React Js Array Join Method
React Js Array Join Method:The join()
method in React.js is used to concatenate the elements of an array into a string.
It takes an optional parameter called "separator," which is inserted between each element in the resulting string.
If no separator is provided, a comma is used by default. The join()
method does not modify the original array; it only returns a new string.
This method is commonly used in React.js applications when working with arrays and needing to display their elements as a single string, such as rendering a list of items or generating dynamic content
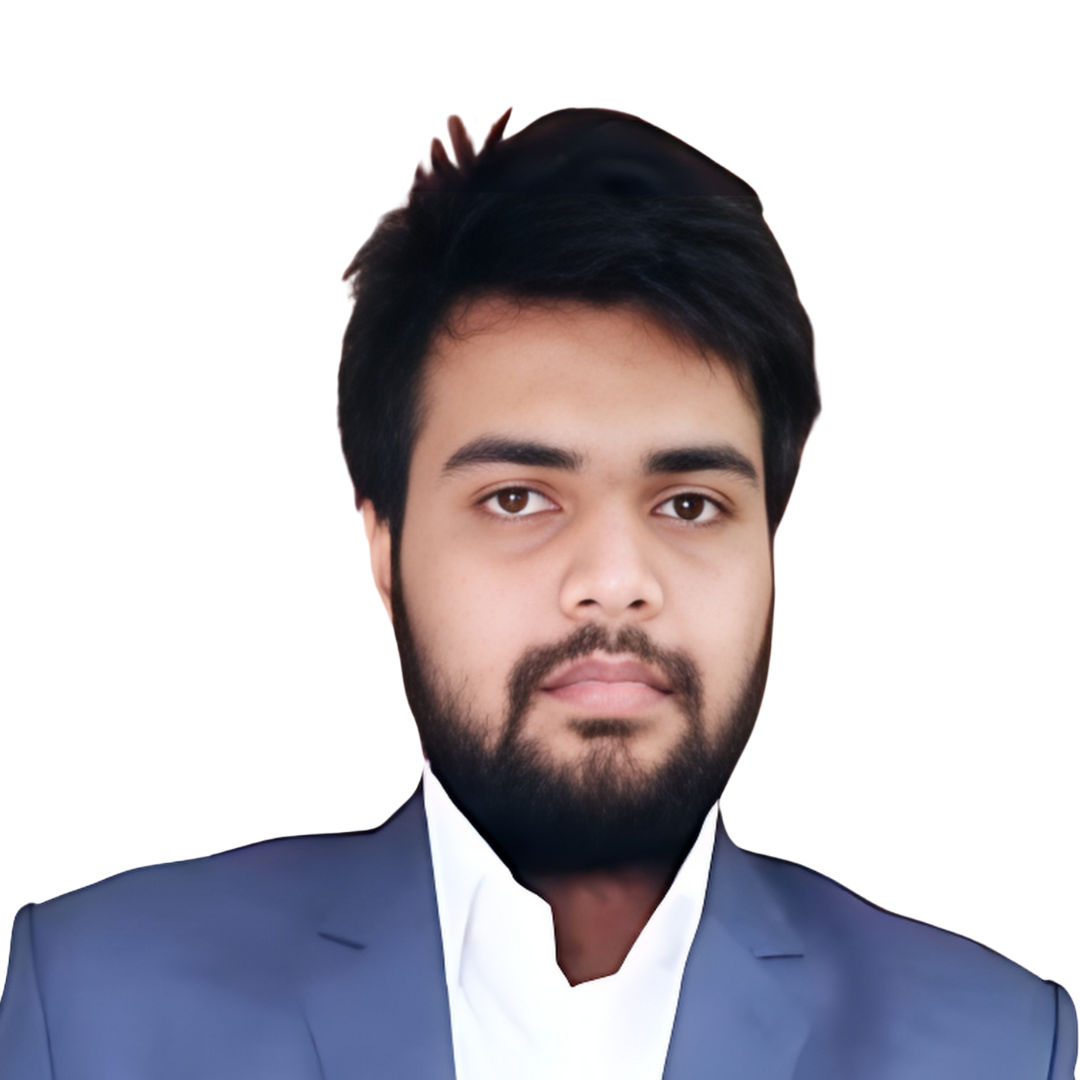
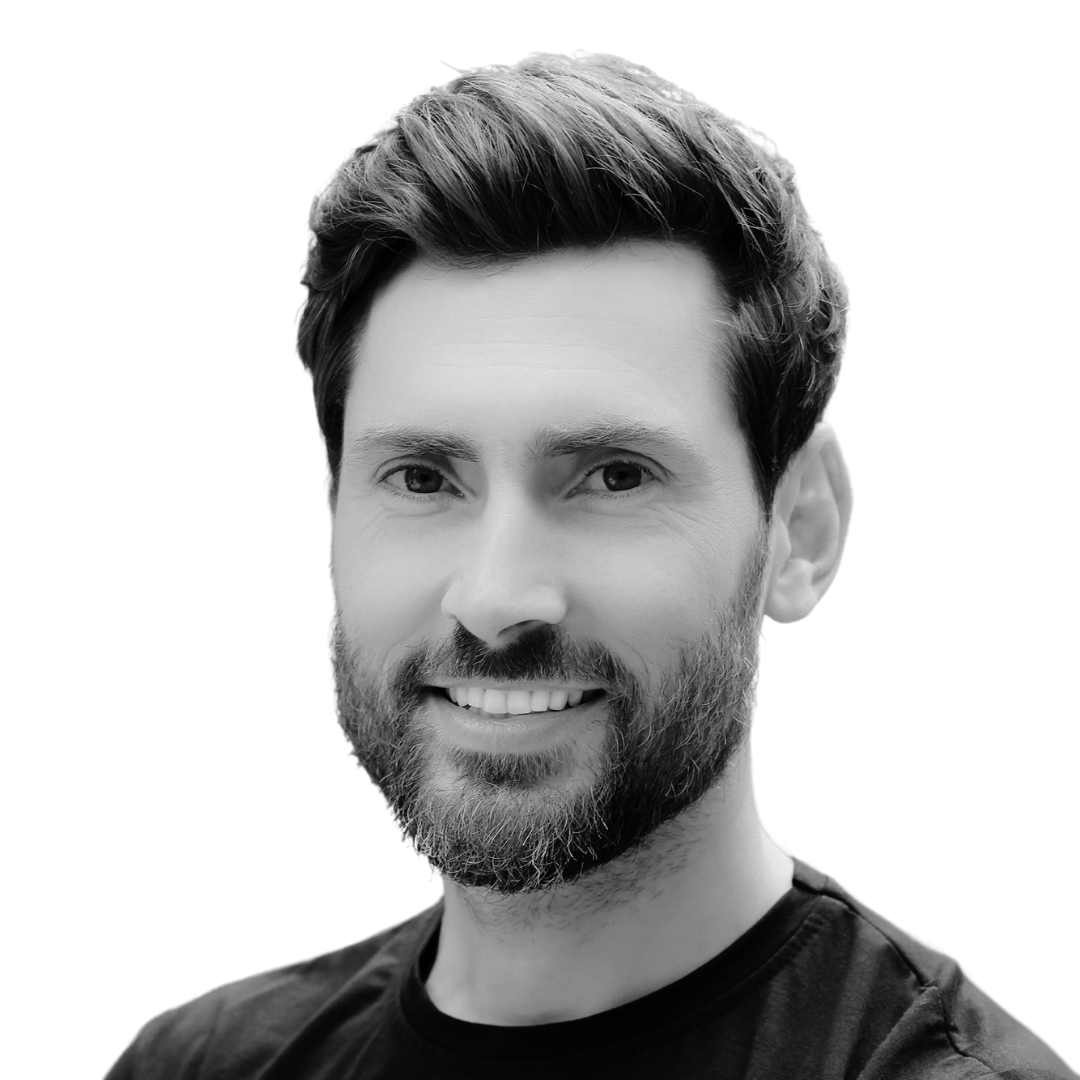
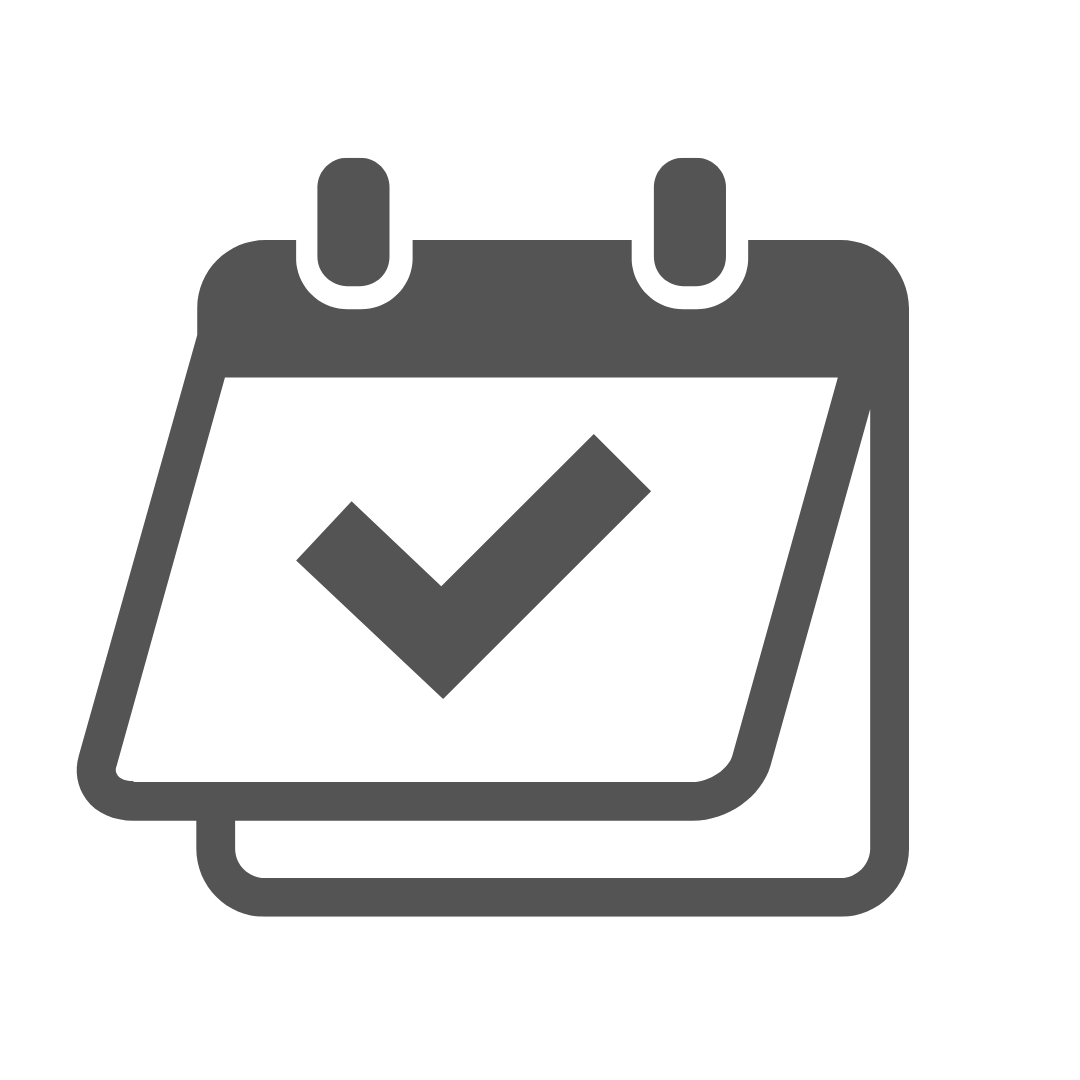
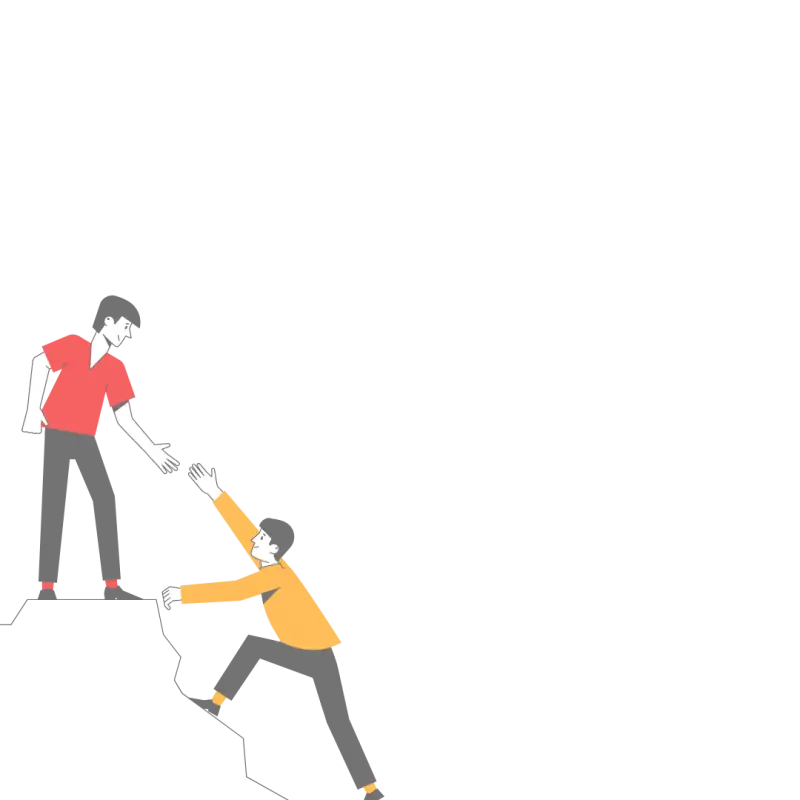
Thanks for your feedback!
Your contributions will help us to improve service.
How can I convert an array to a string in React.js, with each element separated by commas?
In ReactJS, you can convert an array to a string with commas using the join
method. By calling array.join(', ')
, the array elements will be concatenated into a string, with each element separated by a comma and a space.
This allows you to easily display or manipulate the array's contents as a single string
How to convert an array to a string with spaces in React.js?
In the provided React.js code snippet, an array containing several strings is converted into a single string with spaces as separators.
The join()
method is used to concatenate the array elements together with a space (' ') as the separator.
How can you convert an array to a string in React.js using a hyphen (-) as a separator?
This React.js code converts an array into a string with a hyphen (-) separator.
It creates a function called App()
that initializes an array with elements 'John', 'Doe', '123', 'Main', and 'Street'. The join()
method is then used on the array, specifying the hyphen as the separator.
How can you convert an array to a string in React.js, separating the array elements with a comma and space?
In this React.js code snippet, an array of fruits is defined. The array elements are then joined into a single string using the join
method with a comma and space separator.
How can you convert an array to a string in React.js, with each element wrapped in double quotes?
In the given React.js code, an array of fruits ['Apple', 'Banana', 'Orange', 'Grapes', 'Mango'] is declared. To convert the array into a string representation with double quotes, the JSON.stringify()
method is used on the array.
The resulting string includes the array elements enclosed in double quotes. Additionally, the join()
method is used to create another string representation of the array with elements separated by a comma and a space
How can I convert an array to a string in ReactJS, where the array elements are separated by commas and enclosed in single quotes?
In this React.js code snippet, an array of strings is converted to a single string with comma separation and single quotes.
The map()
function is used to wrap each element of the array in single quotes, and then the join()
function is used to concatenate the elements with commas
How can I convert an array to a string in React.js, separating the elements with spaces and enclosing them in double quotes?
The code snippet provided is a React.js component that converts an array of strings into a single string with spaces and double quotes.
The array
variable contains the initial array of strings. The map
method is used to iterate over each item in the array and wrap it with double quotes. The join
method is then used to concatenate the modified array elements into a single string with spaces between them.
How can you convert an array to a string representation in React.js, including brackets around the elements?
In the given code snippet, an array of strings is defined as const array = ['Apple', 'Banana', 'Orange', 'Grapes', 'Mango'];
.
To convert this array to a string representation with brackets, the JSON.stringify()
method is used, and the result is stored in the arrayString
variable.
The string representation of the array is then rendered in the React component using {arrayString}
within the JSX code
How can I convert an array to a string in React.js while adding slashes between each element?
In this React.js code snippet, an array is converted to a string with slashes. The array contains three items: 'item1', 'item2', and 'item3'.
The join()
method is used to concatenate the array elements with slashes as the separator.
Then, the resulting string is processed using replace()
with a regular expression to escape the slashes with backslashes