React Js Array filter Method
React Js Array filter Method:The filter()
method in React.js is used to create a new array containing elements from an existing array that satisfy a specific condition.
It takes a callback function as an argument, which is invoked for each element in the array. The callback function should return true
or false
based on whether the element meets the condition.
The filter()
method then creates a new array with only the elements that returned true
. This is particularly useful for manipulating and rendering arrays of data in React.js applications, allowing for efficient filtering and rendering of specific elements based on certain criteria.
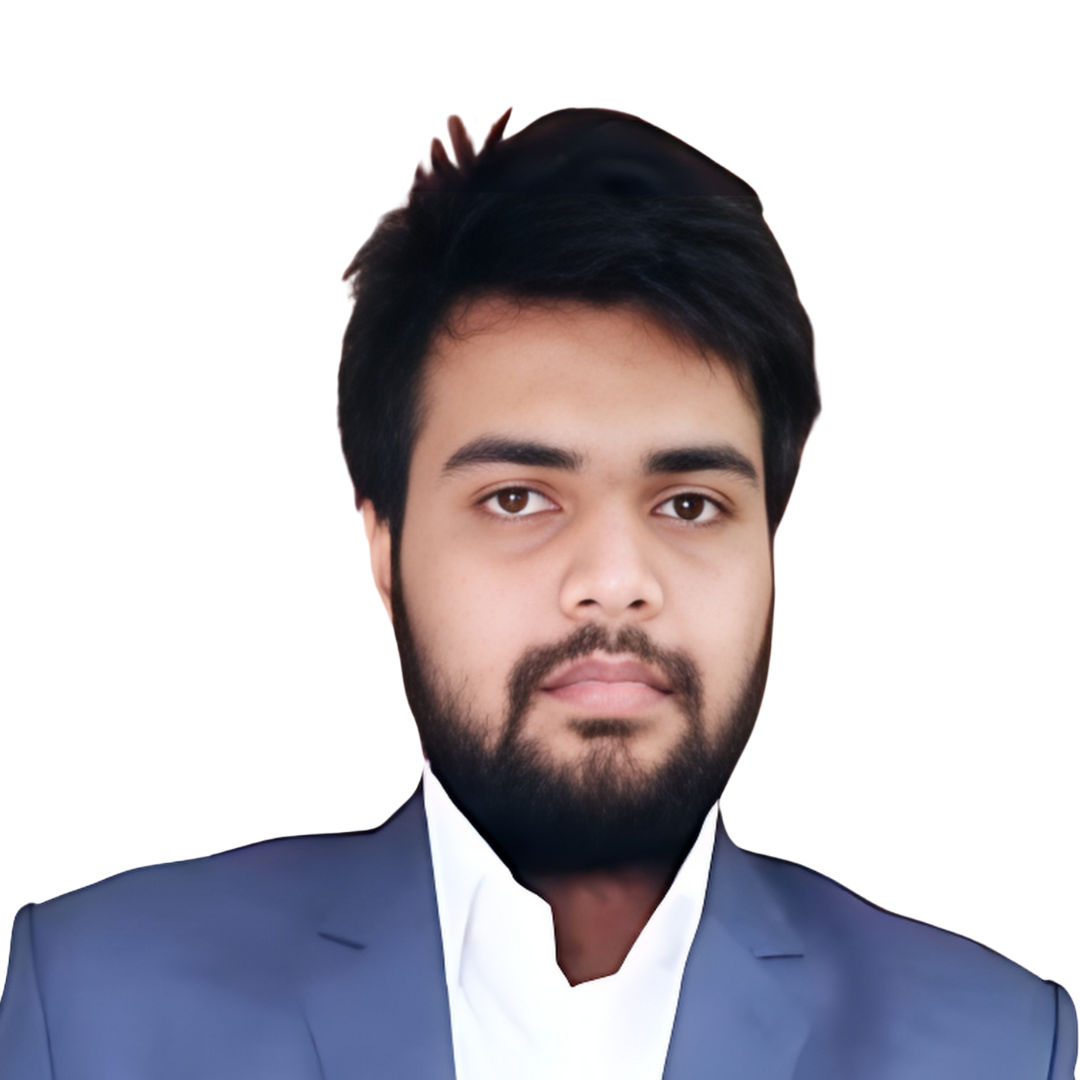
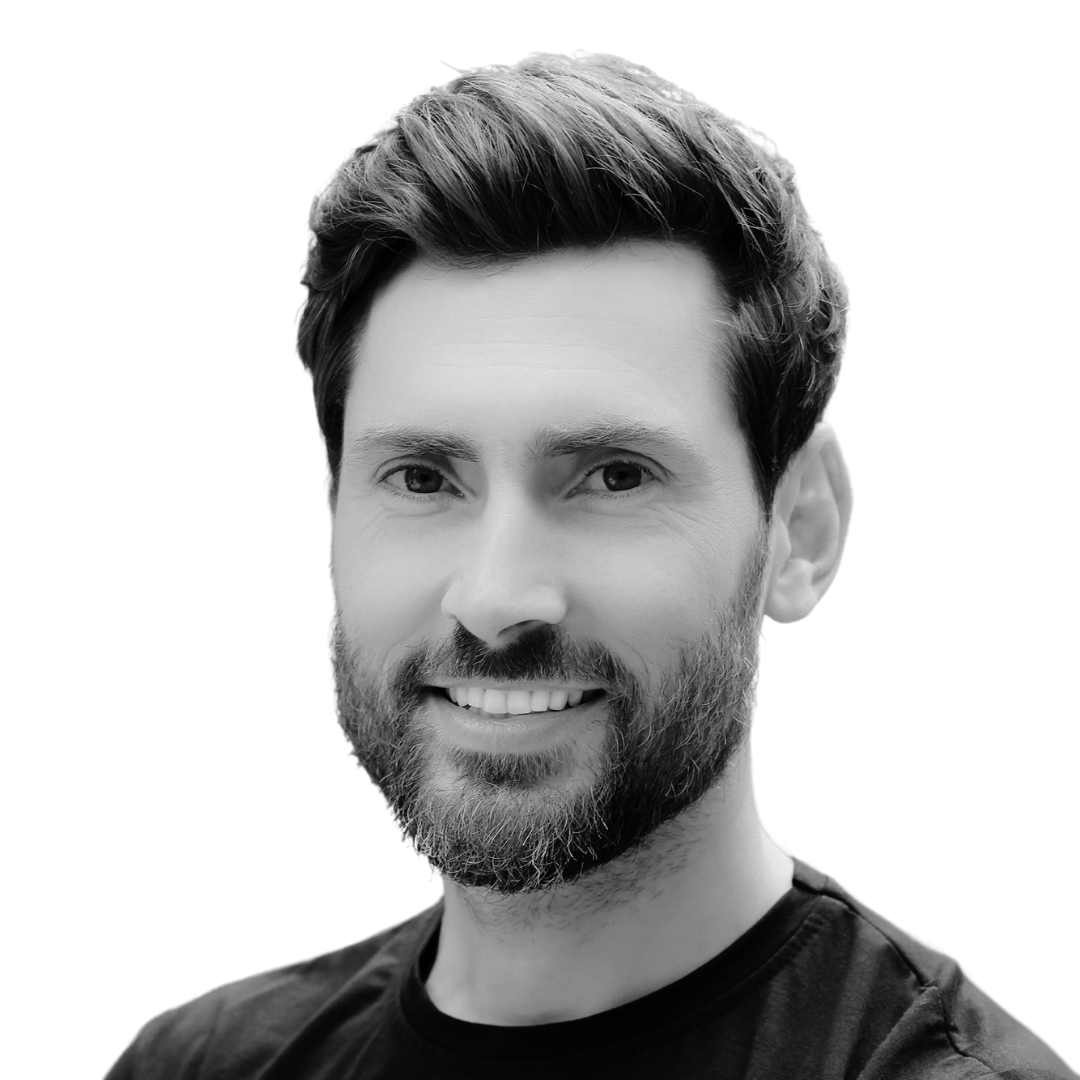
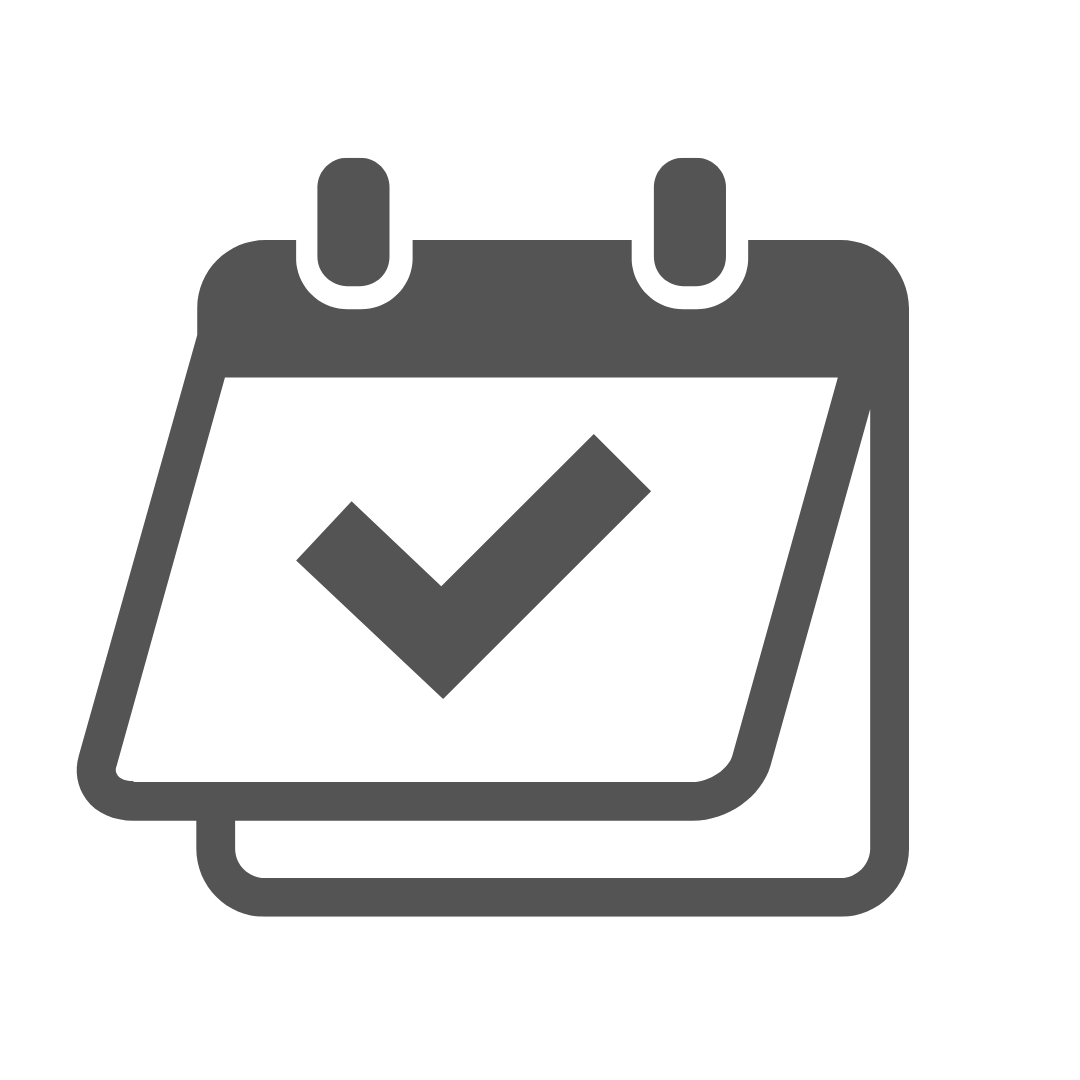
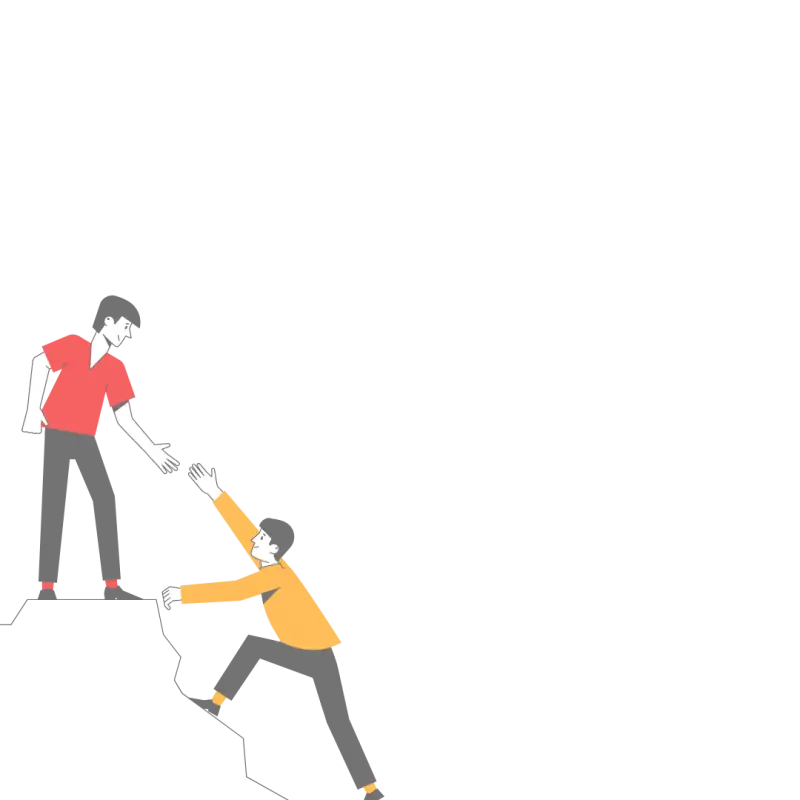
Thanks for your feedback!
Your contributions will help us to improve service.
How can you filter an array in React.js to only include numbers greater than 5?
The code snippet uses React.js to filter an array of numbers. It initializes a state variable called "numbers" with an array containing [2, 8, 4, 10, 6, 1, 7].
The "filter" method is then used on this array to create a new array called "filteredNumbers" that only includes numbers greater than 5. The filtered numbers are rendered as a list using JSX in the React component.
Output of React Js Array filter Method
How can I filter an array of strings in React.js to only include those that contain a specific substring?
This React.js code creates a simple application that filters an array of strings based on a specific substring. It utilizes React's useState
hook to manage the state of the strings array and the filter value.
The filter
function is used to create a new array filteredStrings
that contains only the strings from the original array that include the filter substring.
The filtered strings are then rendered as a list in the UI, with an input field for dynamically updating the filter substring.
Output of React Js Array Filtering strings containing a specific substring
How can I filter objects in React.js based on a specific property value?
The provided code is an example of filtering objects based on a property value using React.js. It sets up a React functional component called "App" that maintains a state variable called "filteredData" using the useState hook.
The component renders a button and a list. When the button is clicked, the "filterData" function is executed, which filters an array of objects based on the condition item.age > 25
.
The filtered array is then stored in the "filteredData" state variable and displayed in the list.
How can you filter an array of objects in React.js based on multiple conditions?
The code snippet demonstrates how to filter an array of objects in ReactJS based on multiple conditions. The data array contains objects with properties like name, age, and gender.
Using the filter
method, the code filters the data based on the specified conditions (e.g., age greater than 24 and gender equal to 'male').
The filteredData array is then rendered in the HTML using a mapping function, displaying the name, age, and gender of each filtered object in a list.
How can I filter out null, undefined values, and empty strings from an array in React.js?
In this React.js code snippet, an array is declared with various values including null, undefined, and empty strings.
The filter
method is used on the array to create a new array, filteredArray
, excluding null, undefined, and empty string values.
The filtered array is then rendered as a list in the React component, displaying only the non-null, non-undefined, and non-empty string values