React Js Array Find Method
React Js Array Find MethodThe Reactjs Array find()
method is a built-in function used to search and retrieve elements from an array that meet certain conditions. It takes a callback function as an argument and returns the first element that satisfies the provided condition. For instance, to find an element in an array that is greater than 10, you would write: array.find((element) => element > 10)
.
To find an object property by its id
, use array.find((obj) => obj.id === targetId)
.
For locating an element in an array with the value "apple", utilize array.find((element) => element === "apple")
.
To find an element with a specific property name
, you can use array.find((obj) => obj.name === targetName)
.
Lastly, to find an object with properties "name" and "age", you'd write array.find((obj) => obj.name && obj.age)
. The find()
method provides a concise way to retrieve elements based on various criteria in React.js applications.
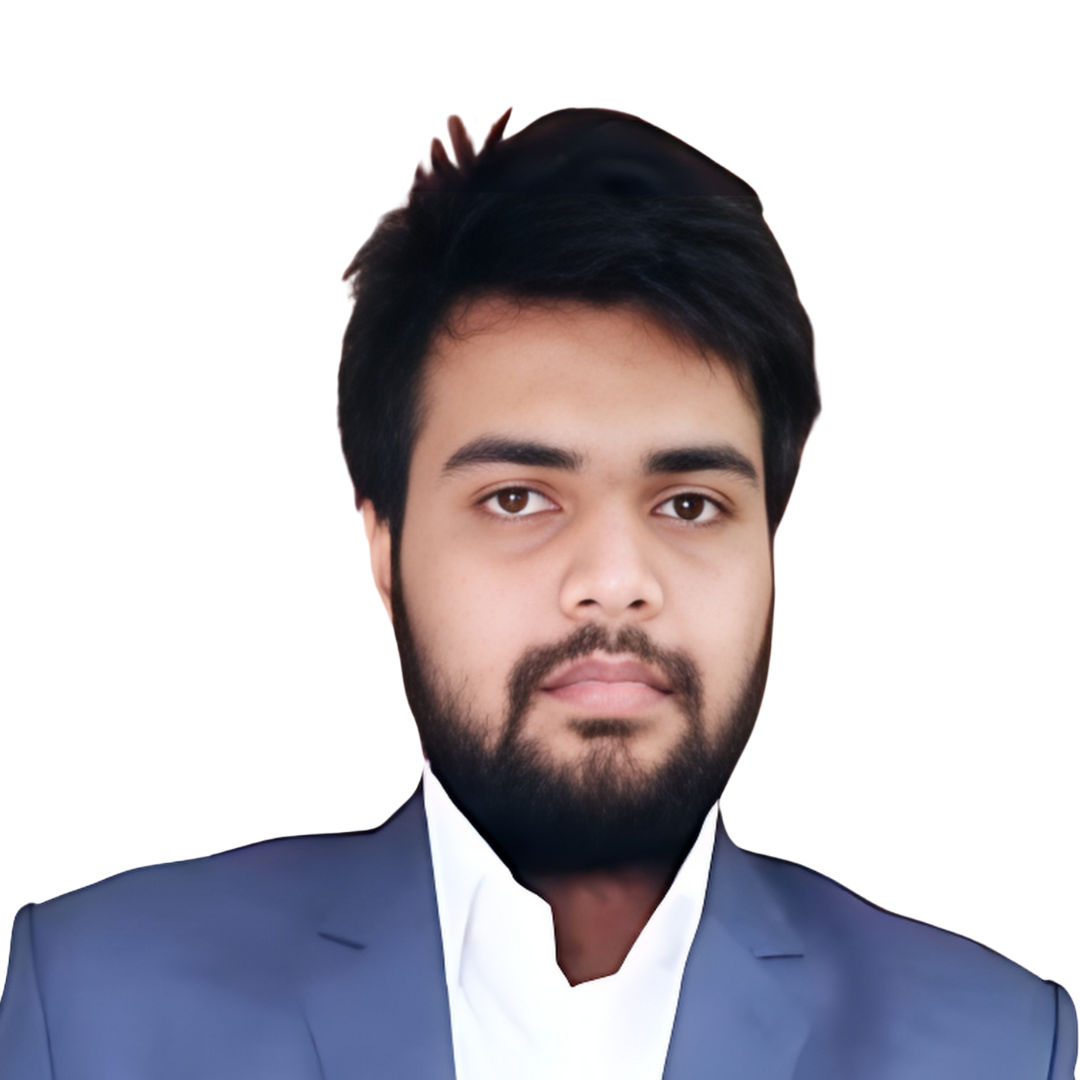
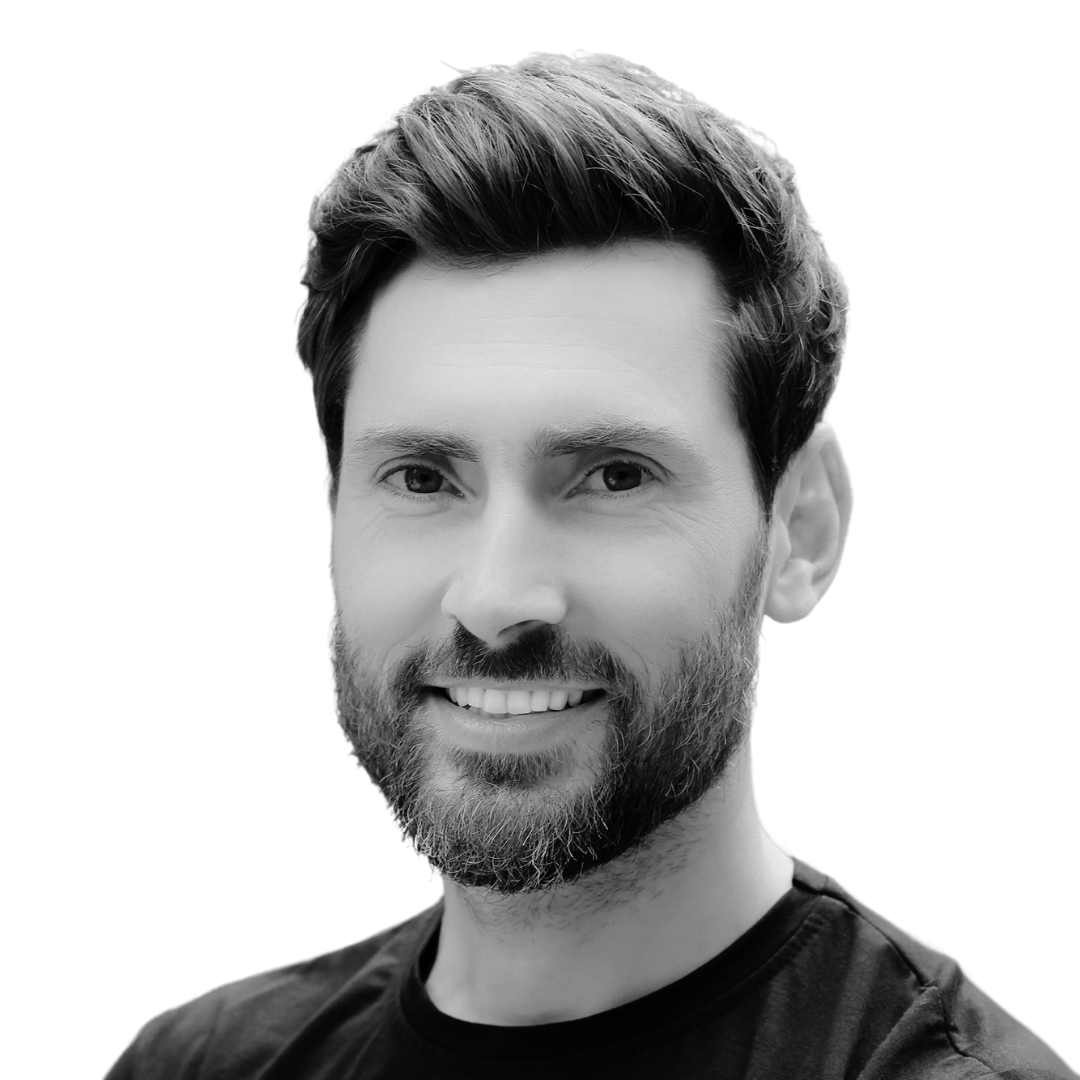
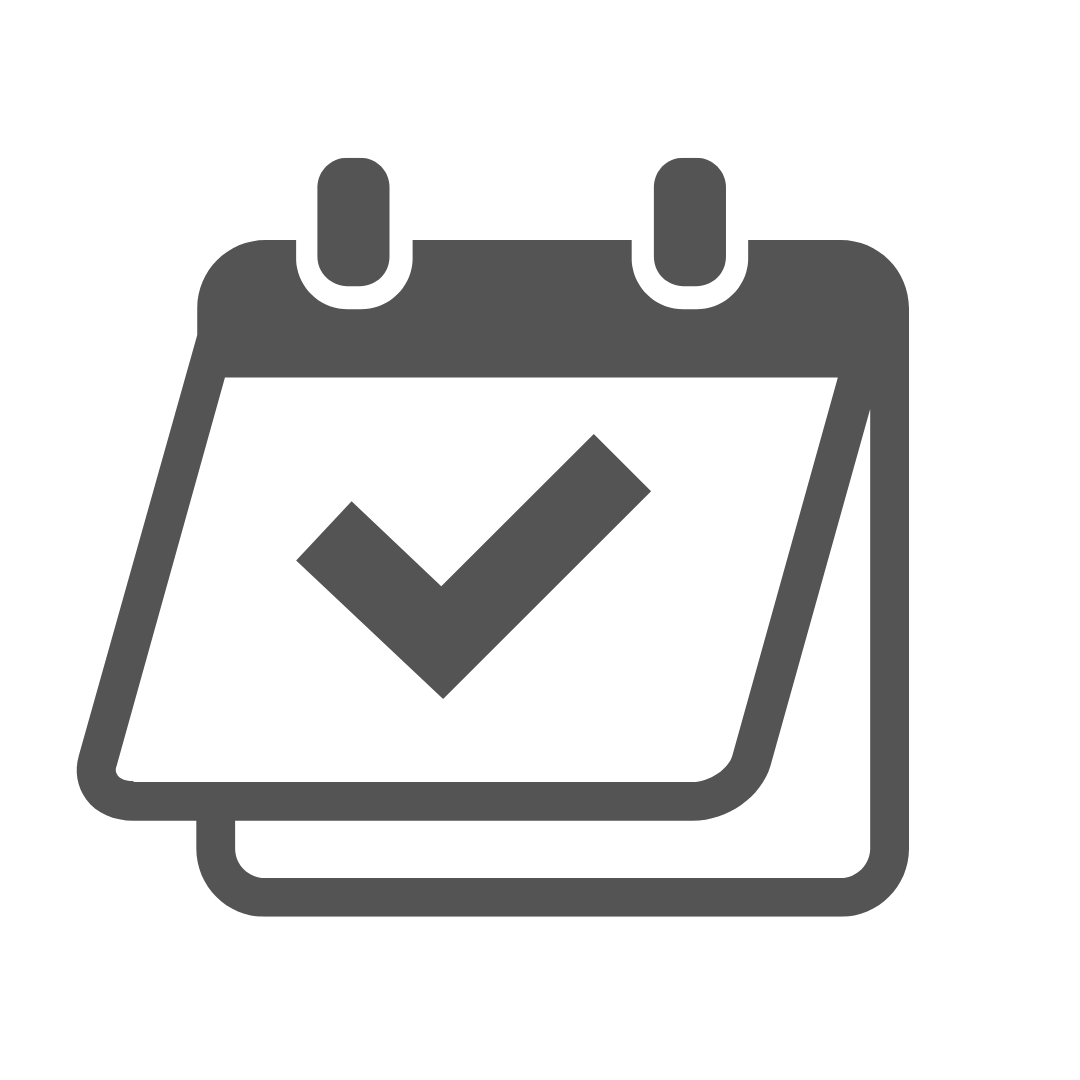
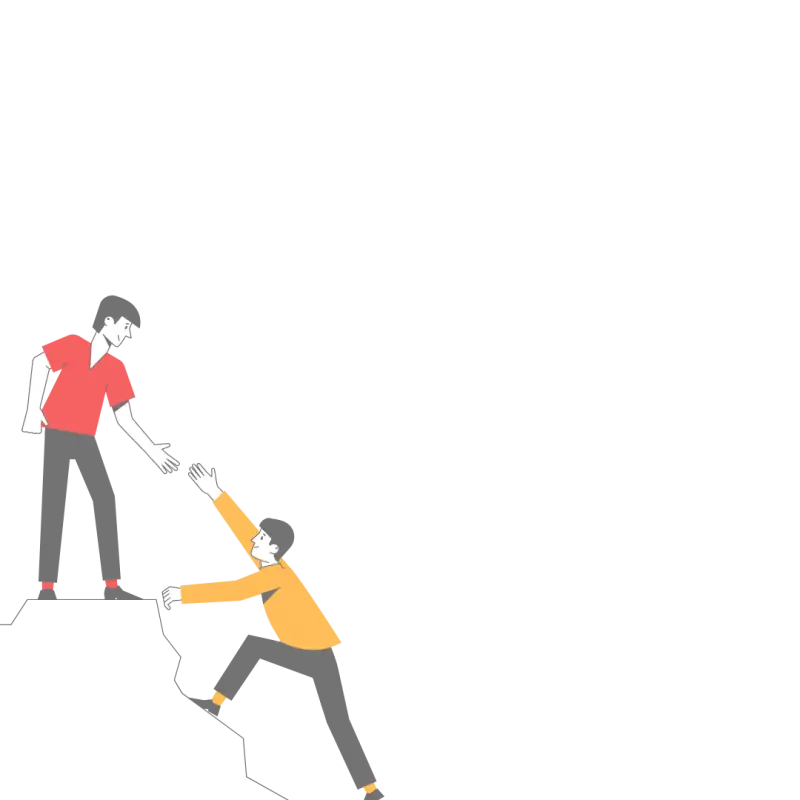
Thanks for your feedback!
Your contributions will help us to improve service.
How can I find an object property in React.js based on its id?
This React.js code defines a functional component named App
that contains an array of employee objects. The component renders a button that, when clicked, invokes myFunction
. This function uses the find
method to search for an employee object with the id
property equal to '4'. If found, it sets the result
state to the JSON string representation of the employee object. If not found, it sets the result
state to 'Employee not found'. The rendered result displays the search result or the 'Employee not found' message upon clicking the button.
Output of React js Find Object property by its id
What is the React JS code to find an element greater than 10 in an array?
This React JS code finds the first element in the array 'numbers' greater than 10 using the 'find' method. It then displays the result in a container with a heading and paragraph. The sample array contains [5, 12, 8, 15, 3, 20]. The output will be '12' since it is the first element greater than 10.
How can I find the element in a Reactjs array that has the value "apple"?
In this React.js code snippet, the function App()
defines an array of fruits and uses the find()
method to search for the first element with the value "apple". If it finds the element, it displays "Found the fruit: apple" on the webpage; otherwise, it shows "Fruit not found". The ReactDOM.render()
method renders the App
component in the HTML element with the ID "app".
How can I find an element in an array property by name using Reactjs?
In this React.js script, an array of user objects is defined with properties "name" and "age." The find
method is used to locate an element in the array where the "name" property matches "John." The found user is then displayed on the webpage, showing their name and age.
Output of React Js Find element in an array property by name
How can I find an element in an array of objects in React.js that has properties "name" and "age"?
In this React.js example, an array called "users" contains objects with properties "name" and "age". The code finds an element in the array where the name is "John" and age is 30 using the find
method. If the element is found, it displays the user's name and age. Otherwise, it shows a message stating "User not found." The UI is created using React components, and the found user's details are rendered in a styled container element.