React js get first two character of string from json
React js get first two character of string from json:In React.js, to retrieve the first two characters of a string from a JSON object, you need to first access the desired property from the JSON data using dot notation or bracket notation. Once you have the string value, you can then use the substring method, passing in the start index as 0 and the end index as 2, to extract the first two characters of the string. This can be achieved in a concise manner, typically requiring a few lines of code to access the property and apply the substring method.
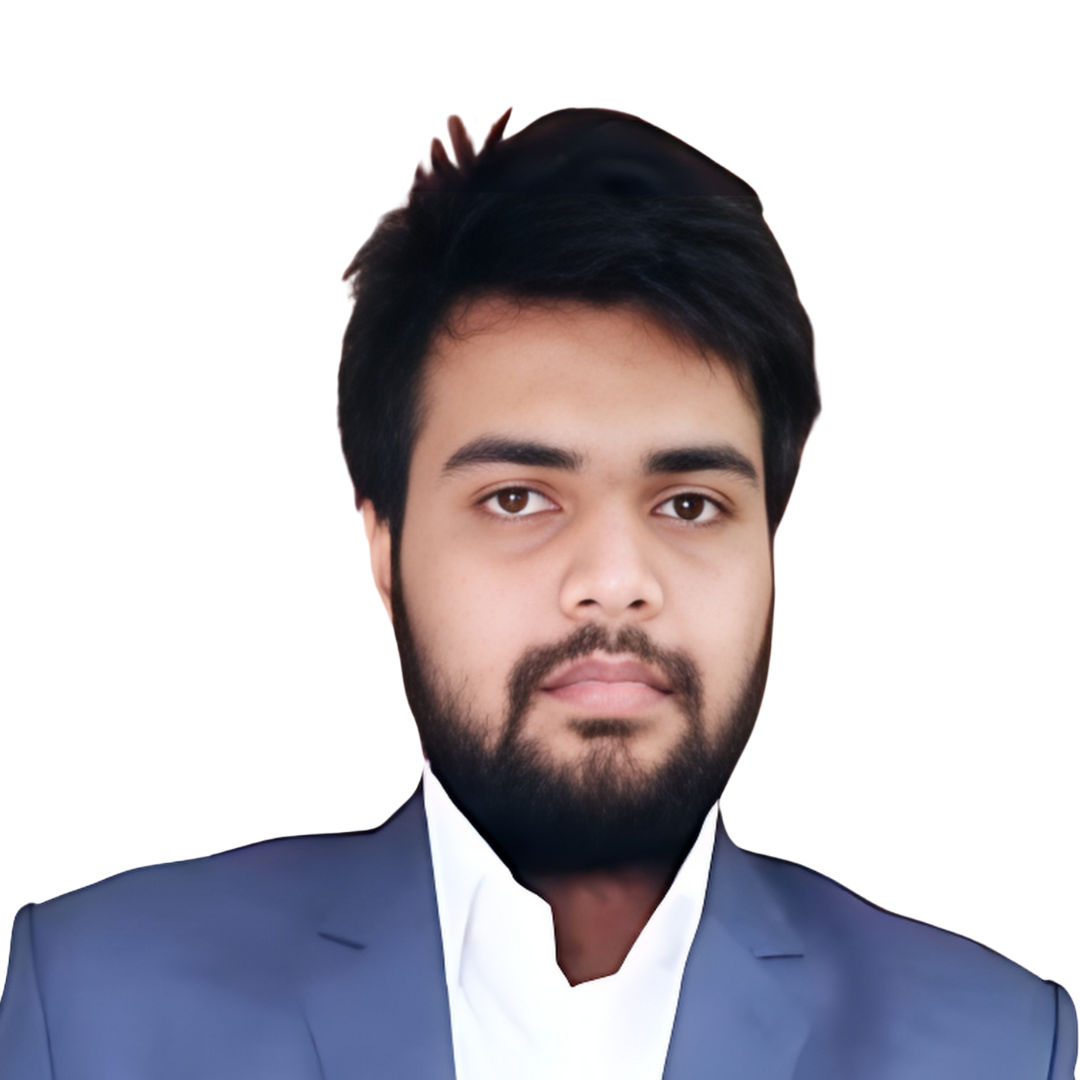
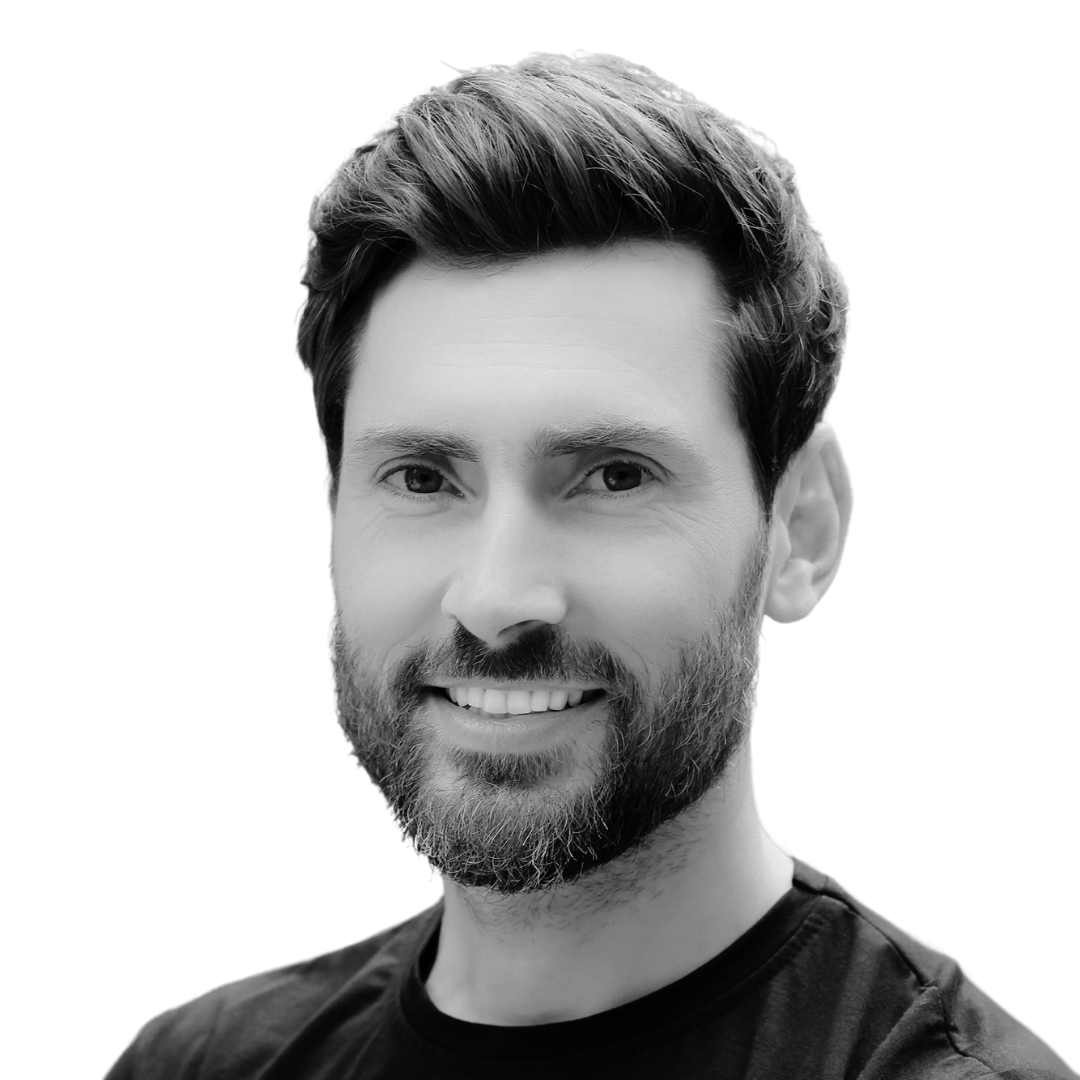
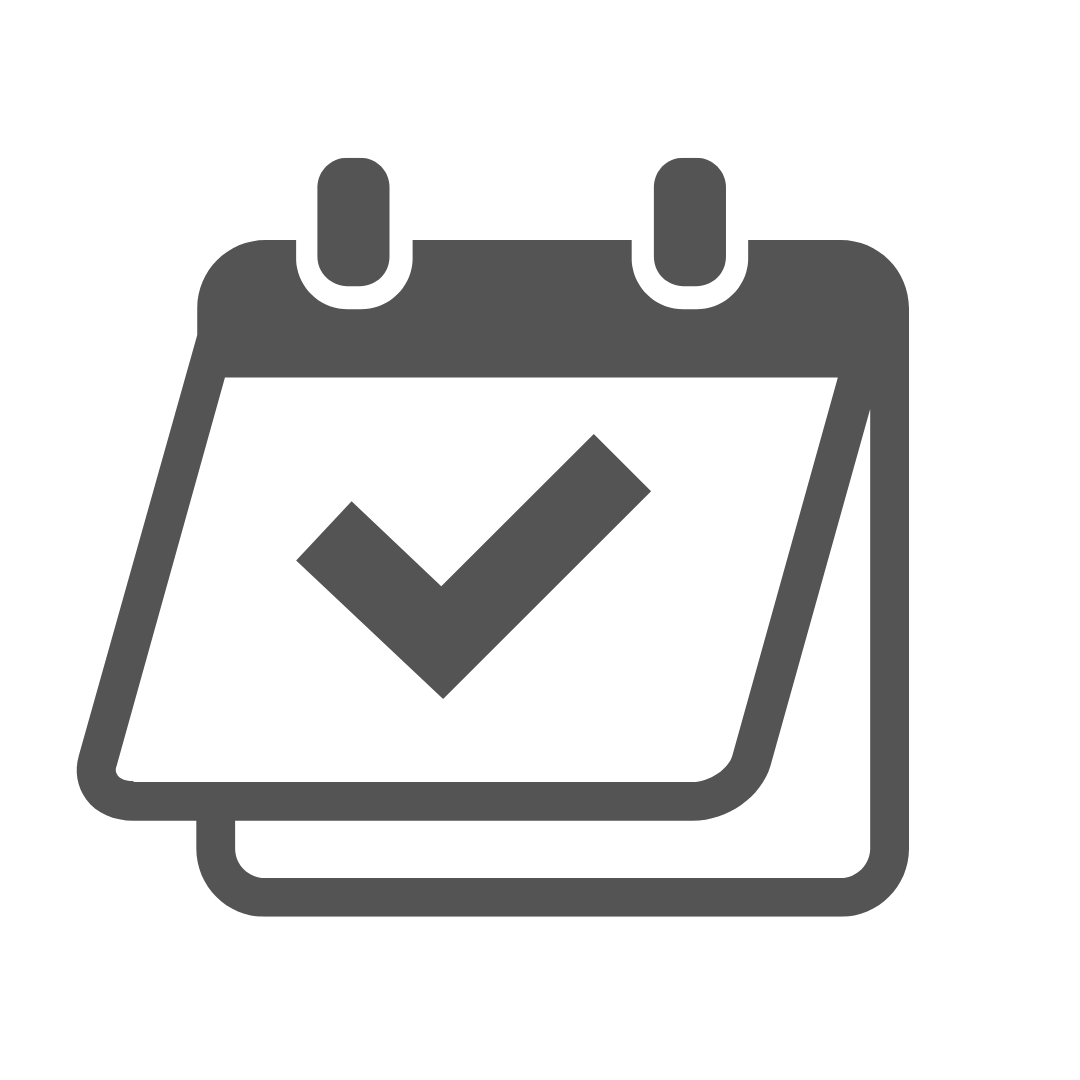
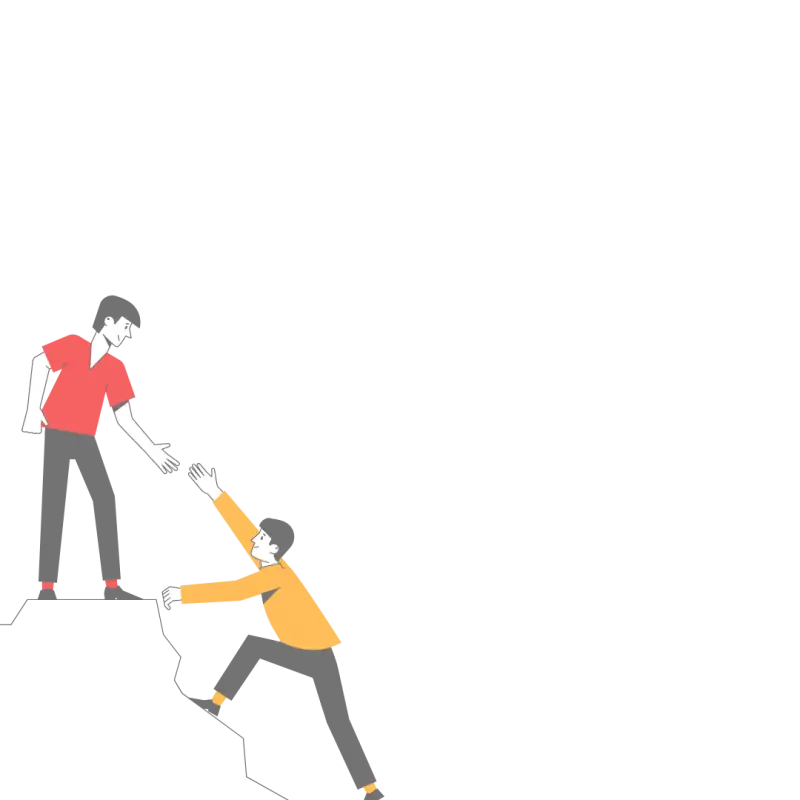
Thanks for your feedback!
Your contributions will help us to improve service.
How can you extract the first two characters of a string from a JSON using Reactjs?
This code snippet uses React.js to extract the first two characters of each month name from a JSON object. The JSON object contains an array called "months" with the names of the twelve months. The code uses the map
function to iterate over each month and uses the substring
method to extract the first two characters. The resulting month abbreviations are then rendered in a list using JSX in a React component.