React Map example with index
React Map Example with Index : A React map example with index involves using the map
function to iterate through an array and render elements with their respective indices. For example, array.map((item, index) => {...})
.
To map an array of objects with an index, use the same map
function and access object properties using item.property
inside the callback.
To map only the first object in an array, use array.slice(0, 1).map((item) => {...})
.
To map a specific index range, use array.slice(startIndex, endIndex).map((item) => {...})
.
To change the style of elements in the map, conditionally apply CSS classes based on data or index within the map
callback function.
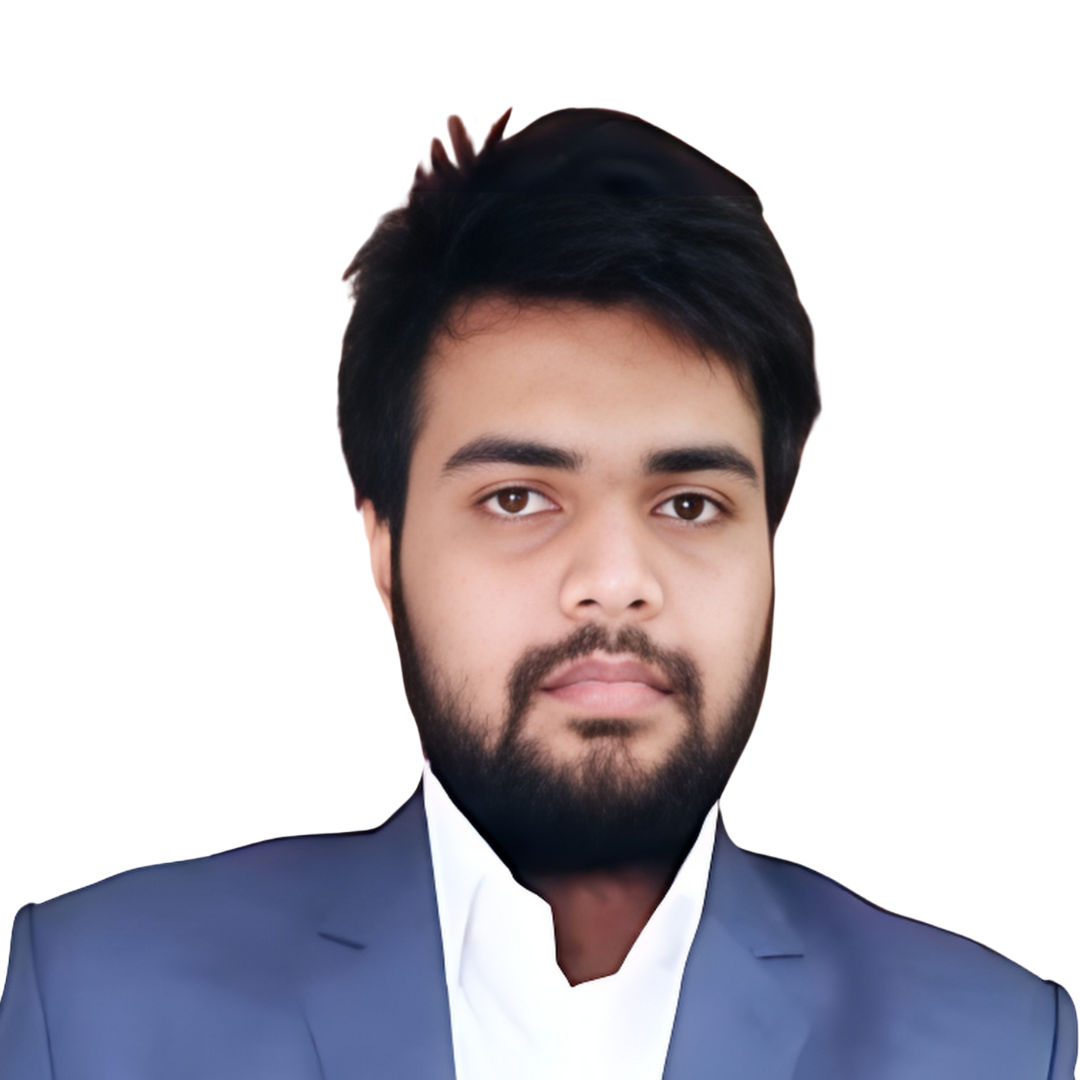
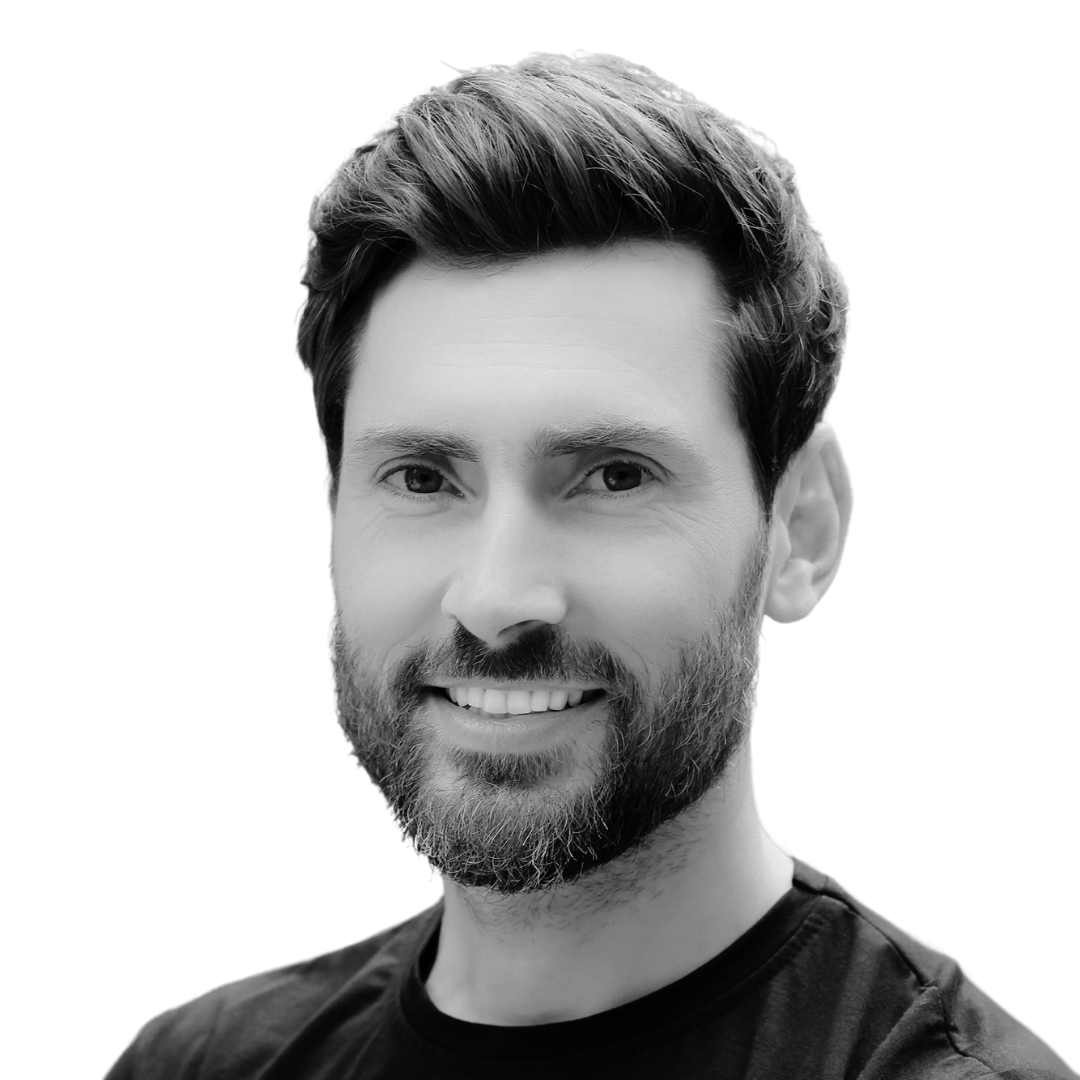
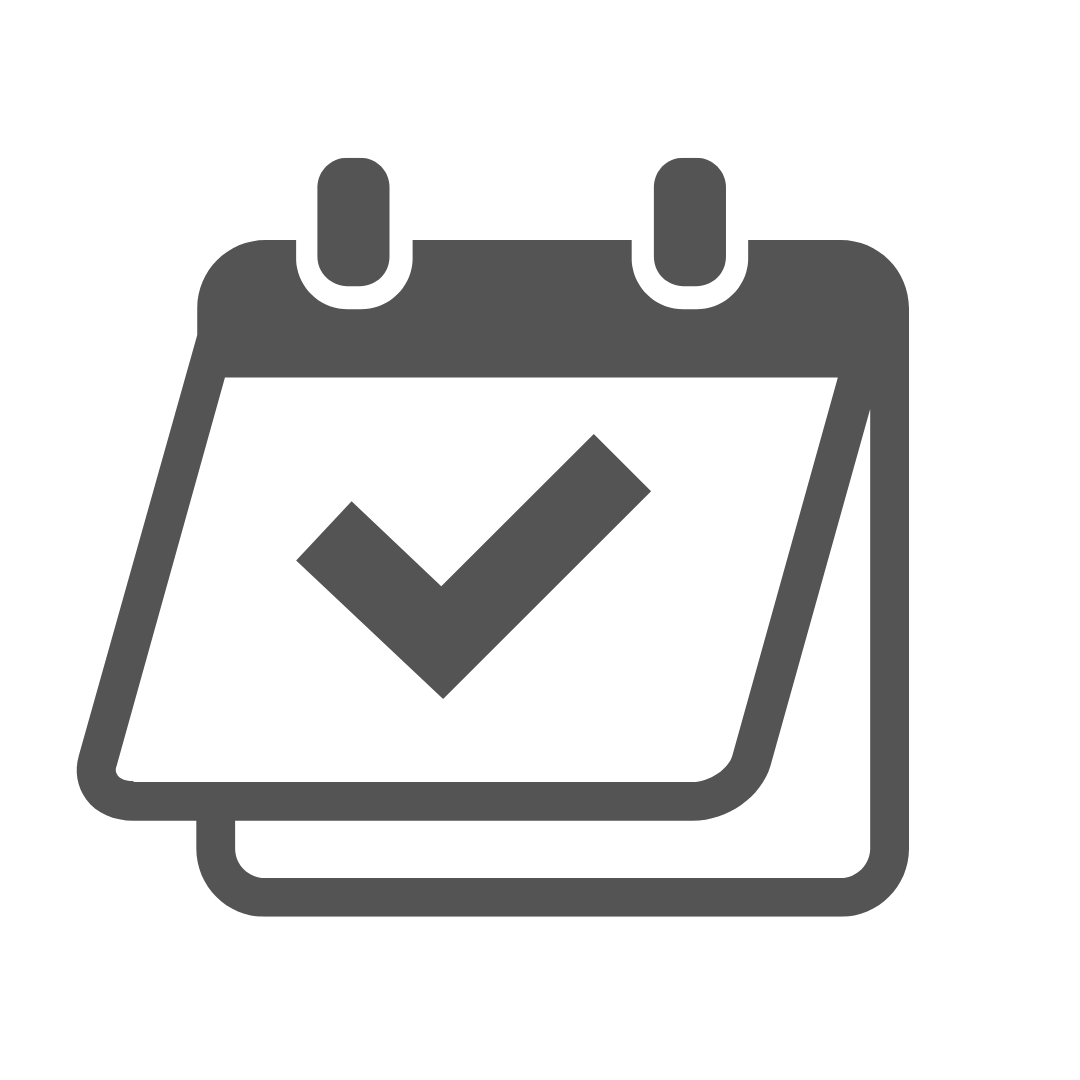
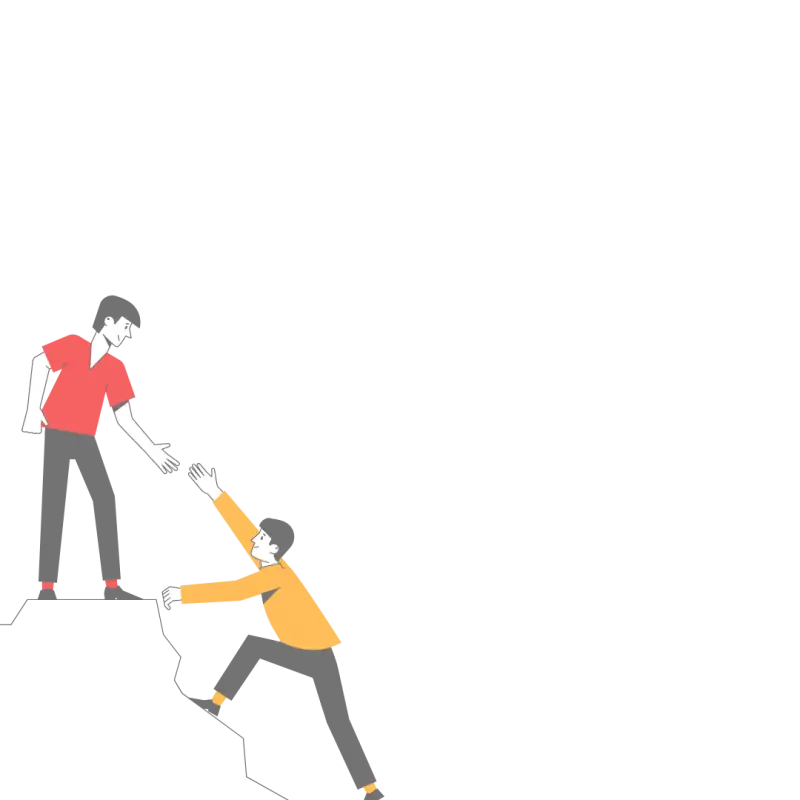
Thanks for your feedback!
Your contributions will help us to improve service.
How can I create a React map example that includes the index of each element in the mapped array?
This React code example displays a list of fruits using the map function, along with their corresponding index. The sample items are ["Apple", "Banana", "Orange", "Grapes"]. It renders an ordered list (ul) with each fruit displayed as a list item (li) in the format "index + 1. Fruit Name."
Output of React map Example with Index
How can I map an array of objects in React, including the index of each object as part of the mapping process?
This React example uses the map
function to iterate through an array of objects (data
) and renders a list of people with their names and ages. The index
parameter of map
is used to provide a unique key to each list item. The output displays each person's name, age, and their corresponding index in the array.
Output of React Map array of object example with index
How to map the first object in an array in react?
This React example renders the properties of the first object in the 'dataArray' array. It checks if the array has at least one object, then displays the ID and Name of the first object using JSX. If the array is empty, it shows "No data available."
Output of React map first object in an array Example
How to map an index range in react?
The given React code demonstrates an example of rendering a specific range of elements from an array using the map
function. The original data array contains objects with 'id', 'name', and 'category' properties. The code selects a range of elements (index 2 to 5) from the array and renders them as components using JSX. Each component displays the name and category of the corresponding item. The result is displayed within a container element with a title "React map index range Example."
Output of React map index range Example
How can you dynamically change the style of an element rendered through a map function in React?
This React code uses the map
function to iterate over an array of data
objects. Each object represents an item with an id
and text
. Depending on the id
, the getStyle
function returns different styles for the items. The div
elements are rendered with dynamic styles based on their respective id
, changing the background color and text color.