ReactJs Sort an array of objects by the length of a nested array
ReactJs Sort an array of objects by the length of a nested array:To sort an array of objects in ReactJS by the length of a nested array, you can use the Array.prototype.sort()
method with a custom comparator function. First, extract the nested array's length from each object. Then, compare these lengths in the comparator function and return the appropriate value for sorting
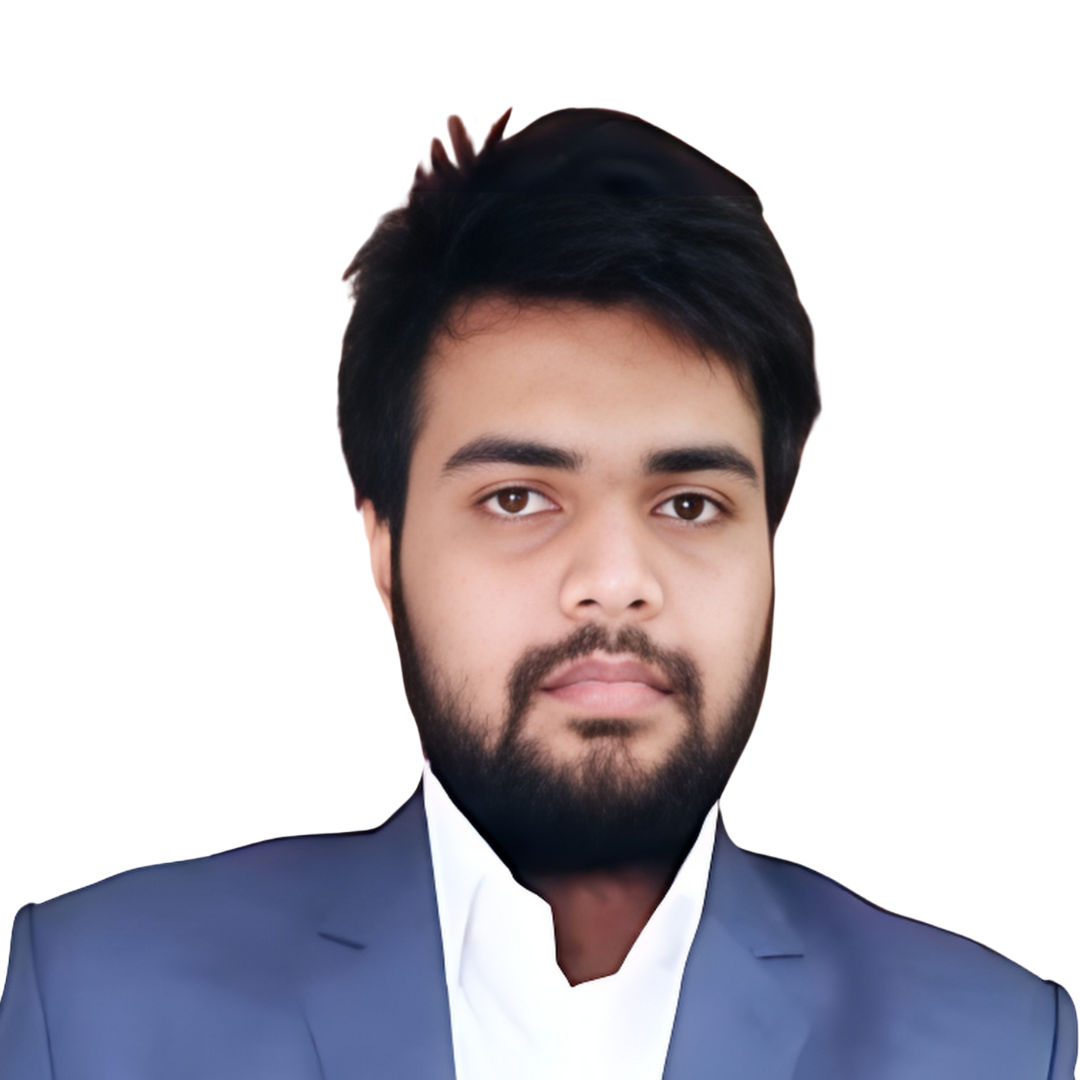
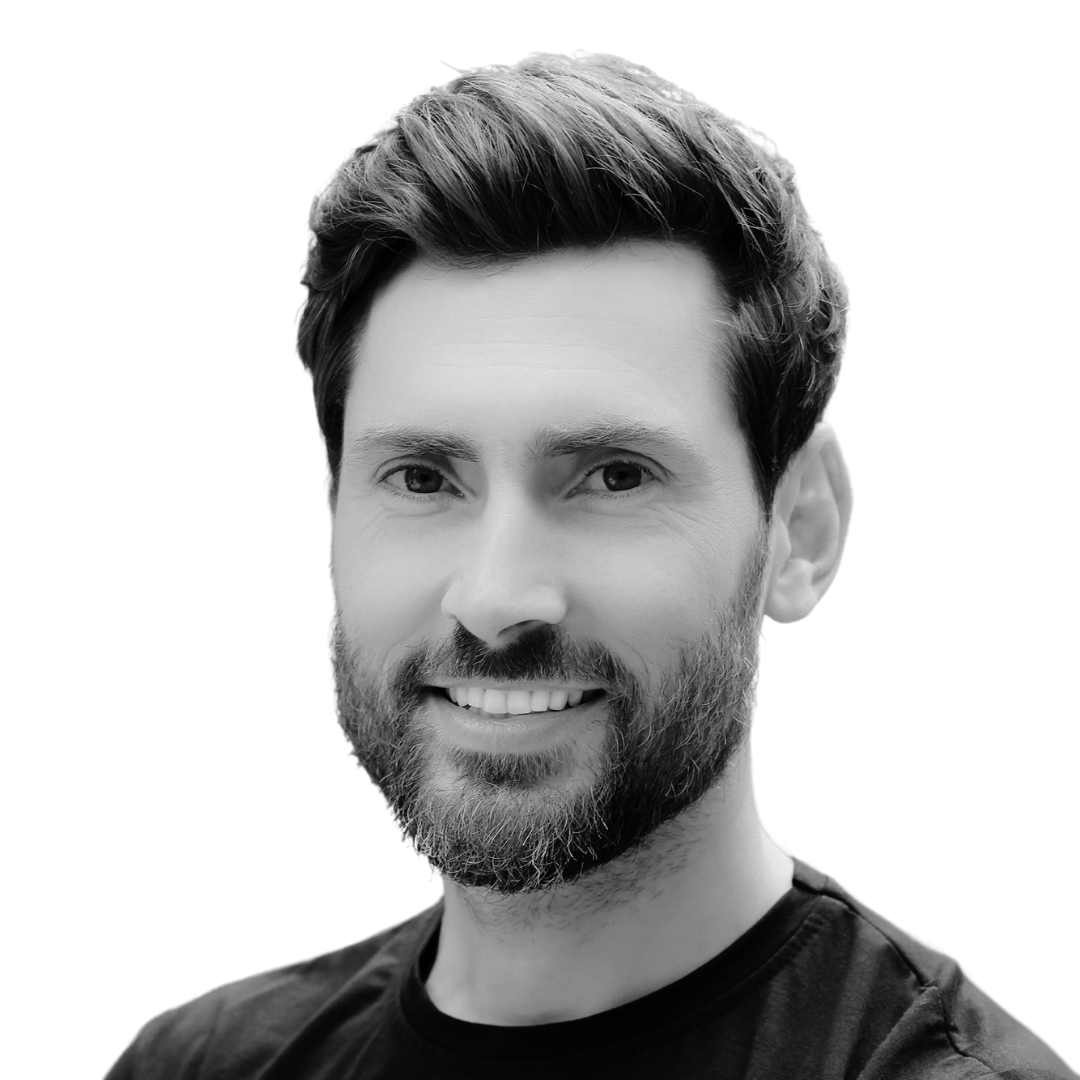
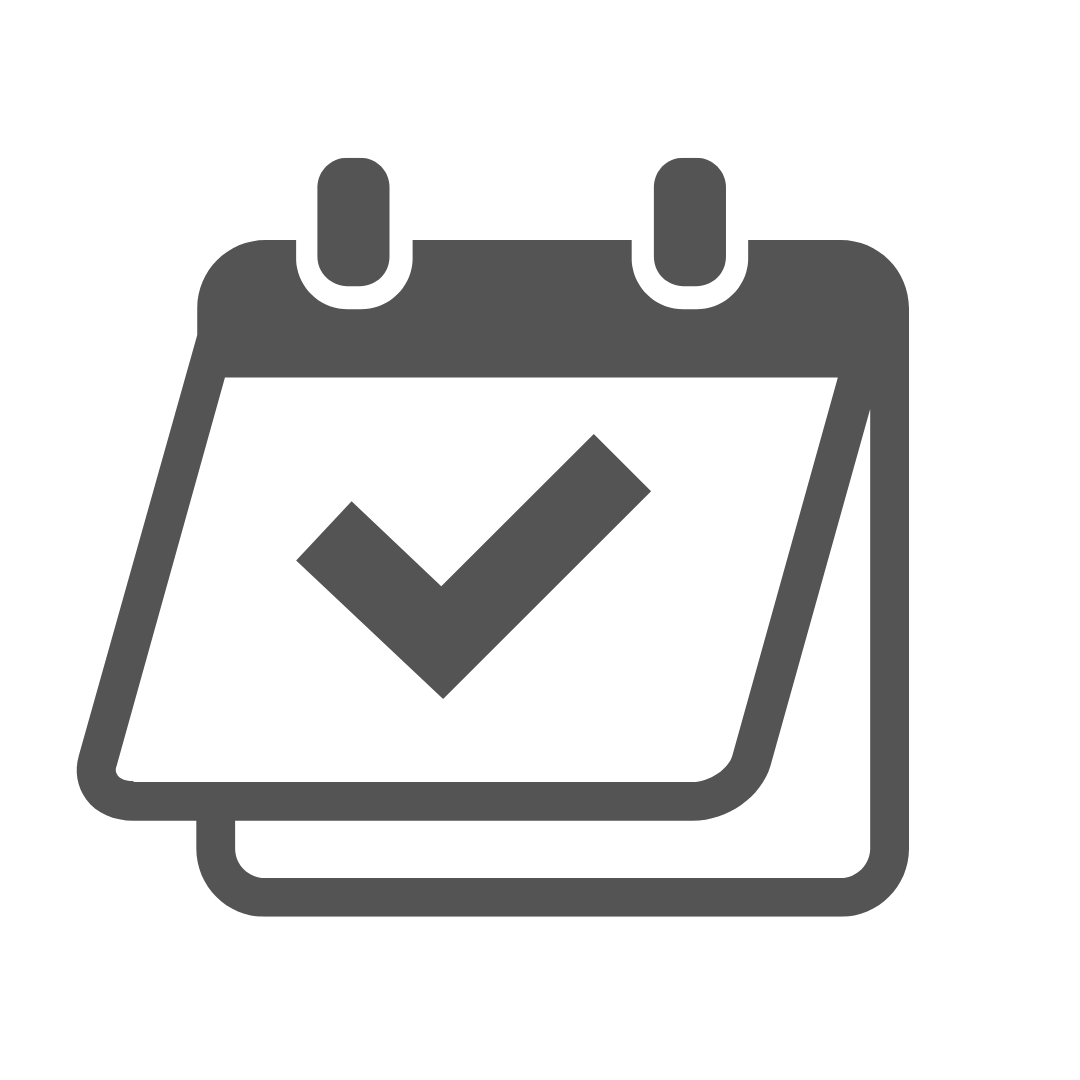
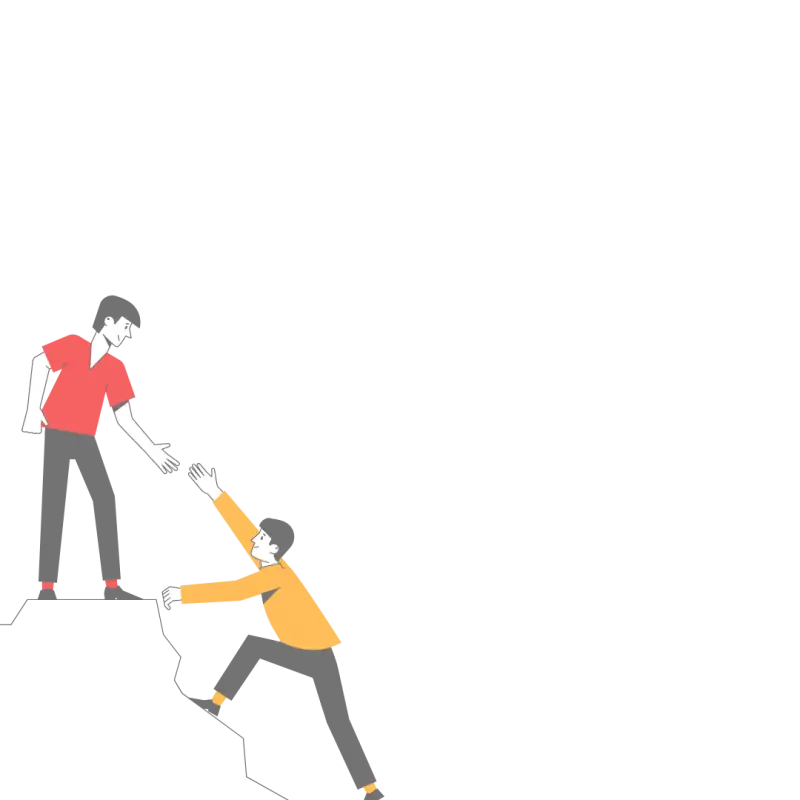
Thanks for your feedback!
Your contributions will help us to improve service.
How to sort an array of objects in ReactJS by the length of a nested array?
This ReactJS code defines a component called "App" that manages an array of objects, each containing an "id" and a nested array called "schools." The code provides a button that, when clicked, sorts the array of objects based on the length of the "schools" array in each object. It uses the useState
hook to manage the state of the data and updates it with the sorted result. The sorted data is then displayed in an unordered list. When the "Sort by School Length" button is clicked, it triggers the sorting function, reordering the objects by the length of their nested "schools" arrays.