React Js Draw a Horizontal Line with Text in the Middle

To draw a horizontal line with text in the middle using React.js, you can create a component that combines HTML elements and CSS. Within the component's render method, include a <div>
with a CSS class to style the line, and a <span>
for the text. Apply CSS to center the text both horizontally and vertically within the <div>
. Set the text content dynamically through a state or prop. You can adjust the line's length and text based on your requirements. This approach combines React's component structure with CSS for a responsive horizontal line with text centered in the middle.
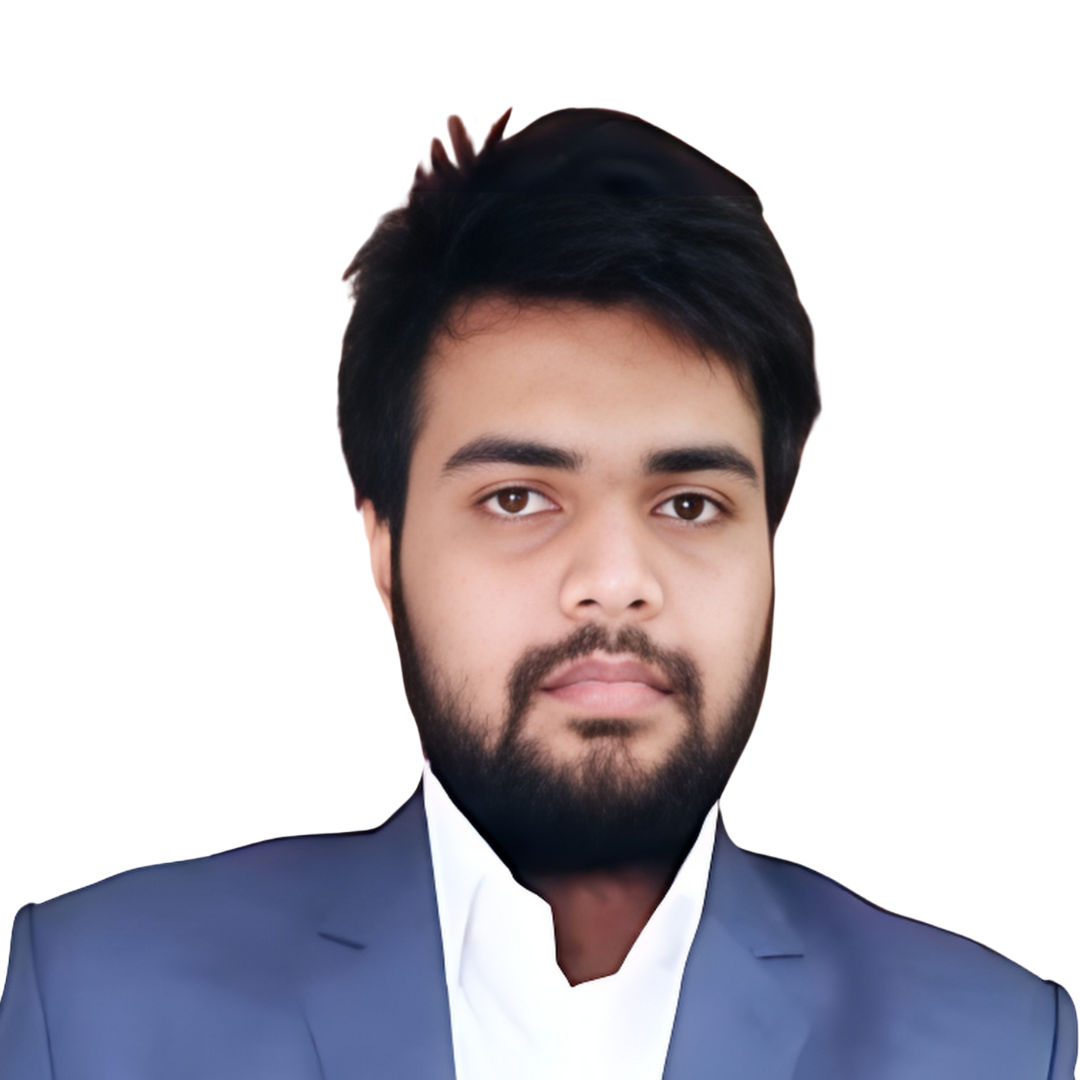
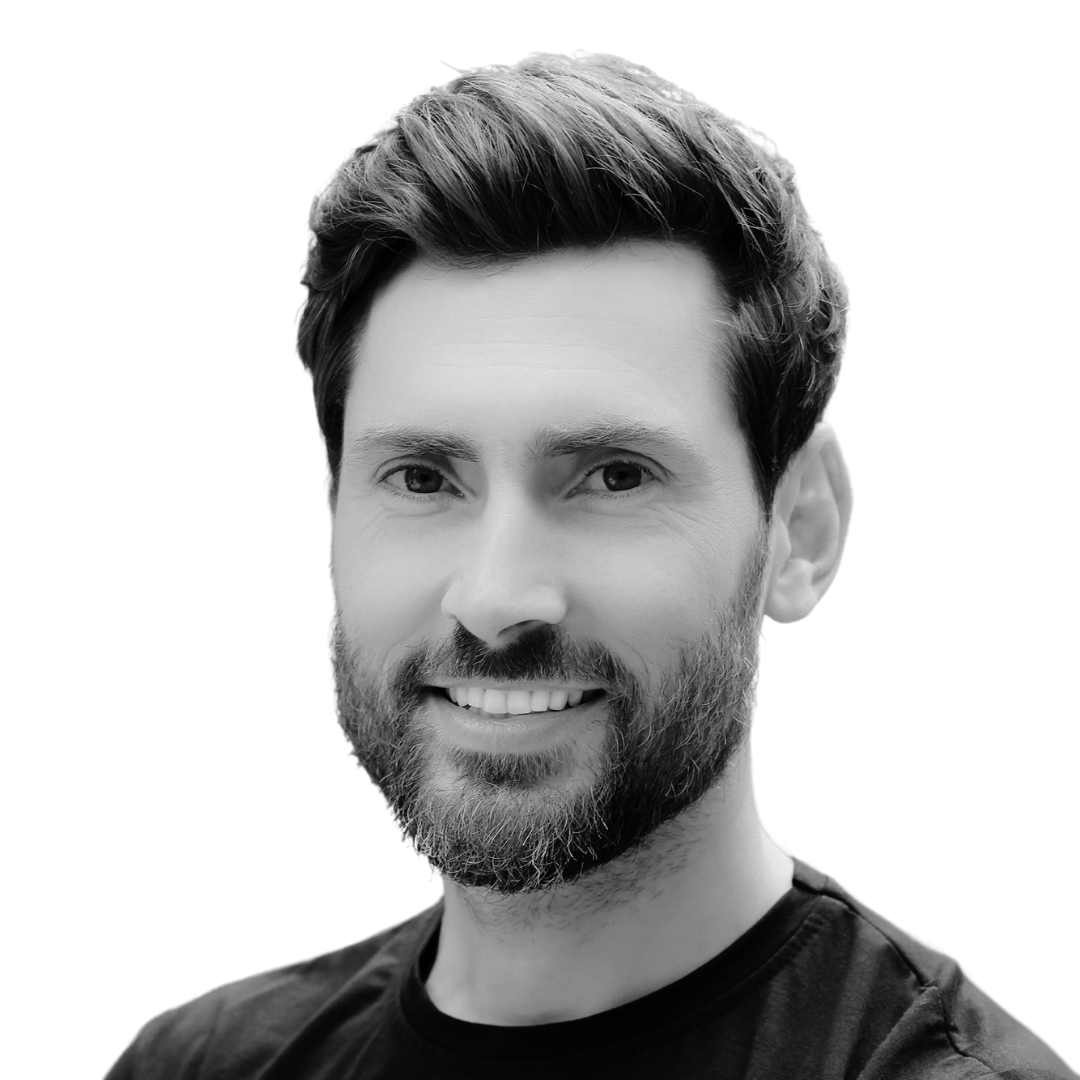
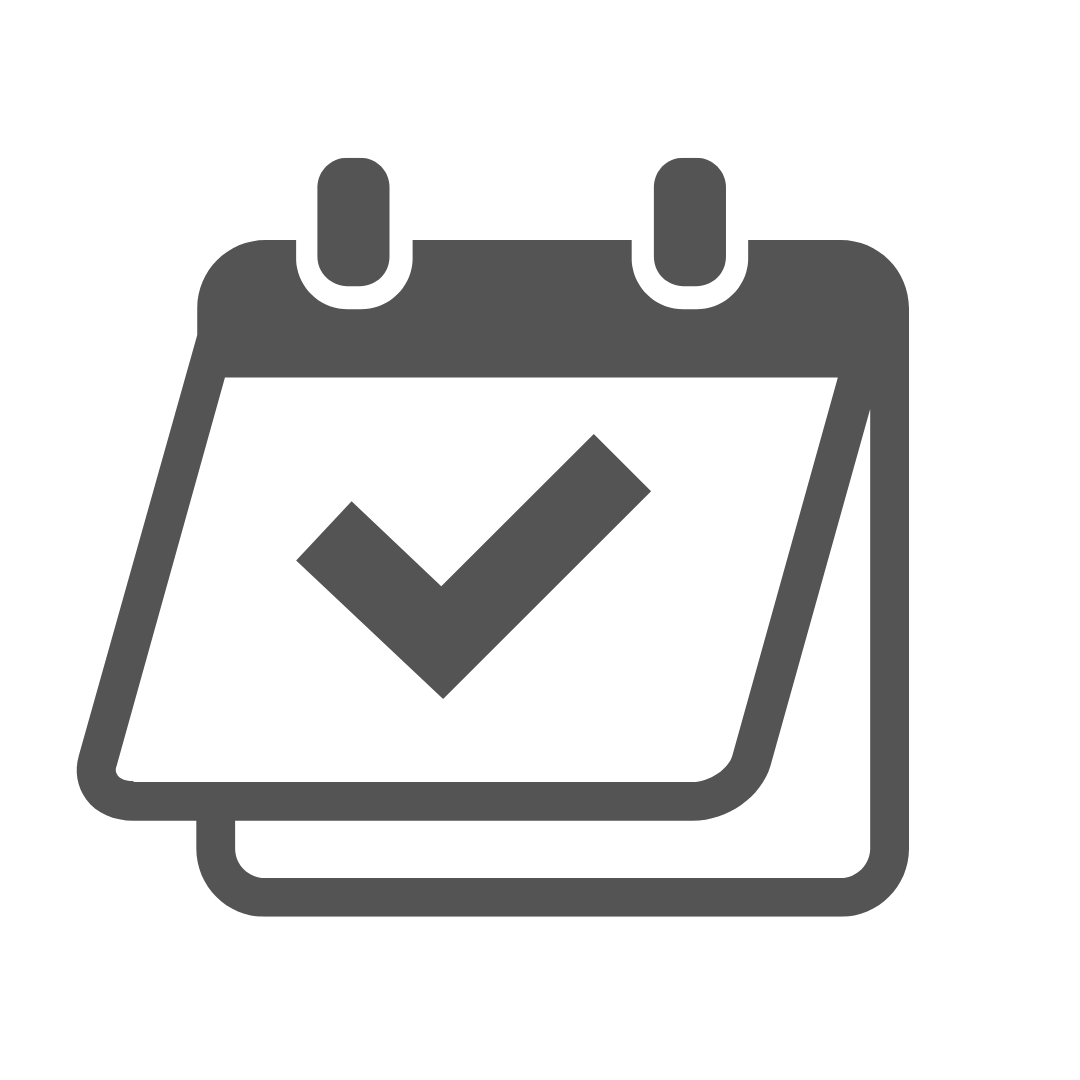
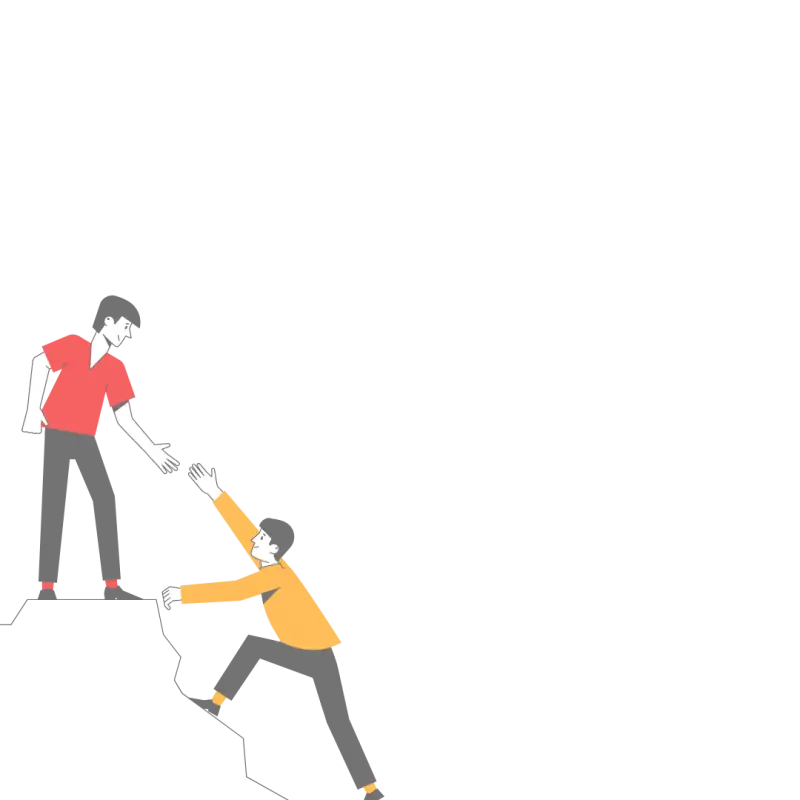
Thanks for your feedback!
Your contributions will help us to improve service.
How can you create a horizontal line with text in the middle using Reactjs?
The given React.js example demonstrates drawing a horizontal line with text in the middle. Inside the App function, a div with a class name 'container' contains a heading and a flex container. The flex container aligns its items (two divs and a paragraph) vertically in the middle. The two divs on the sides create horizontal lines with a height of 3 pixels and a green color (#004D40). The paragraph in the center, with margin applied, displays the text "Fontawesomeicons.com." This results in a visually centered horizontal line with text in a React js.