React Js Reverse String

React Js Reverse String:In React.js, reversing a string can be achieved in various ways. One approach is to use the split('').reverse().join('') method chain, which breaks the string into an array of characters, reverses their order, and then joins them back together. Alternatively, you can use a for loop to iterate through the string's characters in reverse order and build the reversed string. Another option is to employ the reduce method, accumulating characters in reverse order into a new string. Each method serves the same purpose, but the choice depends on readability, performance, and specific project requirements.
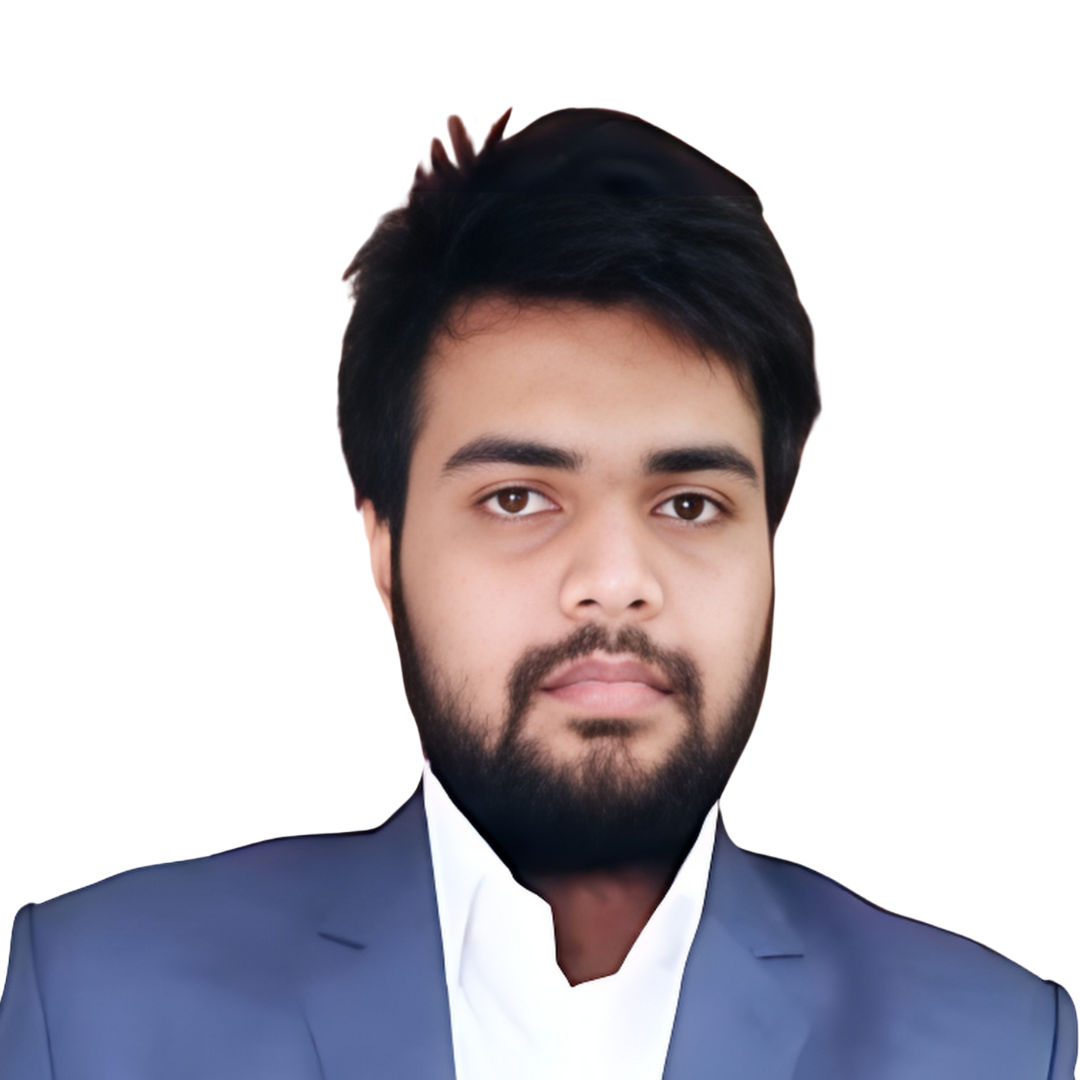
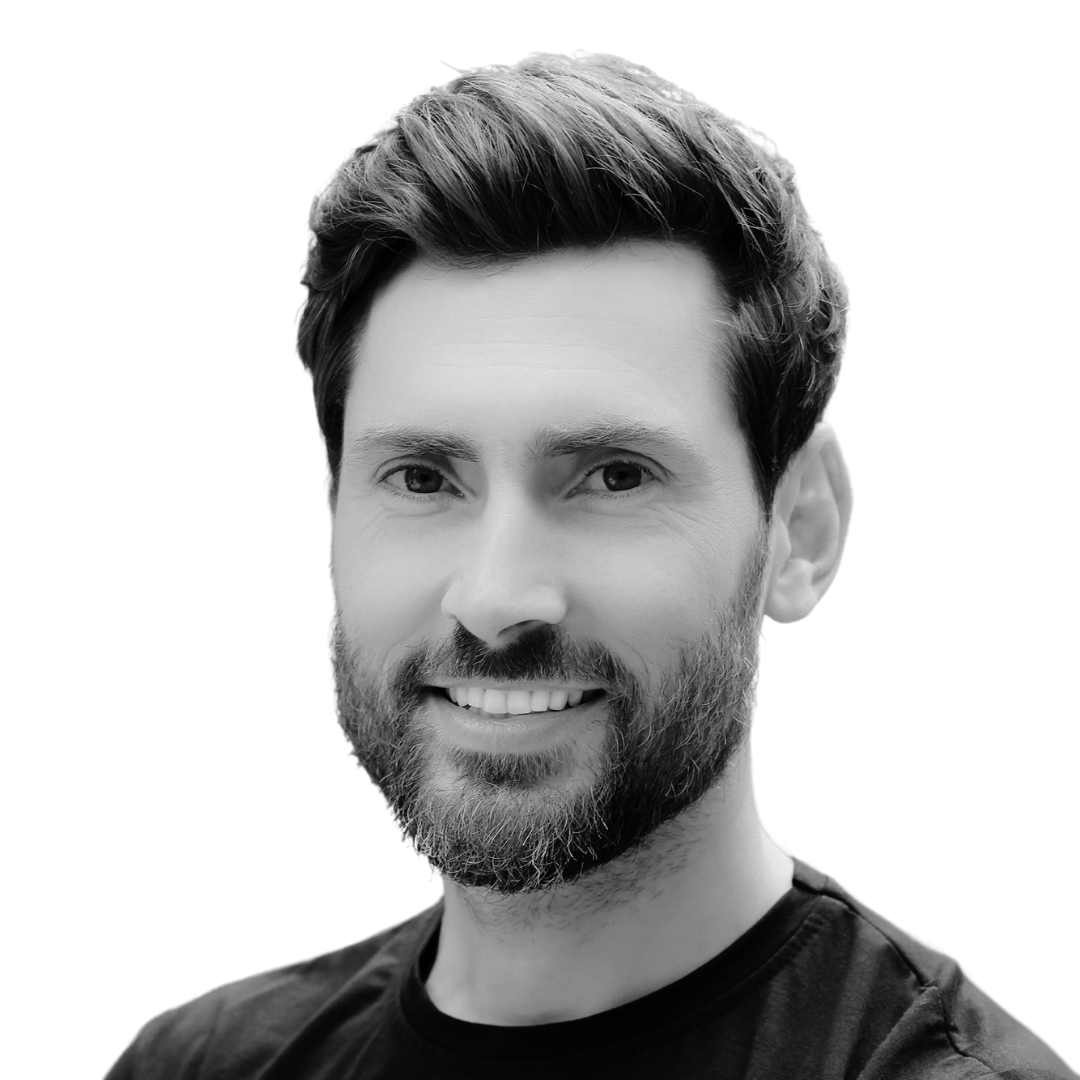
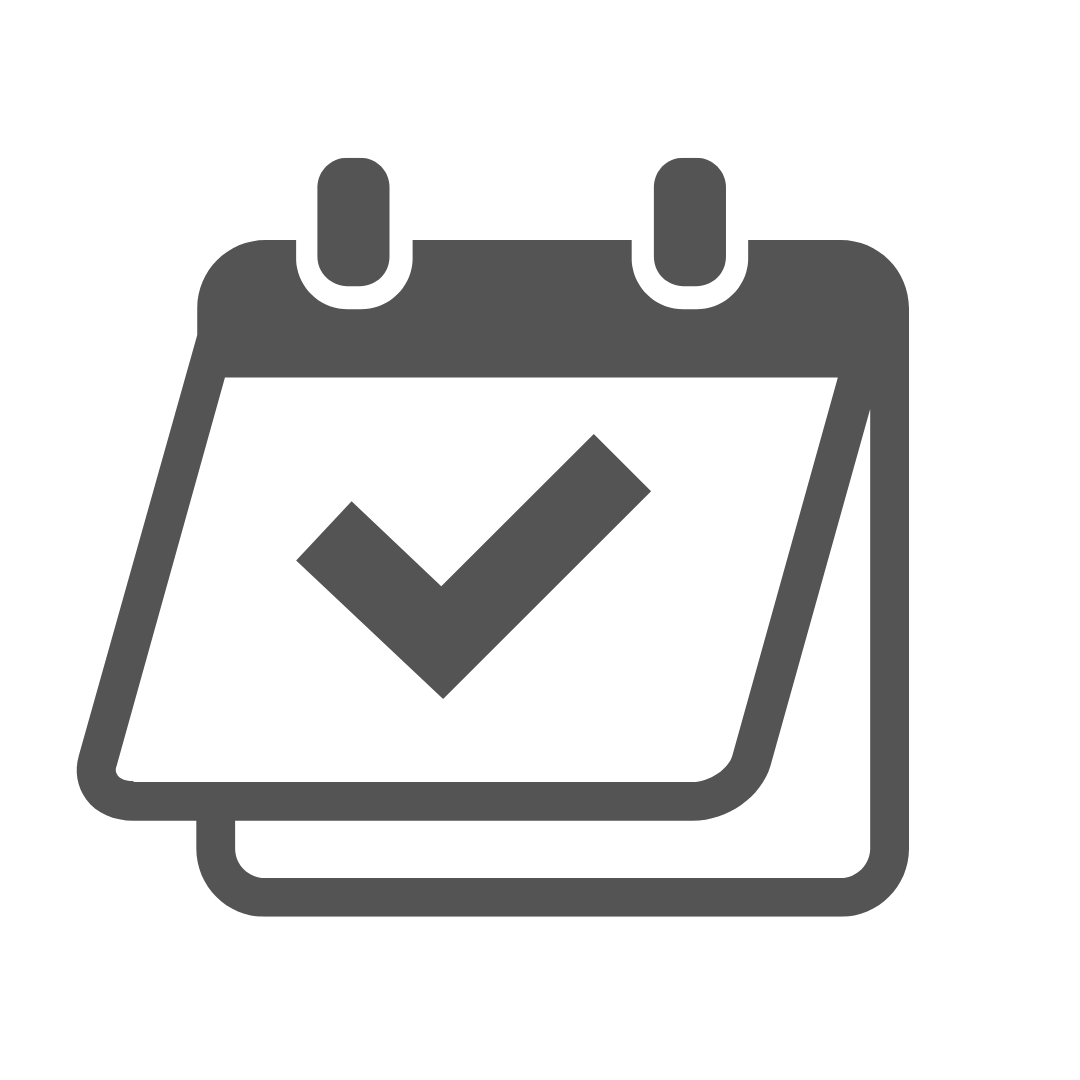
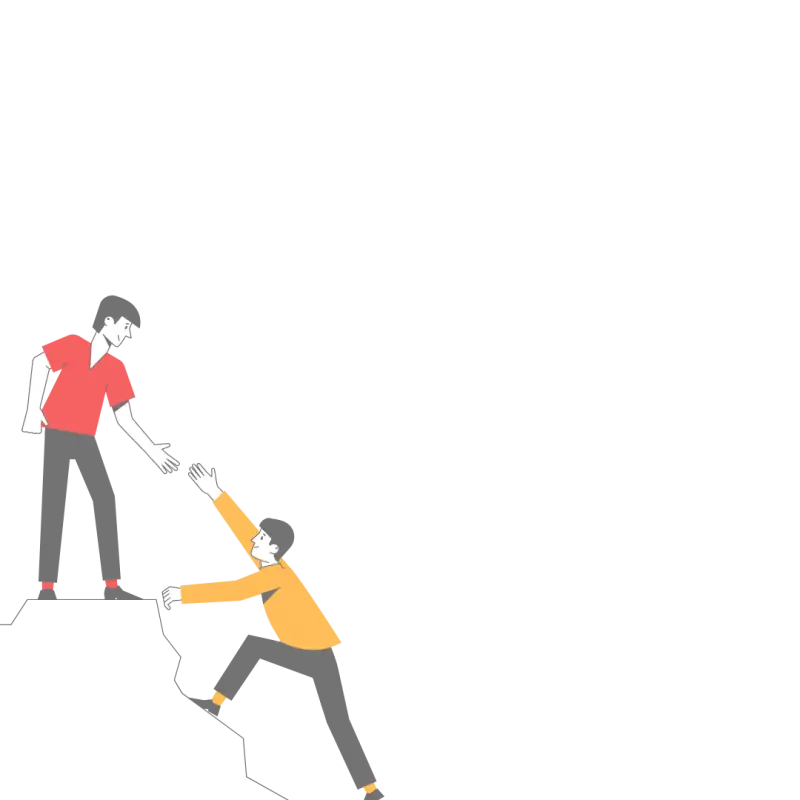
Thanks for your feedback!
Your contributions will help us to improve service.
How can you implement a reverse string functionality in a Reactjs application?
This React.js code snippet creates a simple web application that reverses a given input string. It utilizes React hooks, specifically useState
, to manage the input and reversed strings. Users can input a string in an input field, and when they click the "Reverse" button, the reverseString
function is triggered. This function reverses the input string and updates the reversedString
state
Output of React Js Reverse String
How do you reverse a string in Reactjs using a for
loop?
In this React.js code snippet, a functional component named App
is defined. It manages two state variables: inputString
and reversedString
. The reverseString
function uses a for loop to reverse the characters of the inputString
. Starting from the last character and iteratively adding each character to the reversed
variable, it effectively reverses the string. Finally, the setReversedString
function is used to update the reversedString
state with the reversed result
Output of React Js String Reverse
How can you use the reduce()
method in React.js to reverse a string efficiently?
In this React.js code snippet, the goal is to reverse a given string, "Welcome to Font Awesome Icons." This is achieved by first converting the string into an array of characters using the spread operator [...str]. Then, the reduce() method is applied to this array. During each iteration, it concatenates the next character (next) to the beginning of the accumulated string (prev). As a result, the characters are processed in reverse order, effectively reversing the original string. The final value of the reverse variable will contain the reversed string: "snocI emosewA tnoF ot emocleW."