React Js Convert Decimal / Float Number to Whole / Integer

React Js Convert Float to Integer Number: In React.js, converting a decimal number to an integer can be done using JavaScript's Math object methods.
- Math.floor() rounds down to the nearest integer.
- Math.ceil() rounds up.
- Math.round() rounds to the nearest integer.
- Math.trunc() simply removes the decimal part.
For example, Math.floor(4.7) would result in 4, Math.ceil(4.2) would yield 5, Math.round(4.5) becomes 5, and Math.trunc(4.9) gives 4.
These methods offer flexibility in handling decimal numbers when developing React.js applications, depending on whether you need to round up, down, or simply truncate the decimal portion to obtain an integer value
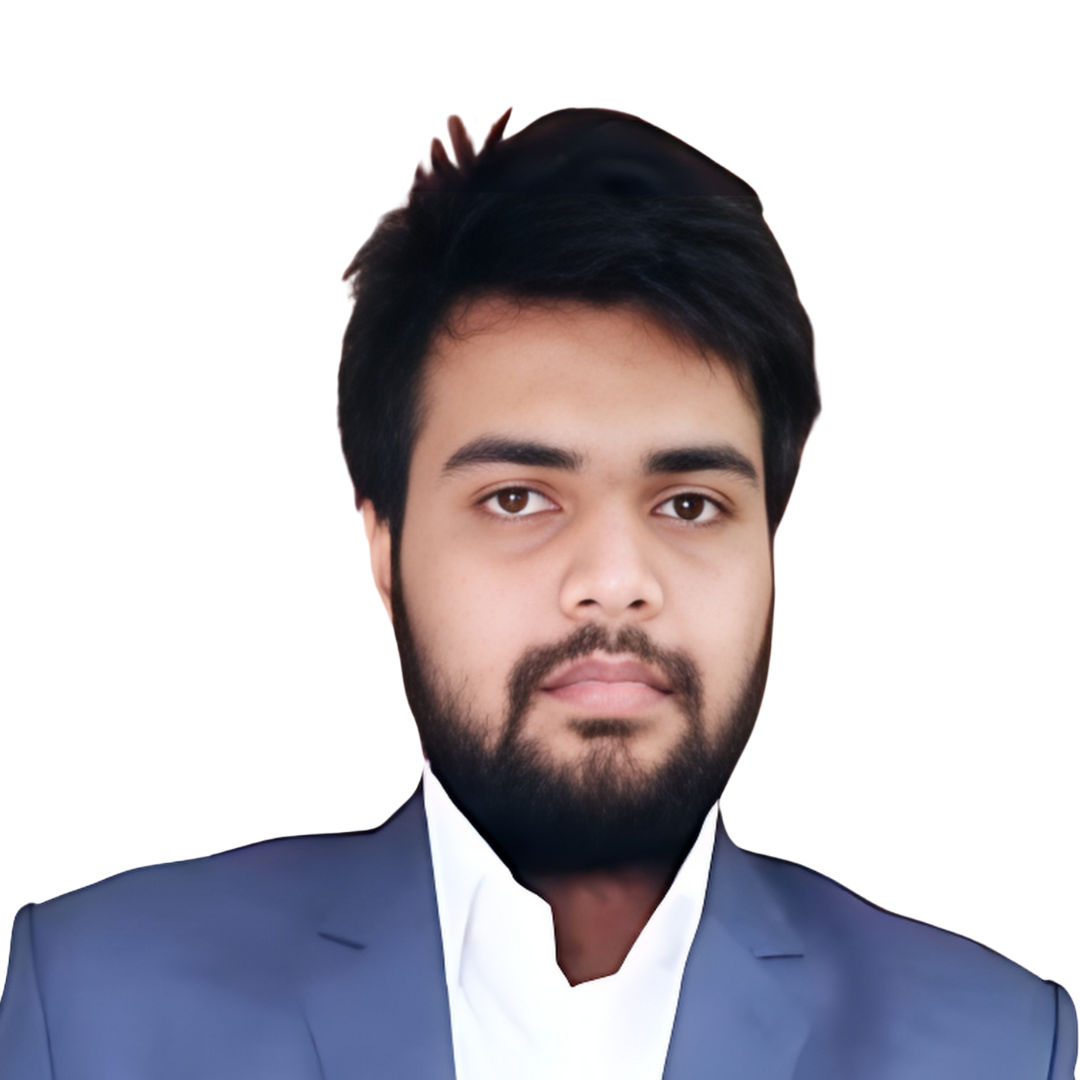
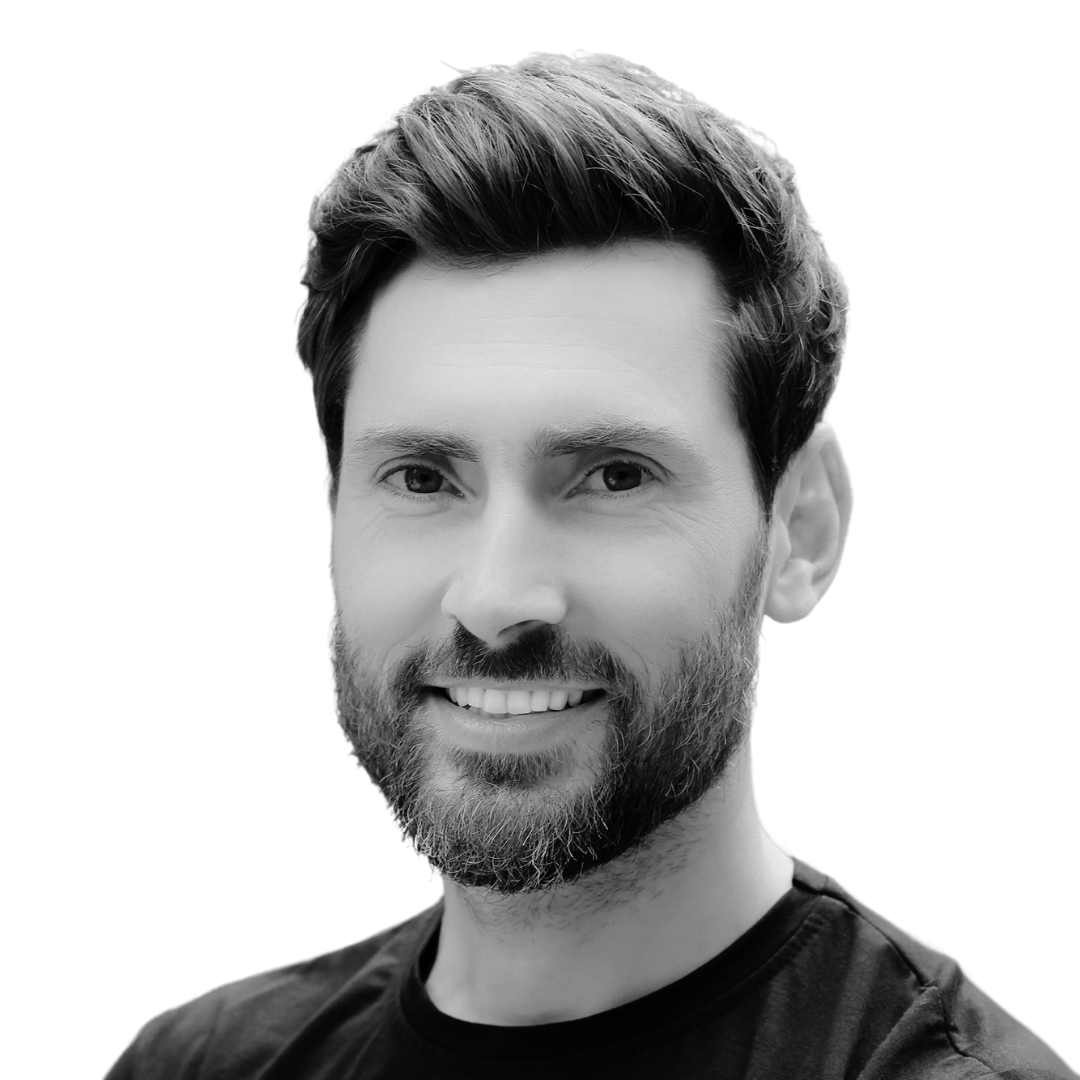
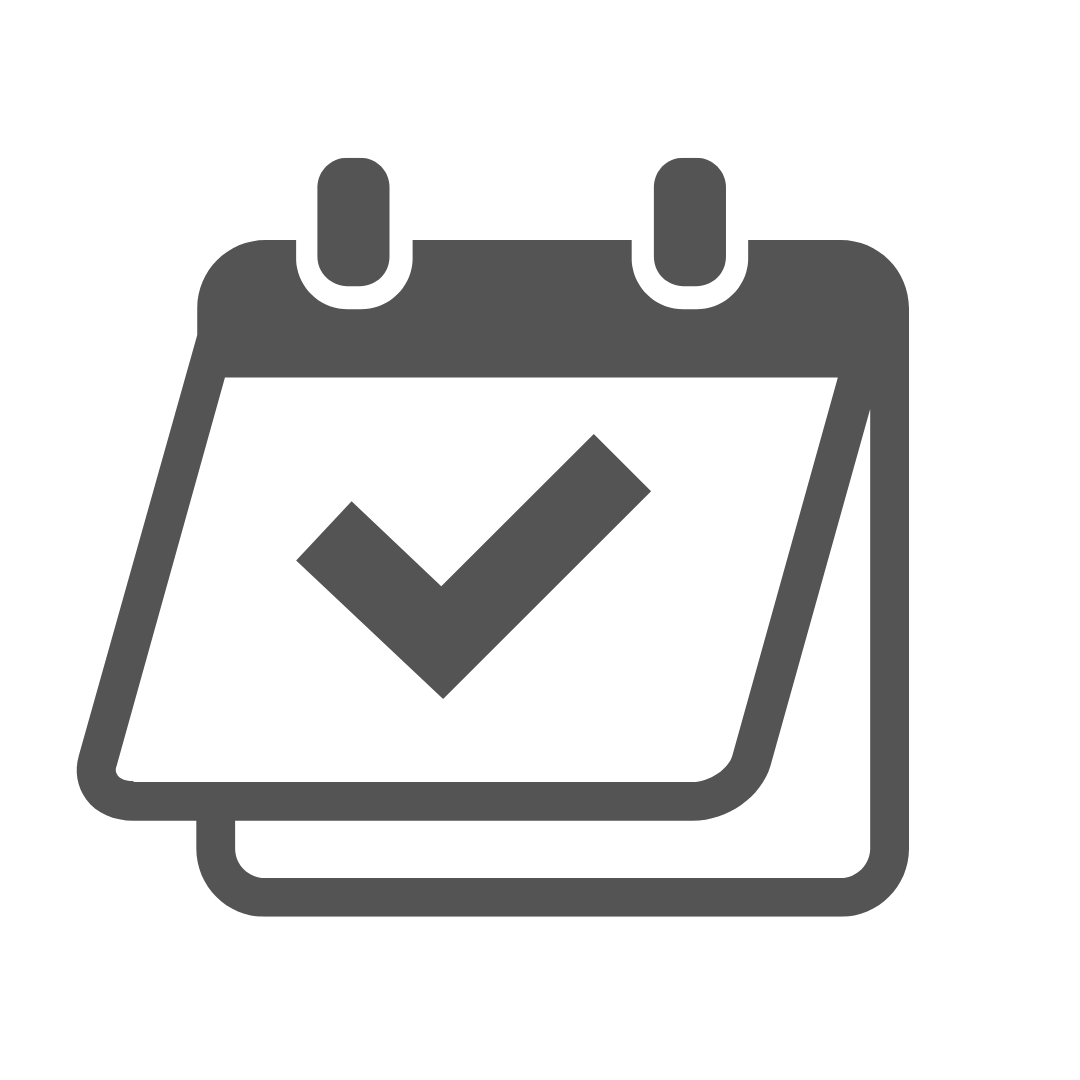
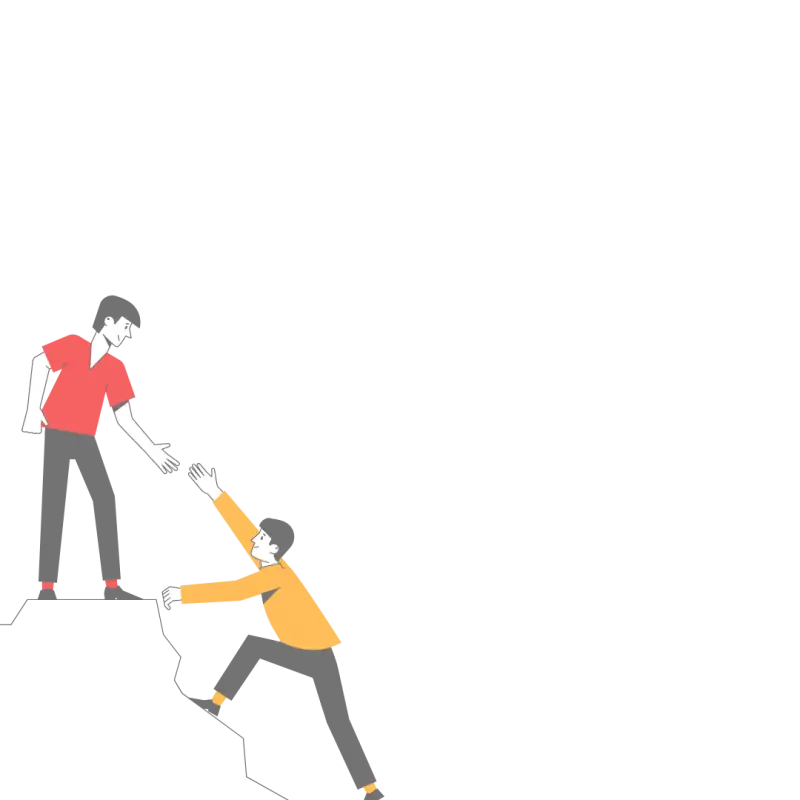
Thanks for your feedback!
Your contributions will help us to improve service.
How do you use Math.floor()
to convert a float to an integer in Reactjs?
In this React.js code snippet, a floating-point value (5.75) is converted to an integer using the Math.floor()
function. The resulting integer value (5) is displayed in a React component, demonstrating how to convert a float to a whole number in a React application.
Output of React Js Convert Float to Integer Number | Math.floor()
How do you convert a float to a whole number using Math.ceil()
in Reactjs?
This React.js code snippet demonstrates how to convert a floating-point number (5.15 in this case) to a whole number using the Math.ceil()
function. It rounds up the float to the nearest integer, resulting in an integer value of 6. The values are displayed in a React component.
Output of React Js Convert Float to Whole Number | Math.ceil()
Explain how to use Math.round()
in React.js to convert a float to an integer.
This React.js code snippet uses the Math.round()
function to convert a floating-point number (5.1 in this example) into an integer. It renders this conversion within a React component. The floatValue
is rounded using Math.round()
, resulting in integerValue
. Both values are displayed in a container using JSX. When rendered, it will show "Floating-Point Value: 5.1" and "Integer Value: 5" on the webpage, demonstrating how to convert a float to an integer in React.js using Math.round()
.
Output of React Js Convert Float to Integer Number | Math.round()
How can you use the Math.trunc() method in React.js to convert a decimal number to an integer number?
In this React.js code snippet, a floating-point value (5.9) is converted to an integer using the Math.trunc()
function. The result, the integer value (5), is displayed in a React component alongside the original floating-point value for demonstration.