React Js Check array or object contains specfic value
React Js Check array or object contains value:In React.js, you can check whether an array or object contains a specific value by using various methods. For arrays, you can utilize the includes()
method to determine if the value is present.
For example, myArray.includes('specificValue')
returns a boolean indicating whether the array contains the specified value. When dealing with objects, you can use the Object.values()
method to extract the object's values as an array, and then apply the includes()
method on that array.
Alternatively, you can iterate through the object's keys and compare the values manually. These techniques enable you to check for the presence of a specific value in an array or object in React.js.
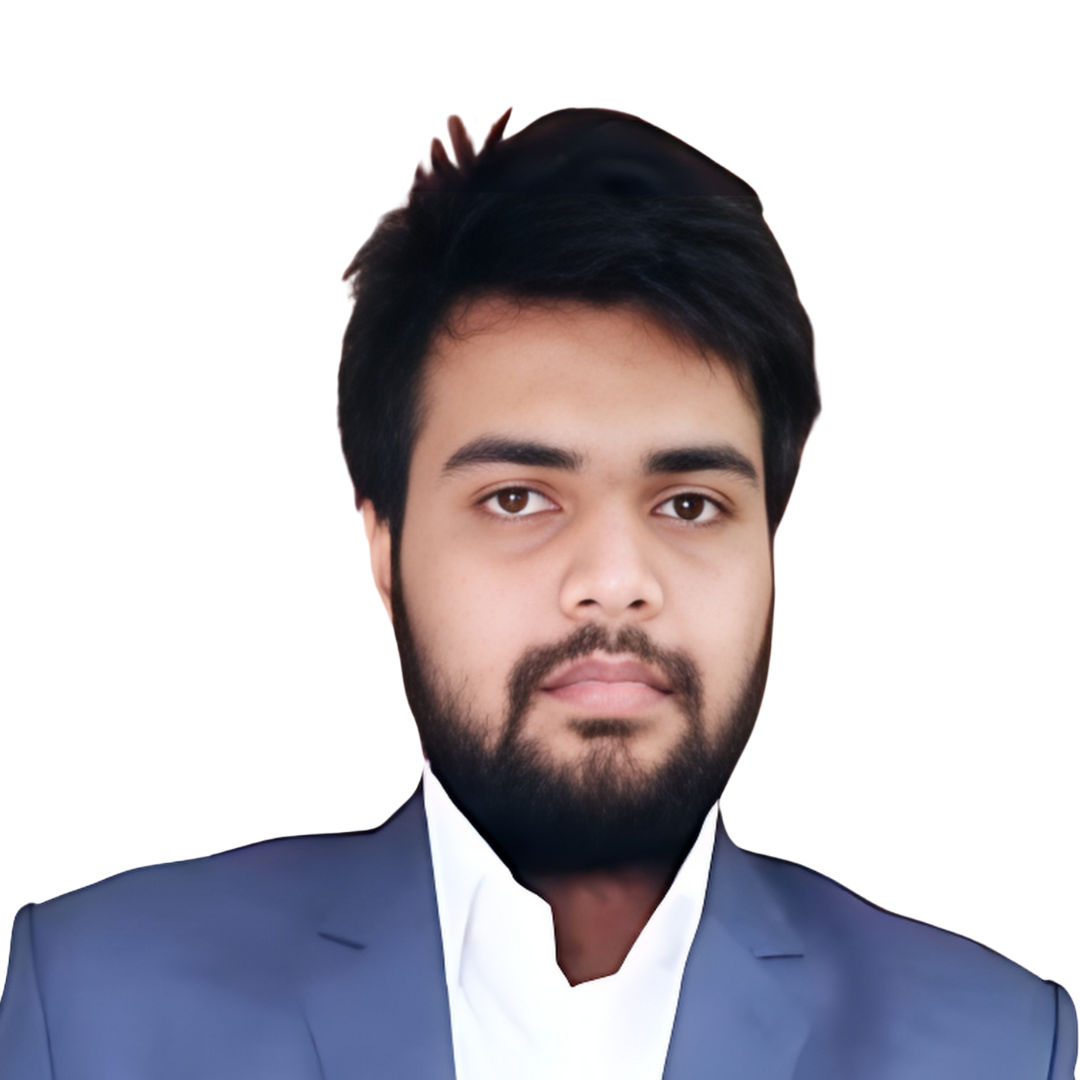
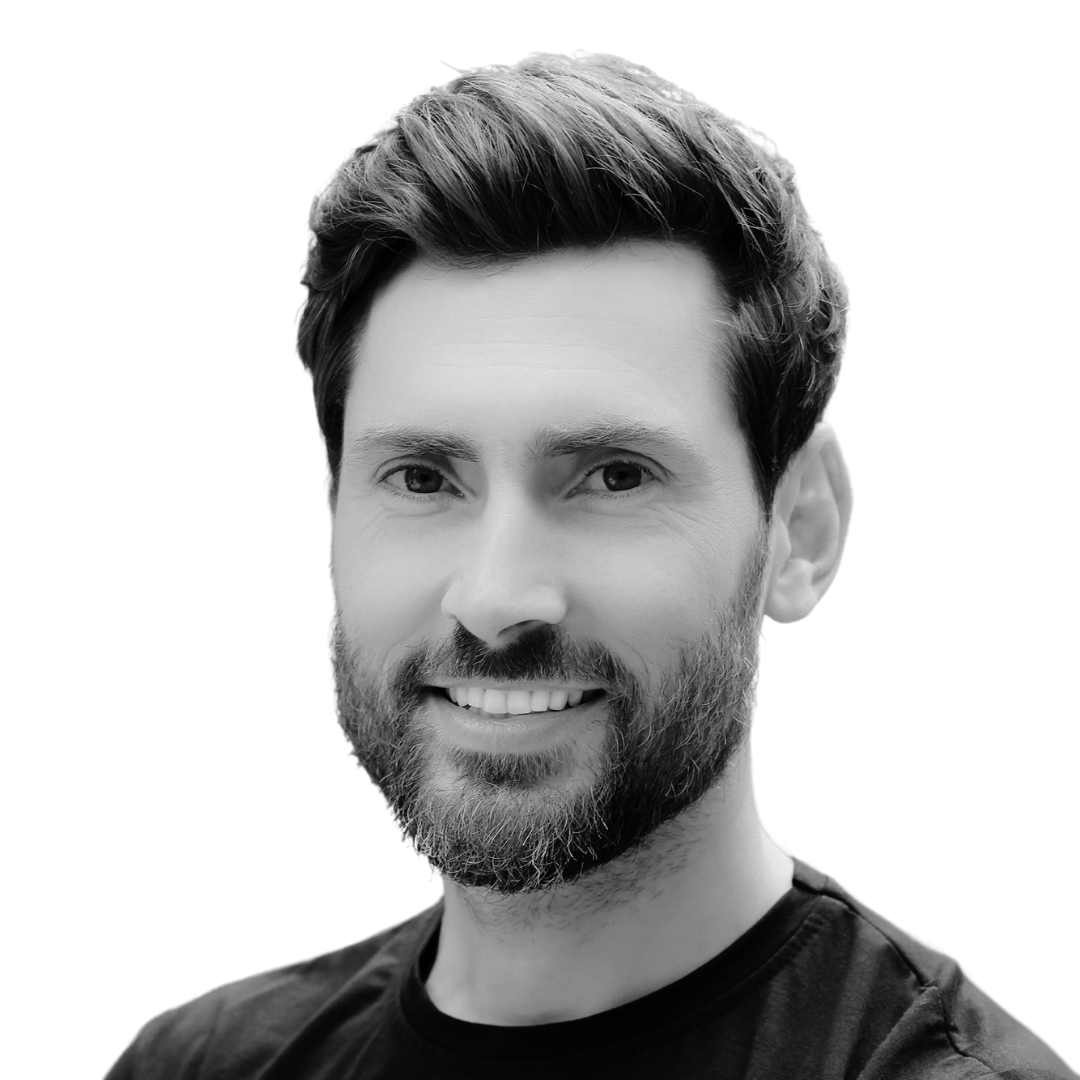
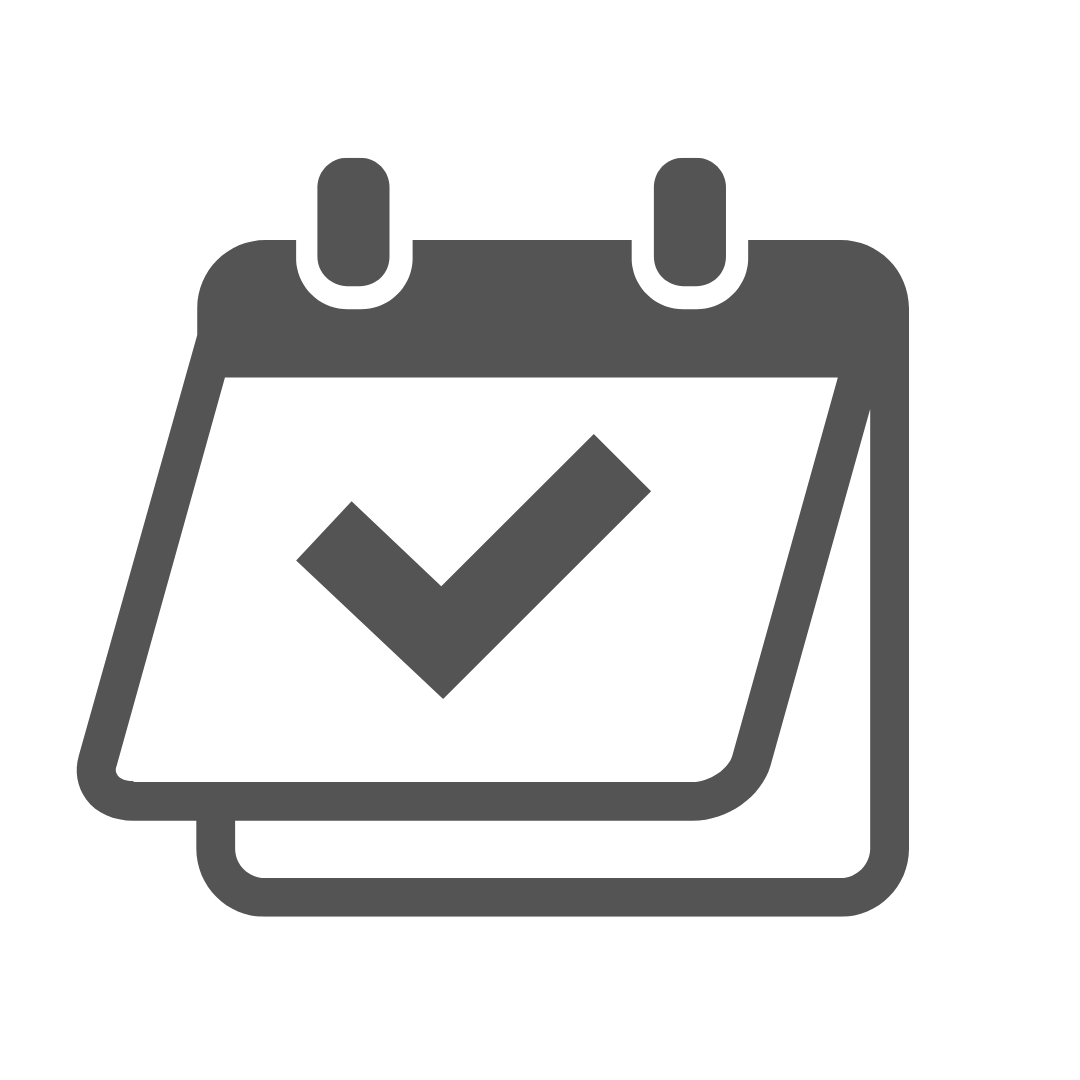
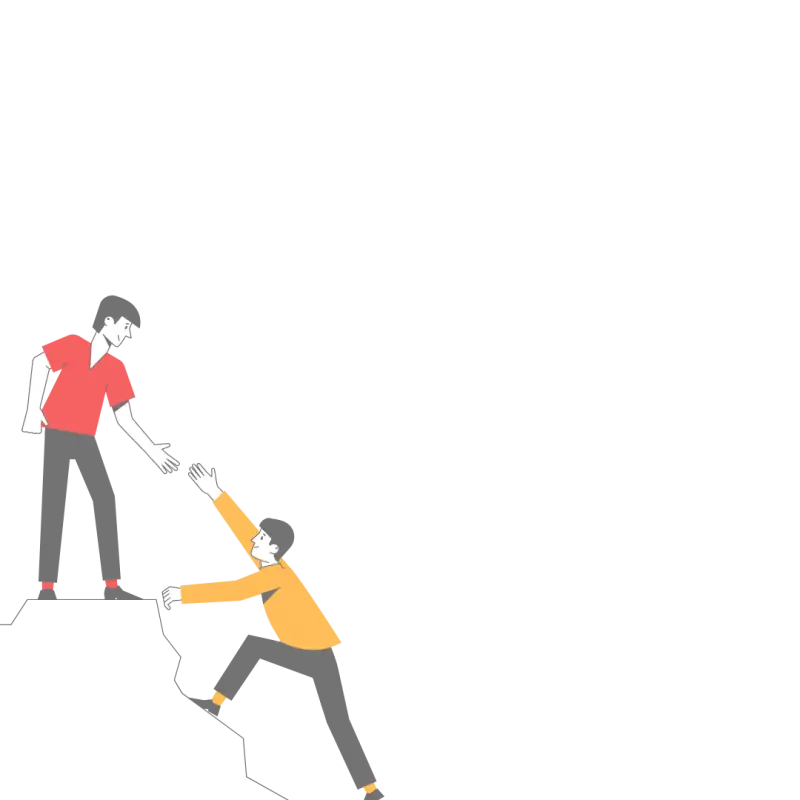
Thanks for your feedback!
Your contributions will help us to improve service.
How can you check if a specific value is present in an array using React.js?
The code snippet demonstrates how to check if a specific value exists in an array using React.js. The includes
method is used to determine whether the valueToCheck
(which is set to 'banana') is present in the myArray
(which contains 'apple', 'banana', and 'orange').
If the value is found in the array (isValueInArray
is true), a message is displayed stating that the array contains the value. Otherwise, if the value is not present in the array, a message is displayed indicating that the array does not contain the value.
How can I check if an array of objects in React.js contains a specific value?
This React.js code defines a functional component called App
that checks if a specific value exists in an array of objects. The array of objects contains three objects with properties id
and name
. The checkValueExists
function uses the some
method to iterate over the array and checks if any object's name
property matches the provided value (case-insensitive).
The component uses the useState
hook to manage the input value and the result. The input field allows the user to enter a value, which triggers the handleInputChange
function to update the inputValue
state. Clicking the "Check existence" button invokes the handleCheckExists
function, which calls checkValueExists
with the input value and updates the result
state accordingly.