React Js Count Number of Vowels in a string

React Js Count Number of Vowels in a string:To count the number of vowels in a string using ReactJS, you can create a component that takes an input string, utilizes JavaScript's string manipulation methods, and then renders the vowel count. Use a state variable to keep track of the count as you iterate through the characters in the string. Filter and count the vowels (a, e, i, o, u) using a regular expression or simple conditional statements
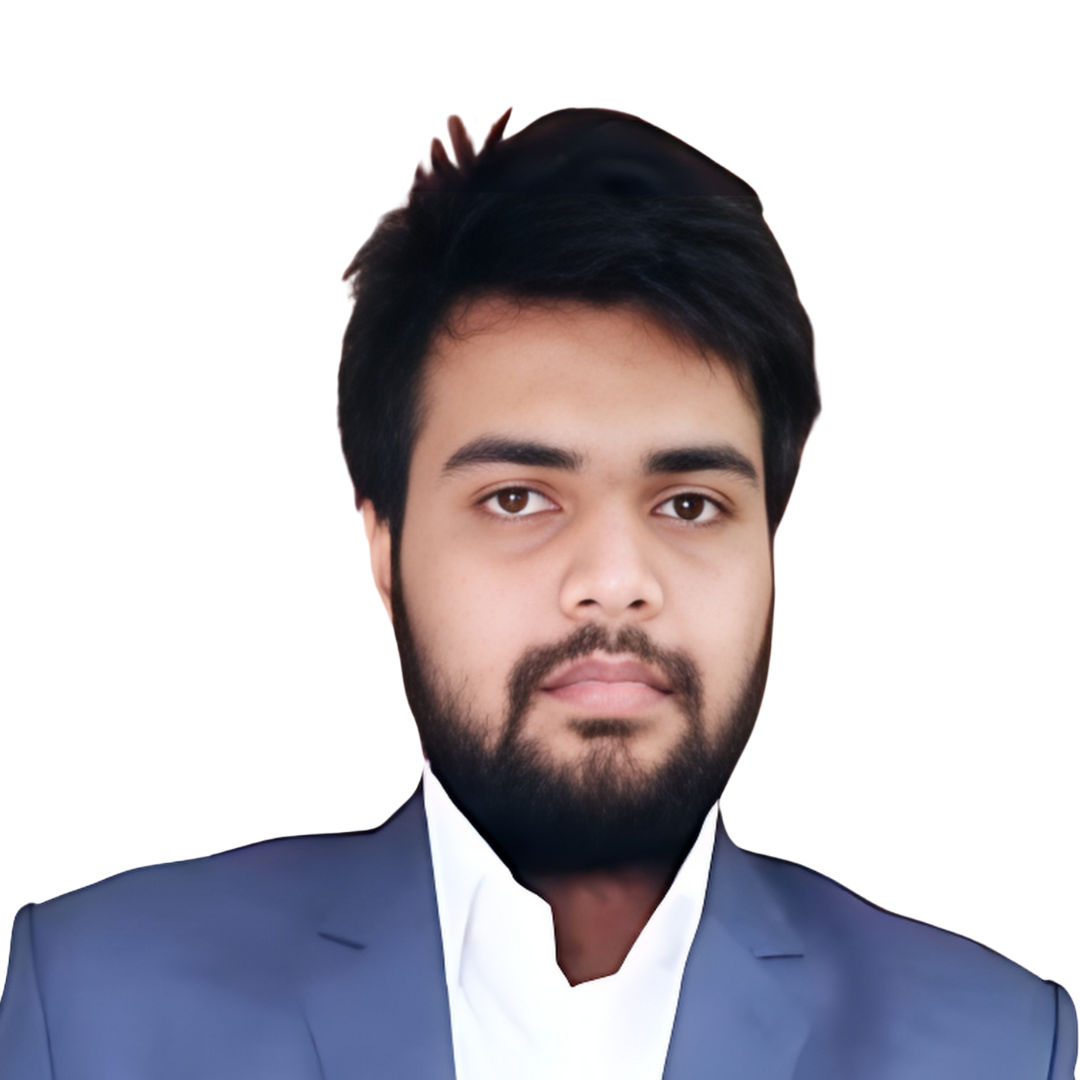
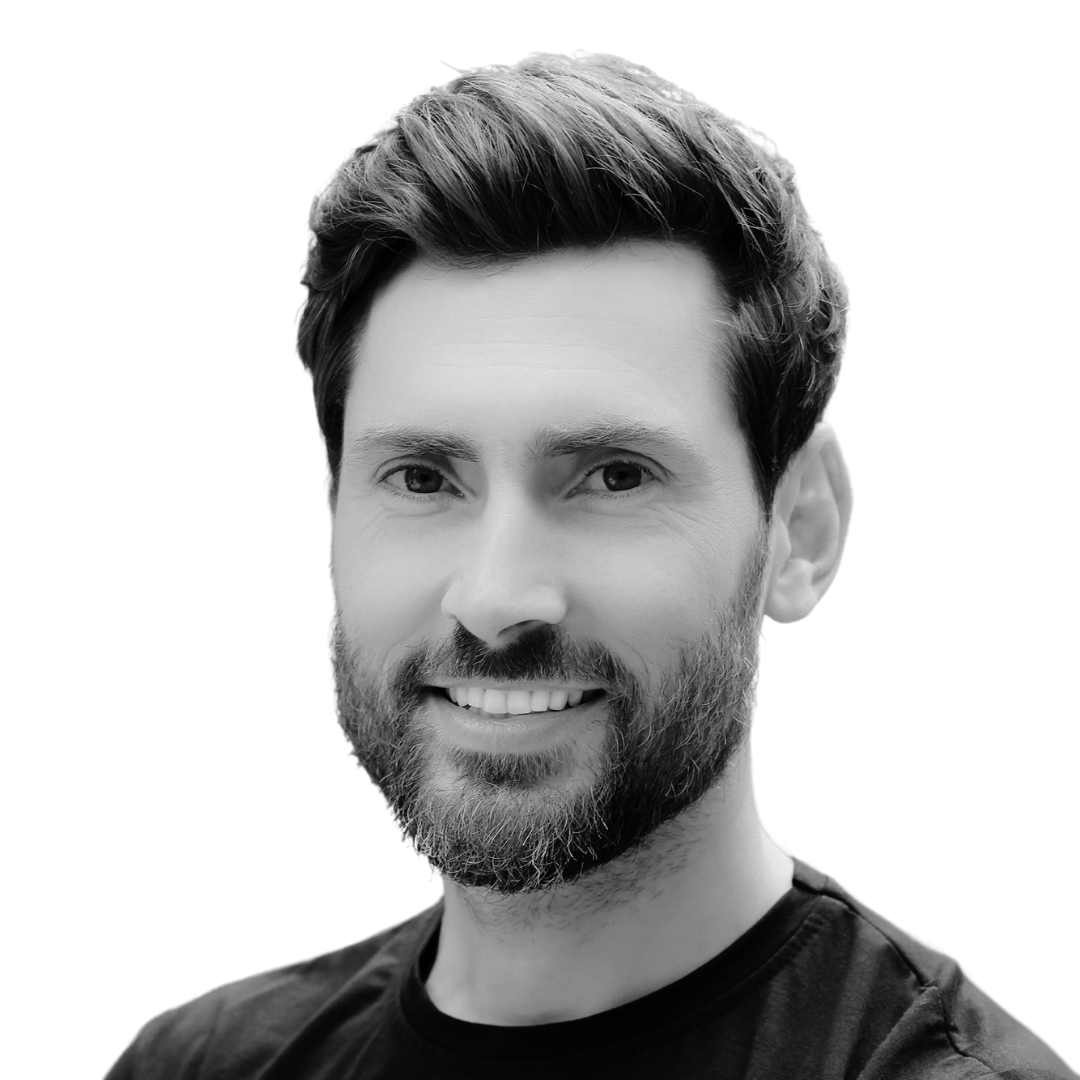
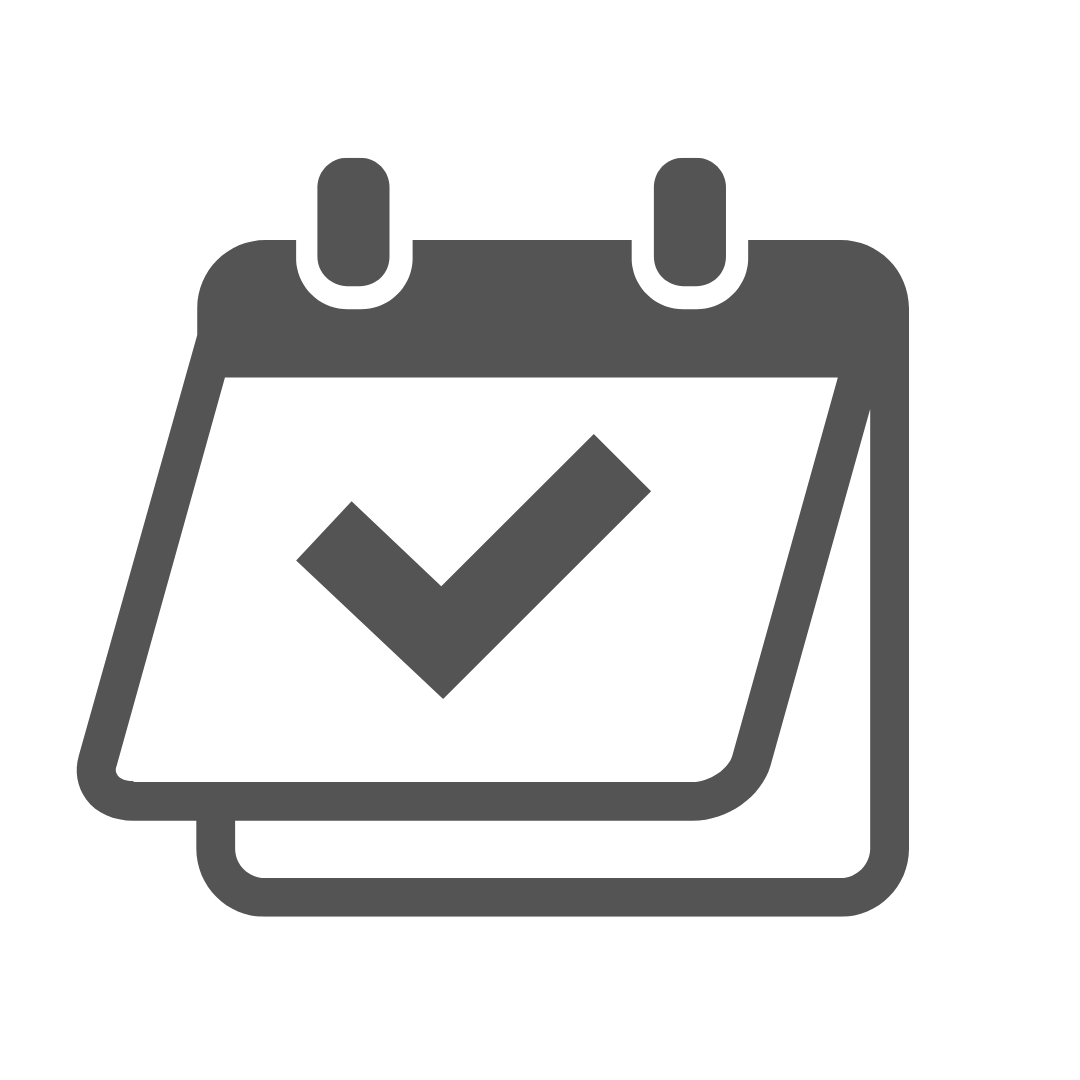
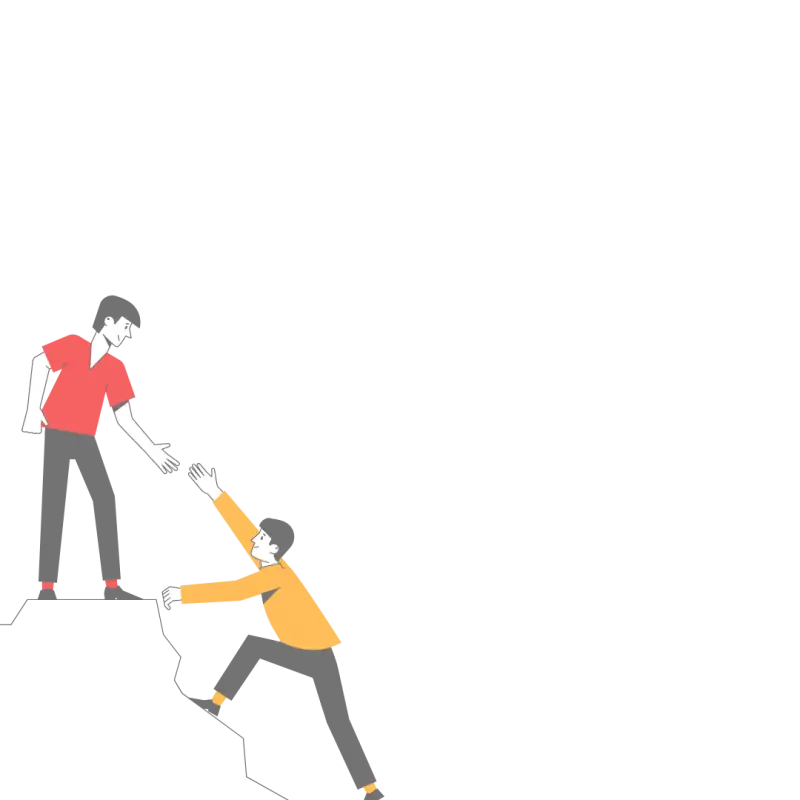
Thanks for your feedback!
Your contributions will help us to improve service.
Output of React Js Count Number of Vowels in a string
Setting Up the React Component:
We start by defining a functional React component called App
. We import the necessary hooks, useState
and useEffect
. The component's state consists of two variables:
inputText
: This holds the user's input string.vowelCount
: This holds the count of vowels in the input string
Counting Vowels:
We define a function countVowels
that takes the input text as an argument. Inside this function, we perform the following steps:
- Convert the input text to lowercase to make the search for vowels case-insensitive.
- Define a regular expression,
vowelPattern
, to match vowels (a, e, i, o, u). - Use the
match
method to find all occurrences of vowels in the lowercase text. - Check if
vowelMatches
isnull
(indicating no vowels found), and if not, retrieve the length of the matched array as the count.
Handling Input Changes:
We define a function handleInputChange
to handle changes in the input field. This function updates the inputText
state variable and then calls the countVowels
function to calculate the vowel count based on the new input text. Finally, it updates the vowelCount
state variable with the calculated count
Rendering the Component:
In the component's render method, we create a simple user interface with an input field where you can type your string. As you type, the number of vowels in the string is updated and displayed below the input field