React Js Compare Two Dates | Current Date

Compare dates in React or JavaScript. Whether you want to check if a date is in the past, present, or future, or if you want to calculate the difference between two dates, understanding how to compare dates in JS is crucial. There are various methods to compare dates in JavaScript, including using the built-in Date object or the React framework. In this article, we will explore some of the methods and provide examples of comparing dates in JavaScript, particularly in React
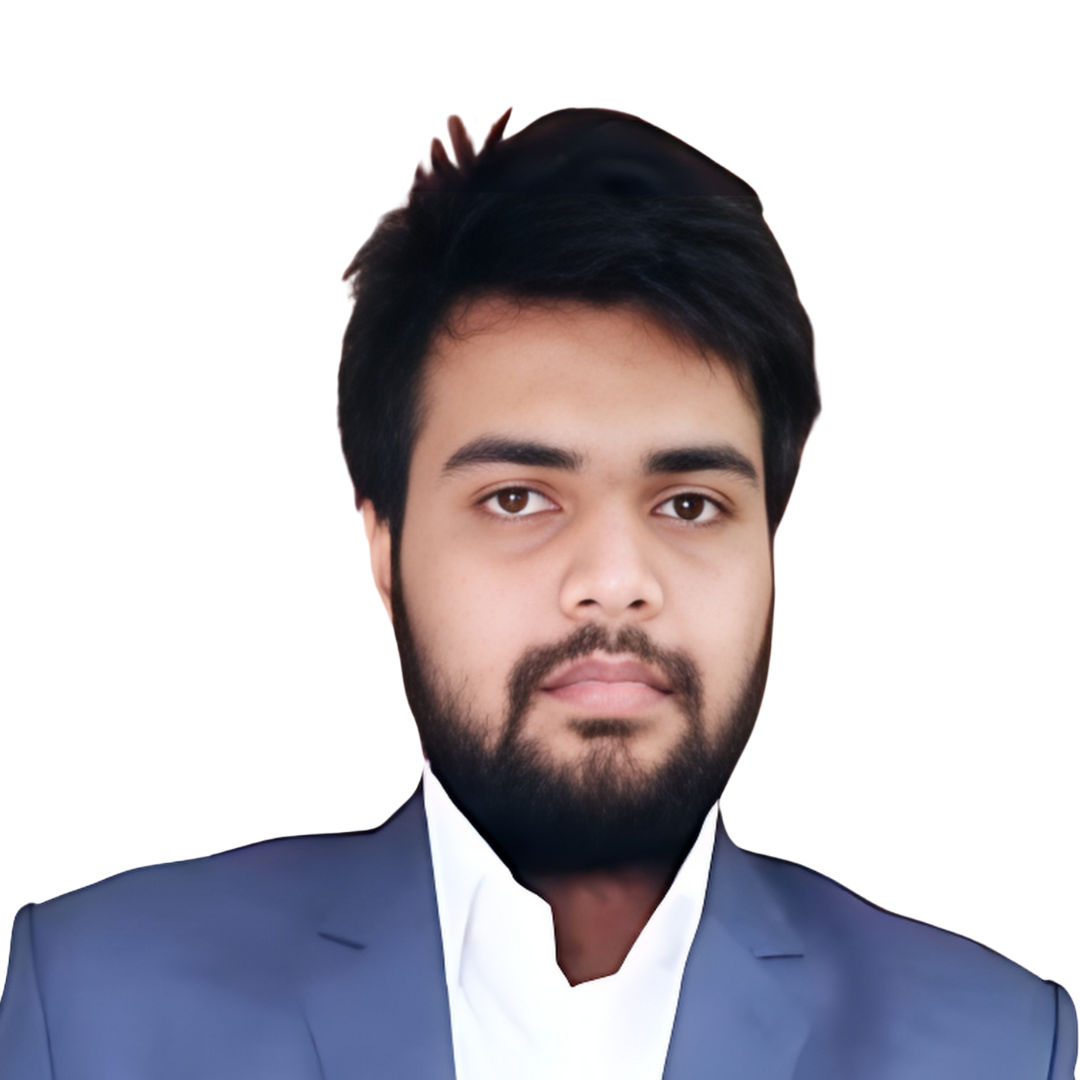
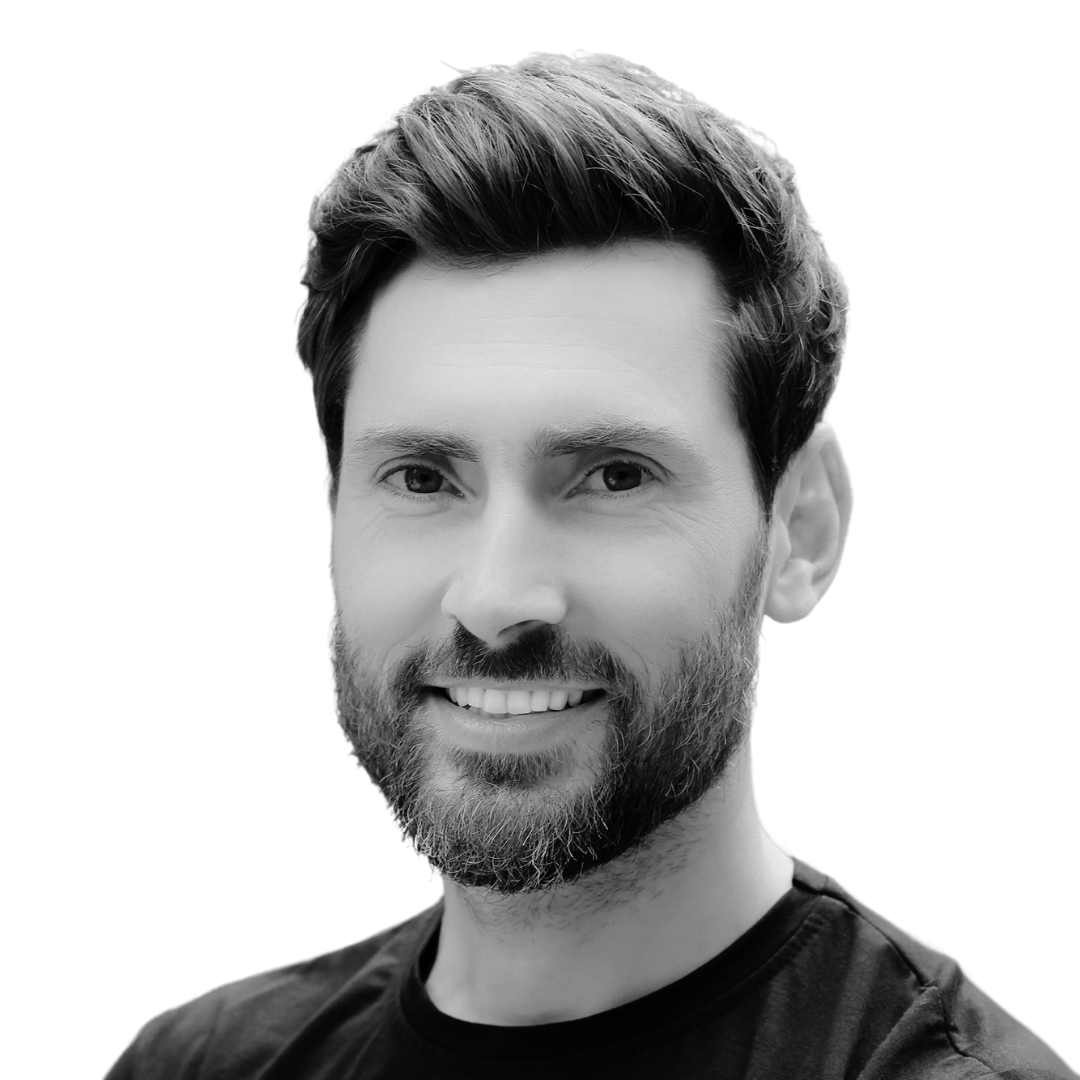
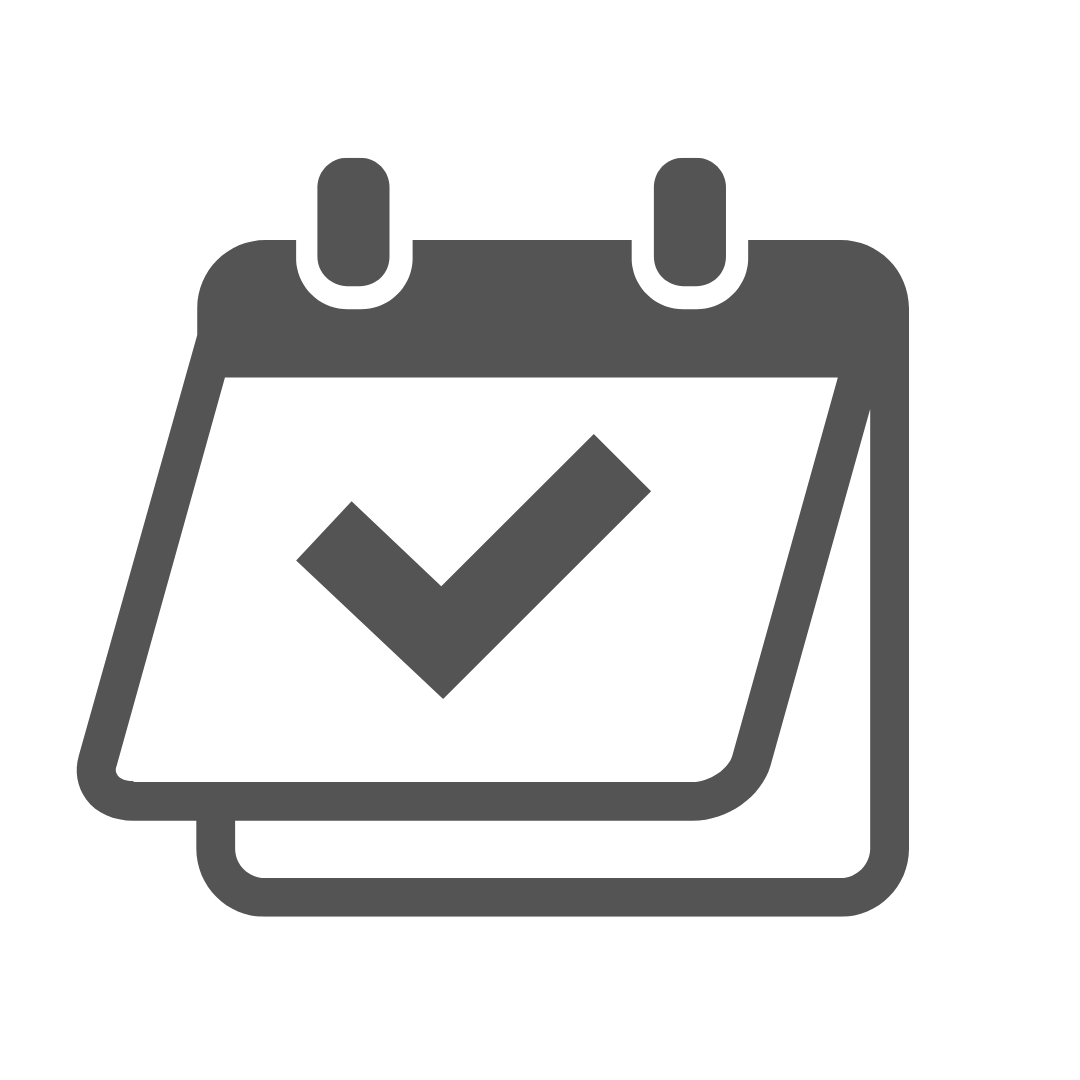
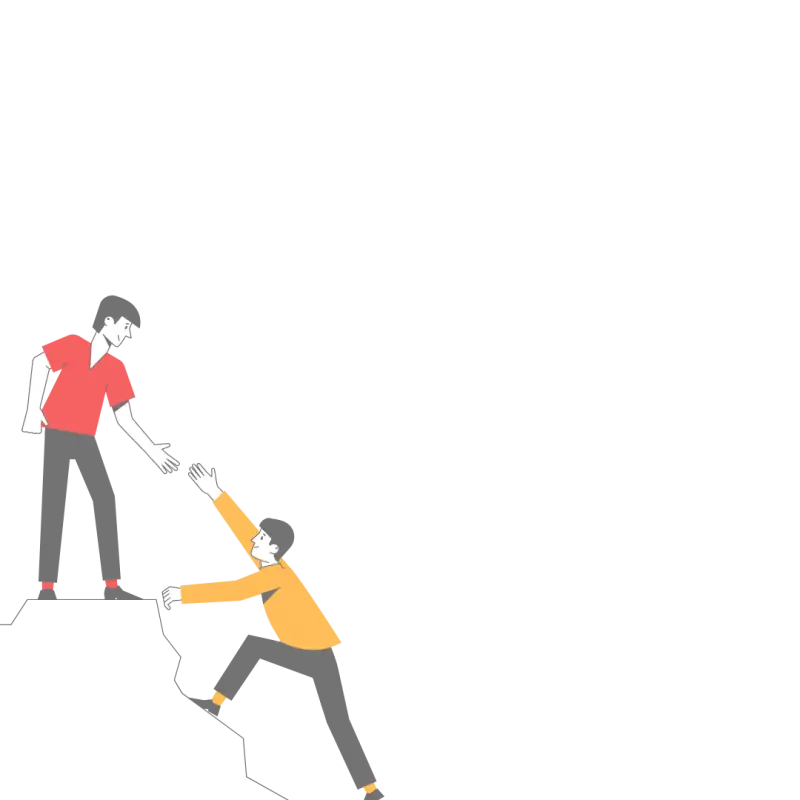
Thanks for your feedback!
Your contributions will help us to improve service.
How to Compare Dates in React?
React Js Compare Two Dates:In React.js, comparing dates typically involves using JavaScript's built-in Date object. You can create Date instances for the two dates you want to compare and then use comparison operators like <, >, or === to determine their relationship. For example, you can check if one date is greater than another to see if it occurs after it.
Output of Compare two Dates in React js
Date Comparison in Javascript Example
Output of Javascript Compare Two Dates
How to Compare Date with Current Date in Javascript?
Compare dates with the current date in JavaScript. This can be useful for validating user input, checking expiration dates, scheduling events, and more. Then, compare them using comparison operators. In this article, we will show you how to compare dates with the current date in JavaScript using different methods and examples