React Js Remove First and last Character of String
React Js Remove First and last Character of String:To remove the first and last characters of a string in React.js, you can use JavaScript's substring
method. First, you get the input string, and then you create a new string by extracting a substring starting from the second character (index 1) up to the second-to-last character (length - 2)
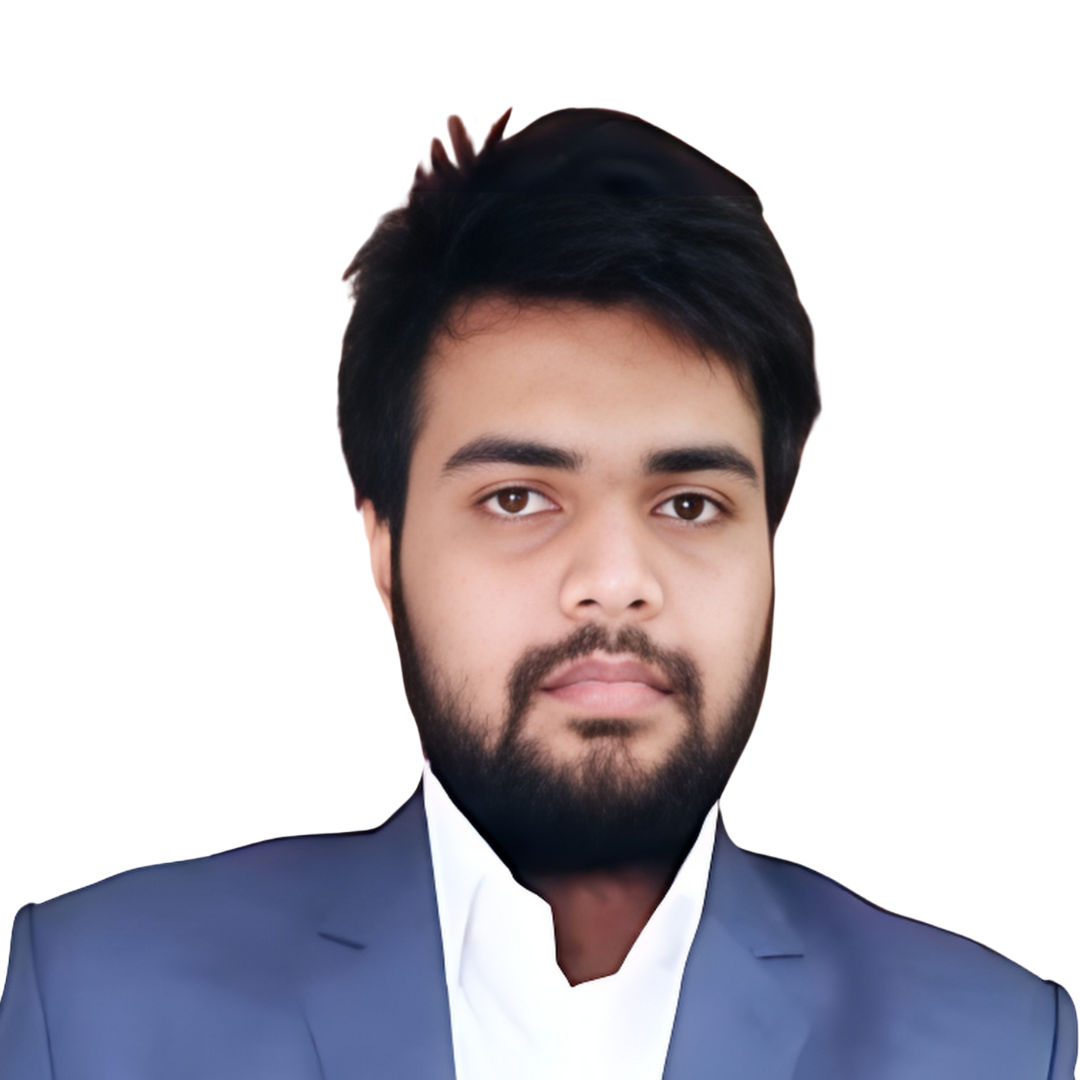
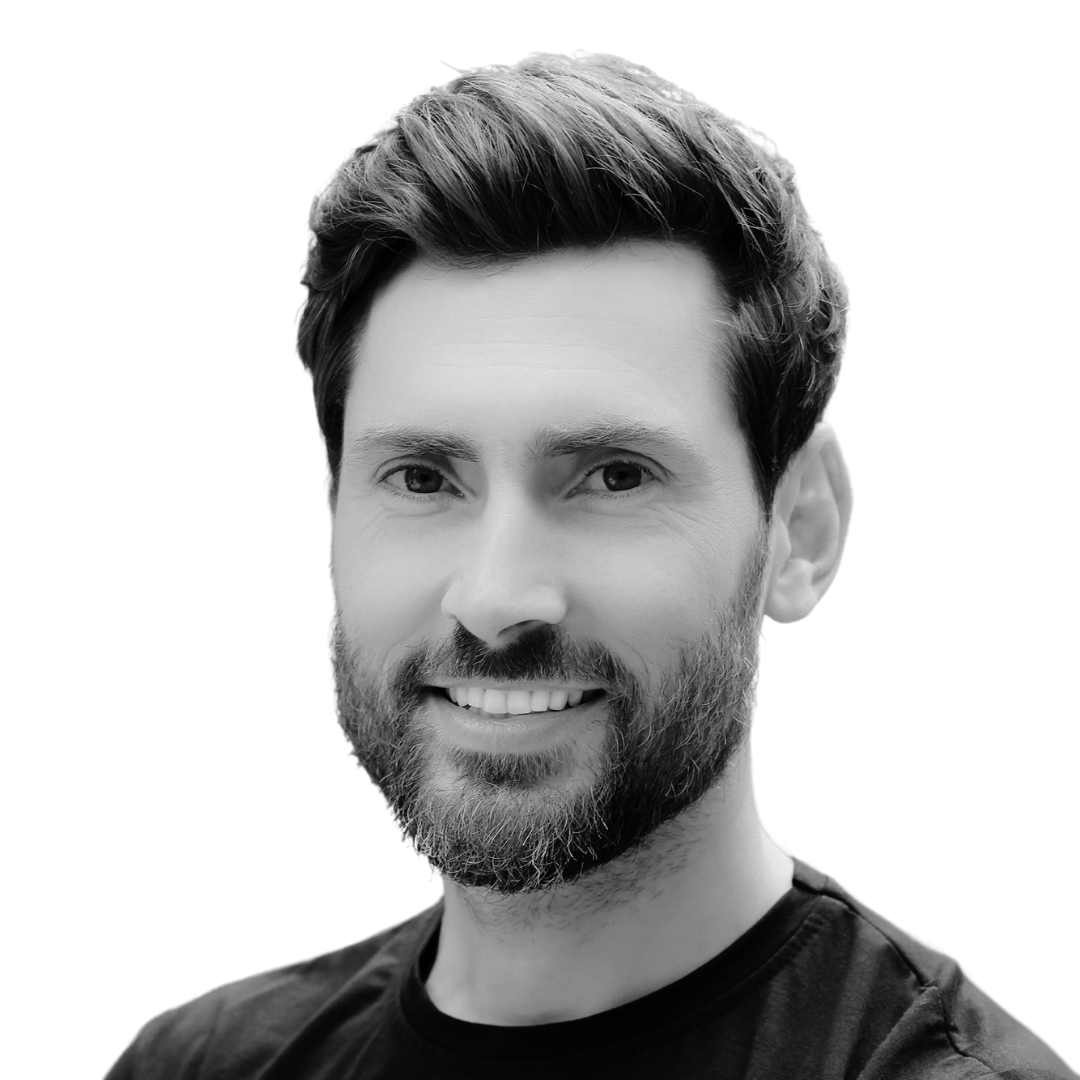
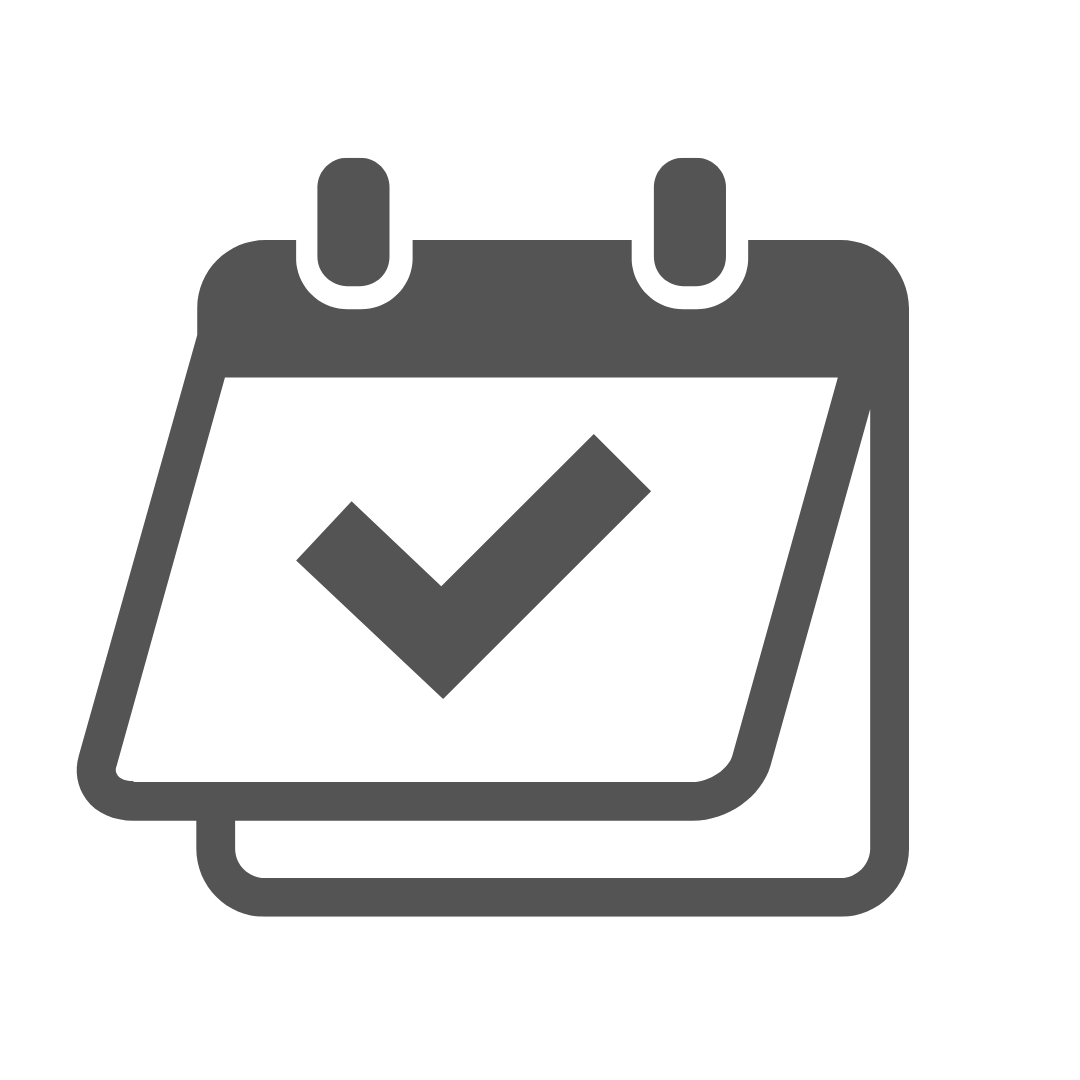
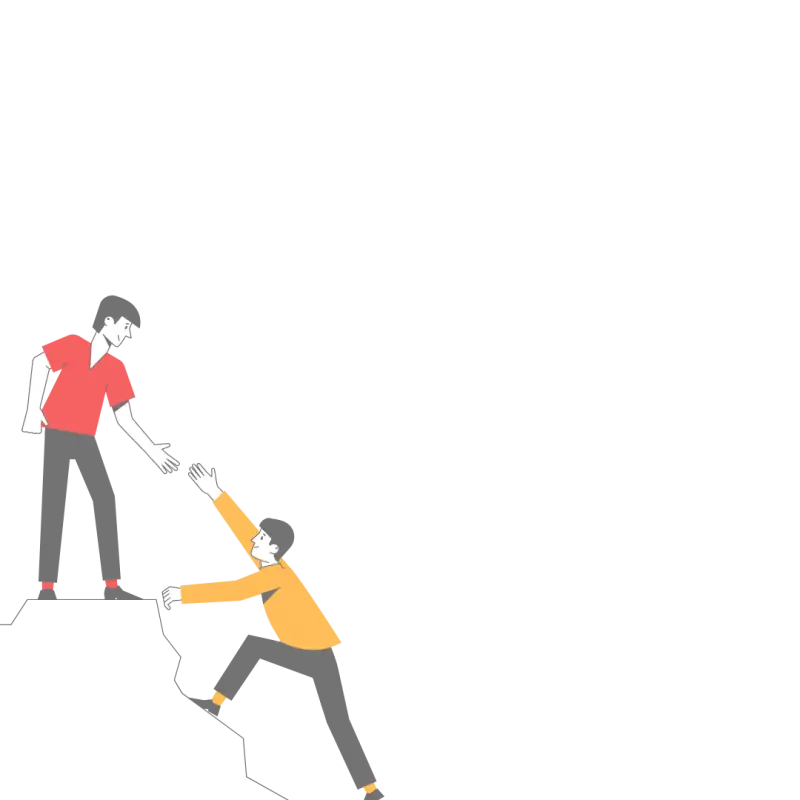
Thanks for your feedback!
Your contributions will help us to improve service.
How can you use Reactjs to remove the first and last characters of a string?
This React.js code defines a function, removeFirstAndLastCharacter
, which removes the first and last characters of a given string, originalString
. It checks if the string's length is less than 3 and returns the original string if so. Otherwise, it uses str.substring(1, str.length - 1)
to extract a substring that excludes the first and last characters. The result, modifiedString
, is displayed along with the original string in a React component, demonstrating the removal of the first and last characters from "/Hello, World!/".