React Js String Substr Method
React Js String Substr Method:In React.js, the substr()
method is not specific to React, but rather a built-in JavaScript string method. It allows you to extract a portion of a string based on a starting index and an optional length. The substr()
method takes two parameters: the starting index and the optional length. If the length is not specified, it extracts the remaining characters from the starting index to the end of the string. For example, myString.substr(2, 4)
would extract a substring starting from the third character and include the next four characters. The substr()
method can be useful for manipulating and extracting parts of strings in React.js applications.
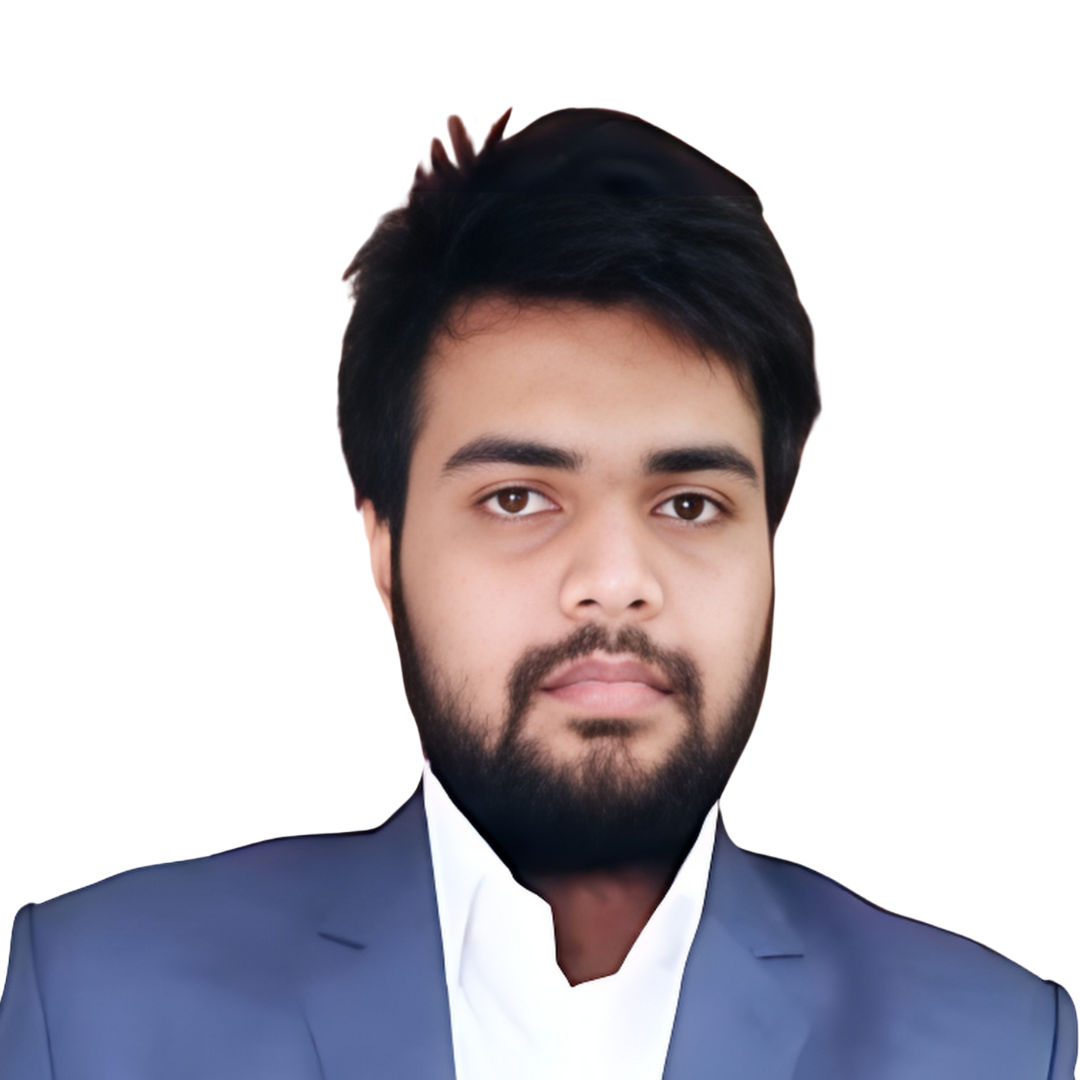
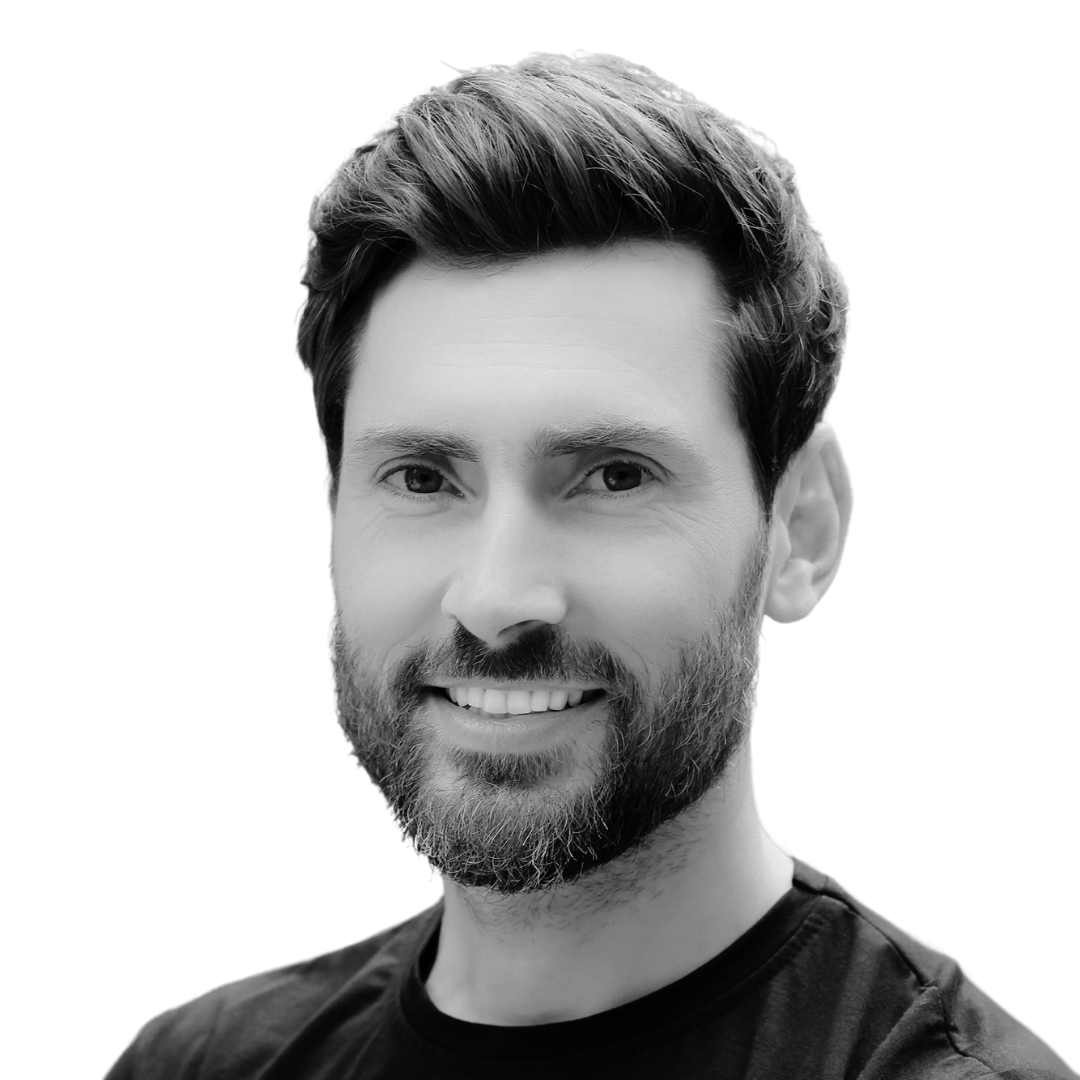
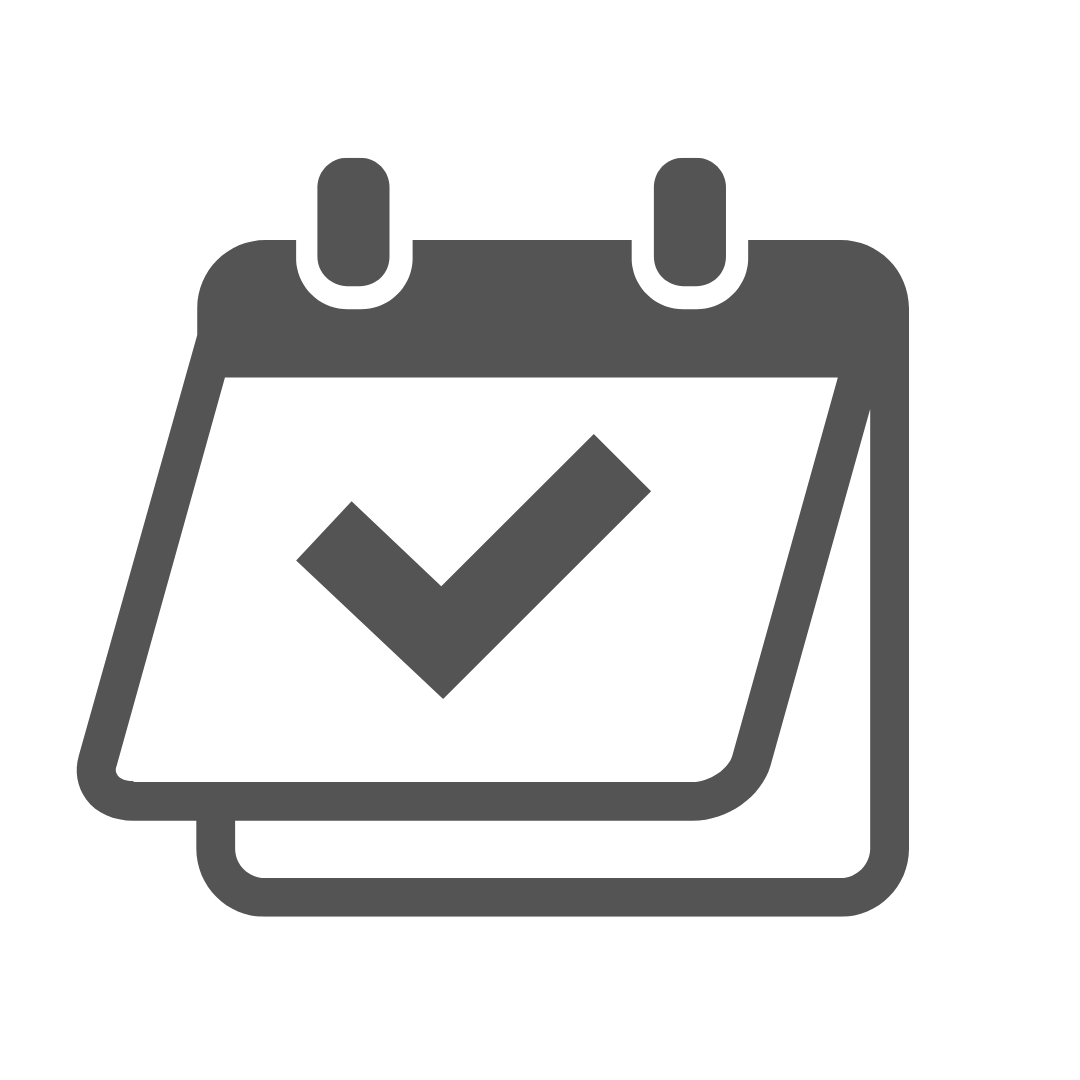
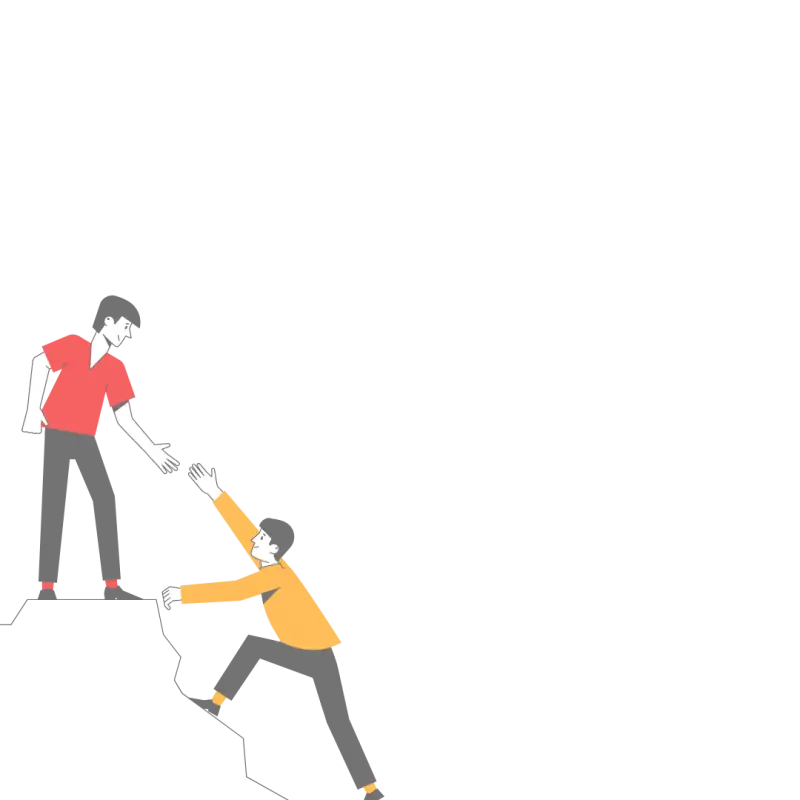
Thanks for your feedback!
Your contributions will help us to improve service.
What is an example of using the `substr` method in React.js for manipulating strings?
The code snippet demonstrates the use of the substr()
method in React.js. It defines a functional component App()
that sets a string myString
to 'font awesome icons'. The substr()
method is then used to extract a substring from the original string, starting from index 5 and including 7 characters.
Output of React Js String Substr Method
How can I extract a substring from the beginning of a string in React.js?
In the given React JS code snippet, a substring is extracted from the beginning of a string. The original string is 'fontawesomeicons', and the substring is obtained using the substr
method. The substr
method takes two parameters: the starting index (0 in this case) and the length of the substring (5 in this case).
How can I extract a substring in React.js using a negative starting index?
In the provided React JS code, a substring is extracted from a given string using a negative starting index. The string is 'Hello, World!', and the substring is obtained using the substr()
method with a negative value of -6. By using a negative index, the counting starts from the end of the string. The extracted substring, 'World!', is then displayed on the webpage using JSX syntax.
How can I extract a substring in React.js when the desired length is longer than the remaining characters in the original string?
In the given React.js code snippet, a substring is extracted from a string using the substr
method. The original string is "Hello, World!" and the substring is extracted starting from index 7 with a length of 20. However, since the remaining characters in the string are less than the specified length, the substring is limited to the available characters. The extracted substring, in this case, is 'World!'
React Js Extracting substring with a longer length than the remaining characters
How can I extract a substring from a variable in React.js?
In the given React.js code, a substring is extracted from a variable called name
. The substr()
method is used to extract a portion of the string starting from a specified index (startIndex
) until the end of the string. In this case, the substring extracted from name
is 'Doe'
, starting from index 5. The resulting substring is displayed in the rendered output of the React component.
How can I use the `substr()` method within a React component to display a formatted date?
This React.js component utilizes the substr()
method to display a formatted date. The substr()
function is used to extract a portion of a string based on specified indices. In this case, it extracts the day, month, and year from the given date
string and formats it as '21/06/2023'. The formatted date is then displayed within the component alongside the original date.