React Js Convert JSON to CSV File
React Js Convert JSON to CSV File:React JS can convert JSON to a CSV file by first processing the JSON data and transforming it into a comma-separated value format. This involves iterating through the JSON objects, extracting values, and structuring them as rows in the CSV.
Build the CSV string and create a download link with the Blob API. Handle data formatting and escaping to ensure CSV validity. Update your component's state to trigger re-renders when needed. Remember to handle edge cases like empty values.
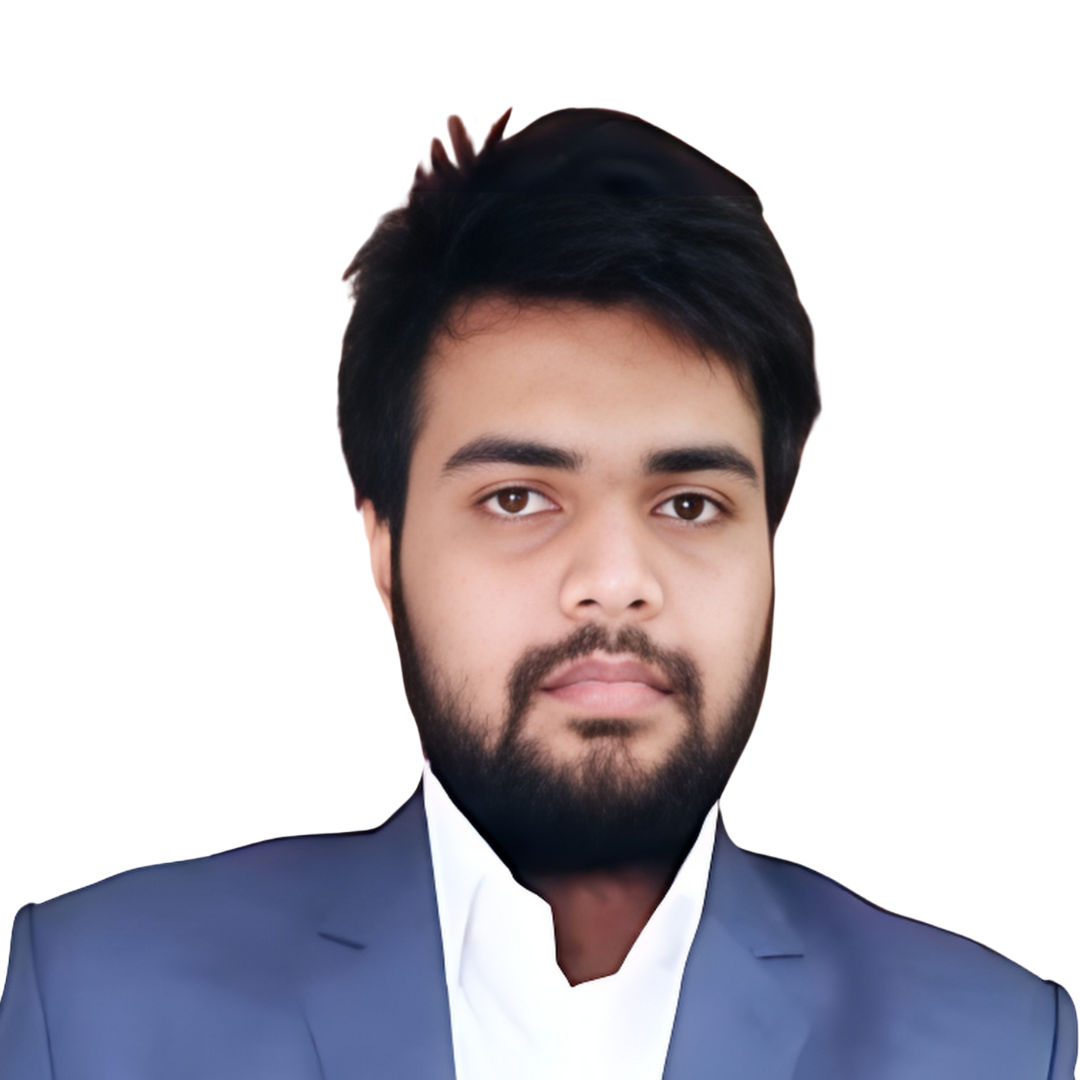
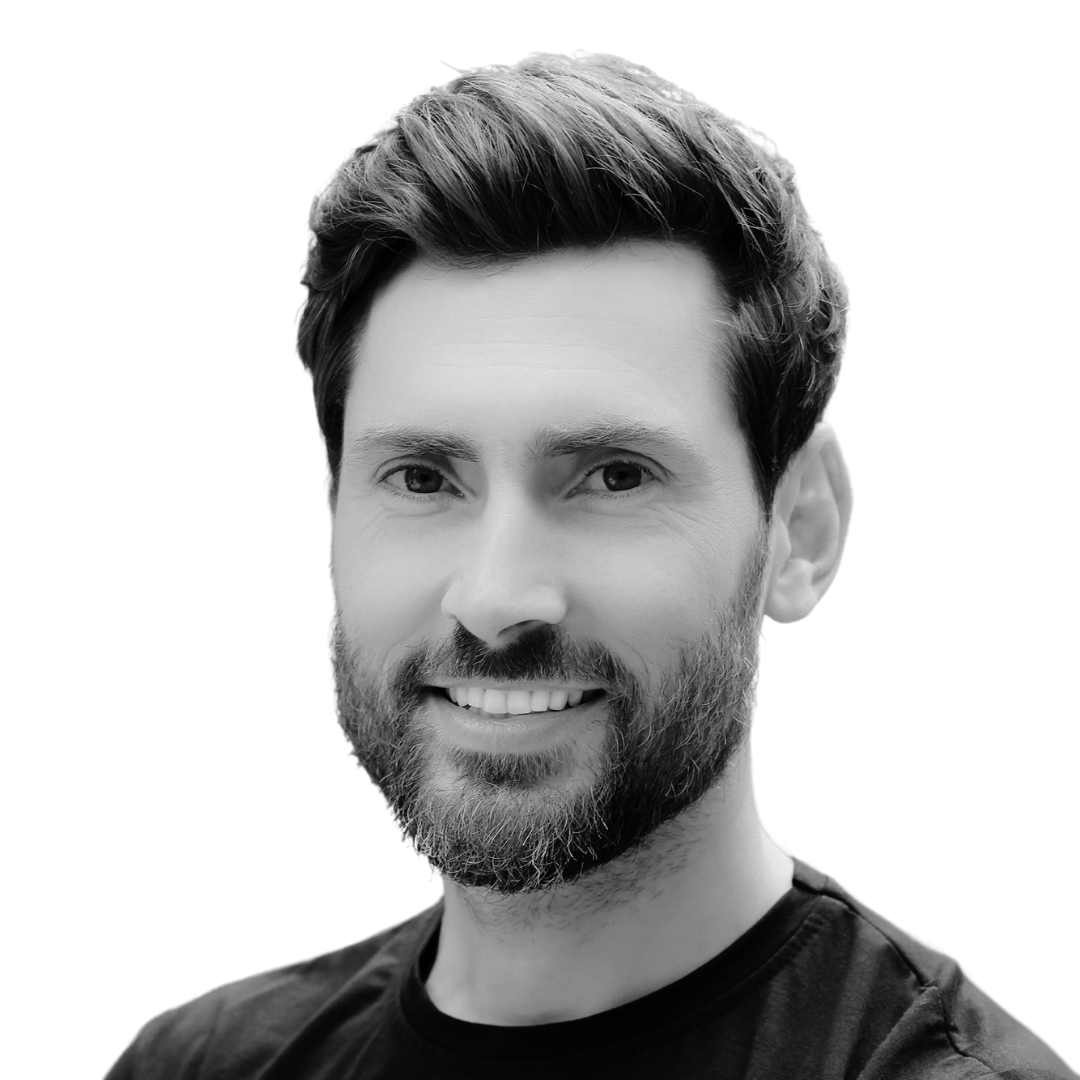
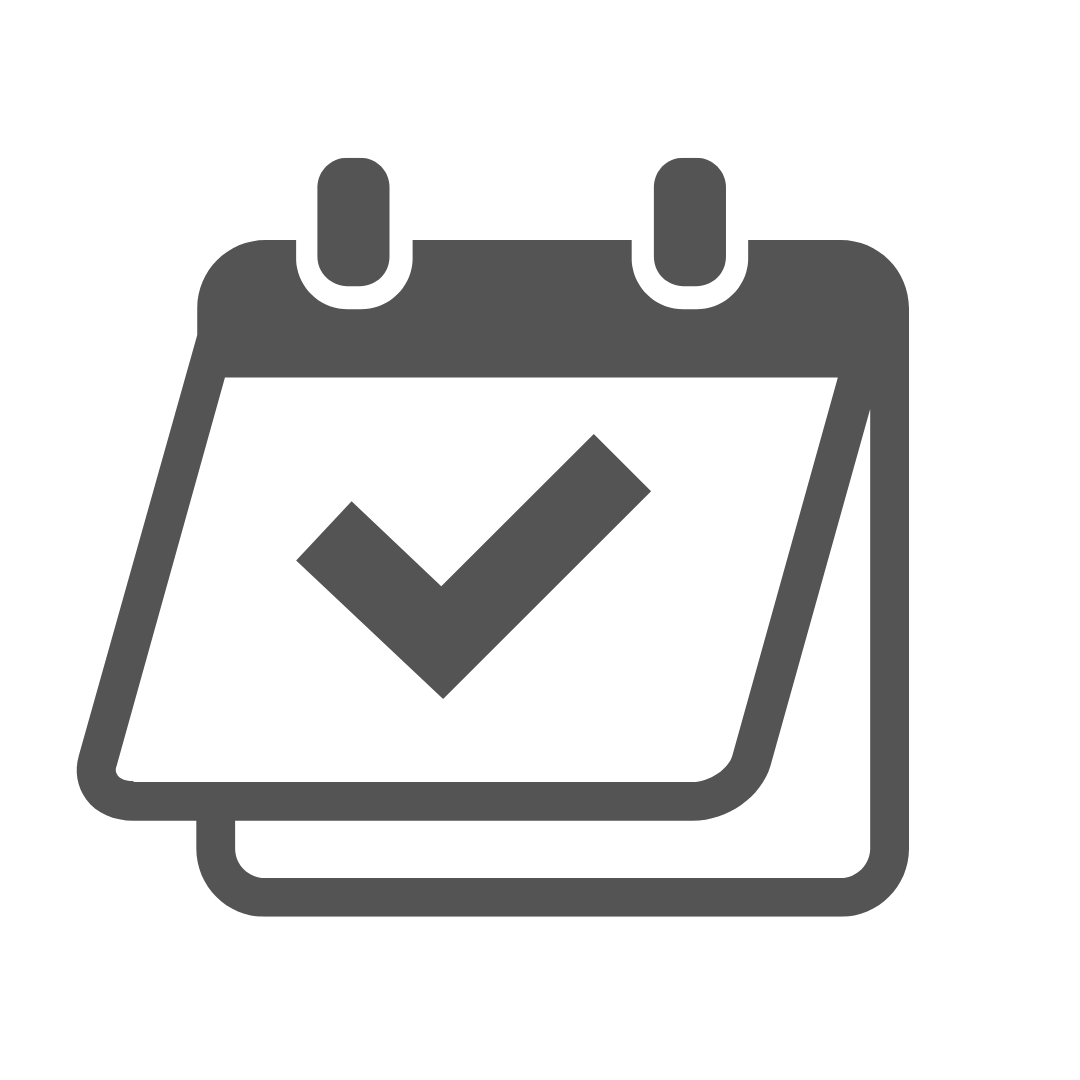
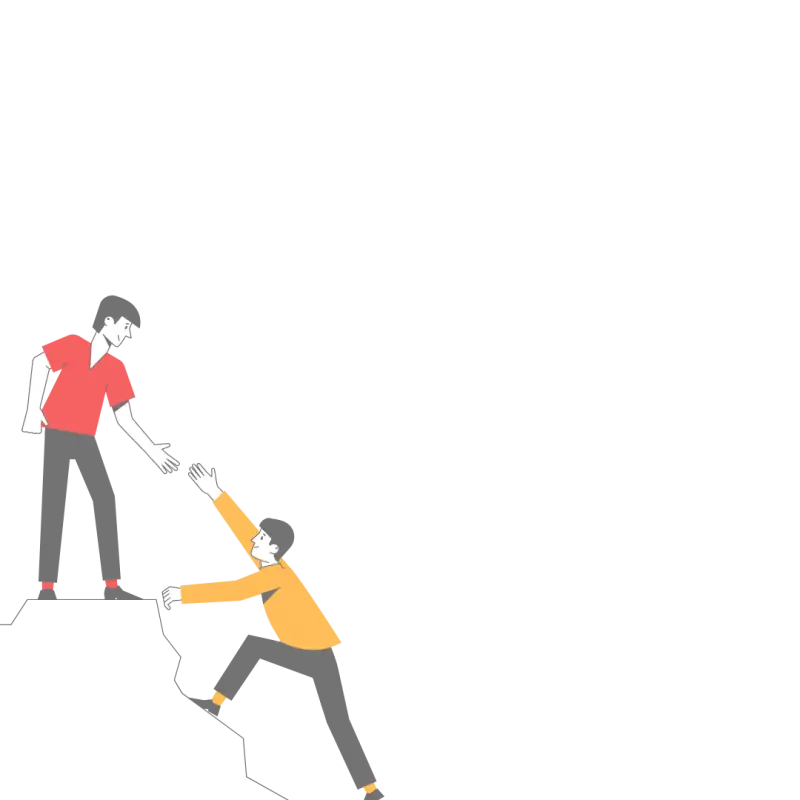
Thanks for your feedback!
Your contributions will help us to improve service.
How can I use Reactjs to efficiently convert a JSON object into a CSV file?
This ReactJS code snippet demonstrates how to convert JSON data into a CSV file. It defines a component that holds JSON data containing names, ages, and cities. Upon clicking a button, the code converts the JSON data into CSV format and triggers a download. The CSV content is constructed by mapping JSON values, joining them with commas, and then combining lines with line breaks. The encoded CSV content is linked to a downloadable anchor element, and after clicking, the link is removed