React Js Get First Element/item of Array
React js Get first element/item of array:To get the first element/item of an array in React.js, there are several approaches.
-
Array Indexing: Access the first element using index 0, e.g.,
array[0]
. -
Array Destructuring Assignment: Destructure the array and assign the first element to a variable, e.g.,
[firstItem] = array
. -
Slice() Method: Use the
slice(0, 1)
method to create a new array with only the first element, e.g.,array.slice(0, 1)
. -
Array Shift() Method: Apply the
shift()
method to remove and return the first element from the array, e.g.,array.shift()
. -
Spread Operator: Use the spread operator to copy the array and then access the first element, e.g.,
[...array][0]
.
All of these methods enable you to obtain the first element/item from an array in React.js, providing flexibility based on your specific use case.
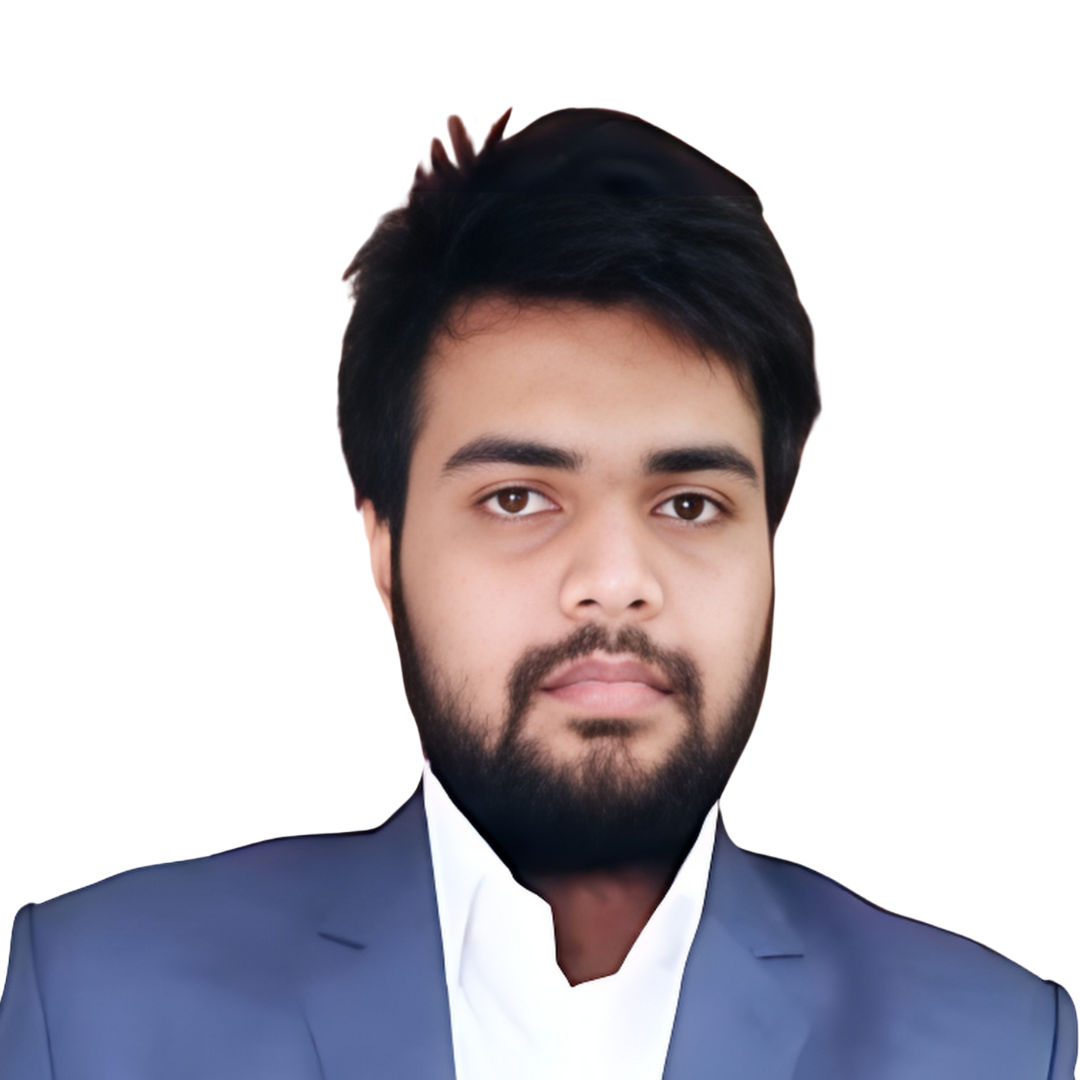
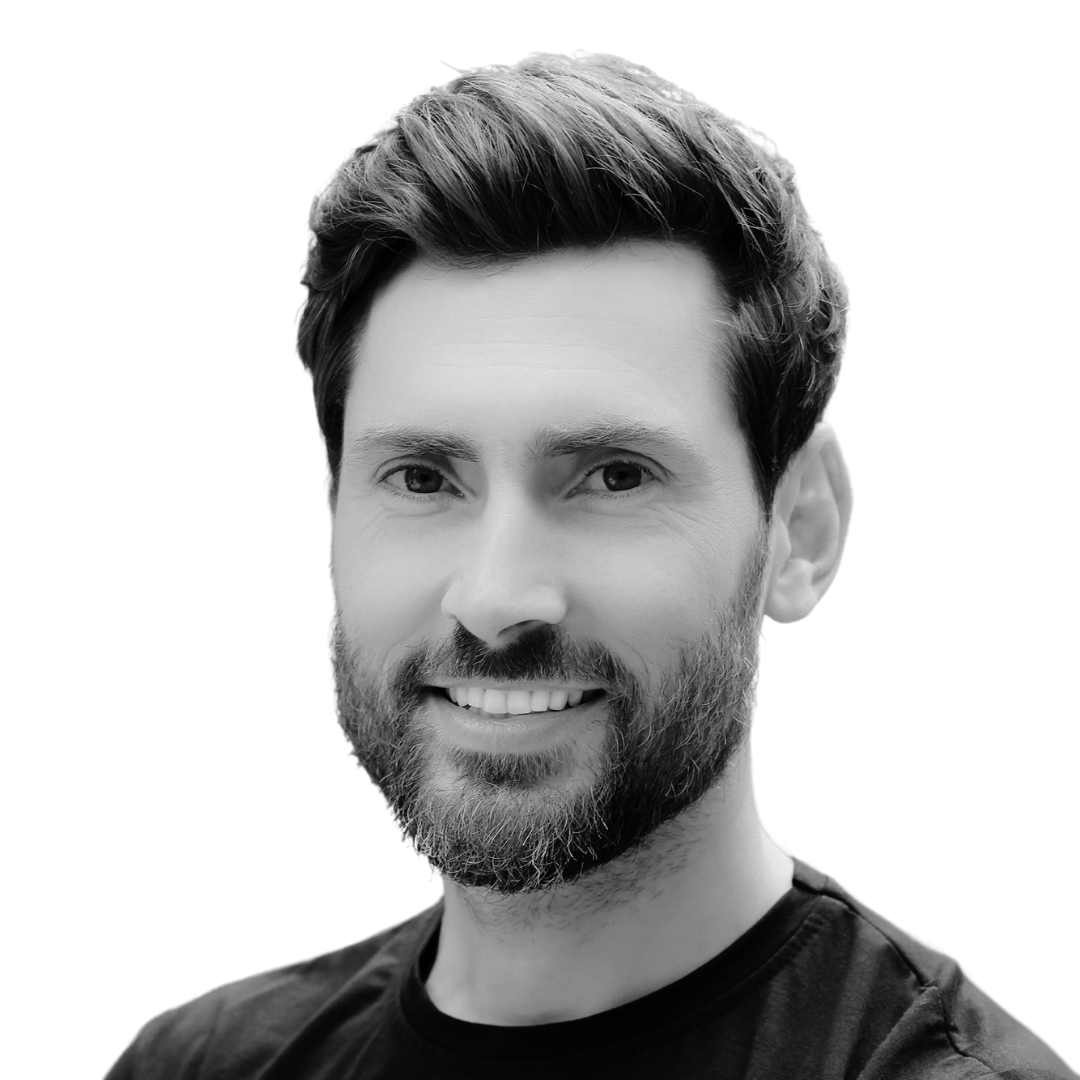
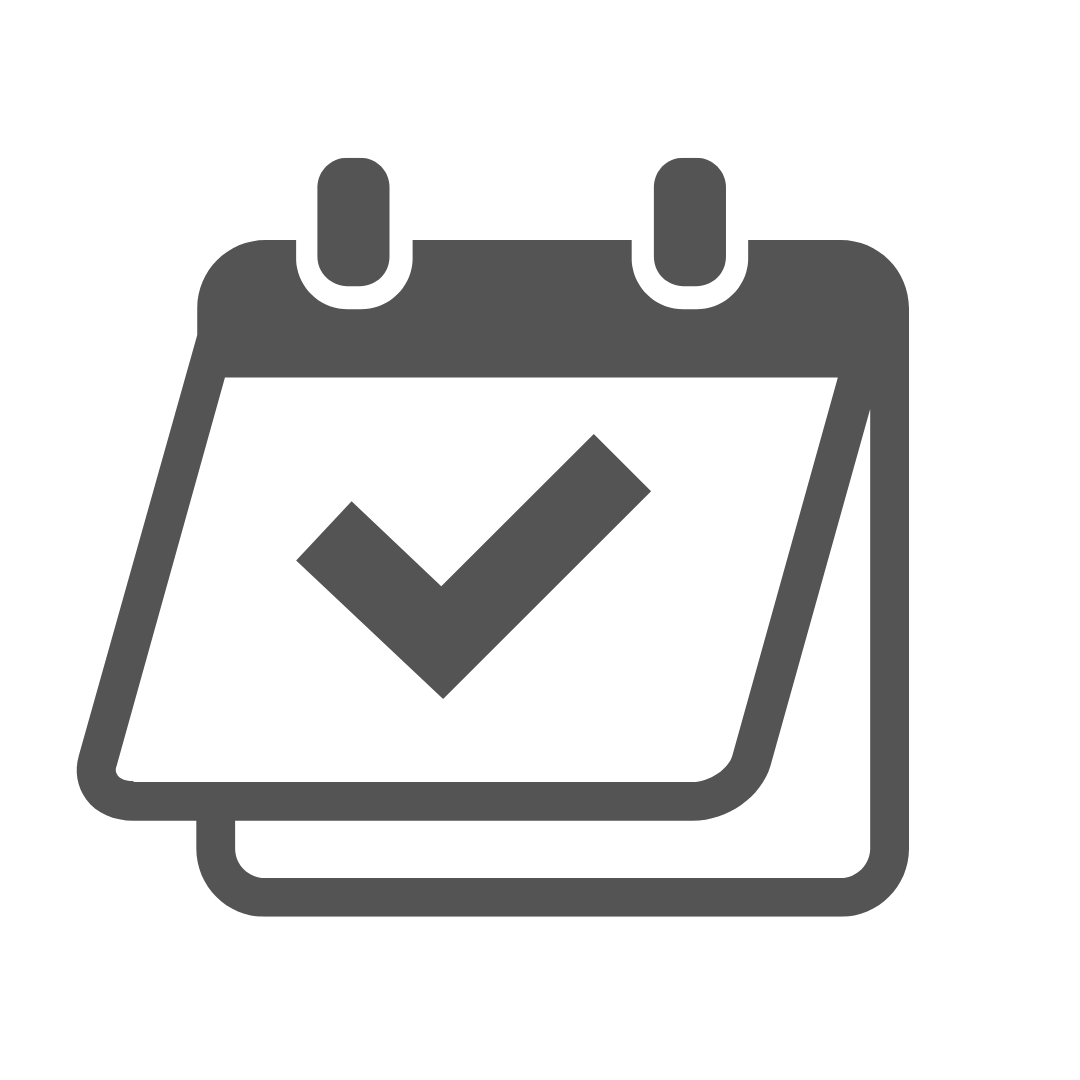
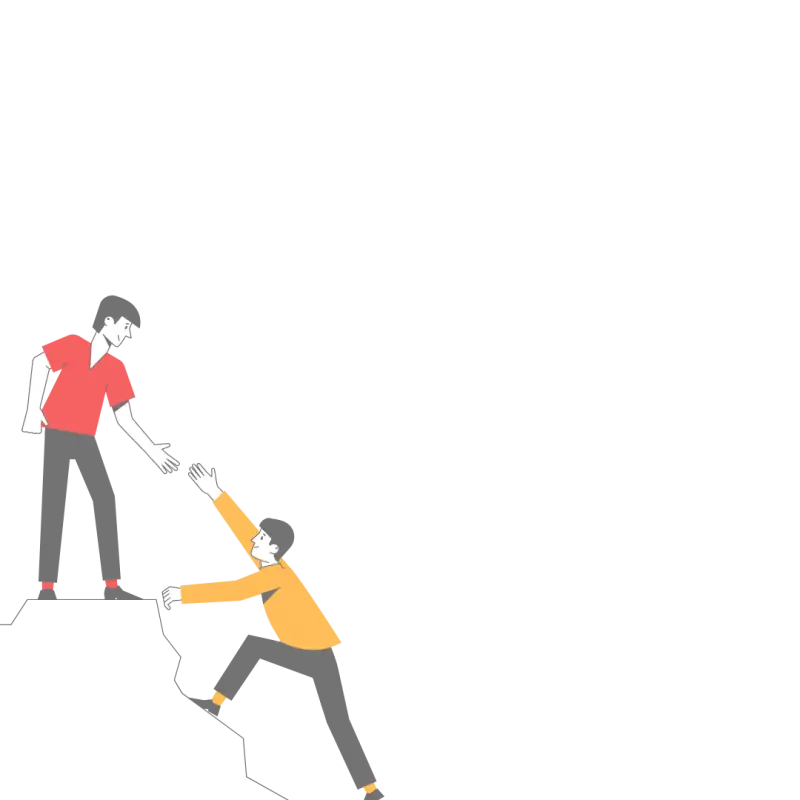
Thanks for your feedback!
Your contributions will help us to improve service.
What is the syntax in React.js to retrieve the first element of an array using array indexing?
The code snippet demonstrates how to retrieve the first element of an array using array indexing in ReactJS. The given array contains the values 'Google', 'Facebook', 'Bard', 'Chatgpt', and 'Instagram'.
By accessing the element at index 0 (array[0]
), the first element, 'Google', is assigned to the variable firstElement
.
The rendered output displays the heading "React Js Get First Element of Array" along with the value of firstElement
in a paragraph tag.
Output of React Js Get first Element of Array
What is the syntax for using array destructuring assignment in React.js to obtain the first element of an array?
In the given React.js code snippet, the first element of the array is obtained using array destructuring assignment. The array contains the values ['Google', 'Facebook', 'Bard', 'Chatgpt', 'Instagram'].
By using the destructuring syntax const [firstElement] = array;
, the first element of the array, which is 'Google', is assigned to the variable firstElement
.
This value is then rendered in the React component as the output.
How can you use the `slice()` method in React.js to retrieve the first element of an array?
In the given React.js code snippet, the slice() method is used to retrieve the first element of an array. The array contains five elements: 'Google', 'Facebook', 'Bard', 'Chatgpt', and 'Instagram'.
The slice() method is called on the array with parameters (0, 1) to extract a new array containing only the first element.
The [0] index is then used to access the first element from the newly created array
How can you use the shift()
method in React.js to retrieve the first element of an array?
In this React.js code snippet, the shift()
method is used to retrieve and remove the first element from an array called array
.
The value of the first element is stored in the variable firstElement
.
The component App
renders a container with a heading and a paragraph displaying the first element of the array.
How can you use the spread operator in React.js to obtain the first element of an array?
In this React.js code snippet, an array called array
is defined with several elements.
Using the spread operator (...
), the array is destructured, and the first element is assigned to the variable firstElement
.