React Js Delete Image From Server Using CodeIgniter
React Js Delete Image From Server Using CodeIgniter | Database:To delete an image from the server using React.js on the frontend and CodeIgniter on the backend, you'll need to implement an API on the CodeIgniter server to handle the deletion of the image and then make an HTTP request from the React.js frontend to call that API. Here's a step-by-step guide on how to do it:
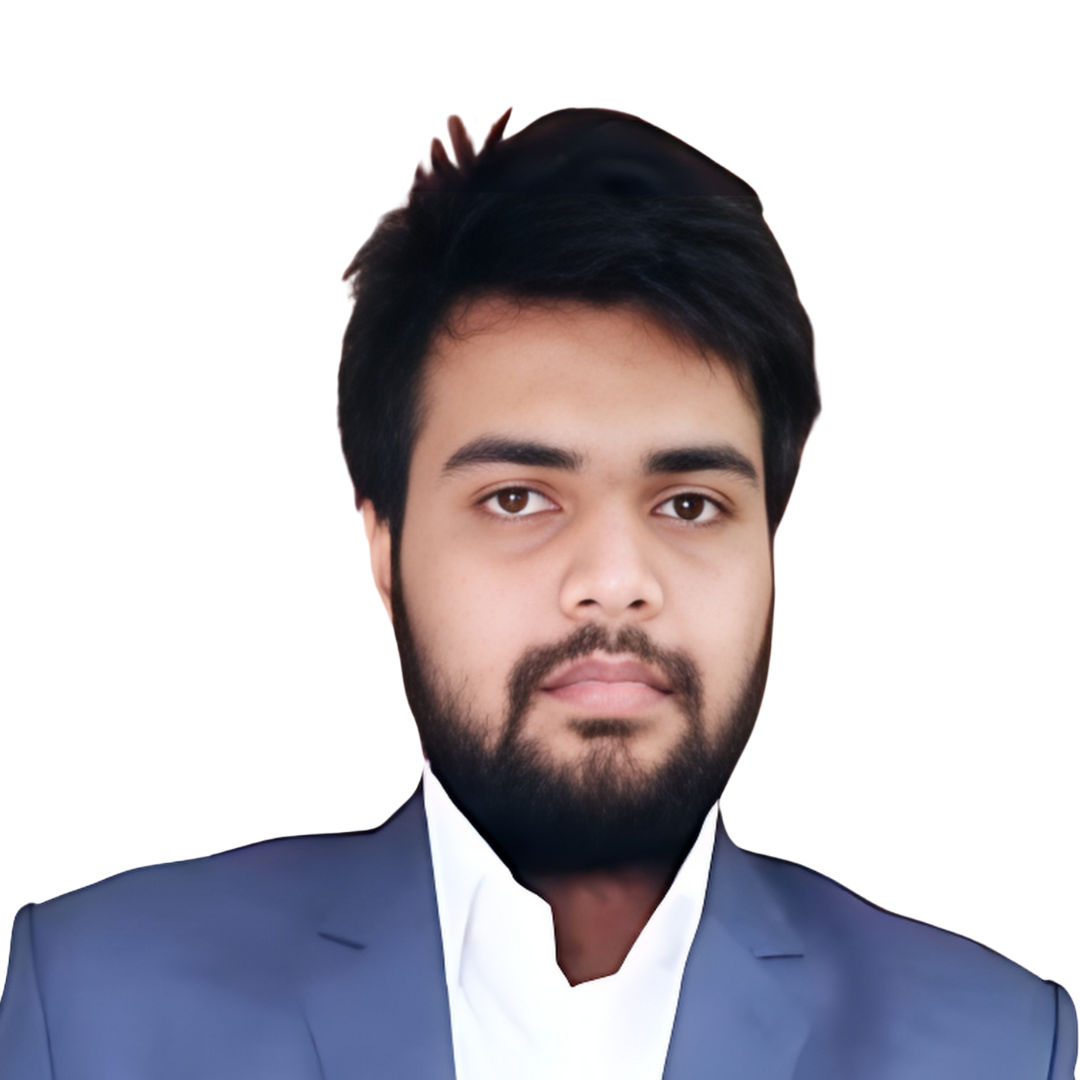
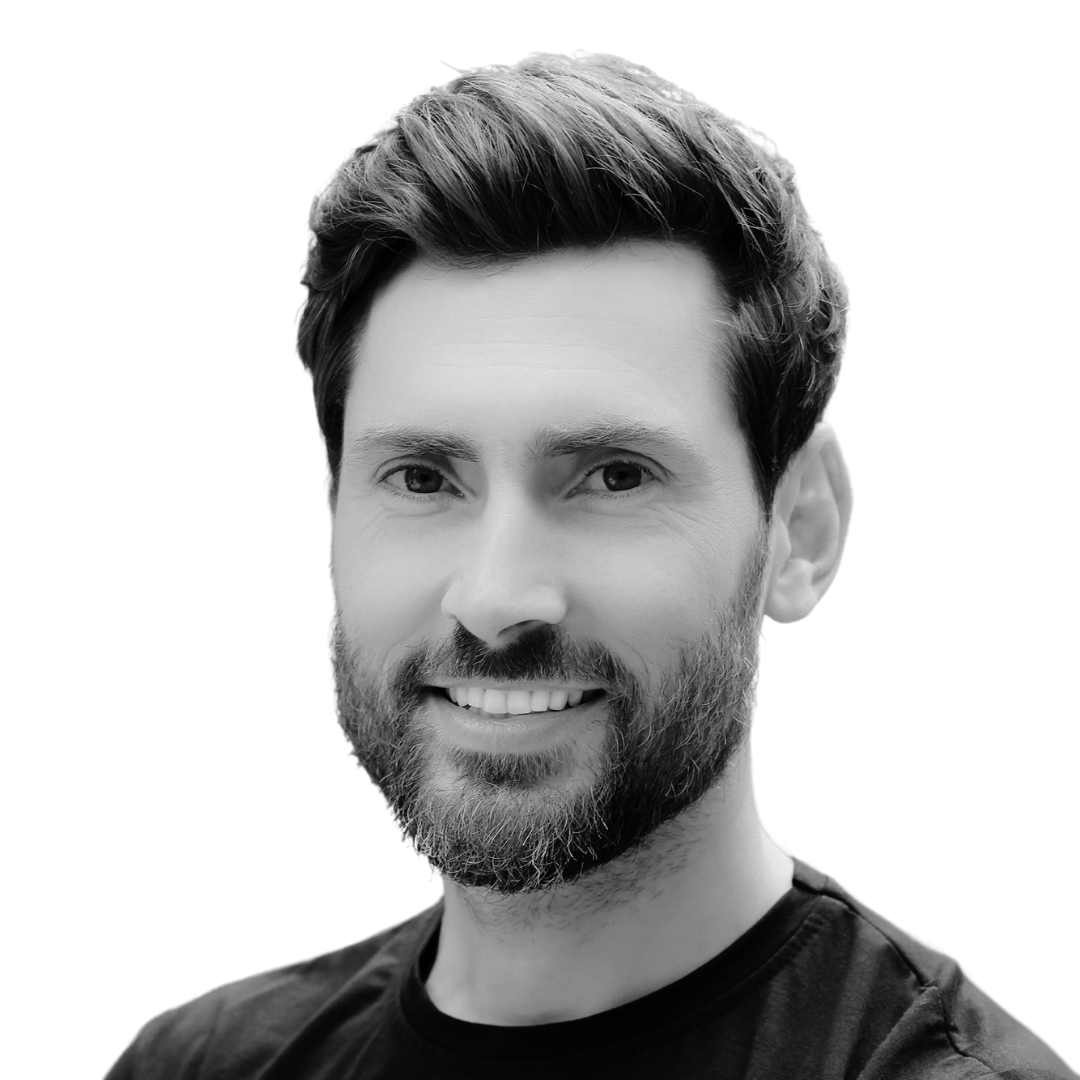
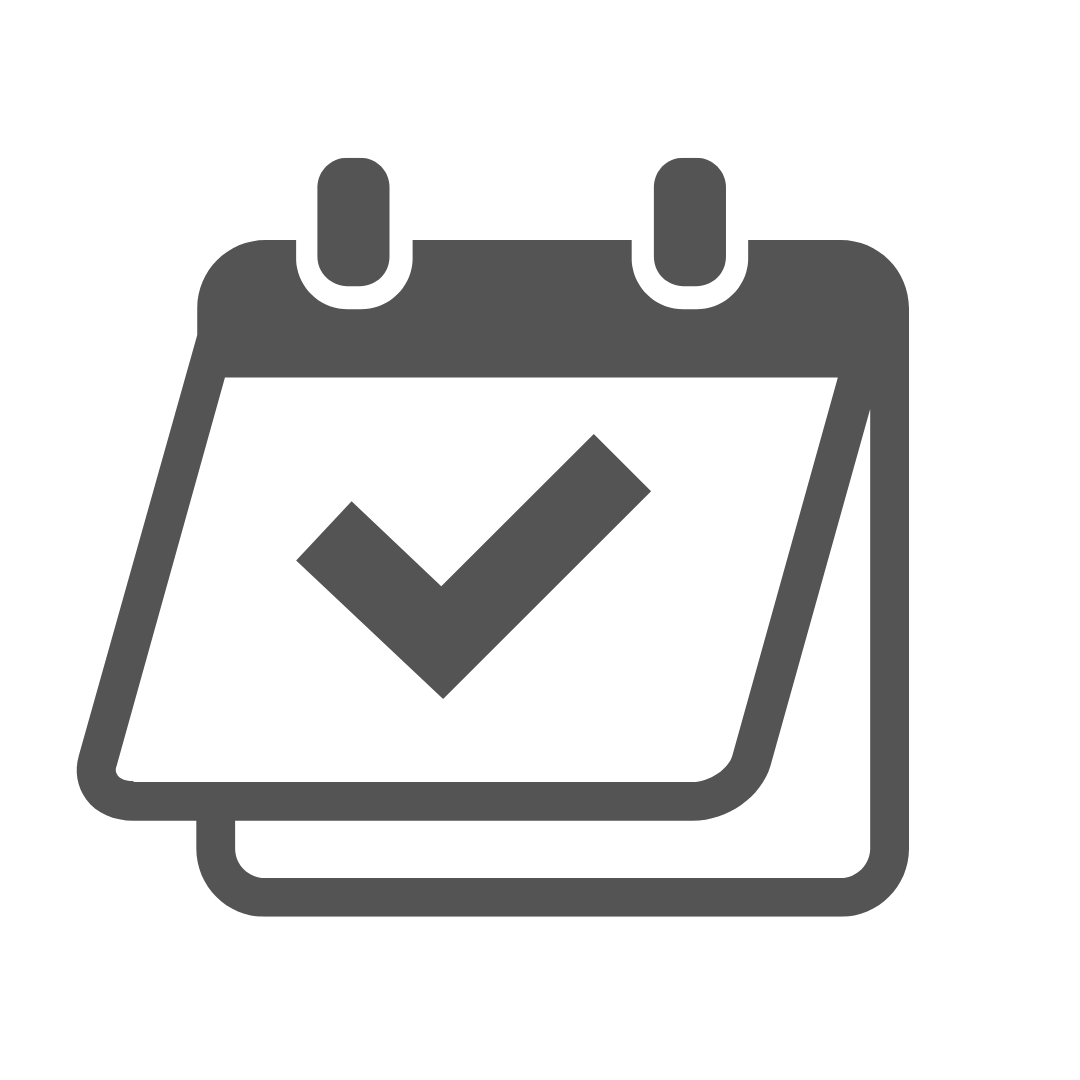
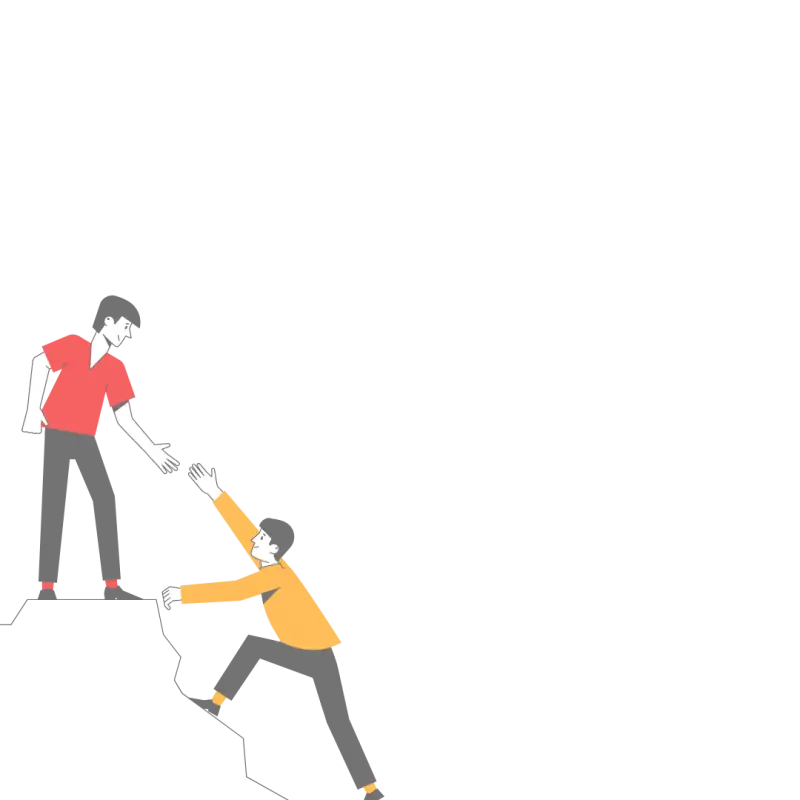
Thanks for your feedback!
Your contributions will help us to improve service.
- Create the CodeIgniter Model:
Create a new model in your CodeIgniter application to handle the database operations related to image deletion. Let's call it Image_model.php
.
Image_model.php
Replace 'path/to/your/images/folder/'
with the actual path to the folder where your images are stored. Replace 'images_table'
with the name of your database table that stores image records.
- Update the CodeIgniter Controller:
Modify the ImageController
to use the Image_model
for image deletion
controllers/ImageController.php
- 3.Set up the React.js frontend:
Assuming you have React.js set up and a component to handle the deletion of the image. In this example, I'll create a simple functional component.
- Route the API request in CodeIgniter:
In CodeIgniter, make sure to configure your routes to map the API request to the appropriate controller method. Add the following line to the routes.php
file in your CodeIgniter project:
Make sure you have properly configured your CodeIgniter project and set up the base URL for React.js to communicate with the server.
Now, when the user clicks the "Delete Image" button in the React.js component, it will make an API call to the CodeIgniter server, which will then delete the image from the server if it exists. The server will send back a response, which will be displayed as a message on the React.js frontend.