React Js Table Data Sort Example
React Js Table Data Sort Example:In a Reactjs table data sorting example, we can implement multiple sorting options. Users can sort data by various criteria such as ID, name, date, price, and gender. By clicking on the respective column headers, JavaScript functions can be triggered to reorganize the table data accordingly. Sorting can be done in ascending or descending order, offering users a dynamic and organized view of the data. React's state management can track sorting preferences, and the UI can be updated in real-time, providing a seamless user experience for navigating and exploring tabular data.
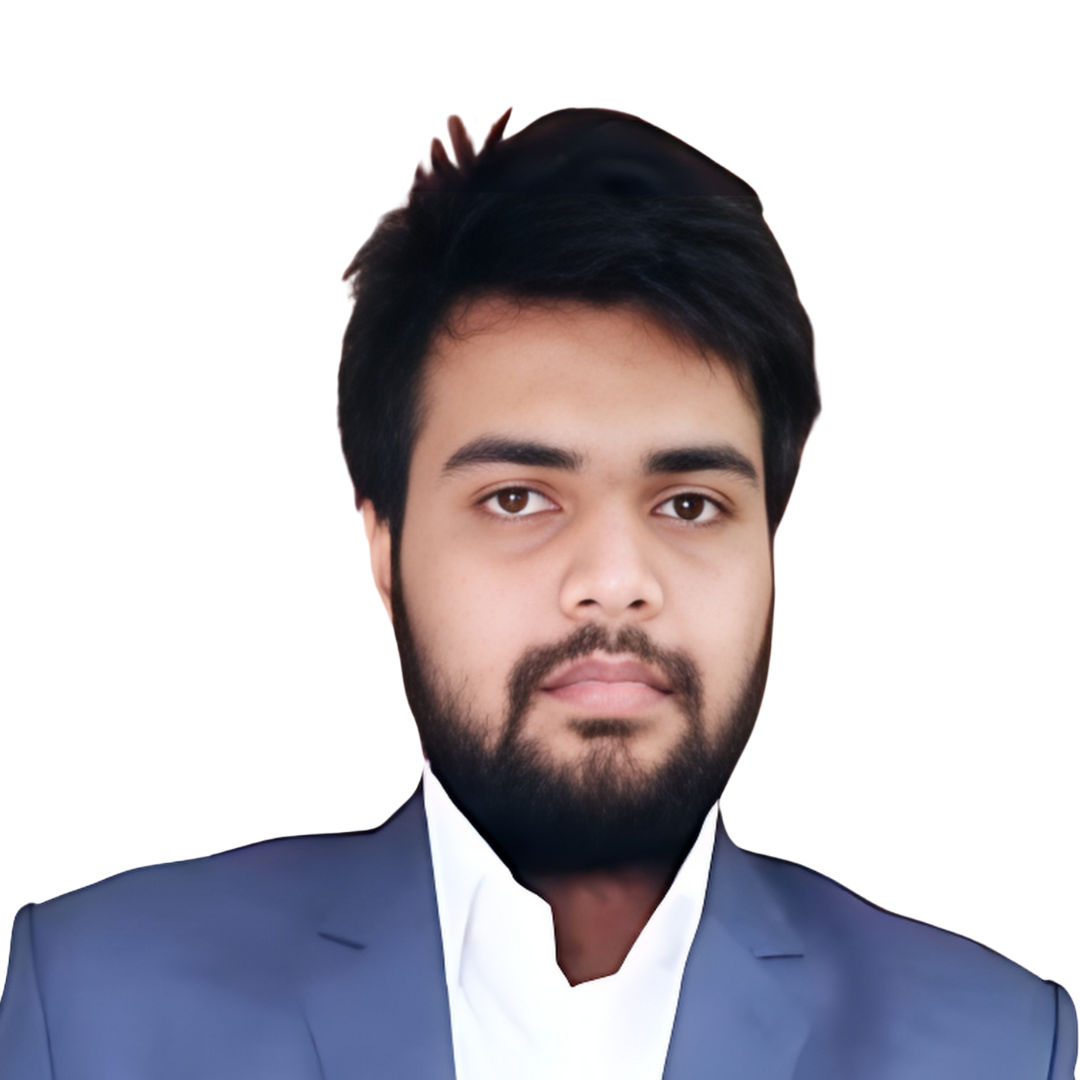
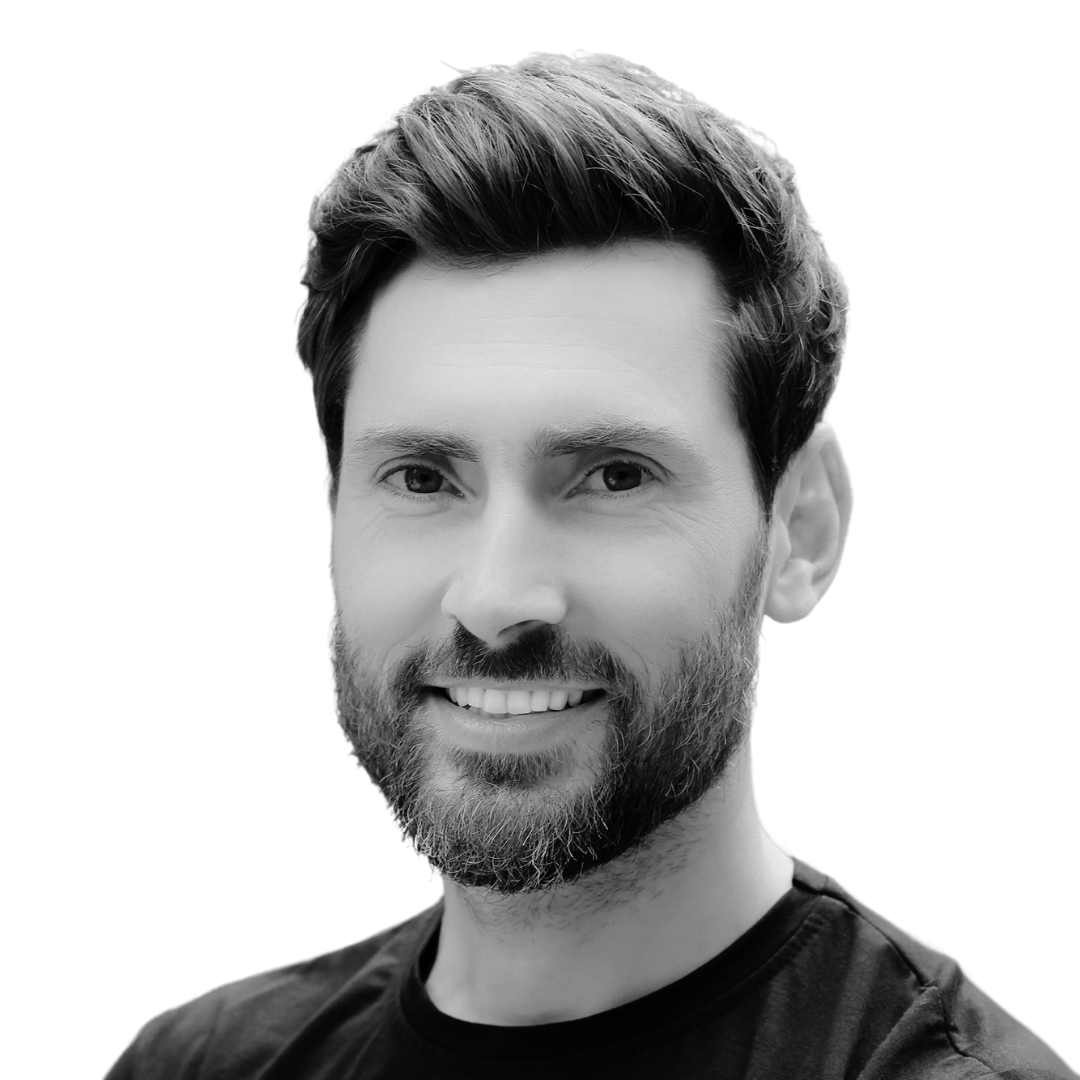
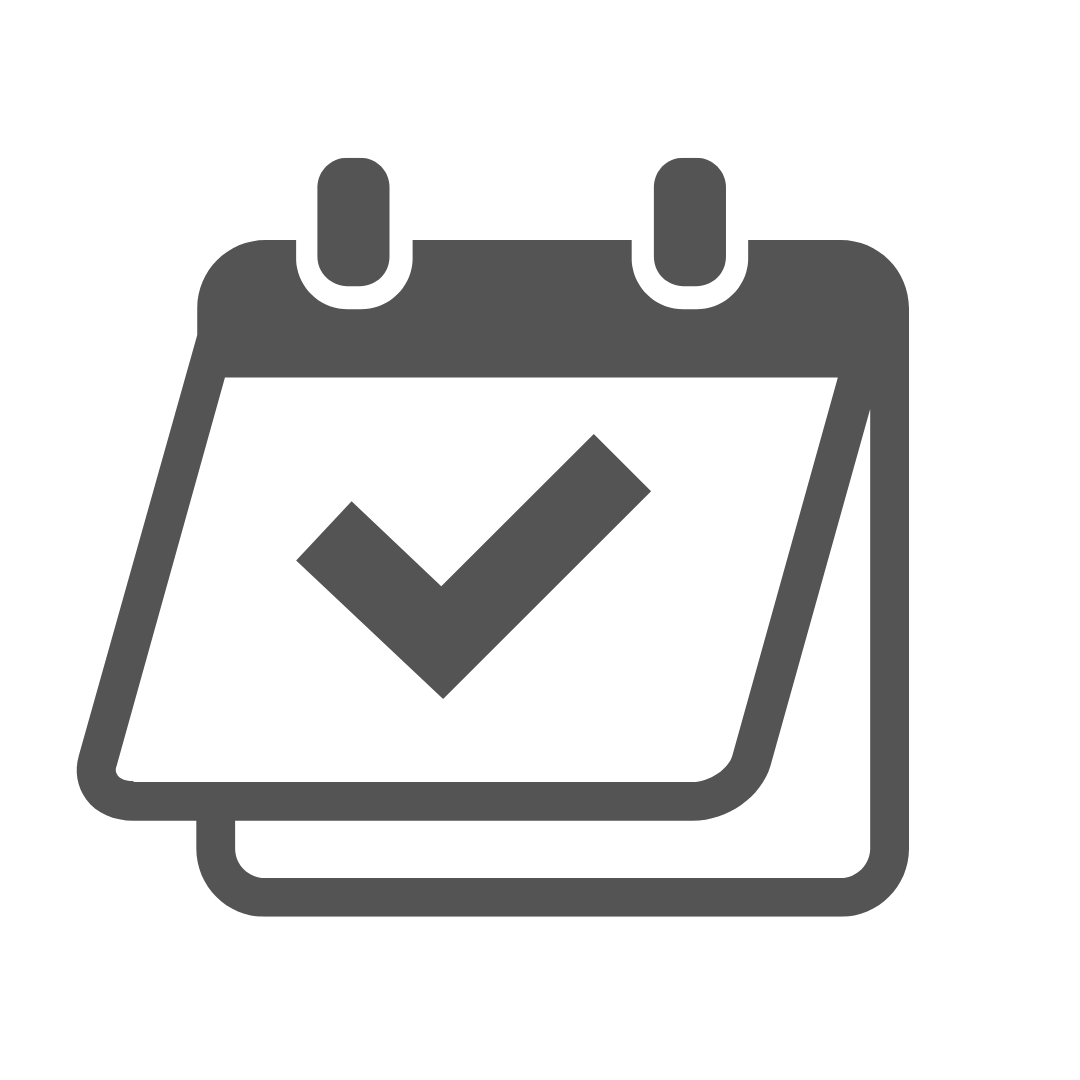
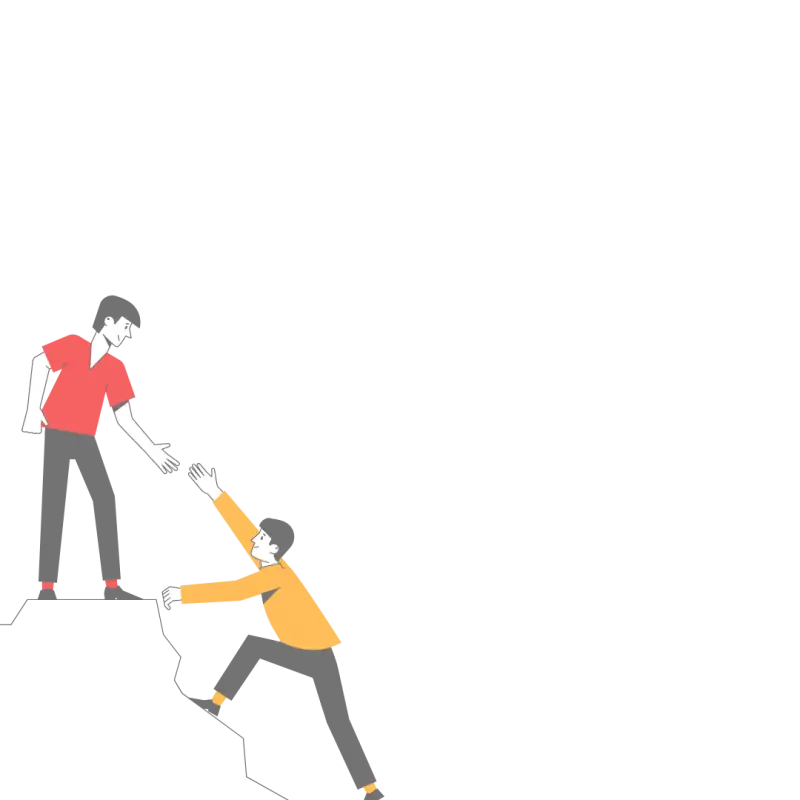
Thanks for your feedback!
Your contributions will help us to improve service.
How can I implement table sorting by name in a React js application?
This React.js code defines a simple table component that displays data with columns "ID" and "Name." It utilizes React hooks, specifically useState
, to manage the data and sorting functionality. Initially, it renders data in ascending order by name. When the "Sort by Name" button is clicked, it toggles between ascending and descending order by using the localeCompare
function to sort the data. The sorted data is then updated, and the table is re-rendered accordingly. This allows users to easily sort the table by name in ascending or descending order with a click of the button.
Output of React js Table Sort By Name
How do you sort a table by 'Id' in React js?
This React.js code creates a sortable table displaying product data with IDs. It initializes with sample data and two state variables: data
for the product data and sortOrder
to track sorting order. When the "Sort by ID" button is clicked, it sorts the data by ID either in ascending or descending order and updates the UI accordingly. The table's header contains columns for ID and Product, and the data is mapped into rows. Clicking the button toggles between ascending and descending sorting. This code illustrates a simple React component for sorting and displaying data in a table based on the product IDs.
Output of React js Table Sort By Id
How to implement date sorting in a table using Reactjs?
This React.js code creates a sortable table of data items, each with an ID, name, and date. Initially, the data is displayed in ascending order based on the date. Clicking the "Sort by Date" button toggles between ascending and descending order. The data is sorted using JavaScript's sort
method and the Date
objects, with the sorted result updating the component's state. The table's content is dynamically rendered based on the sorted data. This code leverages React's state management and event handling to create a user-friendly date sorting feature in a web application.
Output of React js Table Sort By Date
How do I enable table sorting by price in a Reactjs application?
This Reactjs component that renders a table of products and allows users to sort the products by price in ascending or descending order. It looks like you've done a good job with the code, but I'll provide a brief explanation of how it works:
-
Import React and useState: You import the necessary modules from React, including
useState
, which you use to manage the component's state. -
Define an array of initial products: You create an array called
initialProducts
, which contains some sample product data, includingid
,name
, andprice
for each product. -
Create the
App
component: Inside theApp
function component, you set up the component's state usinguseState
.products
holds the list of products, andsortOrder
keeps track of the current sorting order ("asc" for ascending or "desc" for descending). -
Define a function to toggle the sorting order: The
toggleSortOrder
function is called when the "Price" header in the table is clicked. It toggles thesortOrder
state between "asc" and "desc" and then sorts theproducts
array accordingly. When sorting in ascending order, it sorts bya.price - b.price
, and in descending order, it sorts byb.price - a.price
. -
Render the table: In the
return
section, you render a table with three columns: "ID," "Name," and "Price." The "Price" column header has a clickable element that invokes thetoggleSortOrder
function to change the sorting order. -
Map over the products: You map over the
products
array and generate table rows for each product. The product data is displayed in each row, including ID, name, and price.
Output of React js Table Sort By Price
How do I create a gender-based sorting functionality for a table in Reactjs?
This React.js code creates a sortable table by gender. It initializes a dataset containing names, ages, and genders. When the "Sort by Gender" button is clicked, it toggles between ascending and descending sorting orders based on gender. It updates the displayed data accordingly. The data is initially sorted in ascending order. The table renders the sorted data, with columns for Name, Age, and Gender. The code demonstrates how to use React hooks like useState to manage state and provides a simple example of sorting data in a React application based on a specific column (gender) and direction (ascending or descending).