React Js Month Picker
.jpg)
React Month Picker | Reactjs Month Picker Format :The ReactJS Month Picker simplifies the process of selecting months and years. Its user-friendly interface and seamless integration make it an ideal choice for developers aiming to enhance their date-related functionalities. Utilizing the popular JavaScript library React.js, developers can create dynamic and responsive web applications effortlessly.
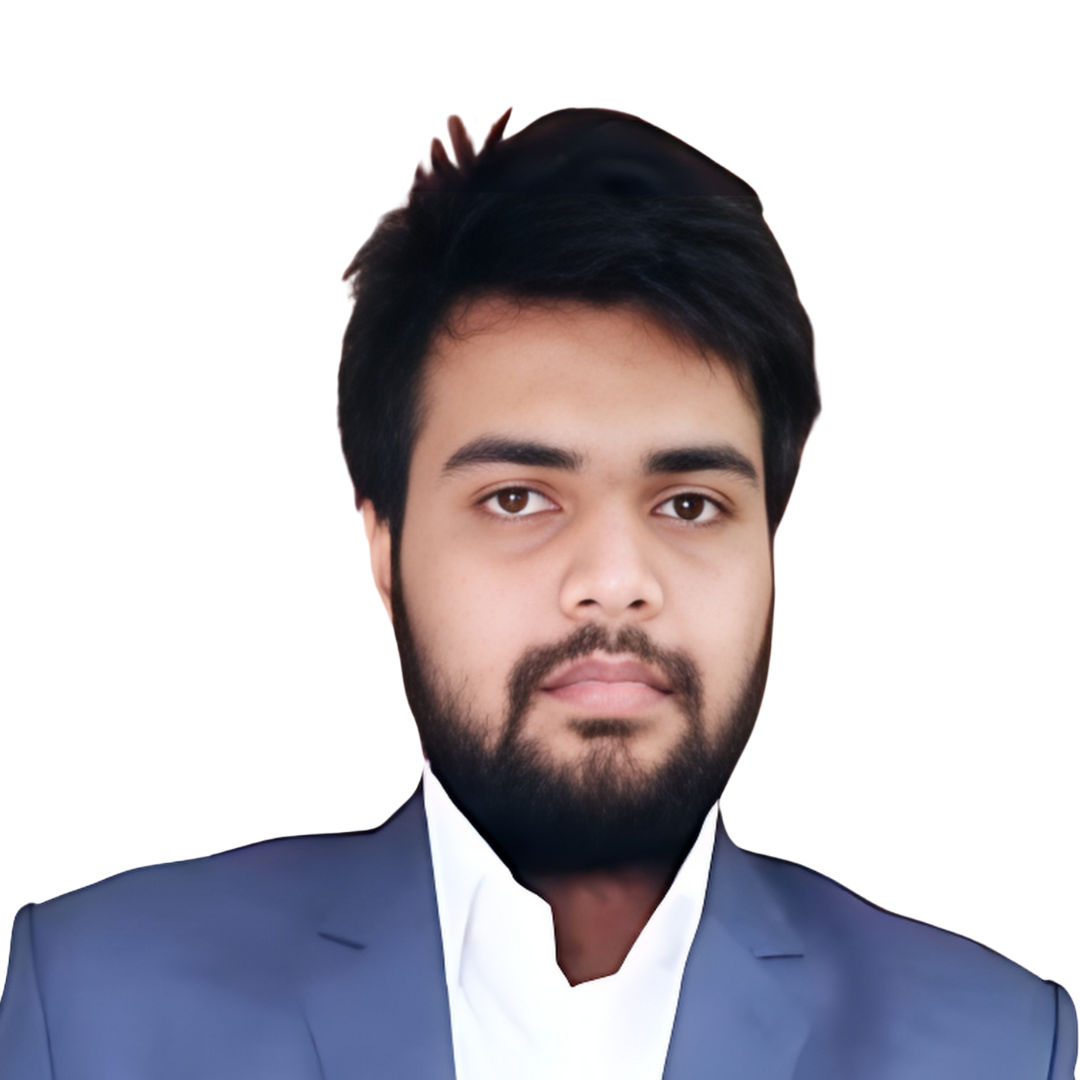
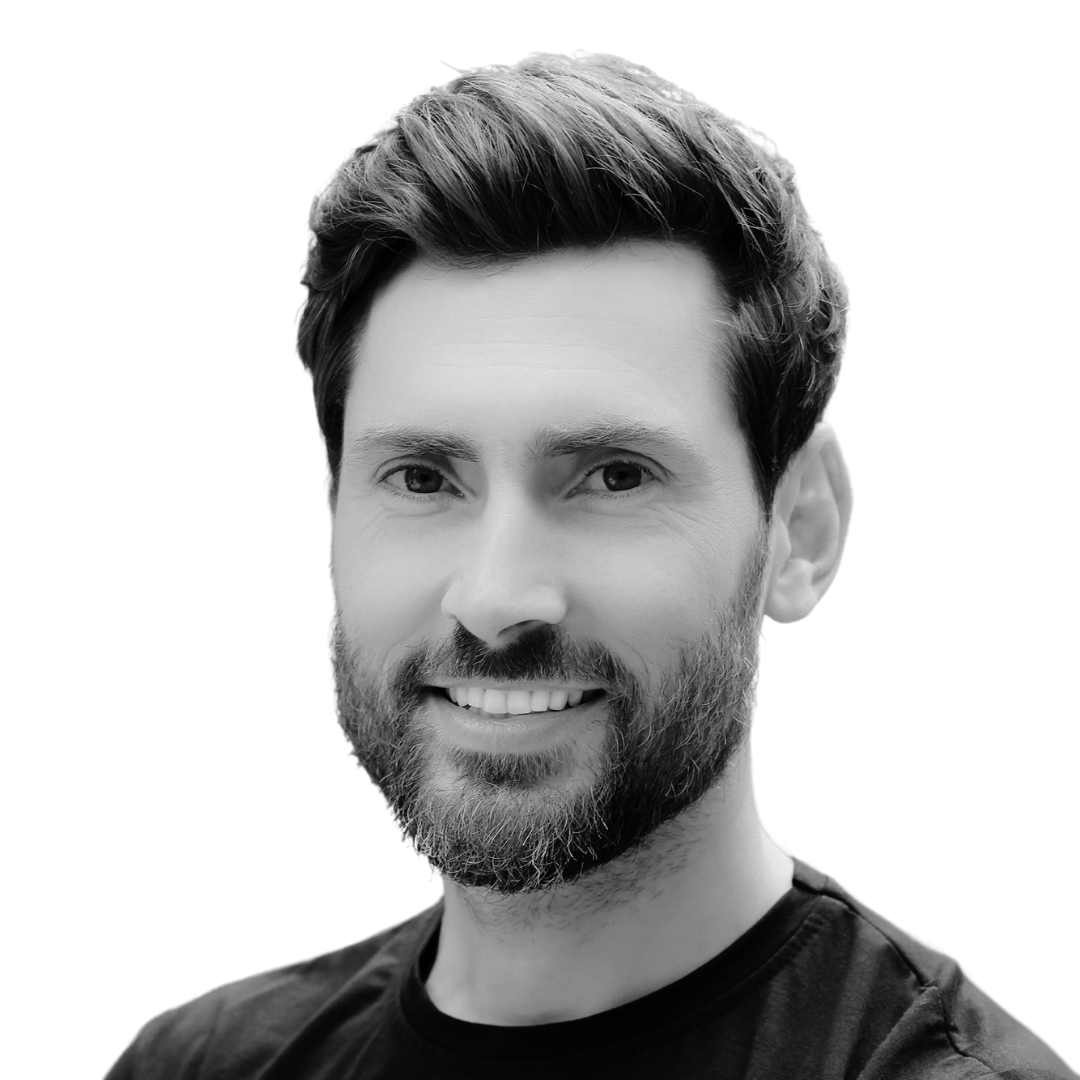
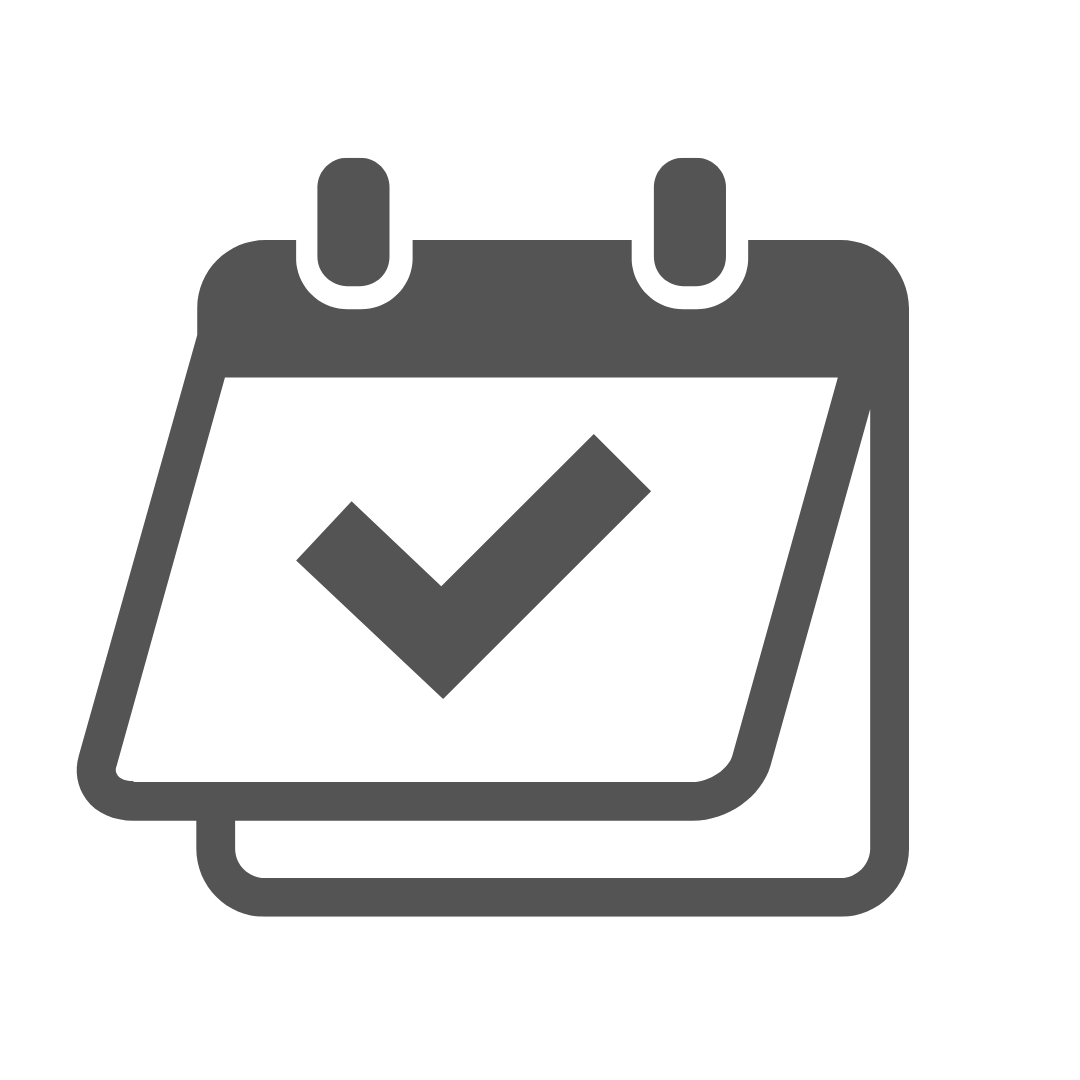
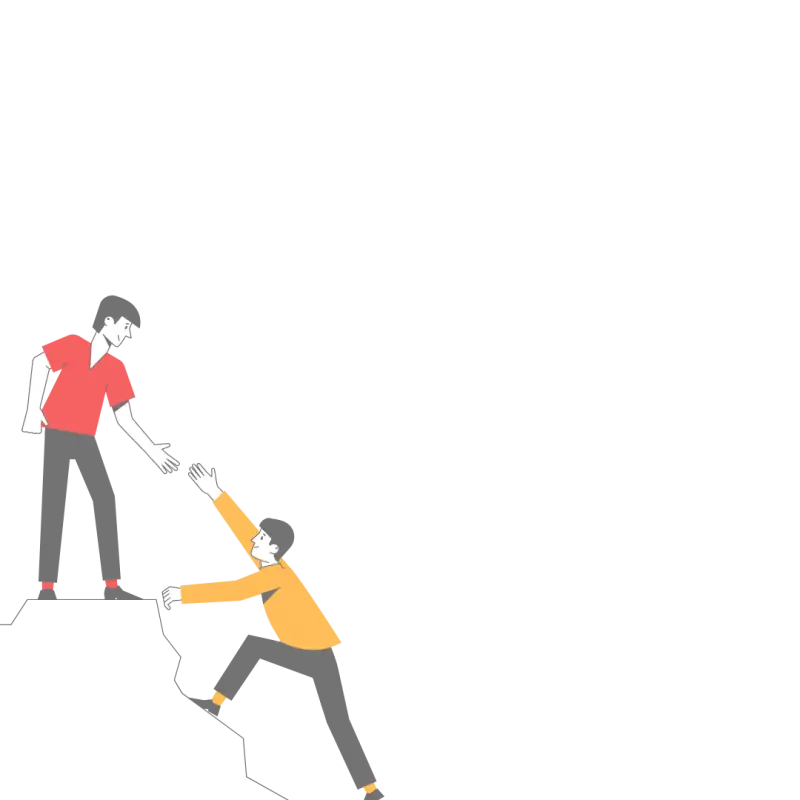
Thanks for your feedback!
Your contributions will help us to improve service.
Implementation 1: Input Field-Based Month Picker
The first implementation utilizes an input field of type "month" to enable users to select a specific month. The underlying code employs React’s useState hook, a powerful tool for managing state within functional components. When a user selects a month, the component extracts the year and month, converting it into a formatted string (e.g., "January 2023"). This formatted month is then displayed as a chip below the input field, enhancing the user experience.
Output of React Js Month Picker Example
Implementation 2: Dropdown-Based Month Picker
The second implementation offers an alternative approach by employing a dropdown menu. Users can select a month from the dropdown, triggering the handleMonthChange function. This function updates the selectedMonth state, reflecting the chosen month in the UI. The dropdown-based approach provides a classic and widely recognized method for users to pick their desired month
Output of React Month Picker in Dropdown
Summary
In this post, we examined two different implementations of the ReactJS Month Picker. You can choose between an elegant input field-based approach or a familiar dropdown-based method to enhance date selection in your web applications.