React Js Change url without page reloading

React Js Change Url Without Page Reloading : In React.js, you can change the URL without page reloading using the history object from JavaScript's History API. By accessing the history object, you can manipulate the browser's history stack, allowing for smooth navigation within your application. To change the URL, you can use the push or replace methods provided by the history object. The push method adds a new entry to the history stack, while the replace method replaces the current entry. This way, you can update the URL dynamically and navigate between different views or components in your React application without triggering a full page reload.
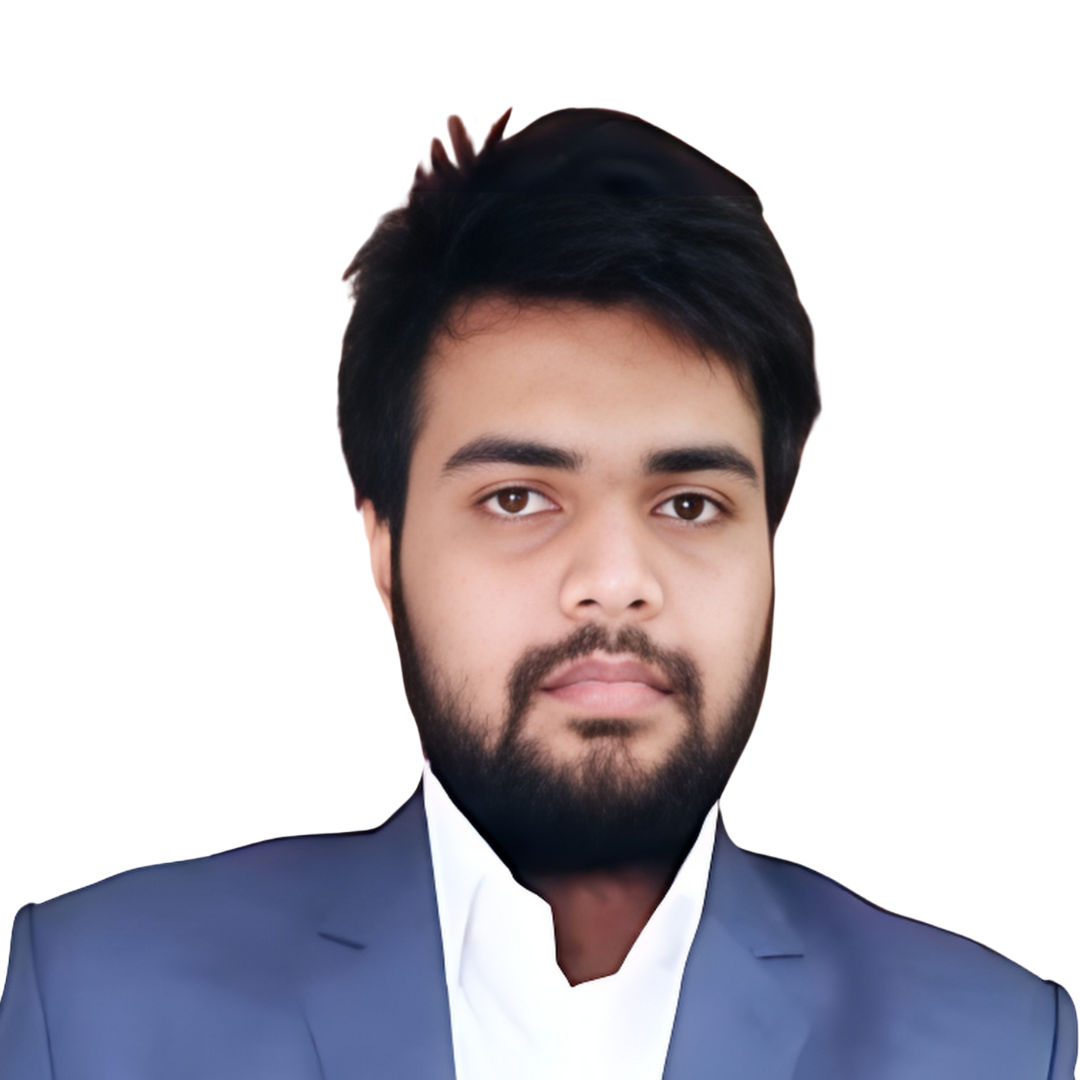
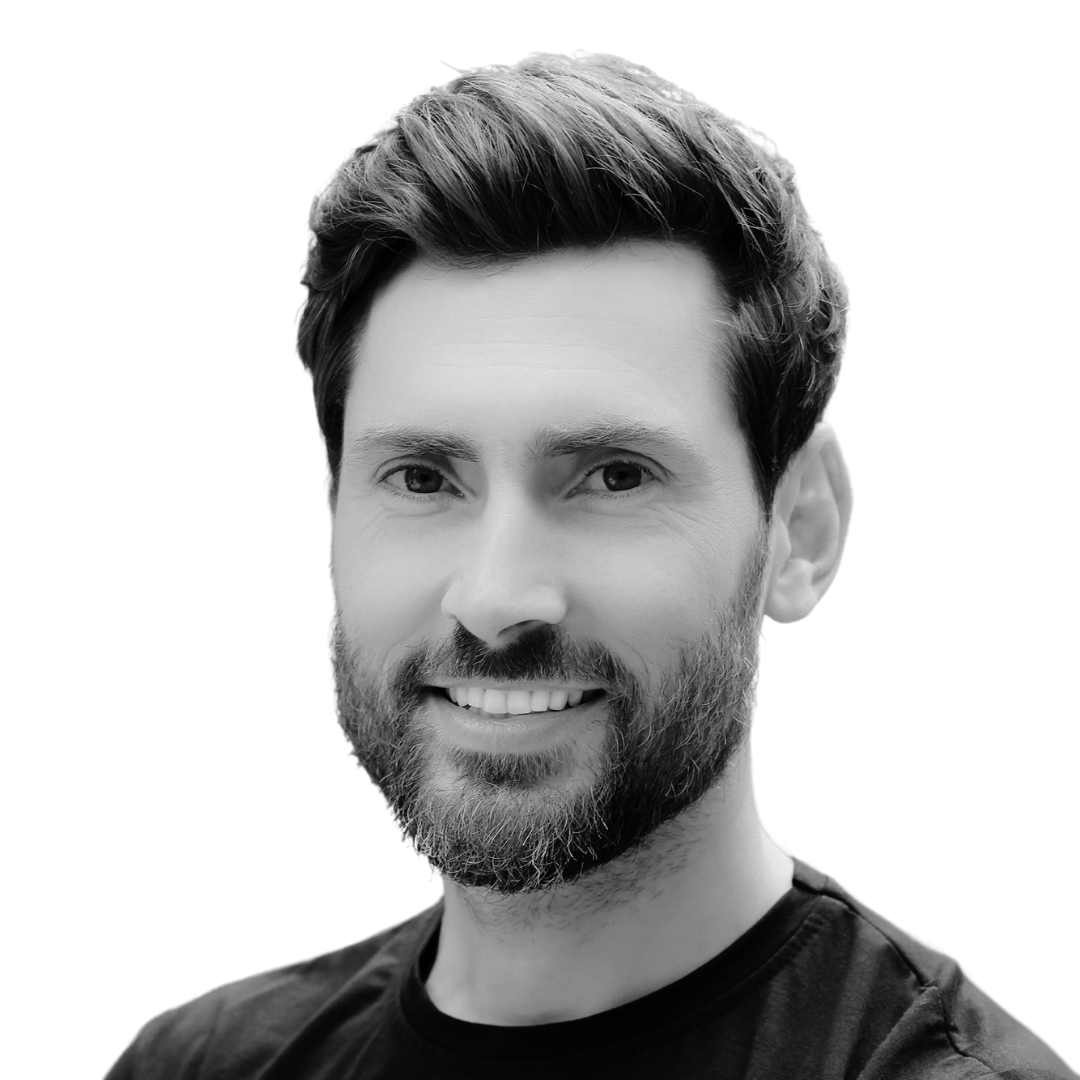
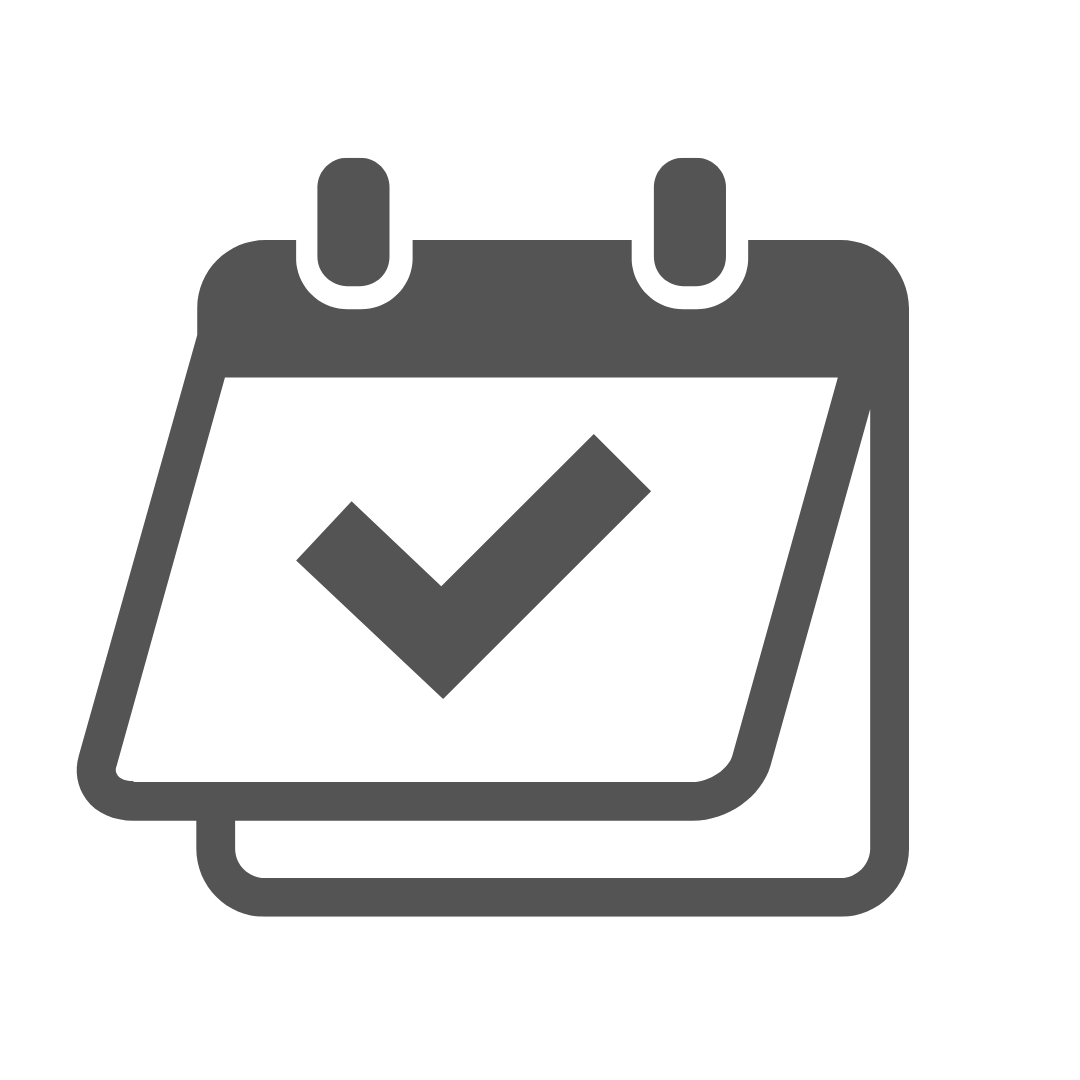
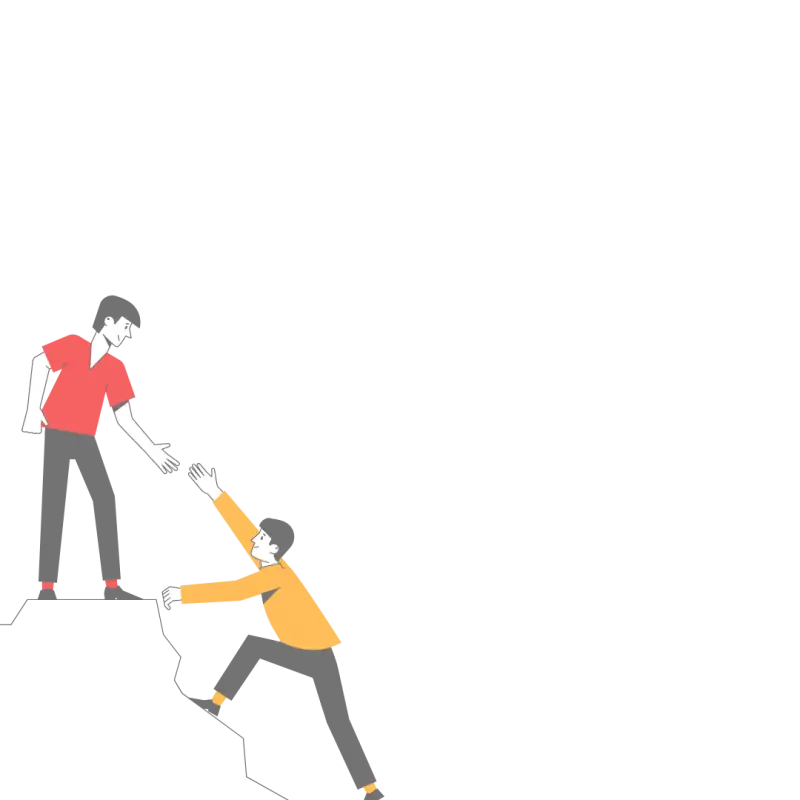
Thanks for your feedback!
Your contributions will help us to improve service.
How can you change the URL in React js without triggering a page reload?
In this React.js code snippet, the handleClick
function is triggered when the "Change URL" button is clicked. Inside the function, a new URL is specified (/new-url
in this example).
To change the URL without reloading the page, window.history.pushState(null, '', newUrl)
is called. This method manipulates the browser history and updates the URL to the specified newUrl
, without causing a page refresh.
After changing the URL, you can perform additional logic or state changes if needed.
Overall, this code demonstrates how to change the URL dynamically in a React.js application without reloading the entire page, providing a smooth user experience.