React Js Enable Disable Textbox on Checkbox Value

React Enable Disable textbox on checkbox value: To enable/disable a textbox in React on checkbox click, you can create a state variable to keep track of the checkbox's status. In the checkbox's onChange event, you can update the state variable with the current checked status. Then, in the textbox's props, you can conditionally set the disabled attribute to true or false based on the state variable's value using a ternary operator.
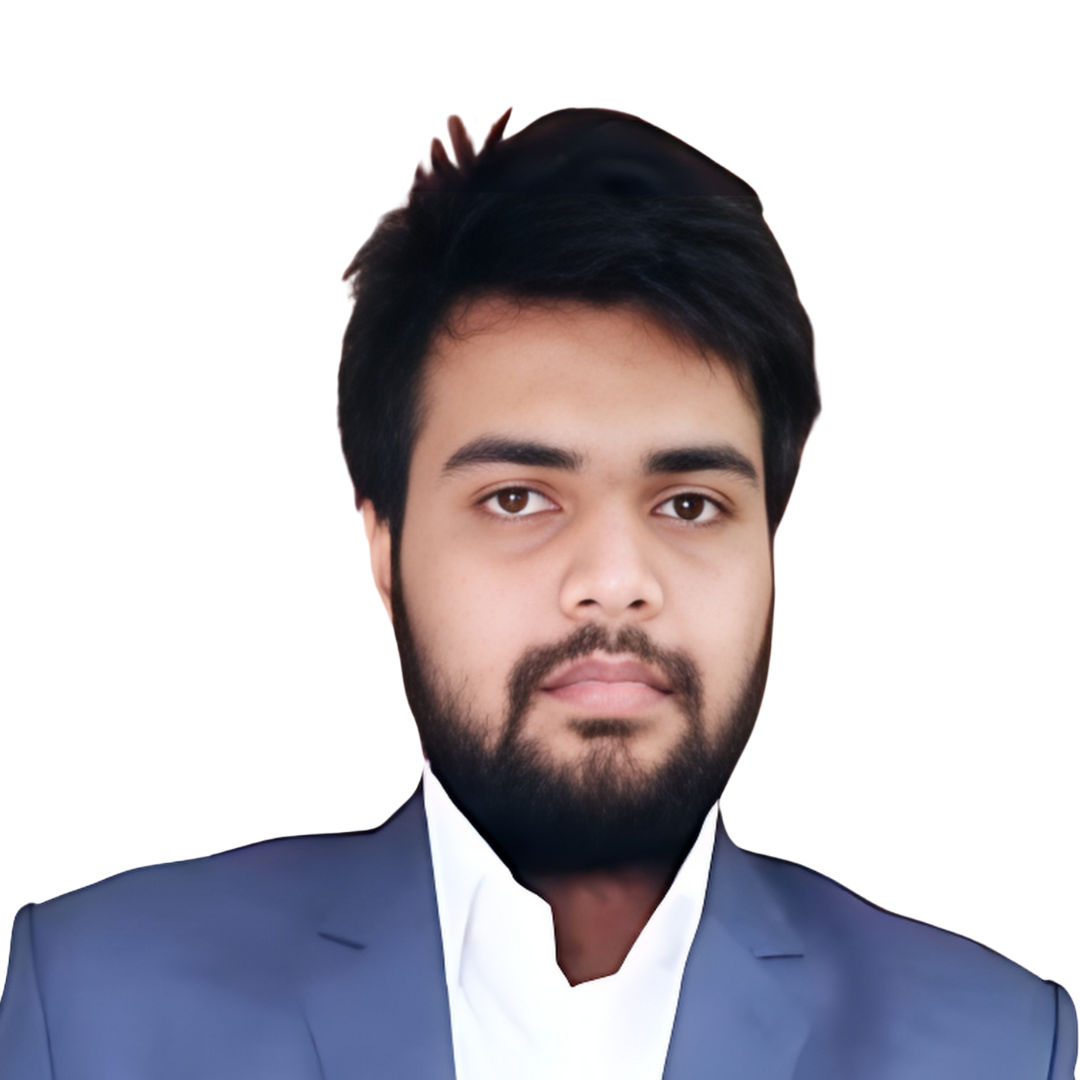
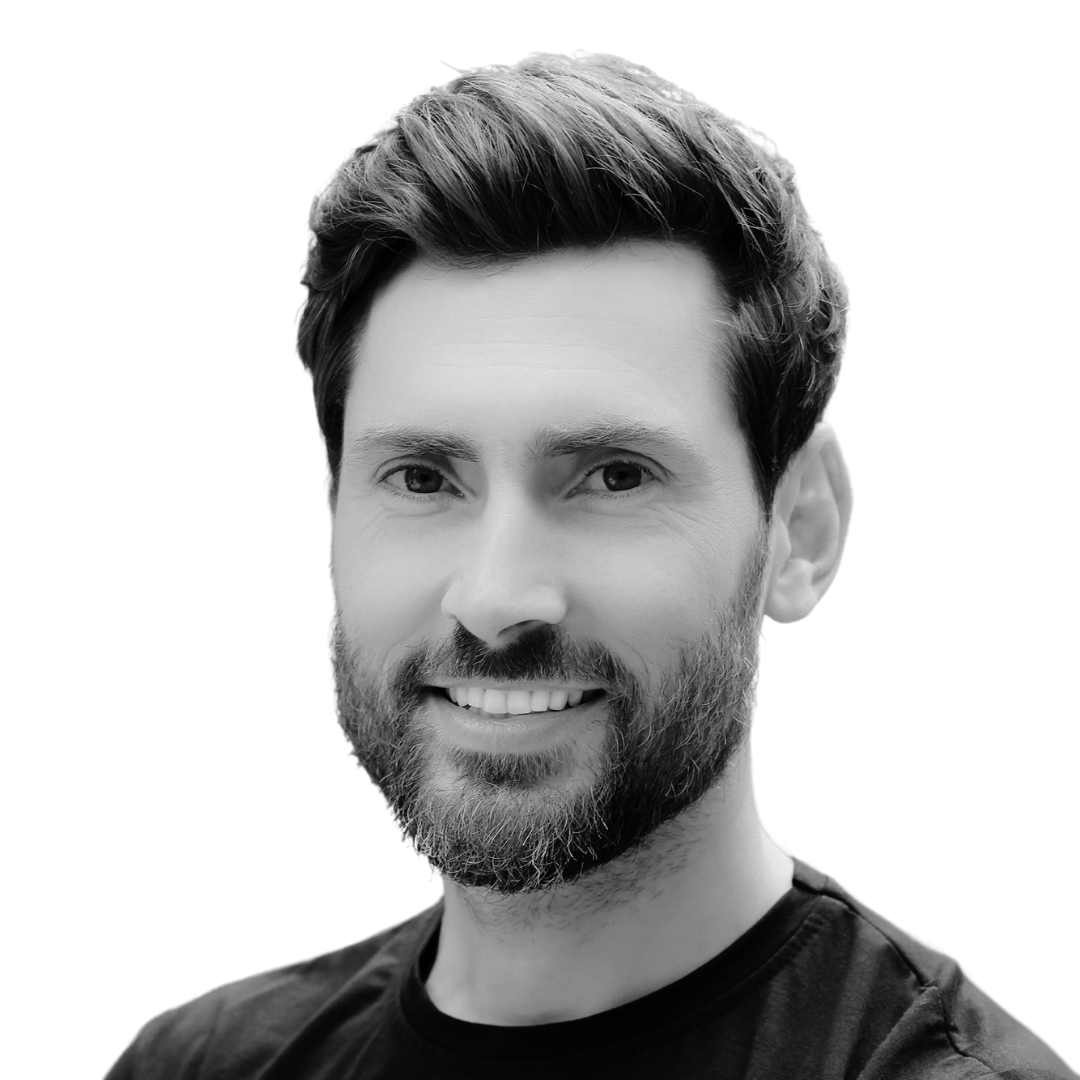
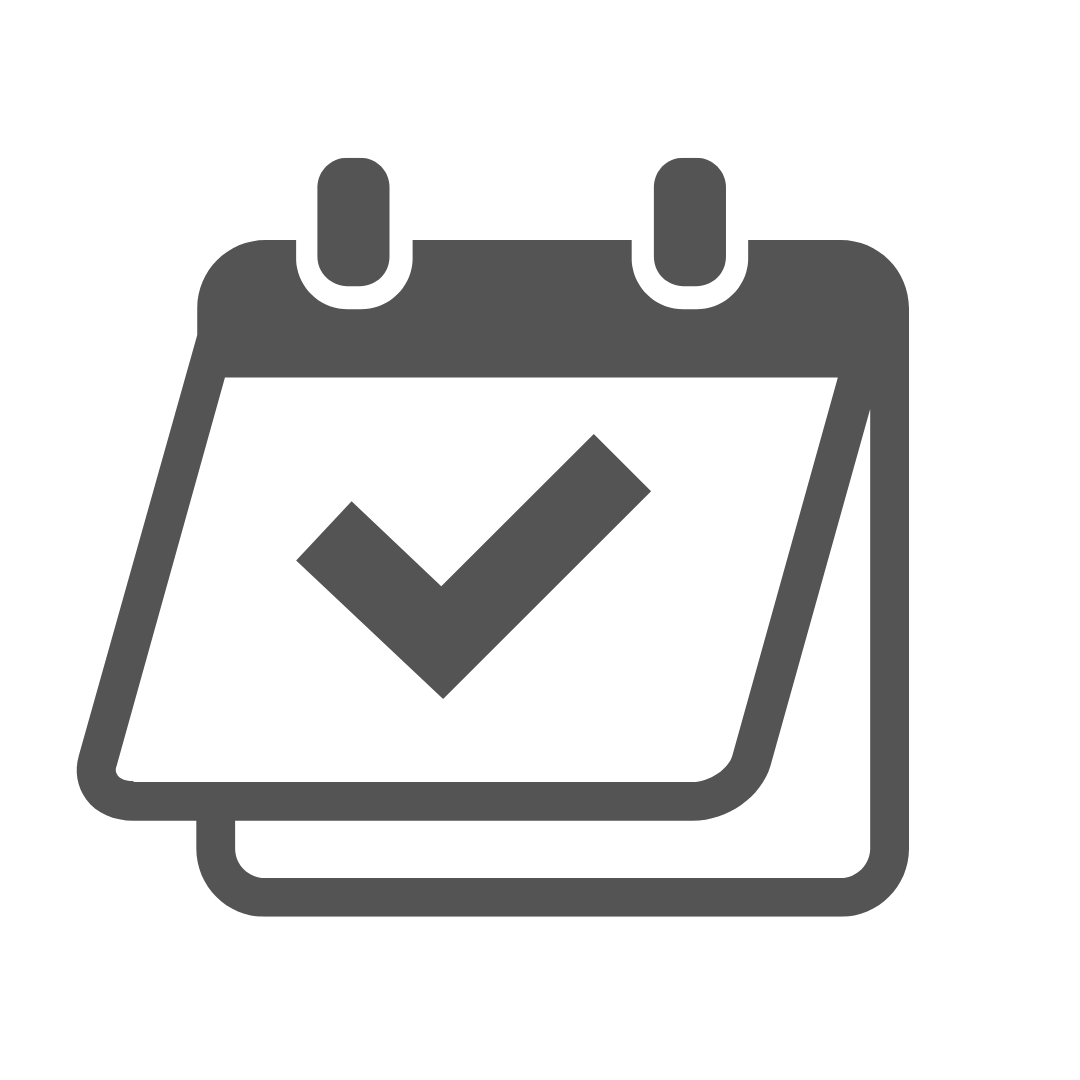
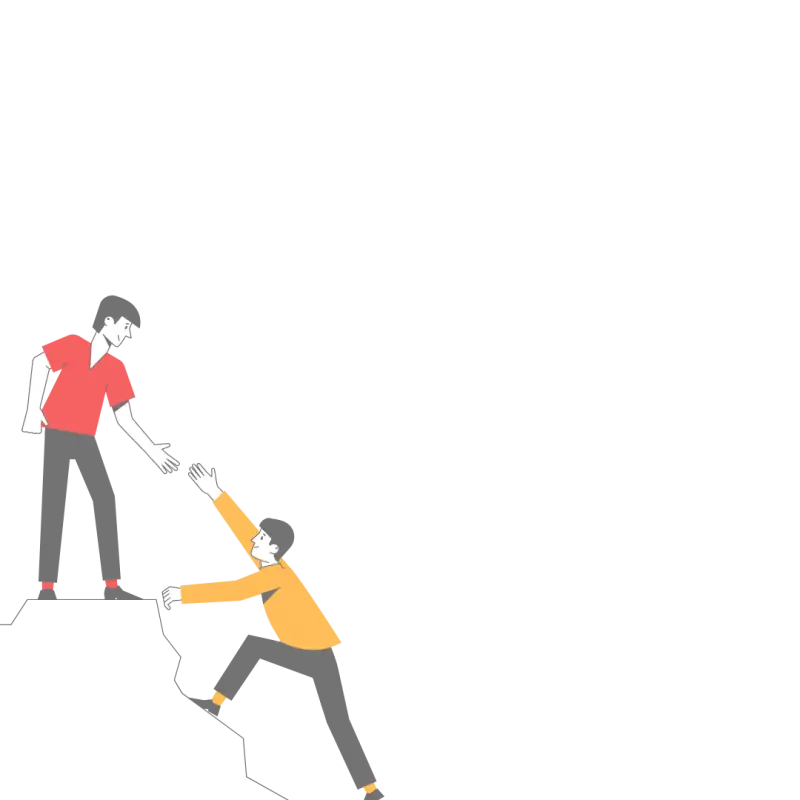
Thanks for your feedback!
Your contributions will help us to improve service.
How can I enable/disable a textbox in React based on a checkbox's click event?
This code is a React application that renders a container with a title, a checkbox, and an input field. The checkbox controls the "disabled" attribute of the input field, so when the checkbox is checked, the input field is disabled, and when the checkbox is unchecked, the input field is enabled.
Here is a breakdown of the code:
-
const { useState } = React;
: This line imports theuseState
hooks from the React library. These hooks are used to manage state and side effects in React components. -
function App() { ... }
: This defines theApp
component, which is a functional component in React. TheApp
component returns JSX, which is a syntax extension of JavaScript that allows you to write HTML-like code within your JavaScript code. -
const [disabled, setDisabled] = useState(false);
: This line uses theuseState
hook to create a state variabledisabled
with an initial value offalse
. ThesetDisabled
function is used to update the value of thedisabled
state variable. -
<input type="checkbox" checked={disabled} onChange={(e) => setDisabled(e.target.checked)} />
: This line renders a checkbox input element. Thechecked
prop is set to the value of thedisabled
state variable, which means that the checkbox will be checked whendisabled
istrue
and unchecked whendisabled
isfalse
. TheonChange
prop is a callback function that updates the value of thedisabled
state variable when the checkbox is checked or unchecked. -
<input type="text" disabled={!disabled} />
: This line renders a text input element. Thedisabled
prop is set to the opposite of thedisabled
state variable, which means that the input field will be disabled whendisabled
istrue
and enabled whendisabled
isfalse
.
Output of React Enable Disable textbox on checkbox click