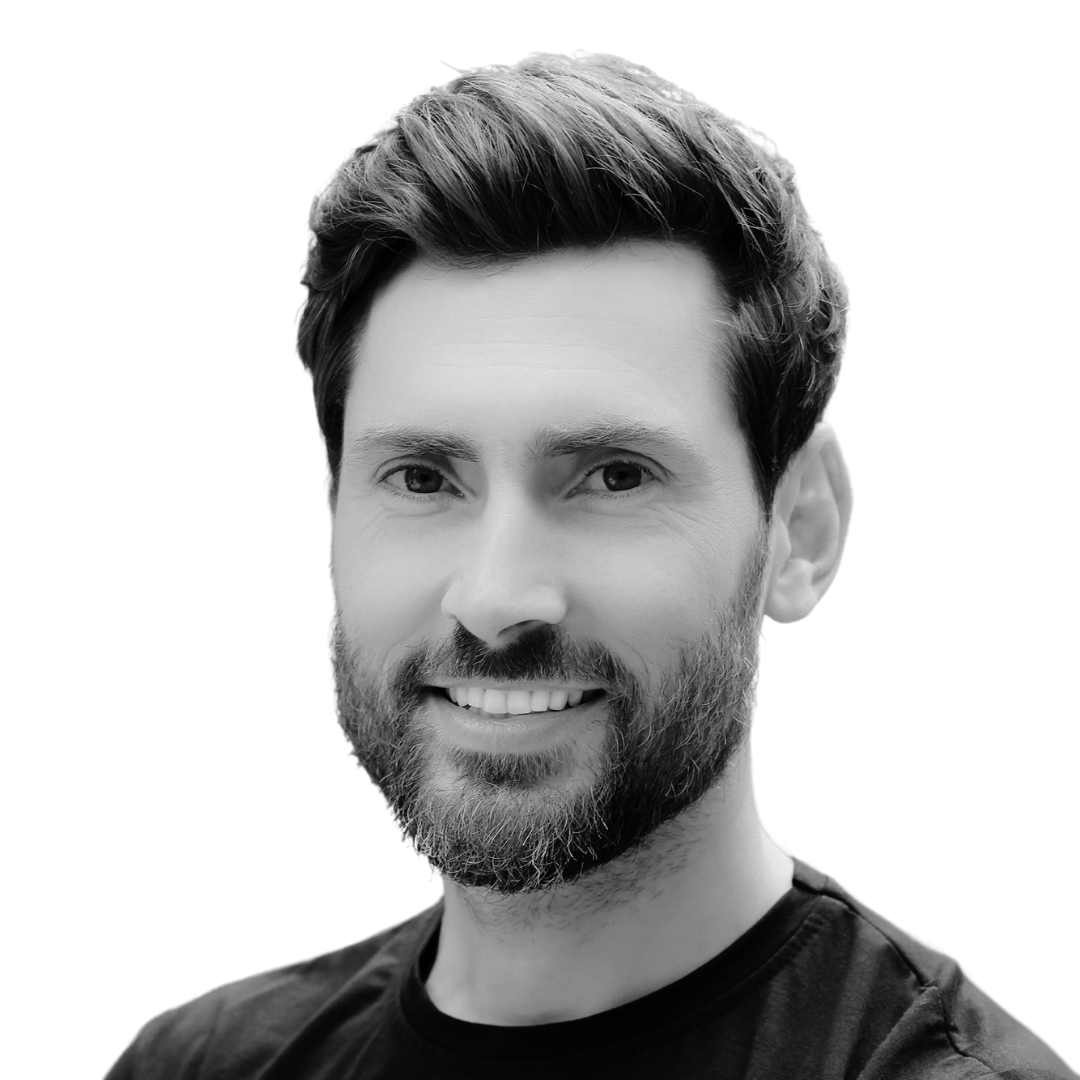
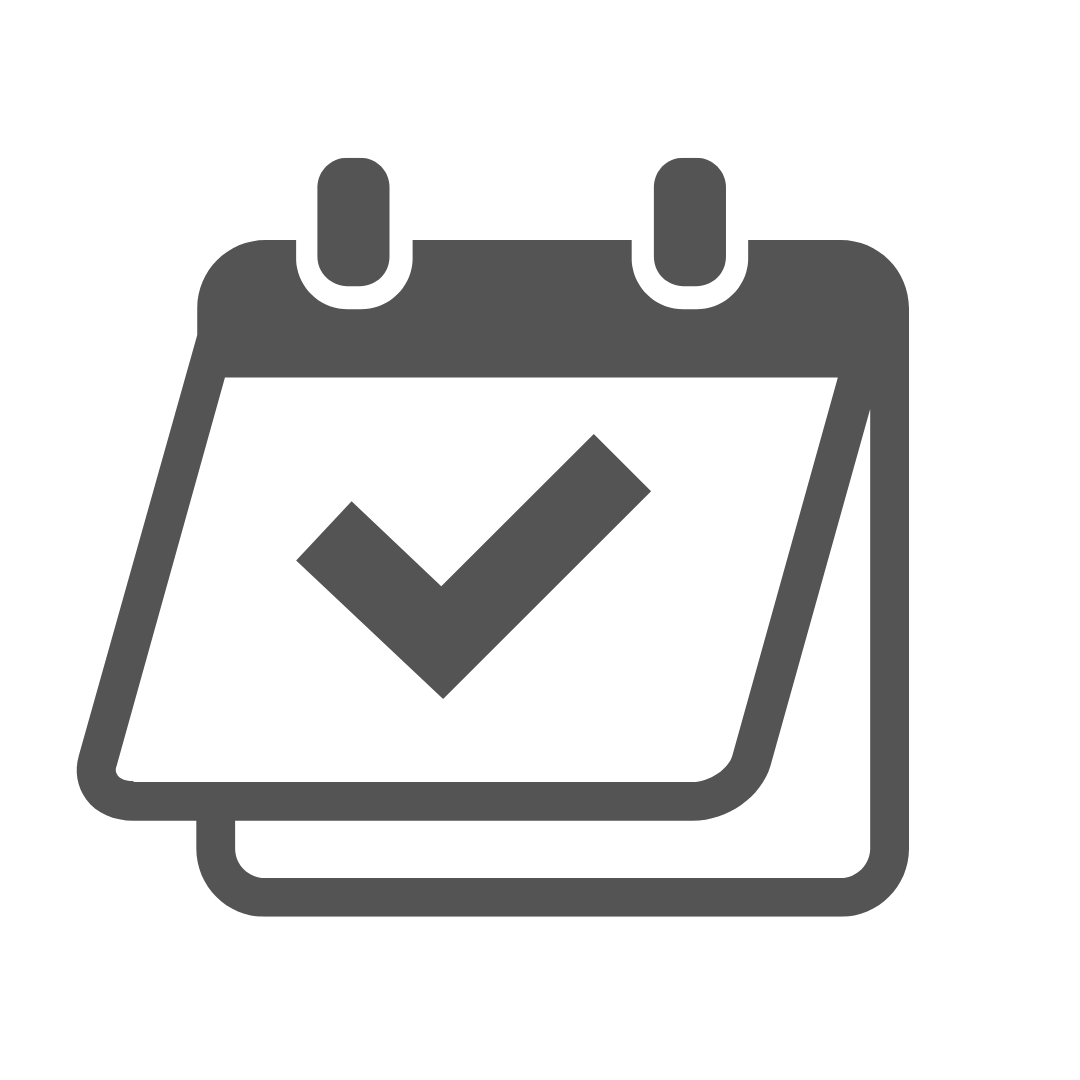
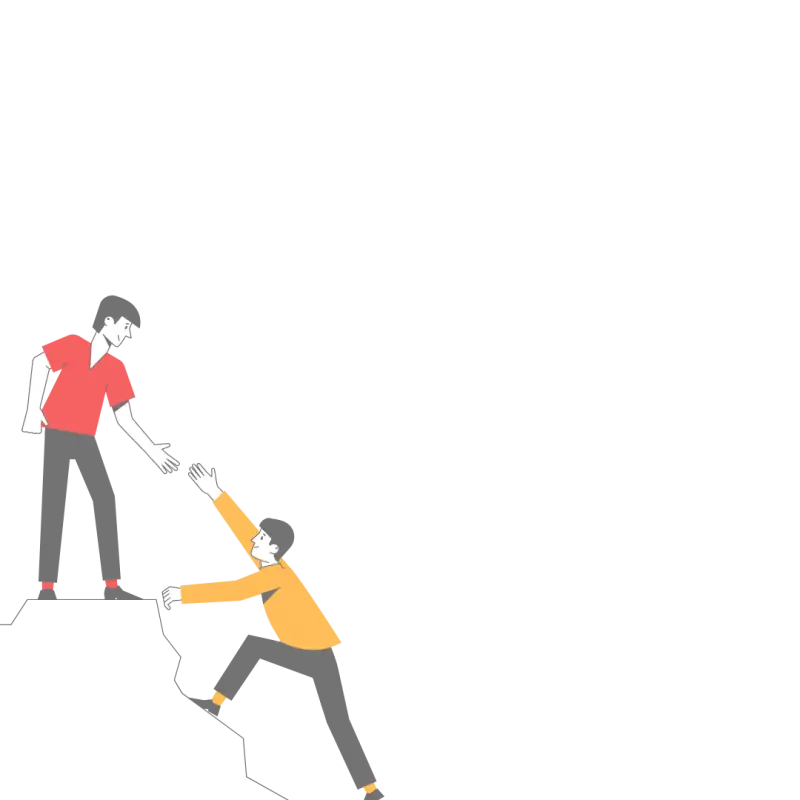
Thanks for your feedback!
Your contributions will help us to improve service.
What is the purpose of the React Js setInterval()
method?
This is a code snippet written in JavaScript using the React library. Here's an explanation of what the code does:
-
The first line imports three React hooks:
useState
,useEffect
, anduseRef
. -
The
App
function is defined. It initializes a state variablemessage
using theuseState
hook. The initial value ofmessage
is an empty string. -
The
useEffect
hook is used to run a side effect in the form of a function that updates themessage
state every 5 seconds. ThesetInterval
function is used to call this function every 5 seconds. TheuseEffect
hook returns a function that clears the interval when the component unmounts. -
The
return
statement of theApp
function returns a JSX expression that renders adiv
with anid
ofapp
. Inside thisdiv
, there are twop
elements, one with the static text "Use of setInterval in React Js" and another with the value of themessage
state variable, which gets updated every 5 seconds. -
Finally, the
ReactDOM.render
the method is used to render theApp
component to the HTML element with theid
ofapp
on the page.
Output of React Js setInterval Method
In React JS, what is the process for stopping an interval?
This code is written in JSX, which is a syntax extension of JavaScript that allows you to write HTML-like code in JavaScript. The code is using React, a JavaScript library for building user interfaces.
- The
useState
,useEffect
, anduseRef
hooks are imported from the React library. - The
App
function is defined. It uses theuseState
hook to create a state variablemessage
and a function to update it calledsetMessage
. It also uses theuseRef
hook to create a reference to the interval ID, which will be used to stop the interval later. - The
useEffect
hook is used to set up the interval that updates themessage
state variable with the current time every 2 seconds. The hook returns a function that clears the interval when the component unmounts. - The
return
statement contains the JSX that renders the component to the DOM. It includes a header, some text, and a paragraph that displays the current time stored in themessage
state variable. It also includes a button that, when clicked, stops the interval by calling theclearInterval
function with the interval ID stored in theintervalIdRef
reference. - Finally, the
ReactDOM.render
function is called to render theApp
component to an HTML element with the ID of "app" in the DOM.
Output of above example