React Js Scrollable List with Dynamic Font Size
React Js Scrollable List with Dynamic Font Size:A ReactJS scrollable list incorporates dynamically adjusting font sizes. As users scroll through the list, the font size of list items adapts based on their position. This enhances readability and conserves space. Utilizing React's state management, scroll position is tracked and translated into font size adjustments using CSS or inline styling. This creates a smooth visual experience, ensuring content remains legible while optimizing the display of information.
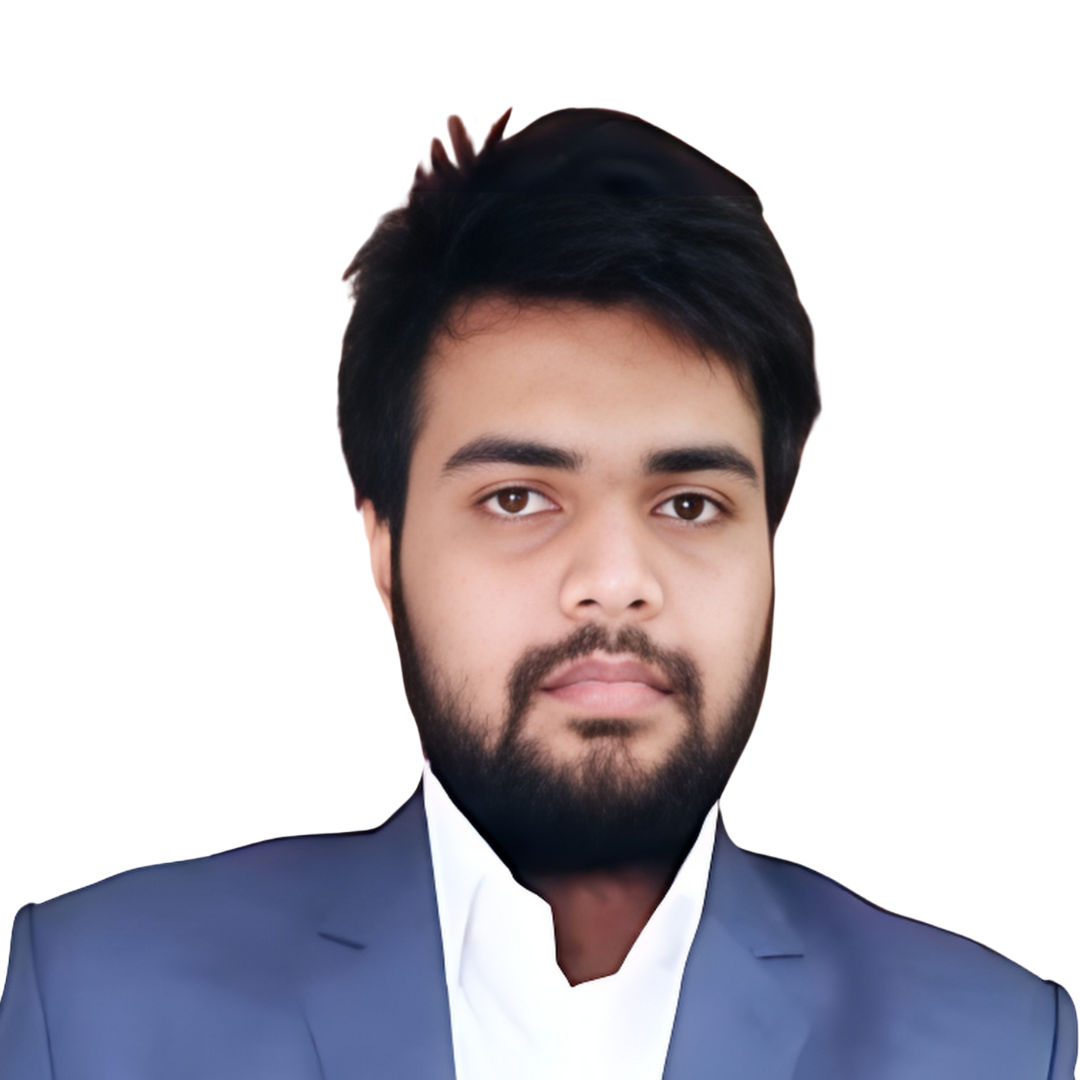
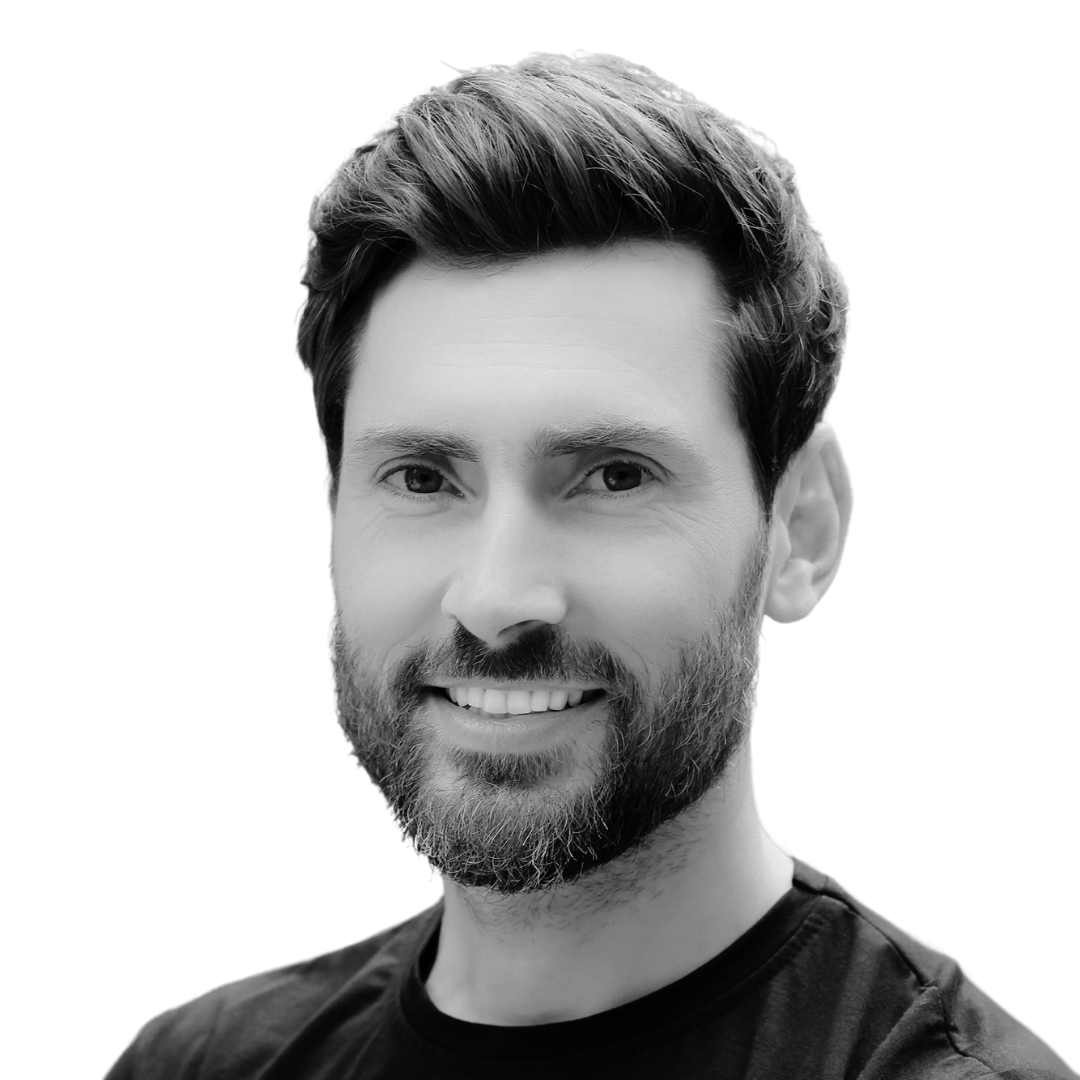
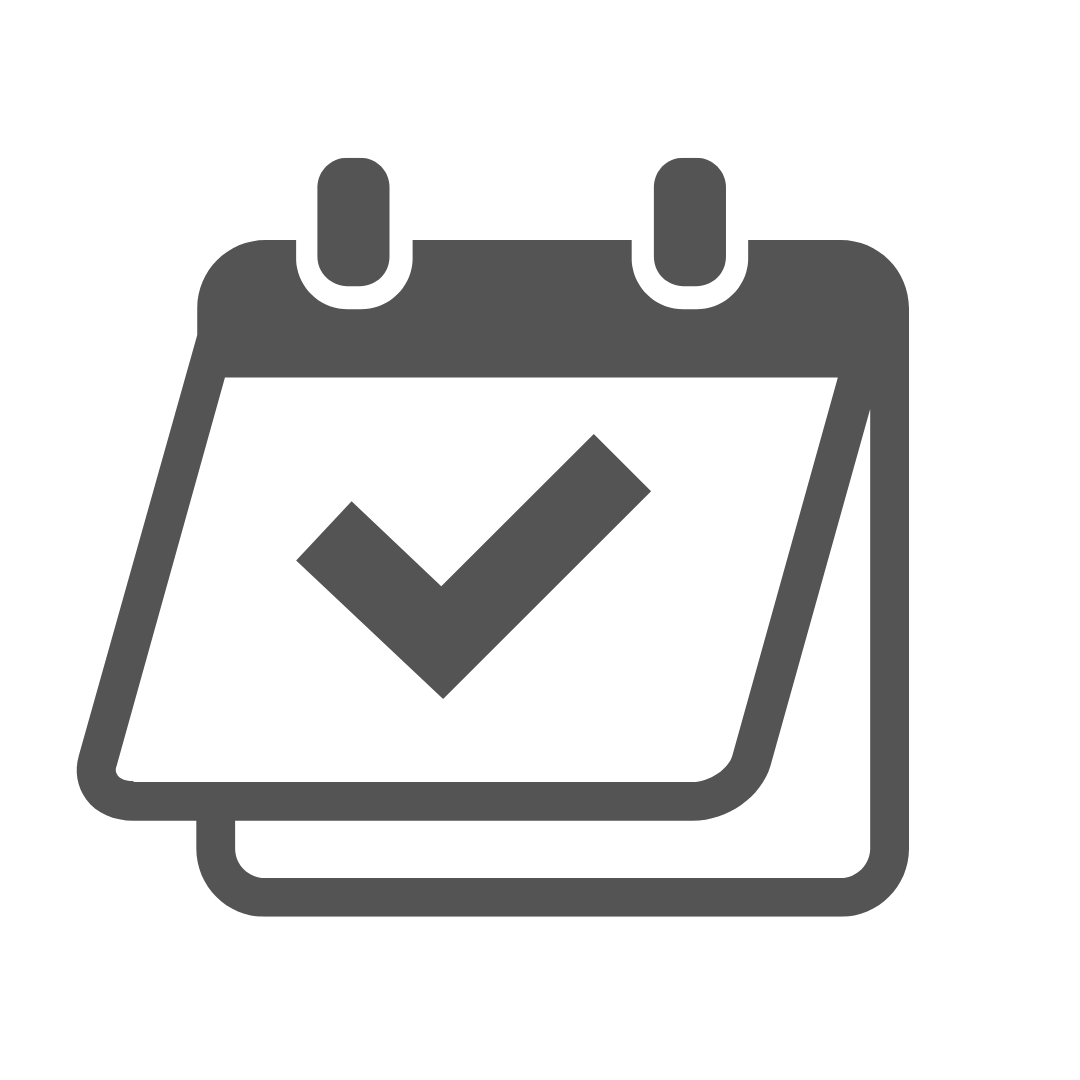
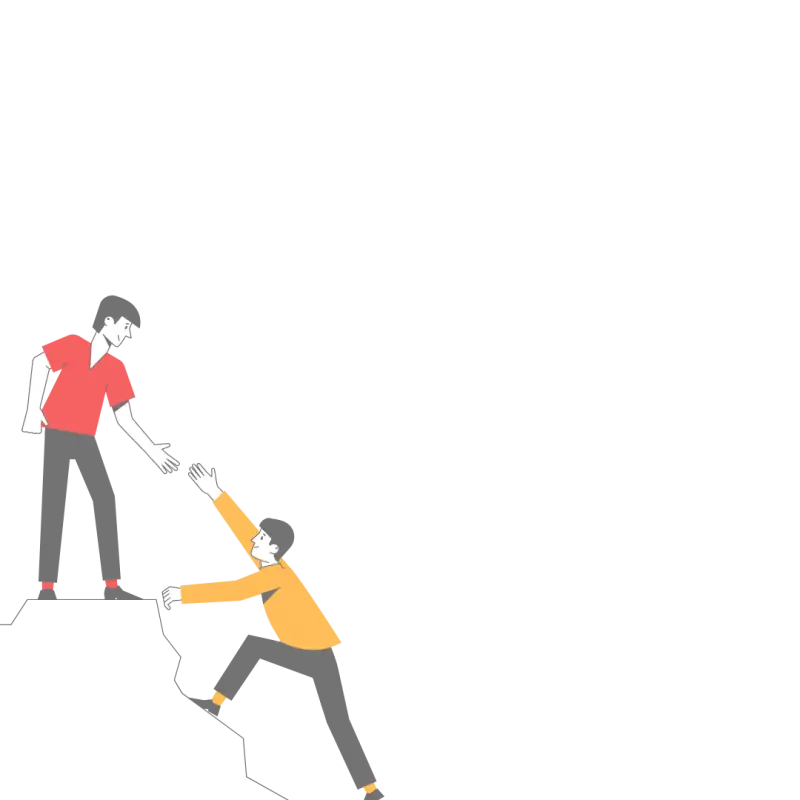
Thanks for your feedback!
Your contributions will help us to improve service.
How can I create a scrollable list in Reactjs with dynamically adjusting font size?
This Reactjs code snippet creates a scrollable list with dynamic font sizes. It imports necessary React functions, defines a functional component 'App', and initializes an array of items. The component uses the 'useState' hook to manage font sizes.
On mouse enter and leave events, the font size for each item is dynamically adjusted using the 'handleMouseEnter' and 'handleMouseLeave' functions. The 'calculateFontSize' function computes font sizes based on item index. The component renders a container, scrollable list, and iterates through items, adjusting font size and applying event handlers.