React Js Change Image Every 5 Seconds

React Js Change Image Every 5 Seconds:Changing images dynamically in a React.js application can enhance user experience and add visual appeal to your website. In this tutorial, we will create a React component that automatically switches between a predefined set of images every 5 seconds. We'll go through the code you provided and explain how it works step by step
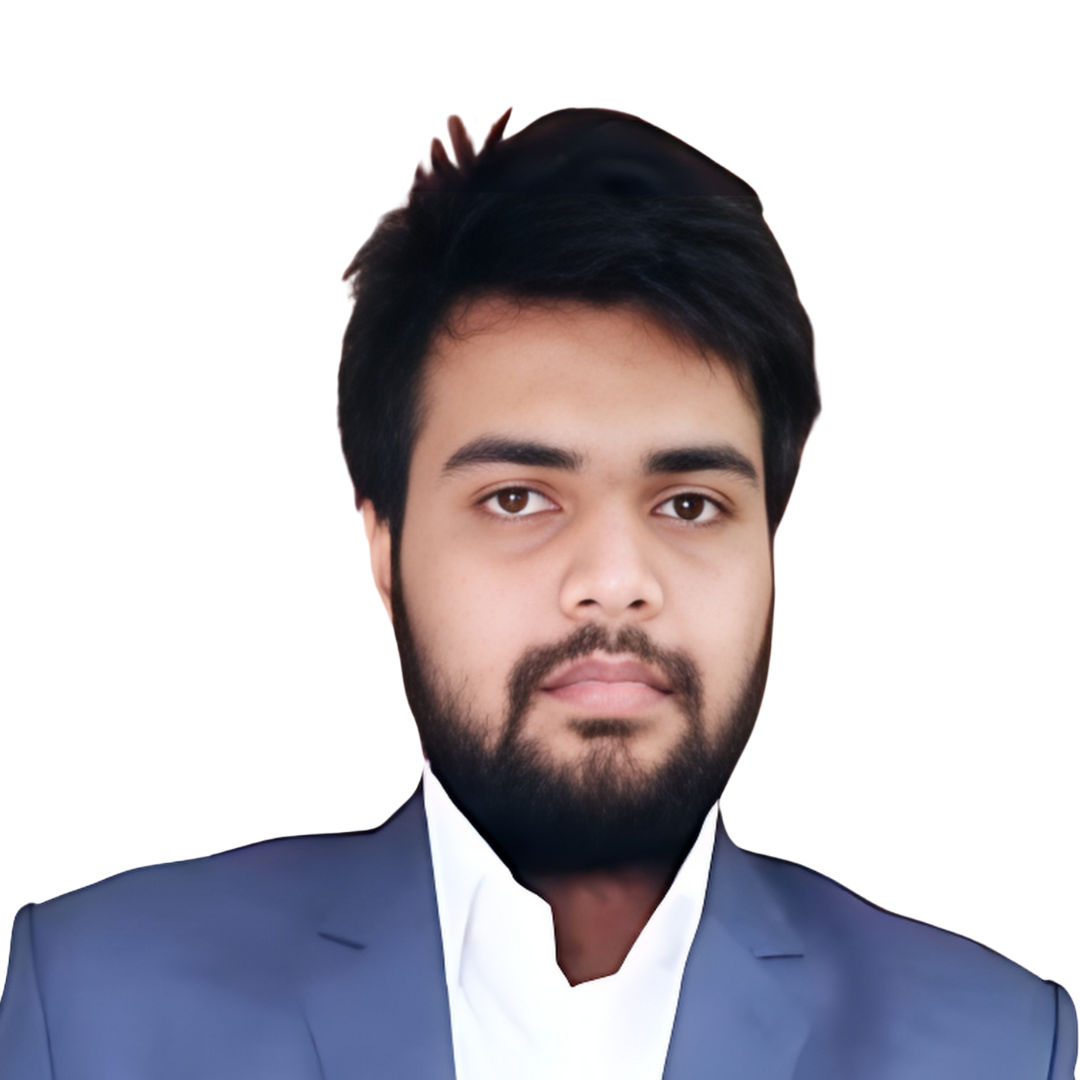
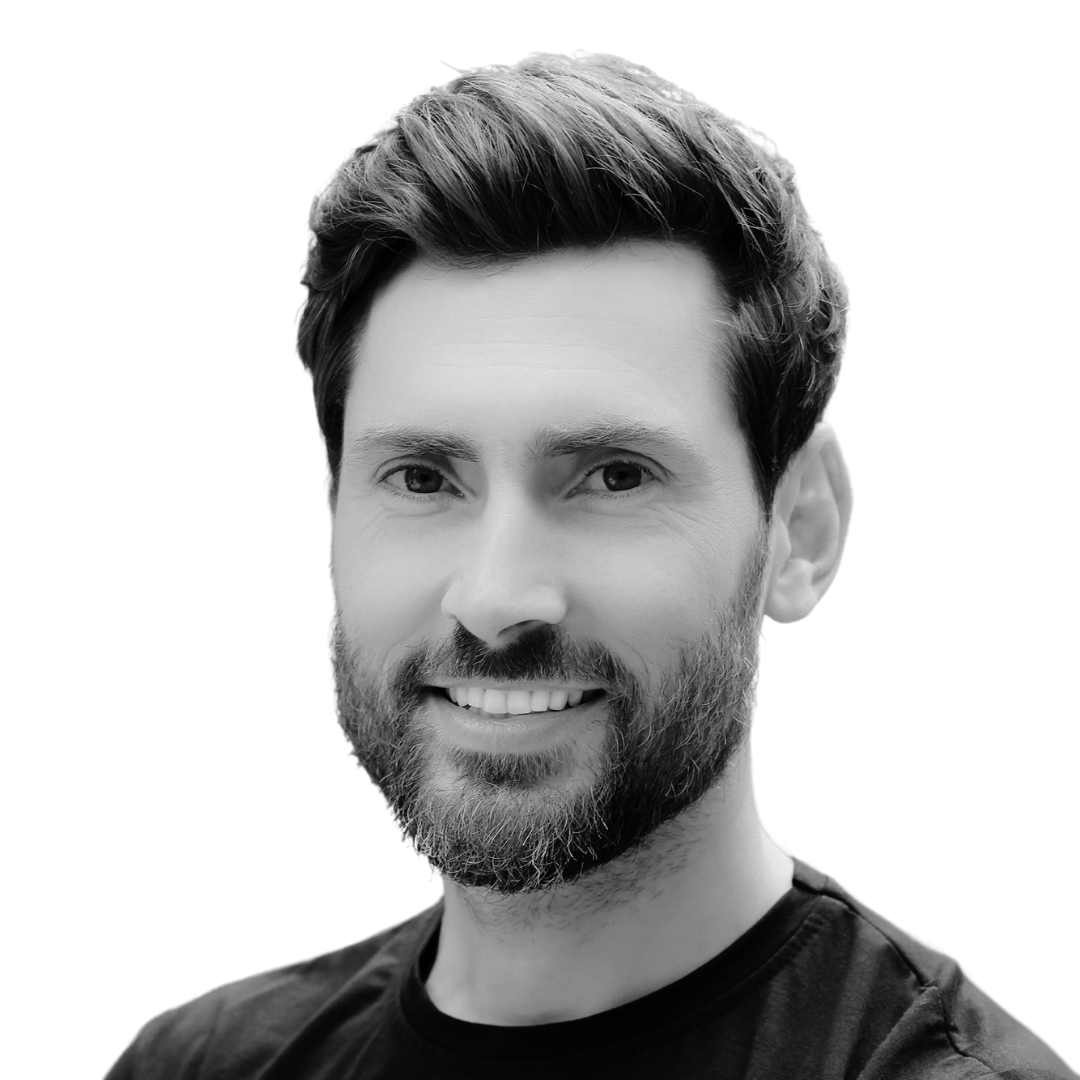
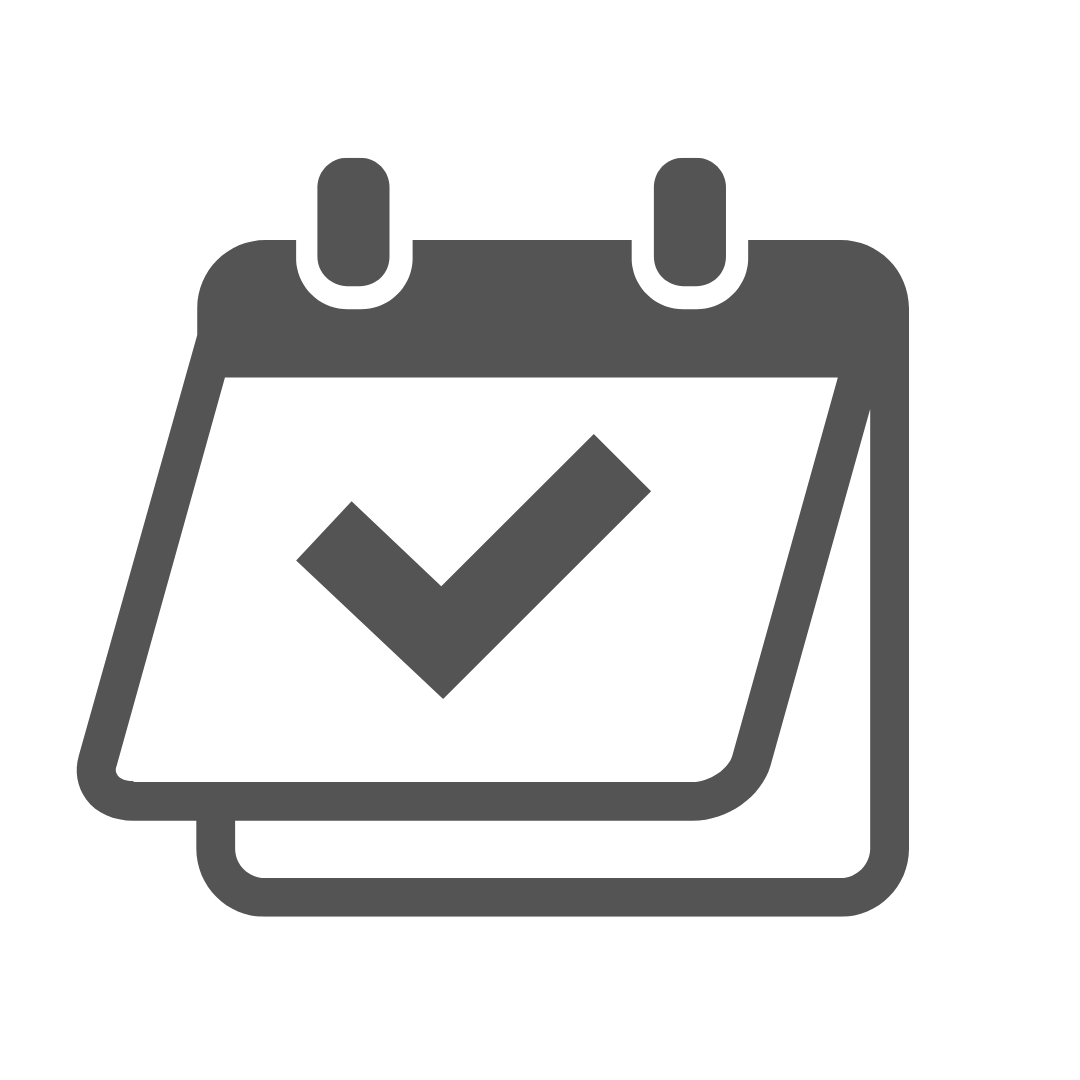
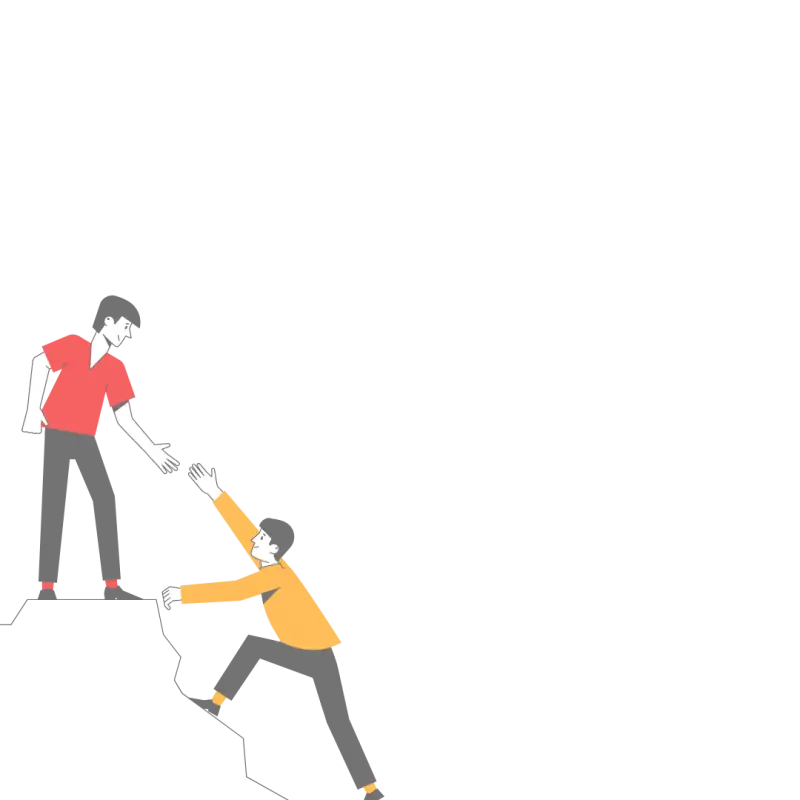
Thanks for your feedback!
Your contributions will help us to improve service.
Output of React Js Change Image Every 5 Seconds
Step 1: Import React and Set Up the Component In the first step, we import the necessary dependencies, including React, and set up a functional component called App
. We also define an array called images
, which contains the URLs of the images we want to display
Here, we use the useState
hook to maintain the index of the currently displayed image, and we initialize it to 0.
Step 2: Implement the Image Rotation We use the useEffect
hook to handle the automatic rotation of images. Inside the useEffect
, we set up a timer using setInterval
to change the currentImageIndex
every 5 seconds. This is done by updating the index based on the previous index, cycling through the images using the modulo operator
The clearInterval
function is used in the cleanup phase to stop the interval when the component is unmounted.
Step 3: Render the Component In the return
statement of the App
component, we render the selected image based on the currentImageIndex
. The image source is dynamically updated, and we set the alt
attribute for accessibility
In summary, the provided code demonstrates how to create a React component that automatically changes images every 5 seconds. This is achieved by using the useState
and useEffect
hooks to manage the image rotation and rendering. The result is a simple but effective image carousel that enhances the user experience on your website