React Js if else Conditional Rendering
React Js if else Conditional Rendering:In ReactJS, conditional rendering can be achieved in three main ways:
-
If...Else Statements: By using traditional JavaScript if...else statements within the render method, you can conditionally render components or elements based on certain conditions. This approach is useful for complex conditions and logic.
-
Ternary Operator: A concise approach, the ternary operator (conditional ? true : false) allows for inline conditionals, simplifying JSX code. It's ideal for rendering different content based on a single condition.
-
Logical Operators within JSX: You can use logical && (AND) or || (OR) operators within JSX to conditionally render components. This approach is great for rendering components when specific conditions are met, making the code more readable and concise.
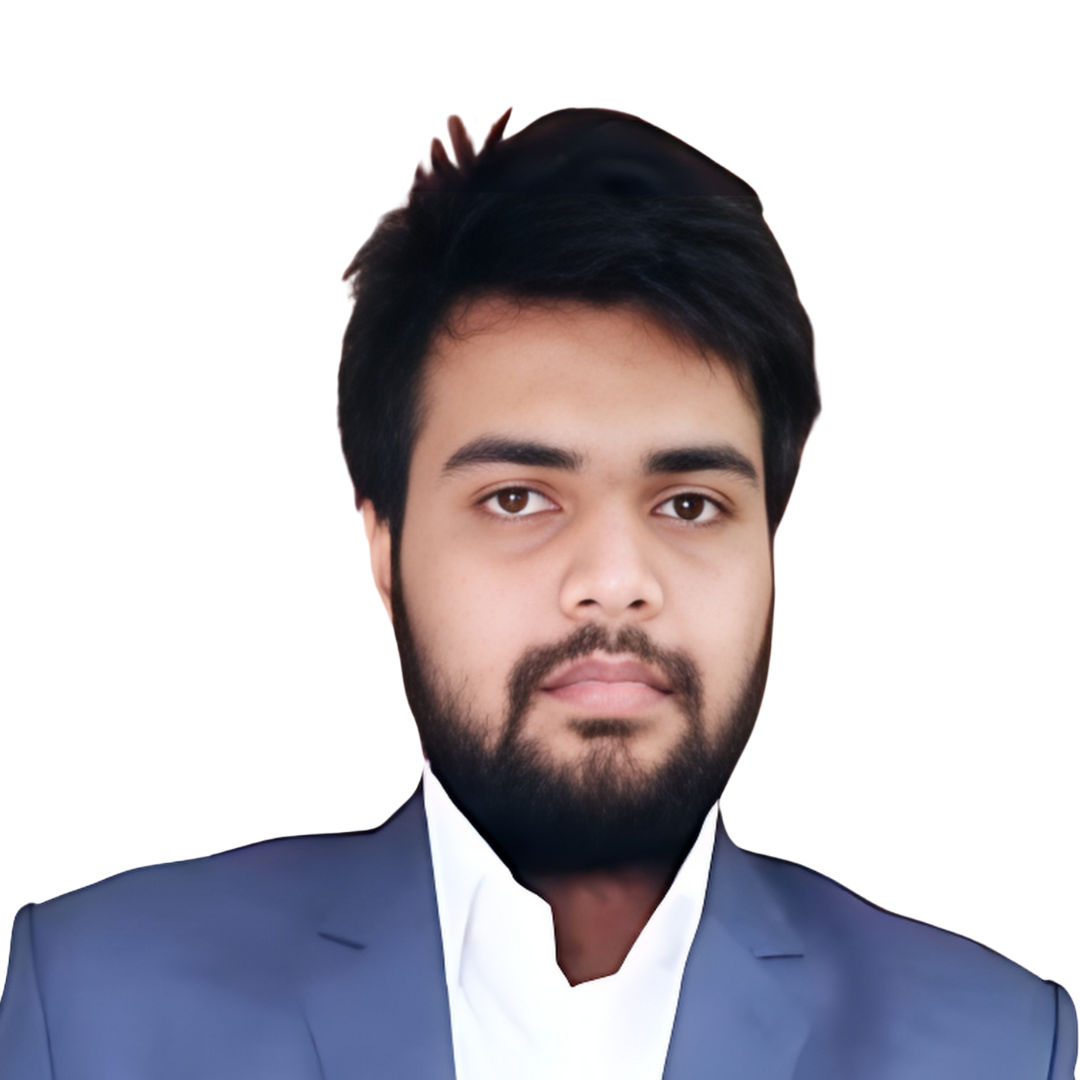
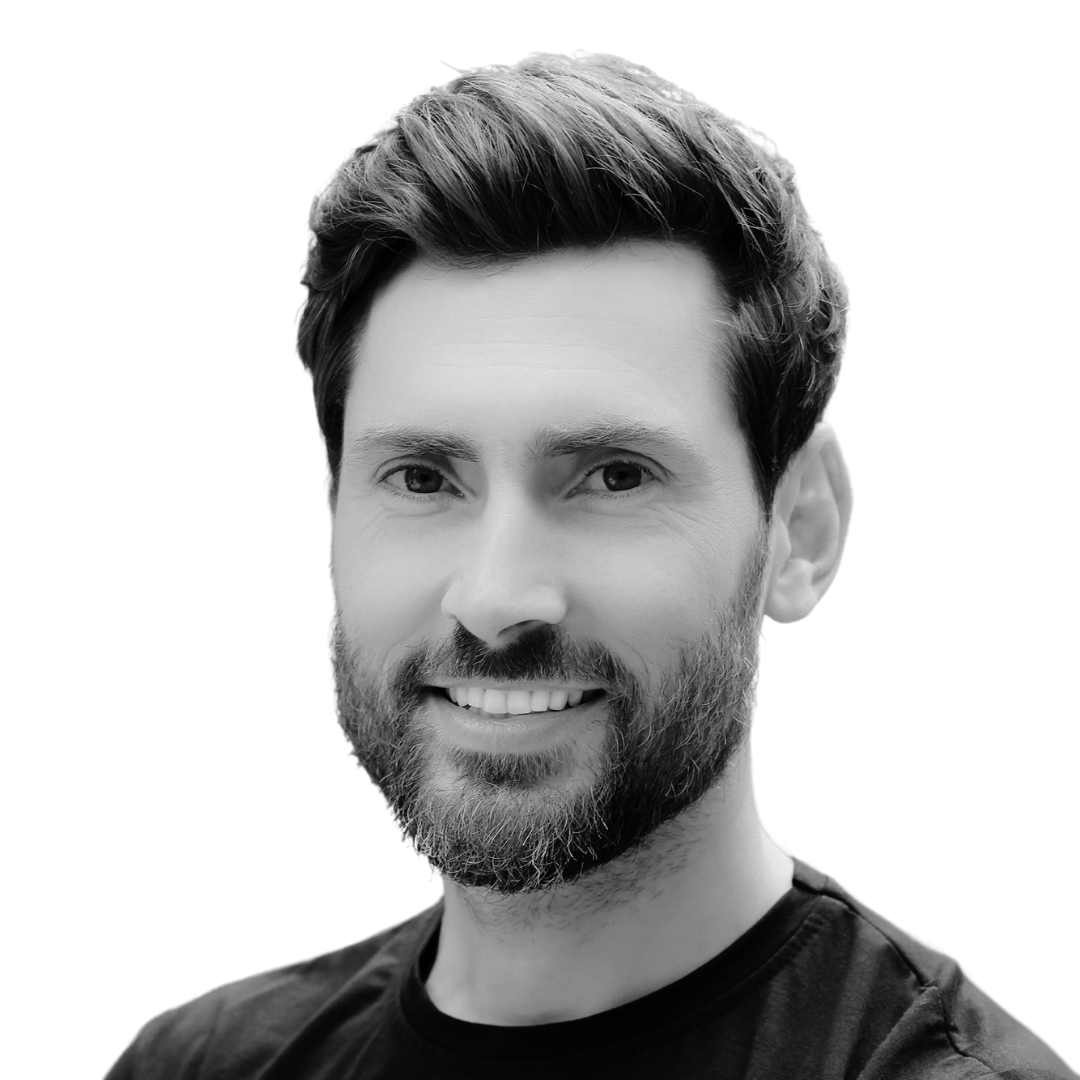
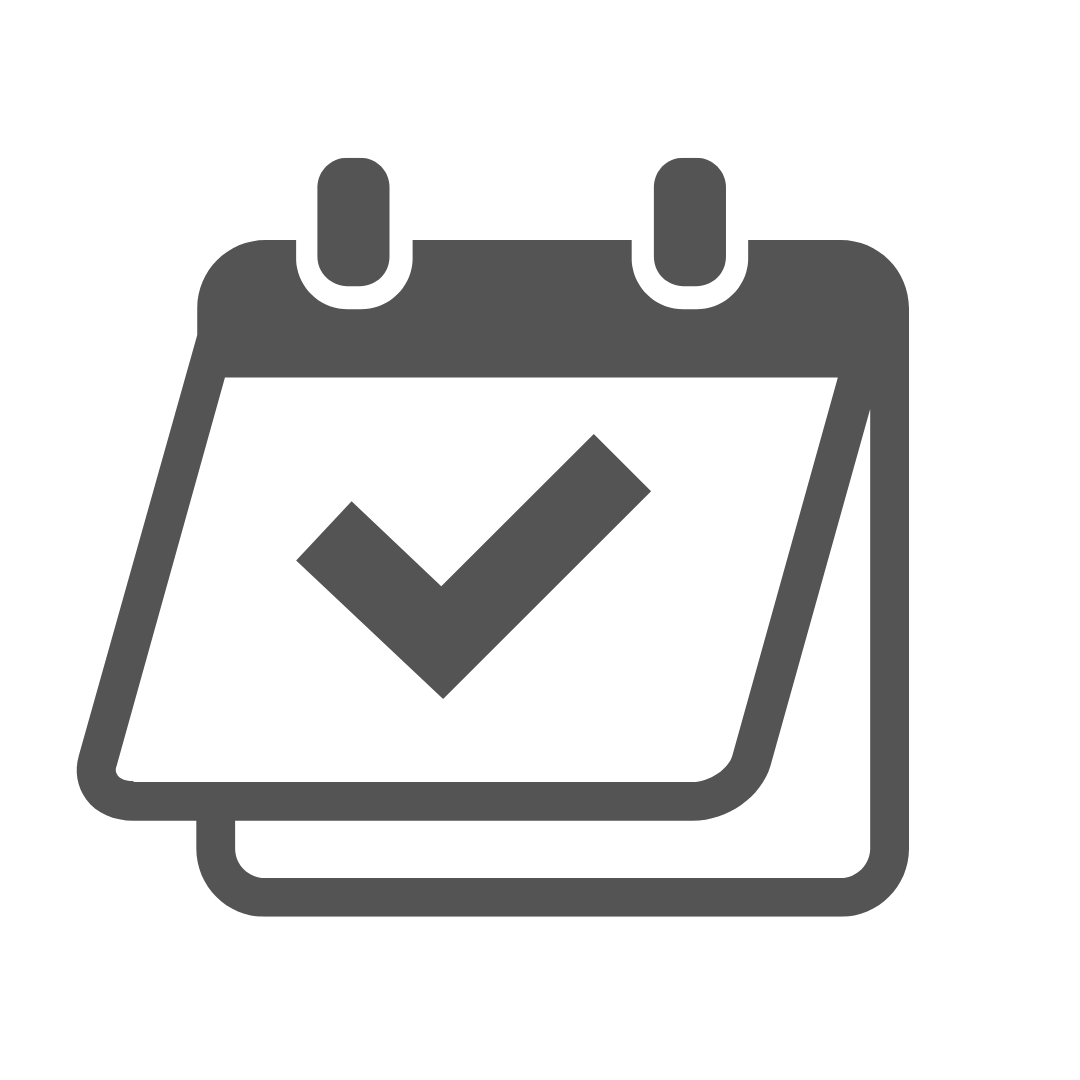
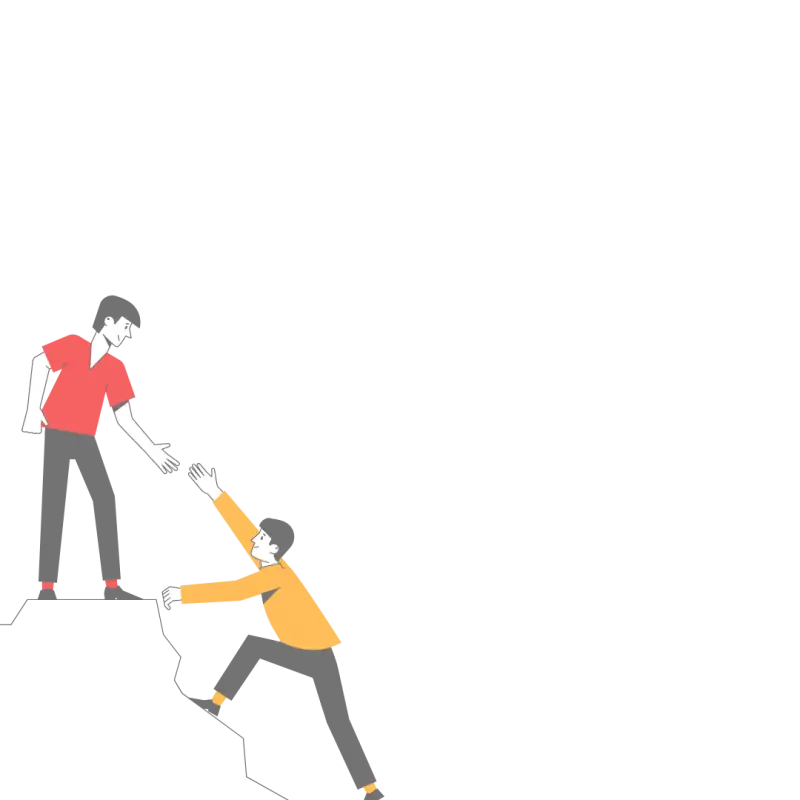
Thanks for your feedback!
Your contributions will help us to improve service.
How can you implement conditional rendering using if-else statements in a Reactjs ?
In this React.js code snippet, we create a component called App
. It contains a boolean variable condition
. Depending on whether condition
is true
or false
, it renders different content. If condition
is true
, it displays "Content when condition is true," and if it's false
, it displays "Content when condition is false." This is achieved using an if-else statement to assign the appropriate JSX element to the content
variable. Finally, the component renders a container with a heading and the content based on the condition
. This demonstrates conditional rendering in React.js based on the value of a variable.
Output of React Js If Else Conditional Rendering
Explain how Reactjs uses the ternary operator for conditional rendering?
In React.js, the ternary operator is employed for conditional rendering as seen in the provided code snippet. It evaluates the someValue
variable and displays different content based on its value. When someValue
is equal to 'someCondition', it renders the content inside the first <p>
tag with the class classNameForPTrue
. Conversely, if someValue
is not equal to 'someCondition', it renders the content inside the second <p>
tag with the class classNameForPFalse
. This approach allows developers to dynamically render components based on specific conditions, enhancing the flexibility and interactivity of React applications.