React Js Array findIndex() Method
React Js Array findIndex() Method:The findIndex()
method in React.js is a function that operates on arrays and is used to find the index of the first element in the array that satisfies a given condition. It takes a callback function as an argument, which is executed for each element in the array until the condition is met.
If the condition returns true, the index of that element is returned. If no element satisfies the condition, the method returns -1. This method is commonly used in React.js applications when you need to find the index of a specific element in an array to perform further operations.
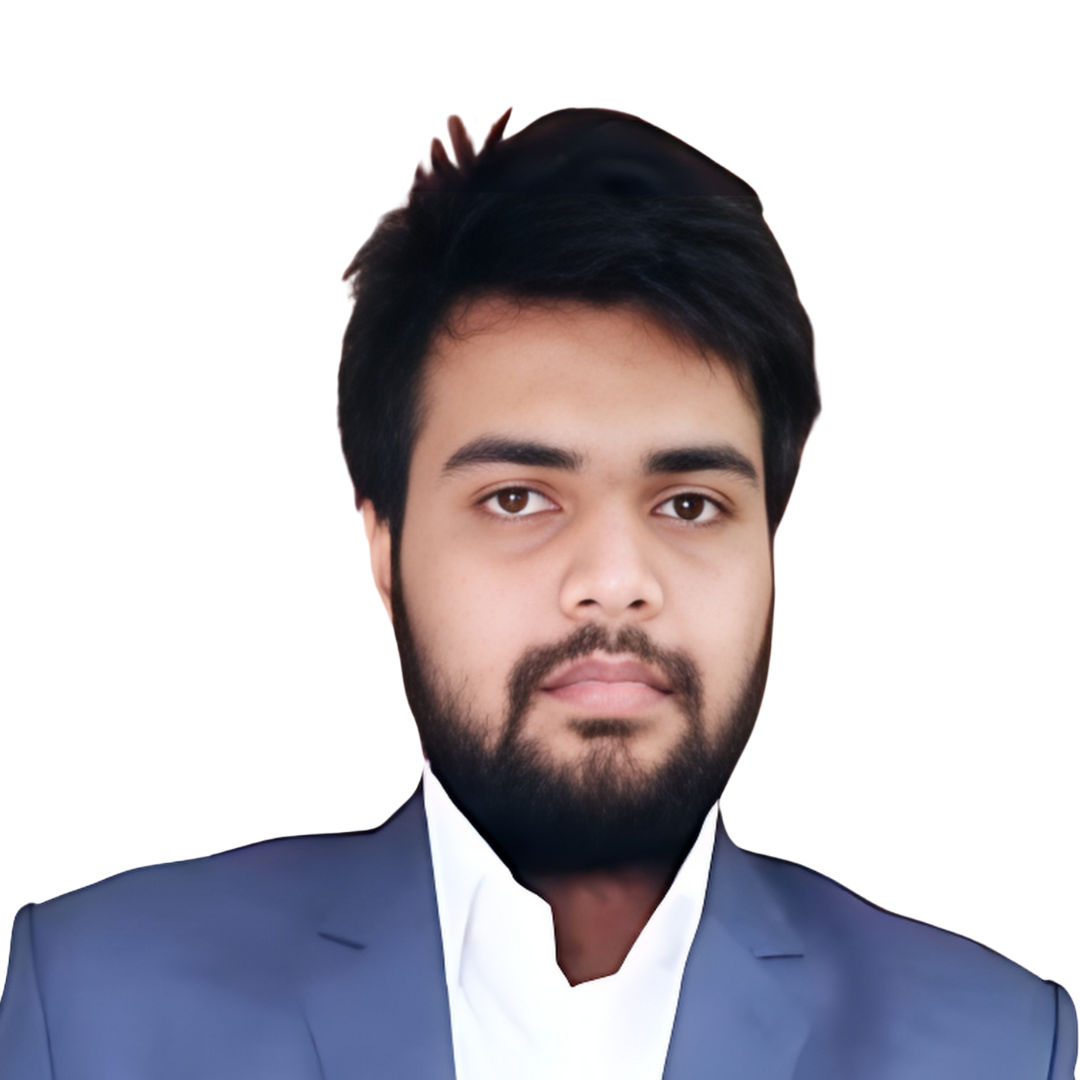
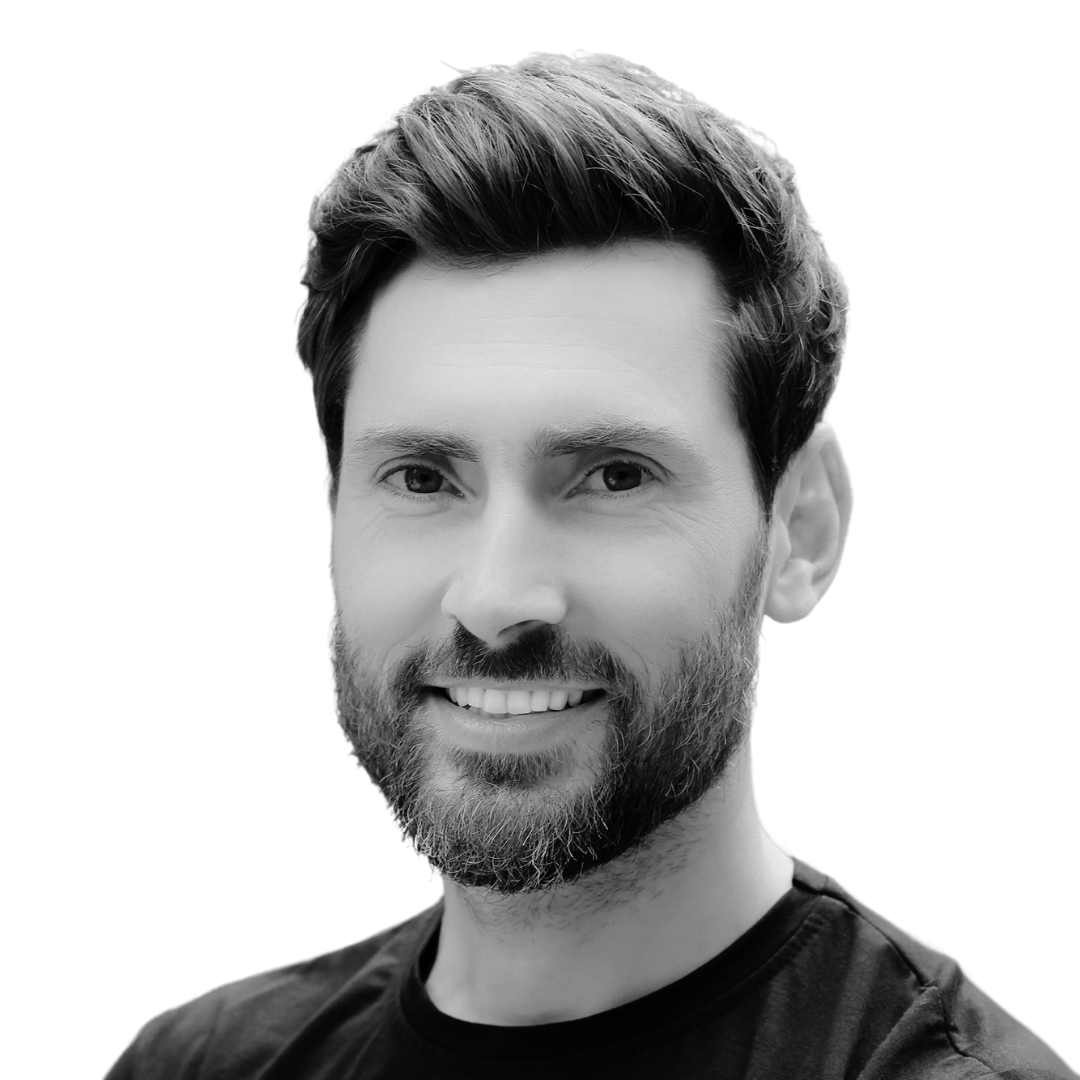
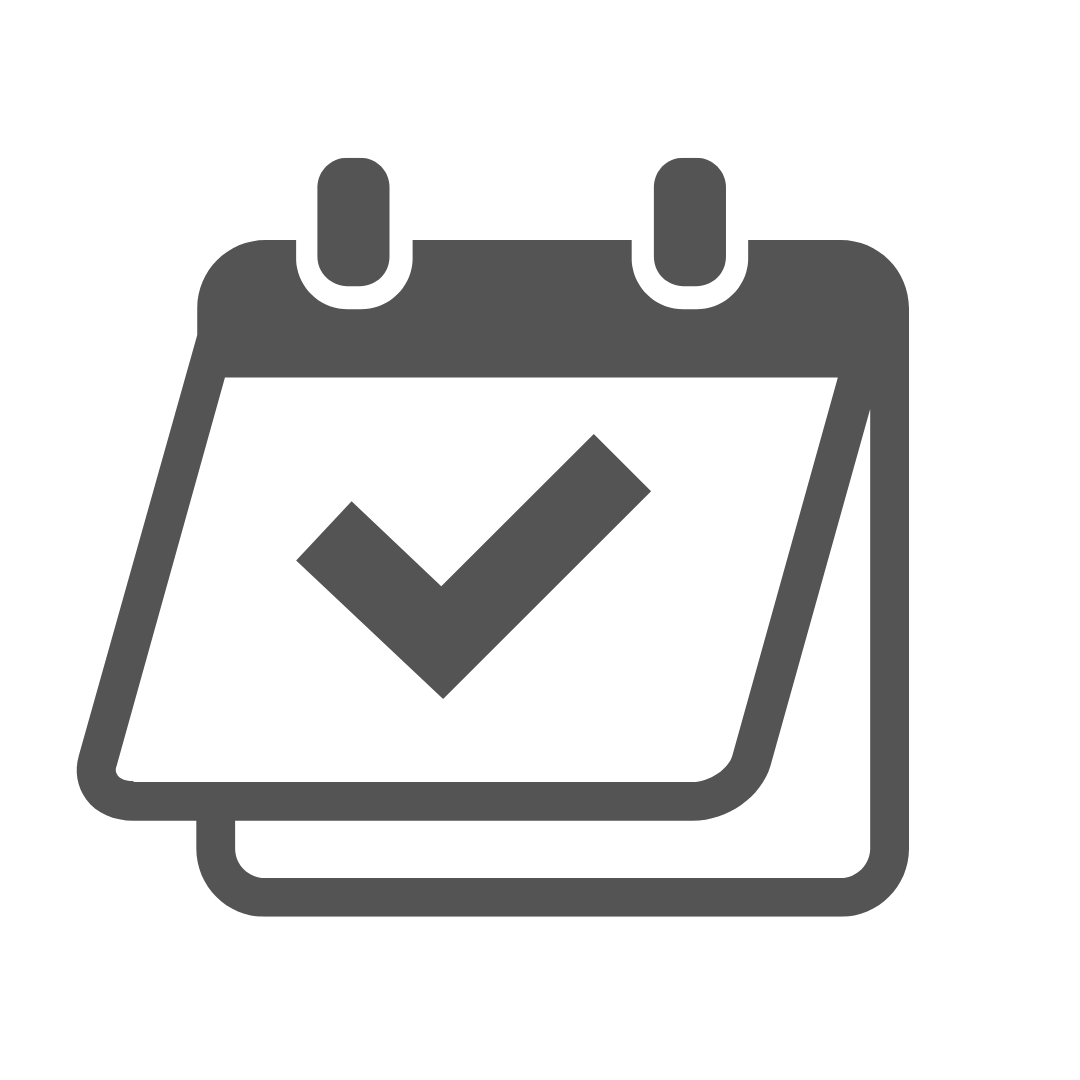
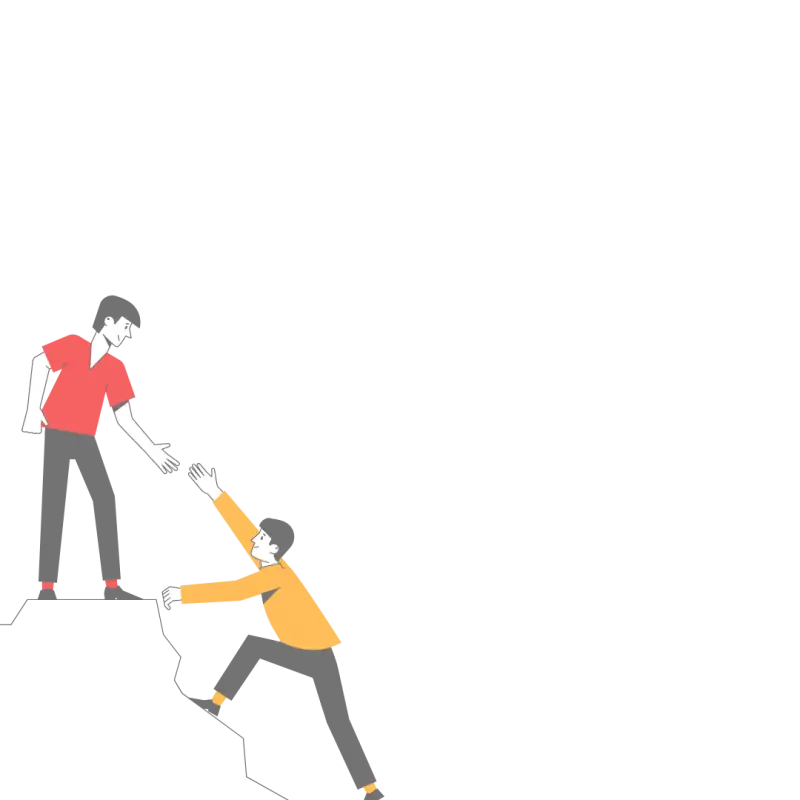
Thanks for your feedback!
Your contributions will help us to improve service.
How can the findIndex()
method in React.js be used to locate the index of the first element that satisfies a specified condition within an array?
The code snippet demonstrates the usage of the findIndex
method in React.js for arrays. The findIndex
function is called when the "Find Index" button is clicked. It searches for the index of the first element in the myArray
that is greater than 30. The found index is stored in the index
state variable using the useState
hook
React Js Array findIndex() Method - Finding the index of the first element that matches a given condition.
Output of React Js Array findIndex Method
What is the syntax and usage of the findIndex()
method in React.js for finding the index of the first element in an array that is greater than a given value?
The code snippet demonstrates the usage of the findIndex()
method in ReactJS. The findIndexGreaterThanValue
function takes an array and a target value as parameters and uses the findIndex()
method to find the index of the first element in the array that is greater than the target value.
React Js Array findIndex() Method - Finding the index of the first element that is greater than a given value.
Output of React Js array findindex Method
What is the JavaScript array method used in React.js to find the index of the first element that is not a string?
The React JS code provided demonstrates the usage of the findIndex()
method on an array. The array contains various elements, such as strings, booleans, and numbers. The findIndex()
method is applied with a callback function that checks if the type of each element is not a string. It returns the index of the first element that meets this condition.
React Js Array findIndex() Method - Finding the index of the first element that is not a string
How can the findIndex()
method in React.js be used to find the index of the first element in an array that is a prime number?
The code snippet demonstrates the usage of the findIndex()
method in React.js. It defines an array of numbers and a helper function called isPrime()
to check if a number is prime. The findIndex()
method is then used to find the index of the first prime number in the array.
React Js Array findIndex() Method - Finding the index of the first element that is a prime number.
How can the findIndex()
method in React.js be used to find the index of the first element in an array that matches a regular expression?
The React JS findIndex()
method is used to find the index of the first element in an array that matches a given condition. In the provided code, an array containing fruits is created. The findIndex()
method is used with a regular expression to search for the first element in the array that contains the characters 'an'. The index of the matching element is stored in the index
variable. The index and corresponding value are then displayed in the React component.