React Js Table with Checkbox | SelectAll
React Js Table with Checkbox | SelectAll:A React.js table with checkbox functionality and a "Select All" option allows users to select multiple rows of data. The table includes checkboxes for each row, enabling users to individually select or deselect specific rows. Additionally, a "Select All" checkbox can be provided at the table's header, allowing users to select or deselect all rows at once. This functionality enhances user experience by providing a convenient way to manipulate data in bulk, such as performing batch actions or applying filters. It is commonly used in applications that require managing large datasets or performing operations on multiple rows simultaneously.
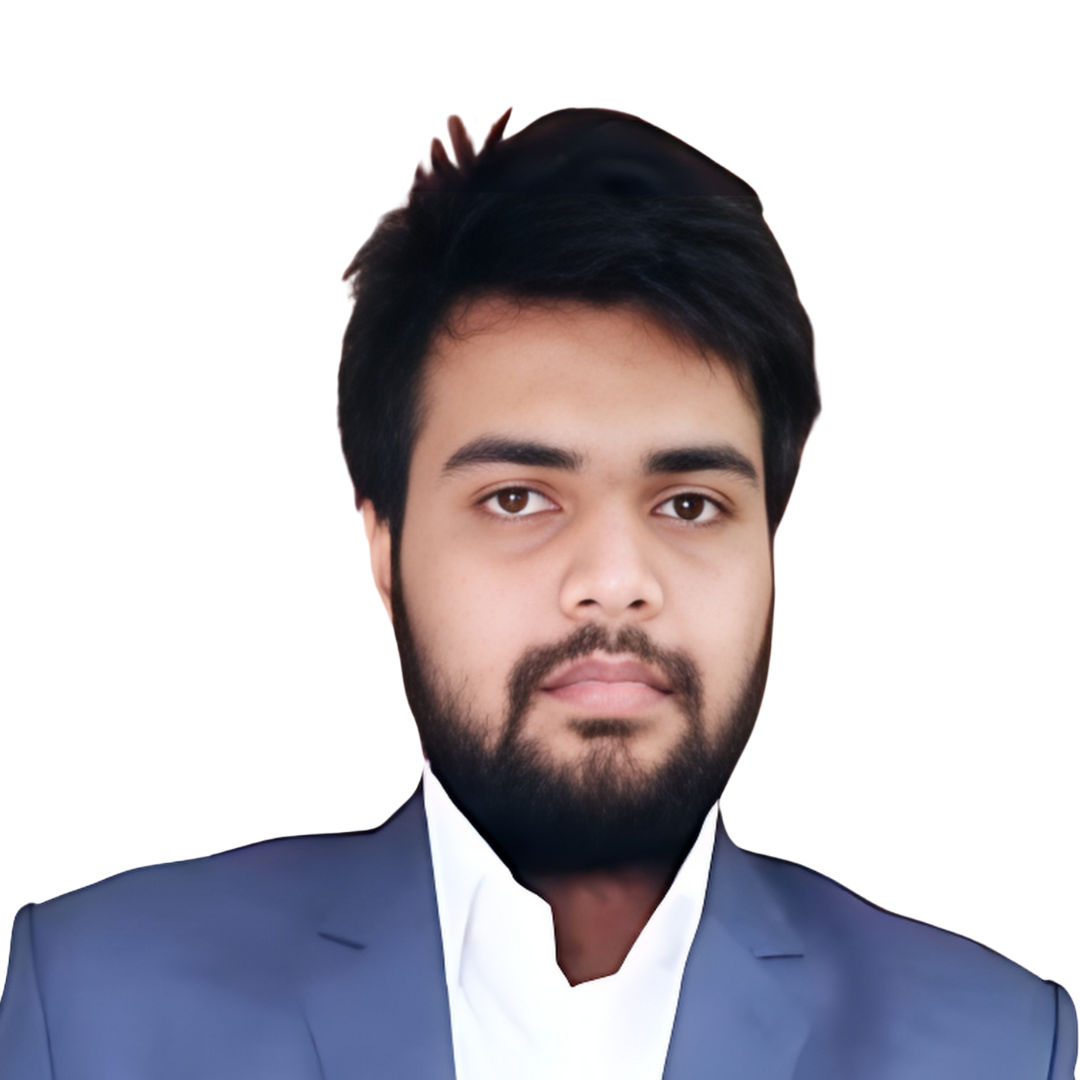
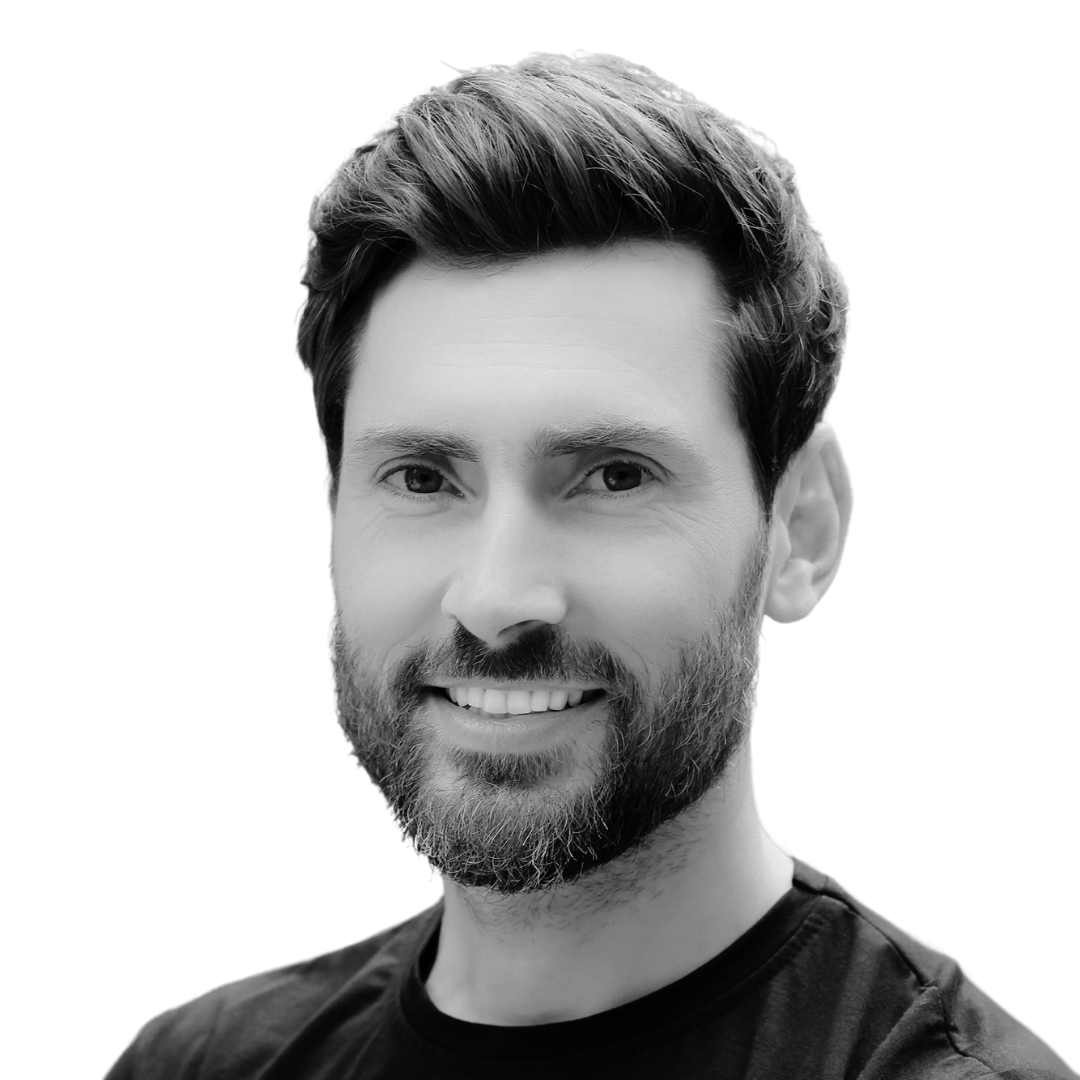
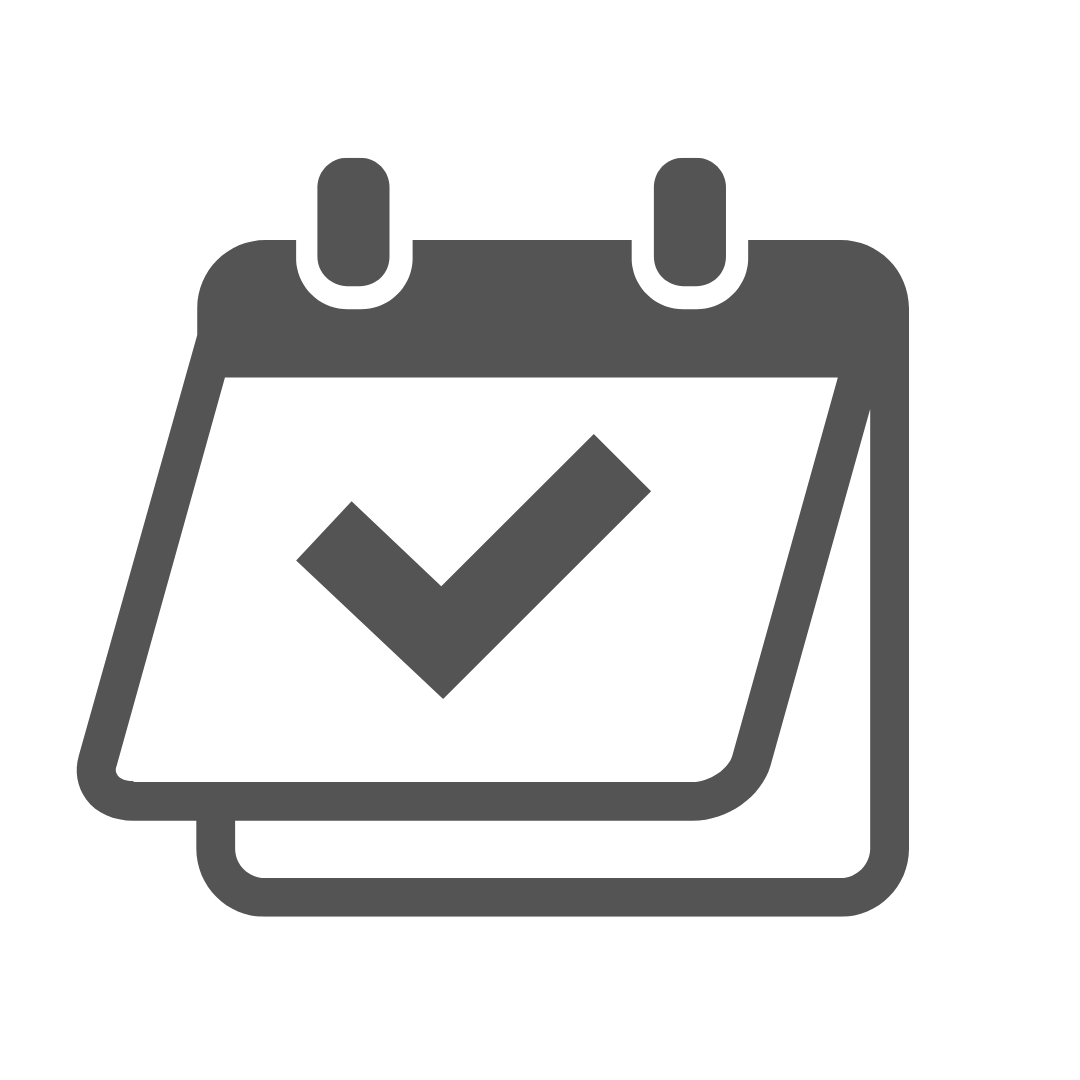
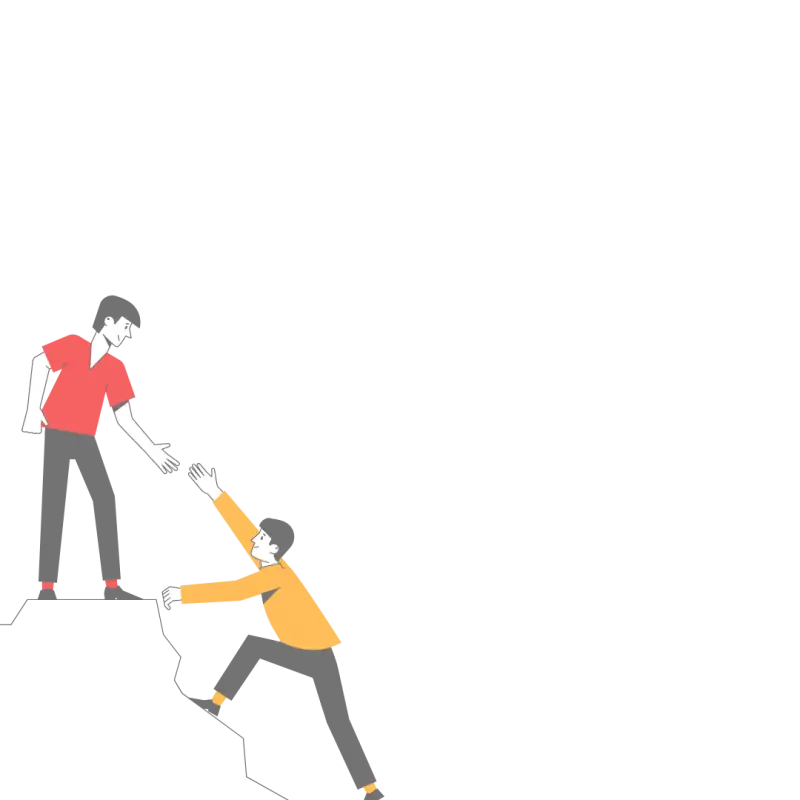
Thanks for your feedback!
Your contributions will help us to improve service.
How can I create a table in React.js with checkboxes for each row and a "Select All" checkbox that selects/deselects all the checkboxes in the table?
his is a React.js component that renders a table with checkboxes and a "Select All" feature. The component uses React hooks, specifically the useState
hook, to manage the state of the component.
The component initializes an array of users
with their properties such as id
, name
, email
, and age
. It also sets up two additional states: selectedUsers
to keep track of the selected users and isAllSelected
to indicate whether all users are currently selected.
The selectAll
function is called when the "Select All" checkbox is clicked. It clears the selectedUsers
array and then either selects or deselects all users based on the current value of isAllSelected
.
The handleUserSelect
function is called when a user checkbox is clicked. It adds or removes the user's name from the selectedUsers
array based on whether it is already selected or not.
The isUserSelected
function checks if a specific user is selected by checking if their name is present in the selectedUsers
array.
The isAllUsersSelected
function checks if all users are selected by comparing the length of the selectedUsers
array with the total number of users.
The JSX code renders the table with checkboxes. The "Select All" checkbox is bound to the isAllSelected
state and triggers the selectAll
function when changed. Each user row has an associated checkbox bound to the isUserSelected
function and triggers the handleUserSelect
function when changed.
The selected user names are displayed in a paragraph with the class "selected-users".