React Js Sort Array of objects by a numeric property in ascending | descending order
React Js Sort array by number Ascending or Descending order:To sort an array of objects in React.js by a numeric property in ascending or descending order, you can utilize the sort() method with a comparison function.
Let's assume the array is named myArray and the numeric property is numericProperty. For ascending order, you can use myArray.sort((a, b) => a.numericProperty - b.numericProperty).
To sort in descending order, you can modify it as myArray.sort((a, b) => b.numericProperty - a.numericProperty).
This approach compares the numericProperty of each object, resulting in the array being rearranged based on the desired order.
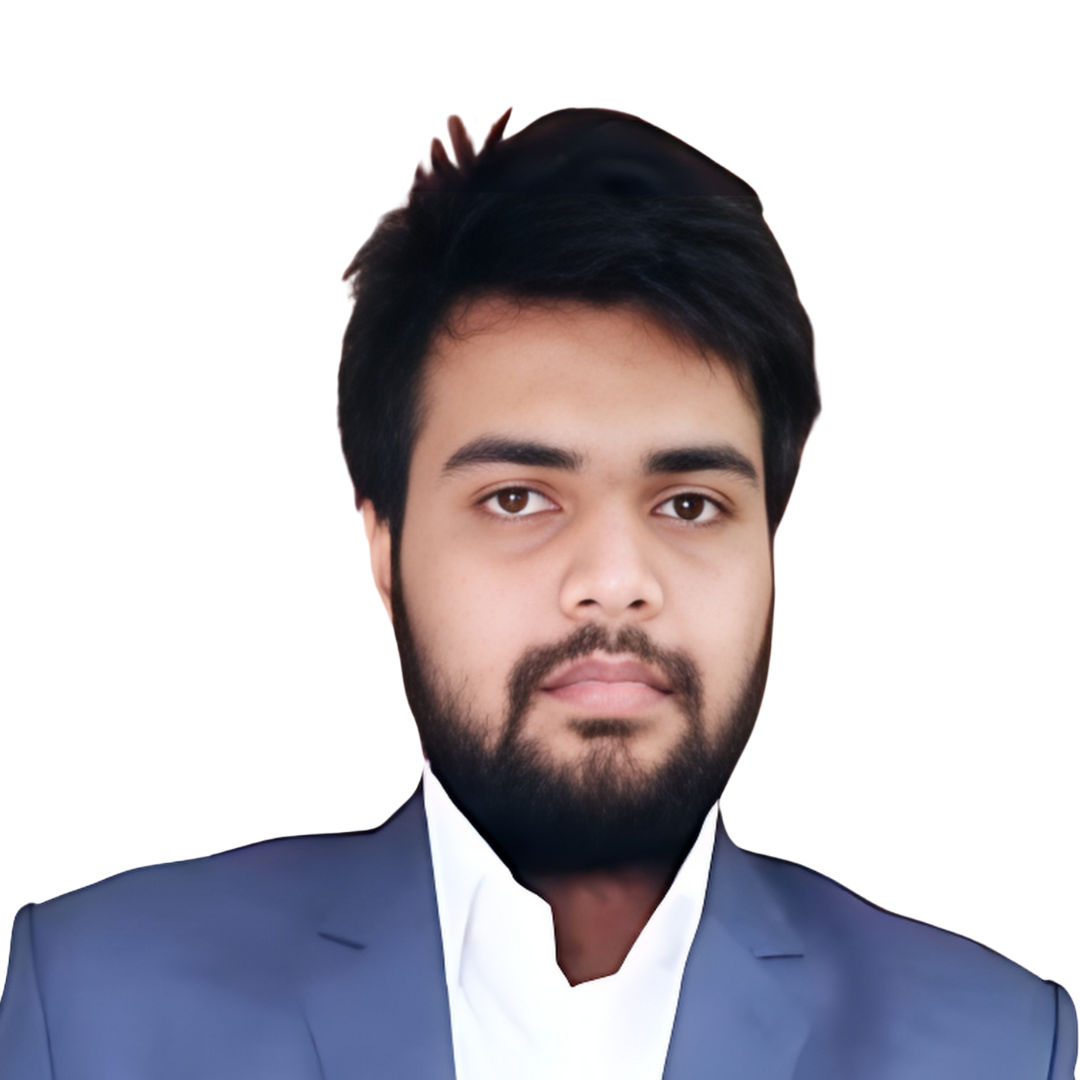
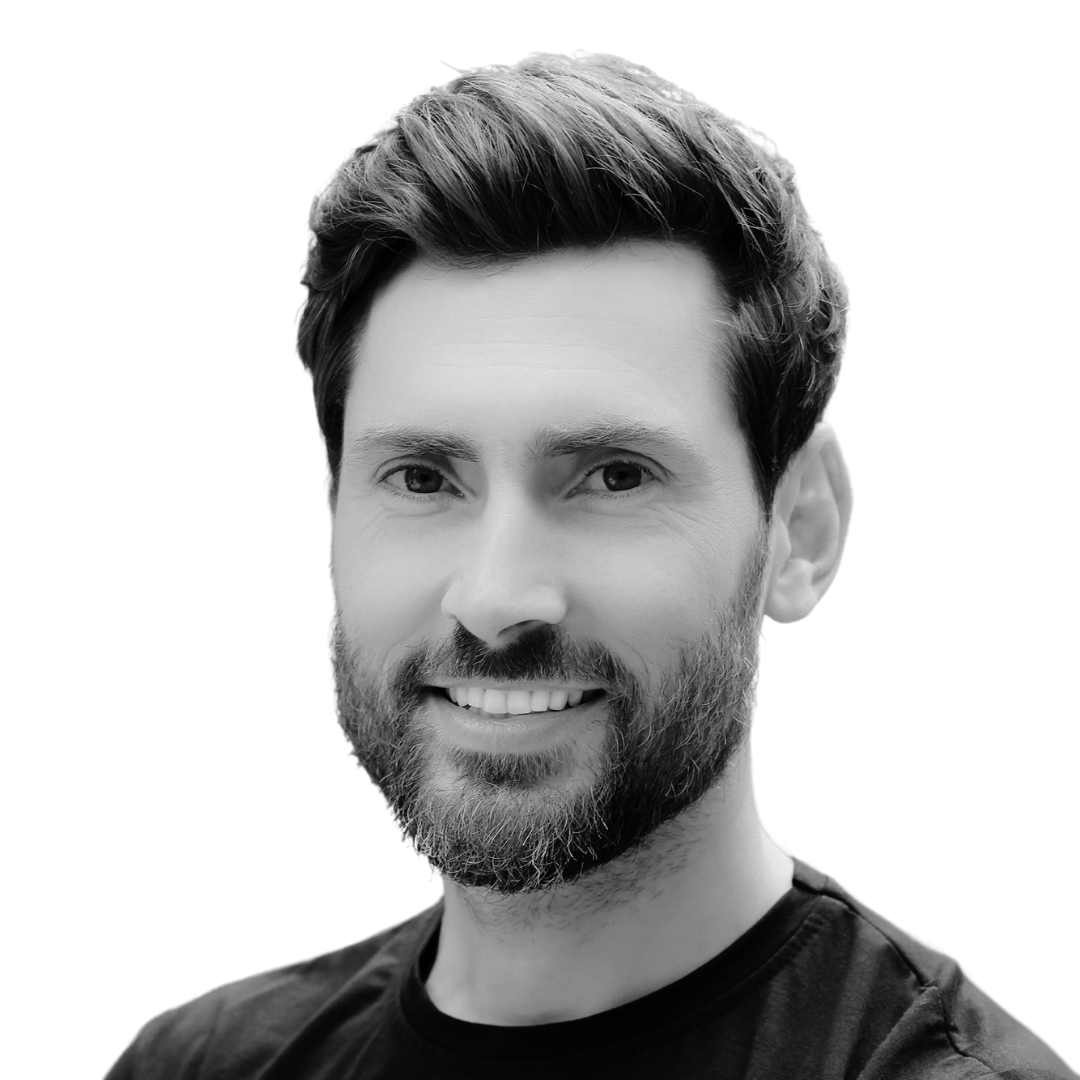
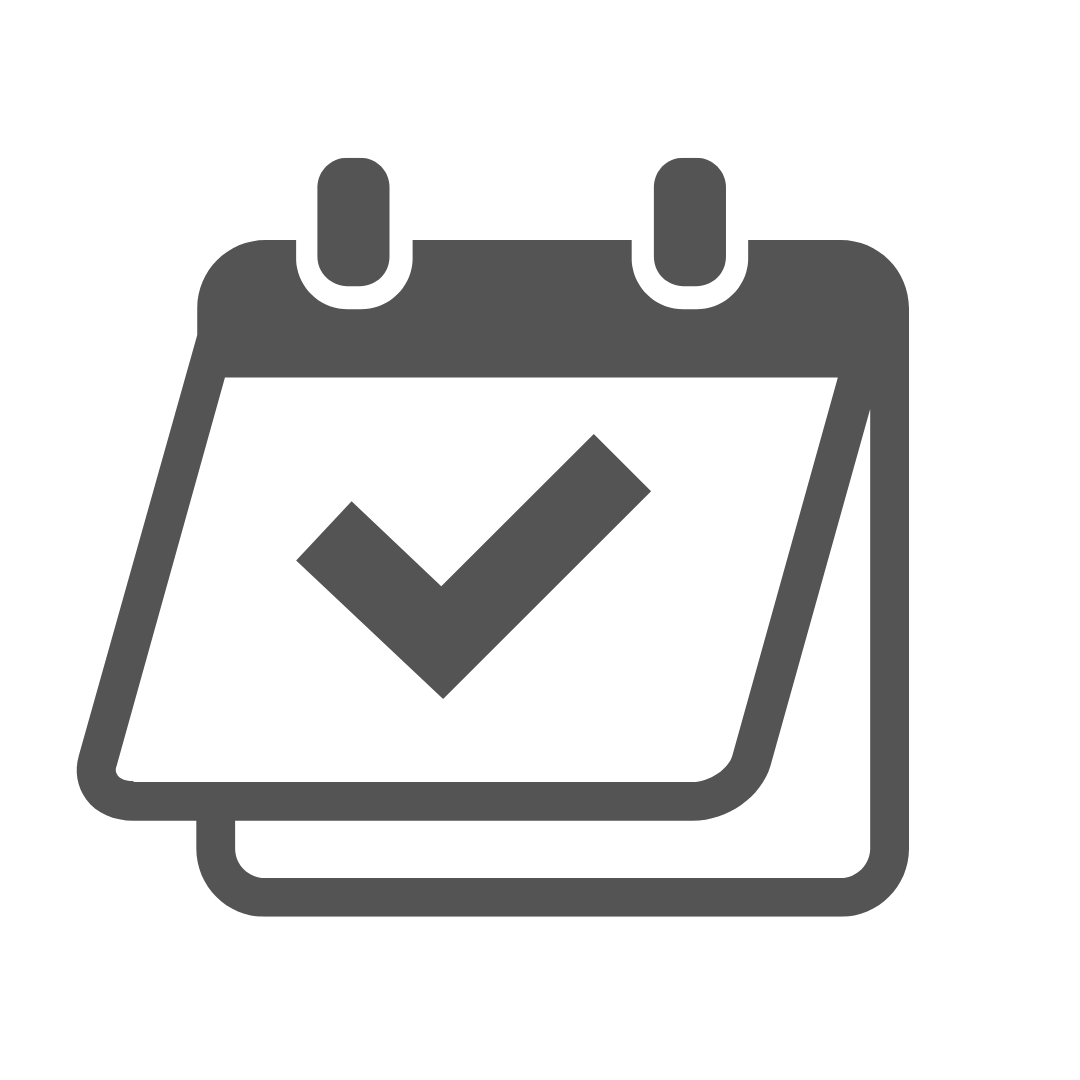
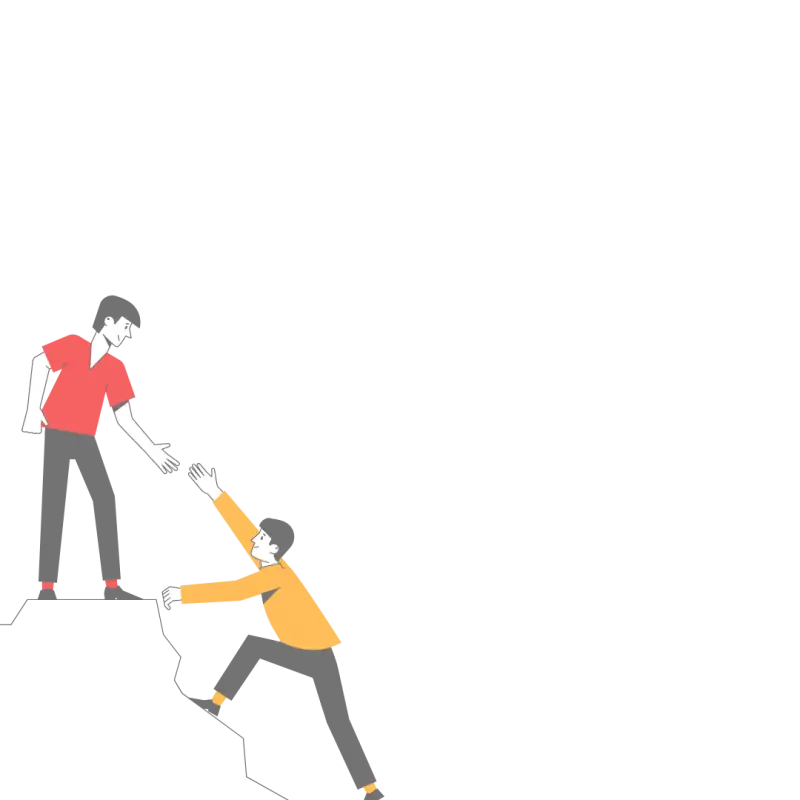
Thanks for your feedback!
Your contributions will help us to improve service.
How can I sort an array of objects in ascending order based on a numeric property using React.js?
This React.js code sorts an array of objects based on a numeric property in ascending order. The array of objects, data
, contains elements with properties id
, name
, and age
.
The code creates a new array, sortedData
, by using the spread operator ([...data]
) to clone the original array and then applies the sort()
method with a comparison function (a, b) => a.age - b.age
to sort the objects by their age
property.
Finally, the sorted data is rendered in an unordered list (ul) on the webpage.
React Js Sort Array of objects by a numeric property in ascending
xxxxxxxxxx
<script type="text/babel">
const { useState } = React
function App() {
const data = [
{ id: 1, name: "John", age: 25 },
{ id: 2, name: "Jane", age: 30 },
{ id: 3, name: "Bob", age: 20 }
];
const sortedData = [...data].sort((a, b) => a.age - b.age);
return (
<div className='container'>
<h3>React Js Sort array of object by numeric property in ascending order</h3>
<h4>Sorted Data:</h4>
<ul>
{sortedData.map((item) => (
<li key={item.id}>{item.name} - {item.age}</li>
))}
</ul>
</div>
);
}
ReactDOM.render(<App />, document.getElementById("app"));
</script>
Output of React js sort array of object by ascending order
How can you sort an array of objects by a numeric property in descending order using React.js?
The code snippet uses React.js to sort an array of objects based on a numeric property in descending order.
The array is duplicated using the spread operator, and the sort function compares two objects based on their "age" property.
By subtracting "a.age" from "b.age", the sort order is determined. The resulting sorted array is stored in the variable "sortedData".
React Js Sort Array of objects by a numeric property in descending order
xxxxxxxxxx
<script type="text/babel">
const { useState } = React
function App() {
const data = [
{ id: 1, name: 'John', age: 25 },
{ id: 2, name: 'Alice', age: 32 },
{ id: 3, name: 'Bob', age: 28 },
{ id: 4, name: 'Sarah', age: 30 },
{ id: 5, name: 'Mike', age: 27 },
// ... more objects
];
const sortedData = [...data].sort((a, b) => b.age - a.age);
return (
<div className='container'>
<h3>React Js Sort array of object by numeric property in descending order</h3>
<h4>Sorted Data:</h4>
<ul>
{sortedData.map((item) => (
<li key={item.id}>{item.name} - {item.age}</li>
))}
</ul>
</div>
);
}
ReactDOM.render(<App />, document.getElementById("app"));
</script>