React Js Set Focus on Input field after rendering

React Js Set Focus on Input:To set focus on an input field in ReactJS after rendering, you can use the useRef hook provided by React. First, create a ref using useRef and attach it to the input element using the ref attribute. Then, in the useEffect hook, use the ref's current property to access the input element and call the focus() method. This will set the focus on the input field when the component is rendered. Remember to include the ref as a dependency in the useEffect hook to ensure it runs only once.
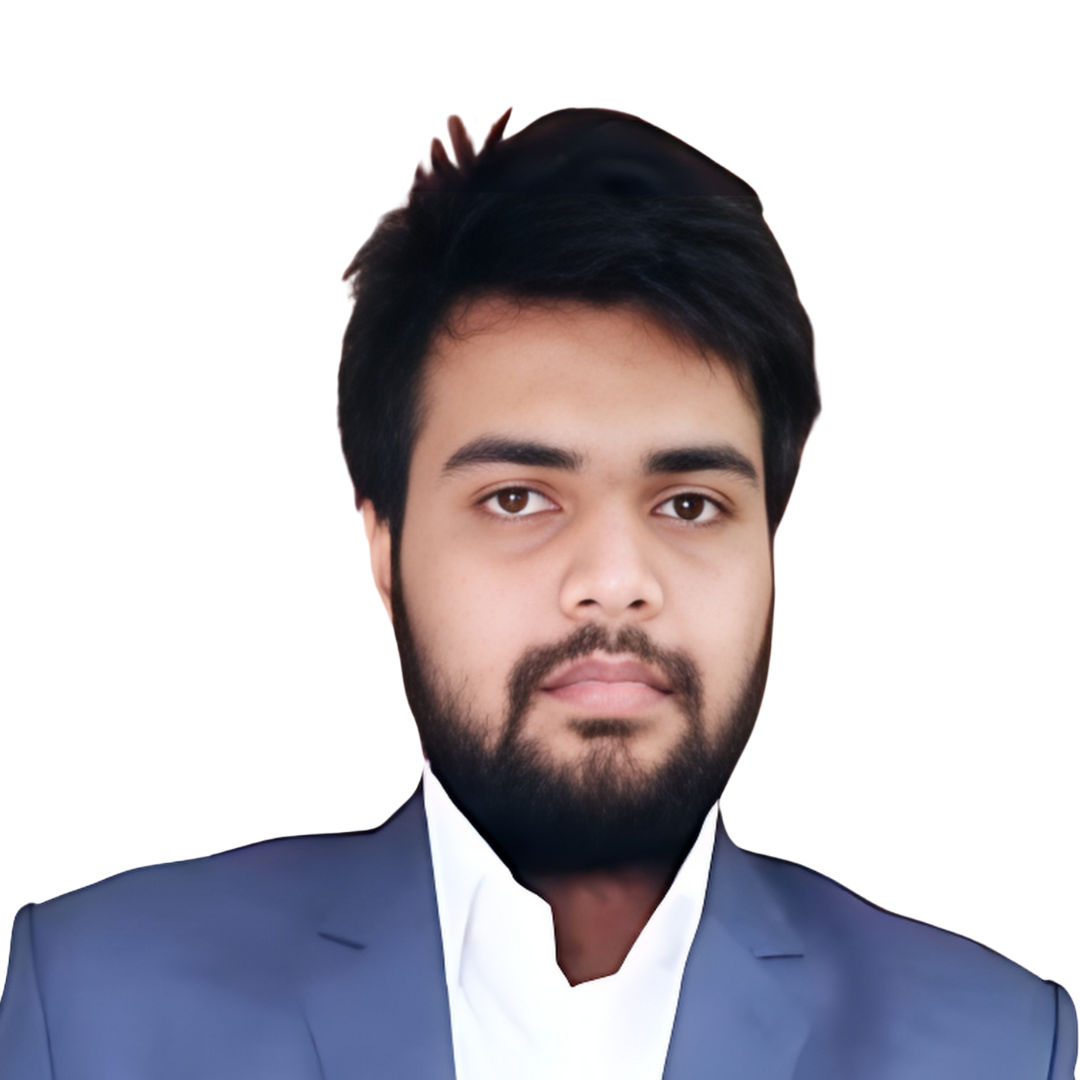
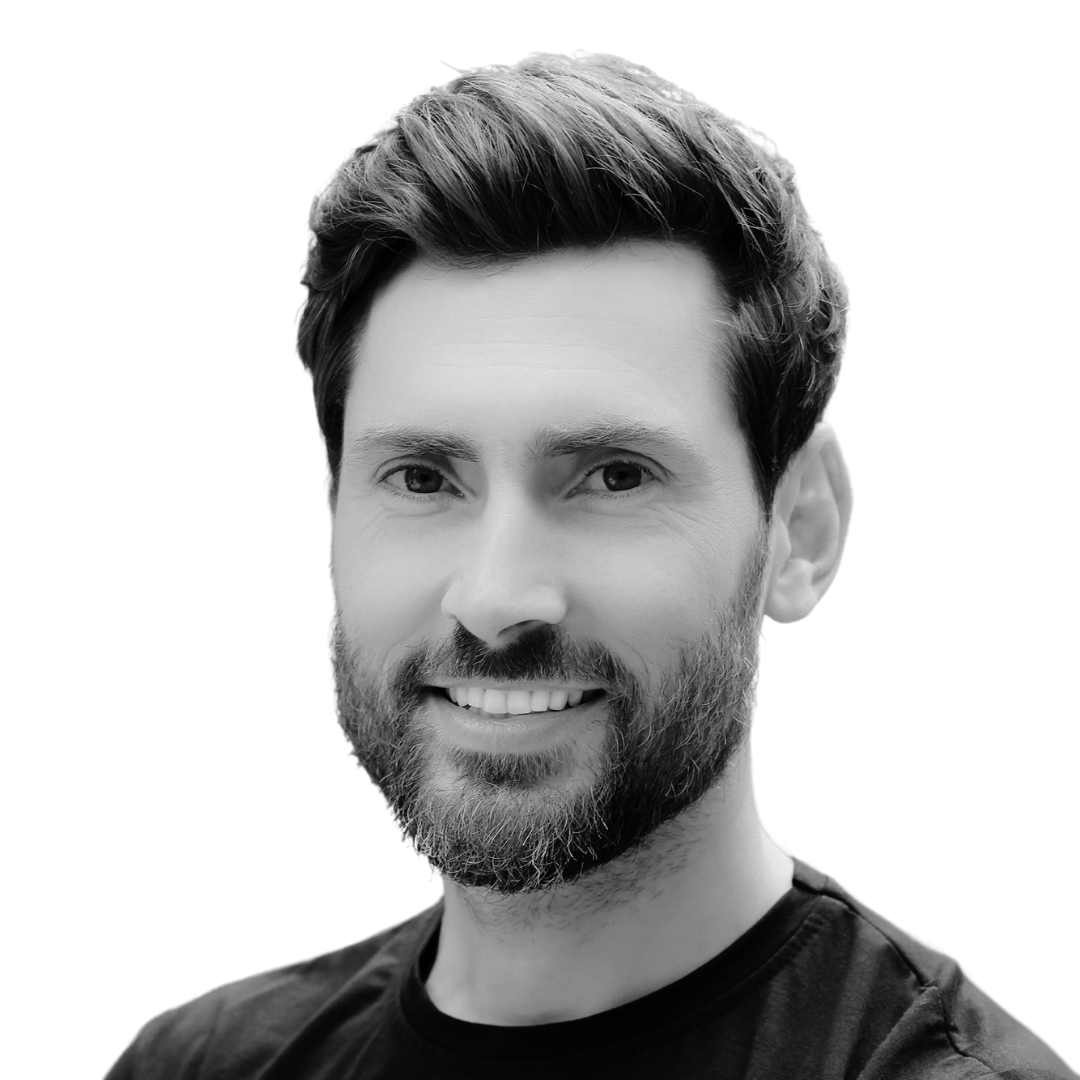
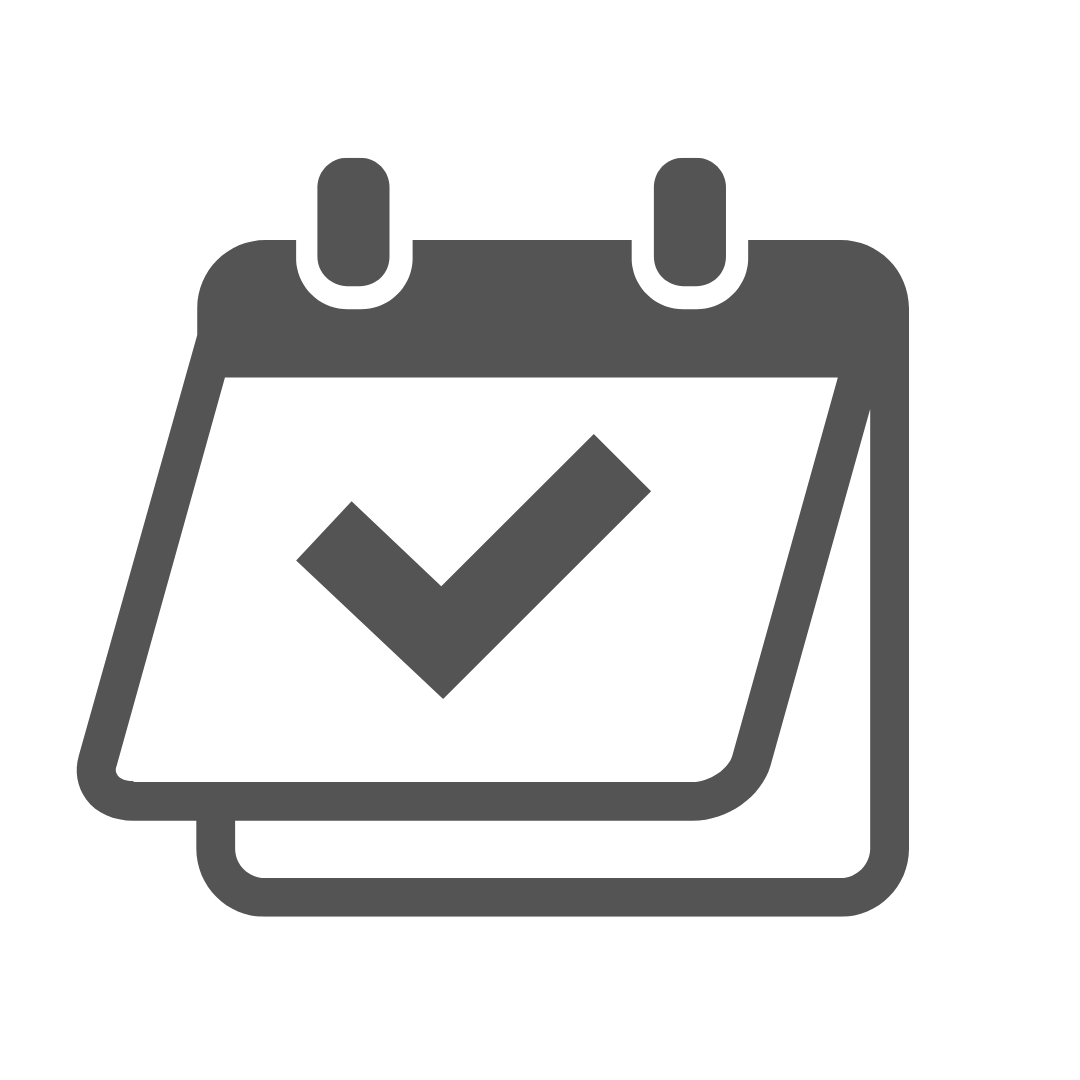
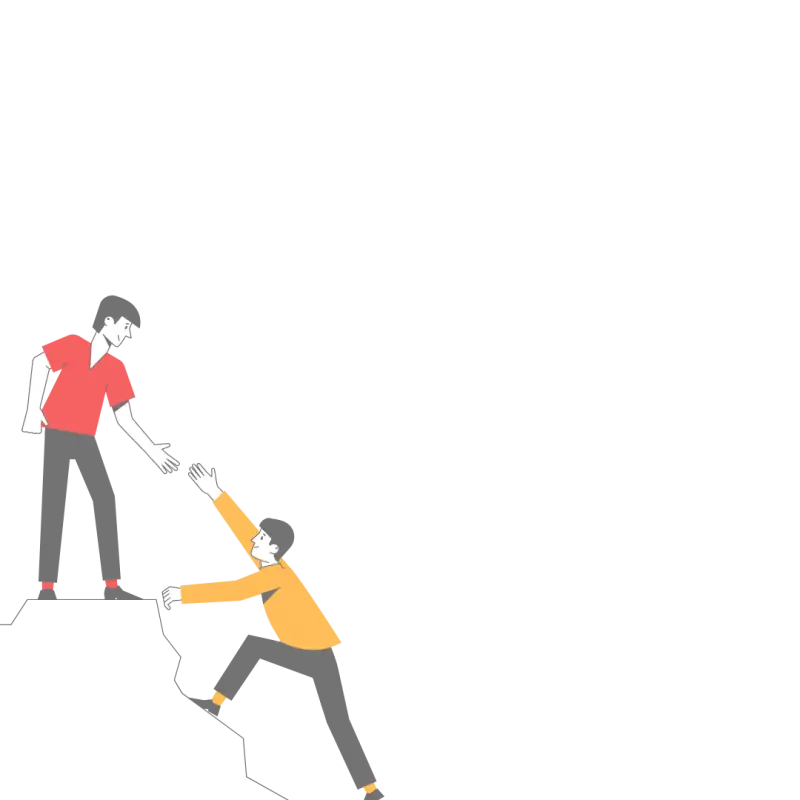
Thanks for your feedback!
Your contributions will help us to improve service.
How can you set focus on an input element in ReactJS?
In this React JS code snippet, the useRef
and useEffect
hooks are imported from the React library. The inputRef
variable is created using the useRef
hook and is assigned to the input element.
The useEffect
hook is used to execute code after the component has rendered. In this case, it's used to set focus on the input field. Inside the useEffect
callback, inputRef.current.focus()
is called, which focuses on the input element.
The App
component renders a container div with a heading and an input field. The input field is assigned the ref
attribute with the inputRef
variable.
Output of React Js Set Focus on Input
How can you set the focus on a specific link in React.js after rendering?
This code snippet demonstrates how to set focus on a link element in React.js after rendering. The code defines a functional component called App
. Inside the component, a useRef
hook is used to create a reference called linkRef
that will be attached to the link element.
The useEffect
hook is then used to handle the focus logic. It runs once, immediately after the component has rendered, indicated by the empty dependency array []
. Within the useEffect
callback, it checks if the linkRef.current
exists (i.e., the link element is rendered) and then calls the focus()
method on it to set the focus on the link.