React Js Display/Show JSON array in table

React Js Display Json Data as Table: To display or show a JSON array in a table using React.js, you can follow these steps. First, import the necessary React components. Then, create a table structure using HTML tags. Within the table, map over the JSON array and create table rows and columns for each item. Access the properties of each item using dot notation. Finally, render the table component in your React application. By doing this, you can dynamically display the JSON array as a table, with each item occupying a row and its properties in separate column
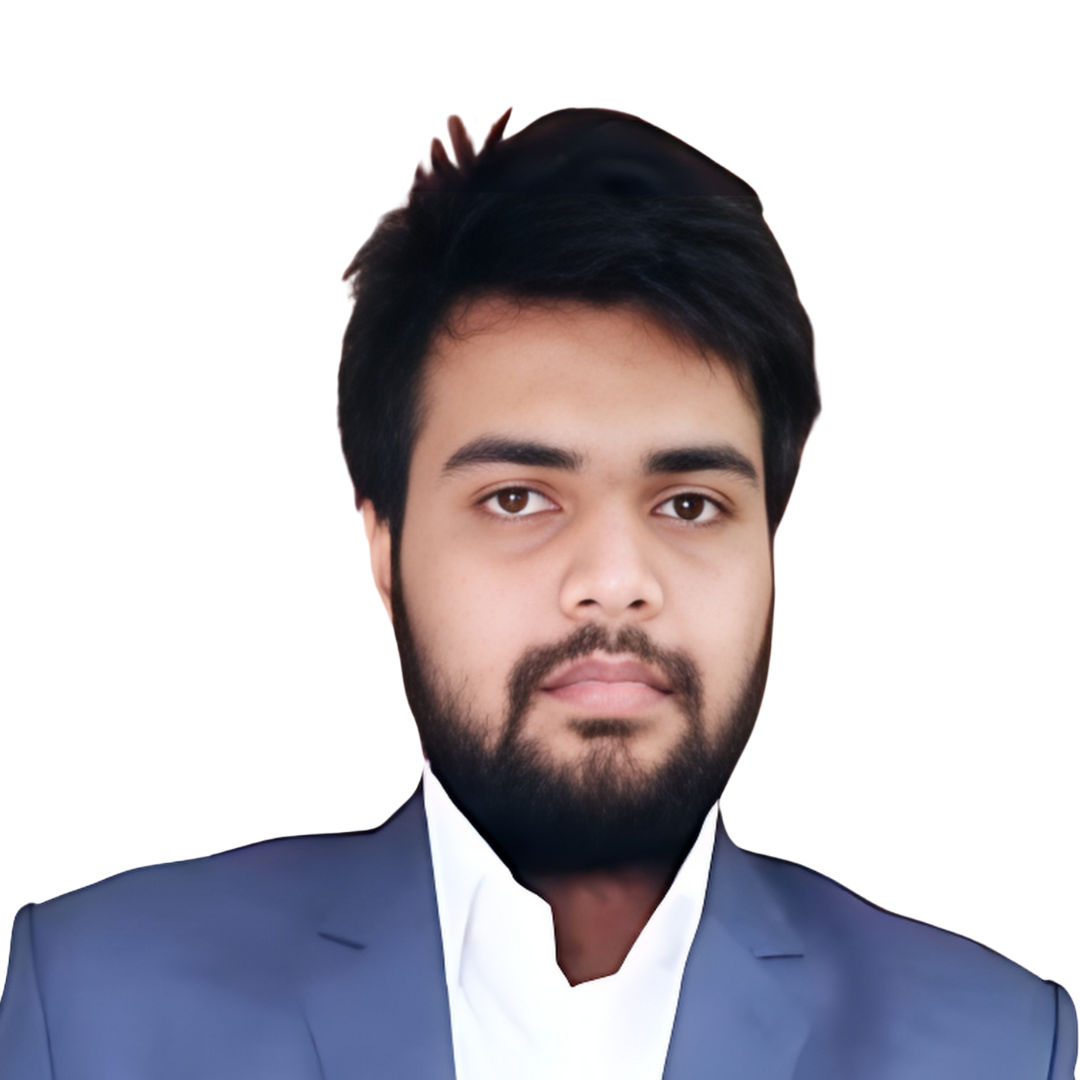
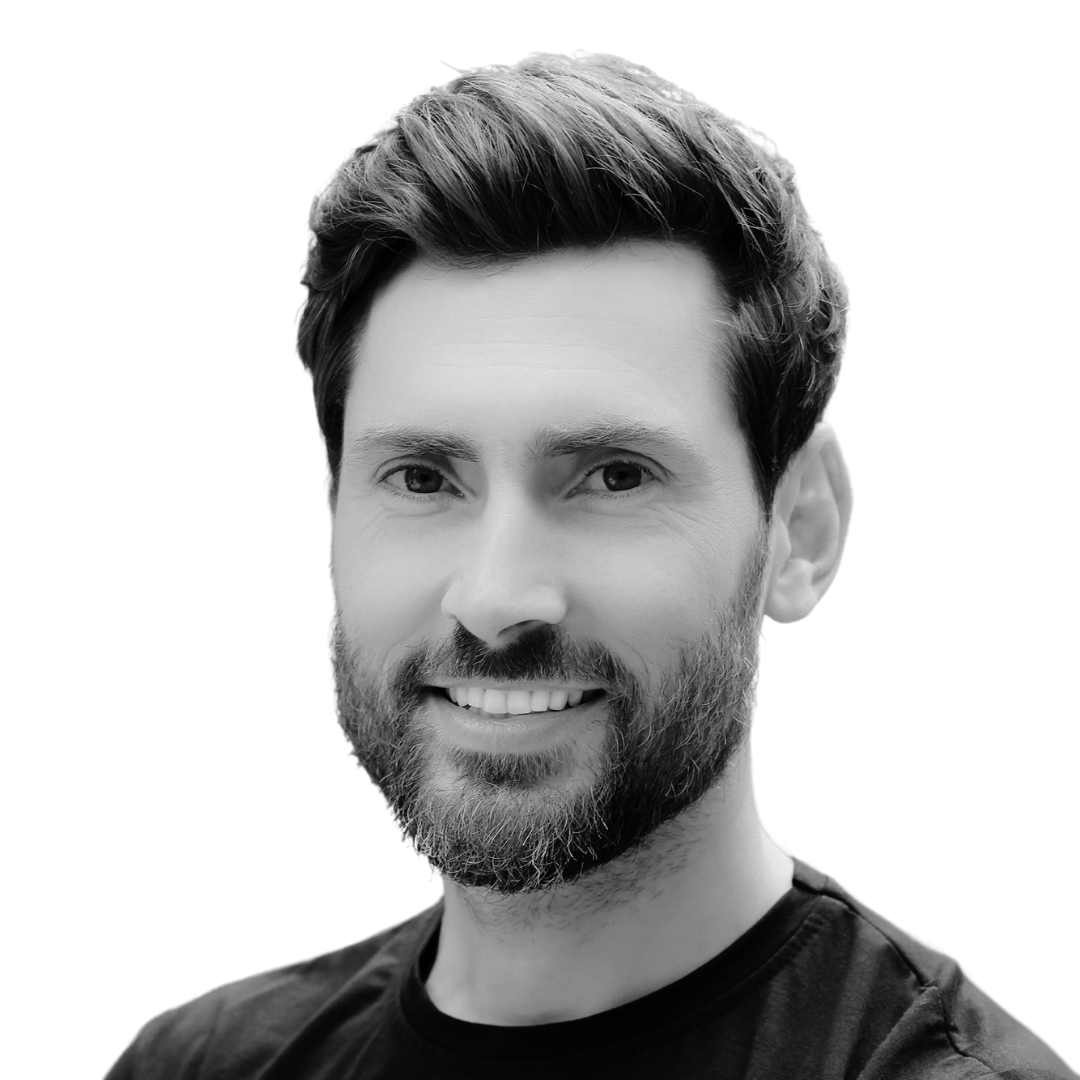
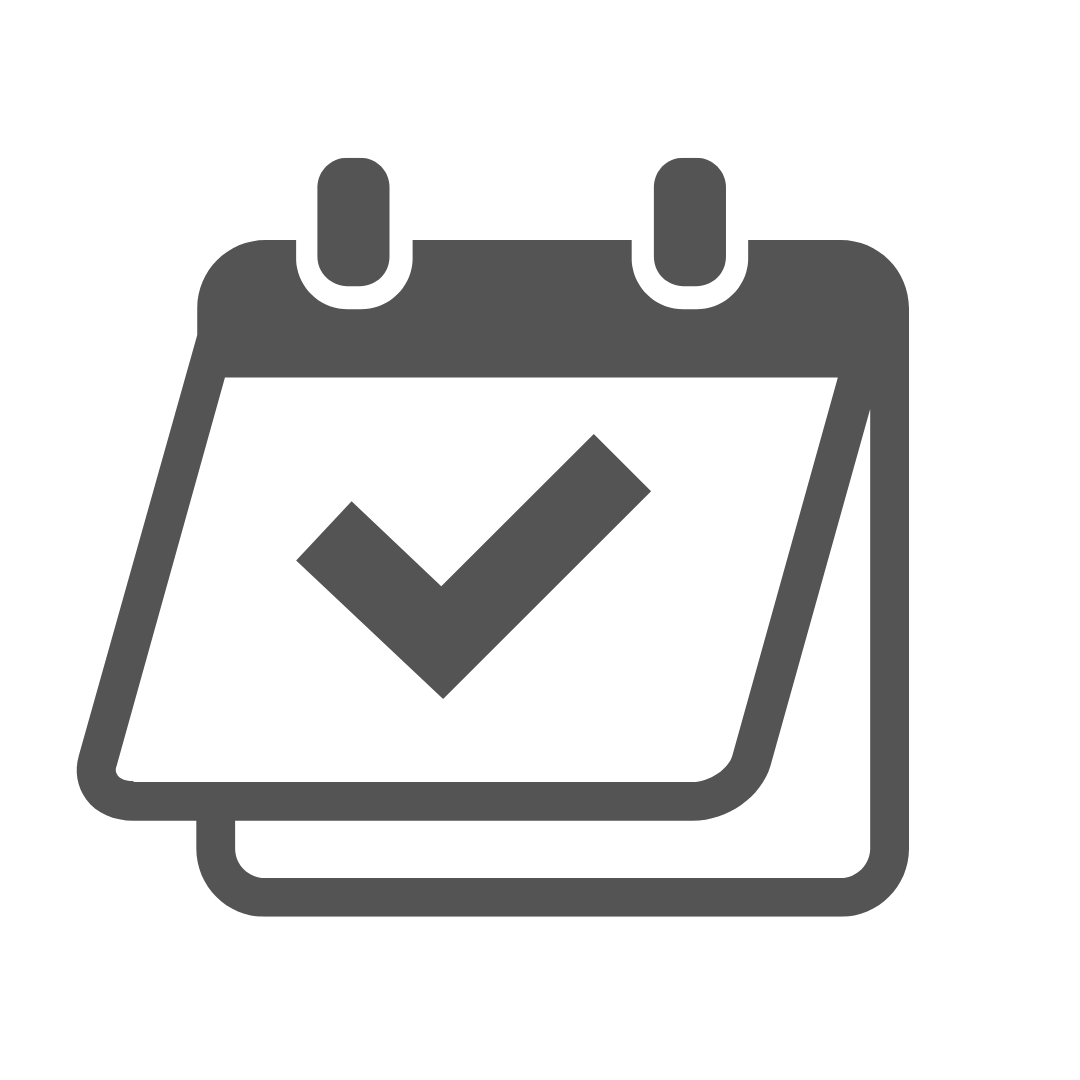
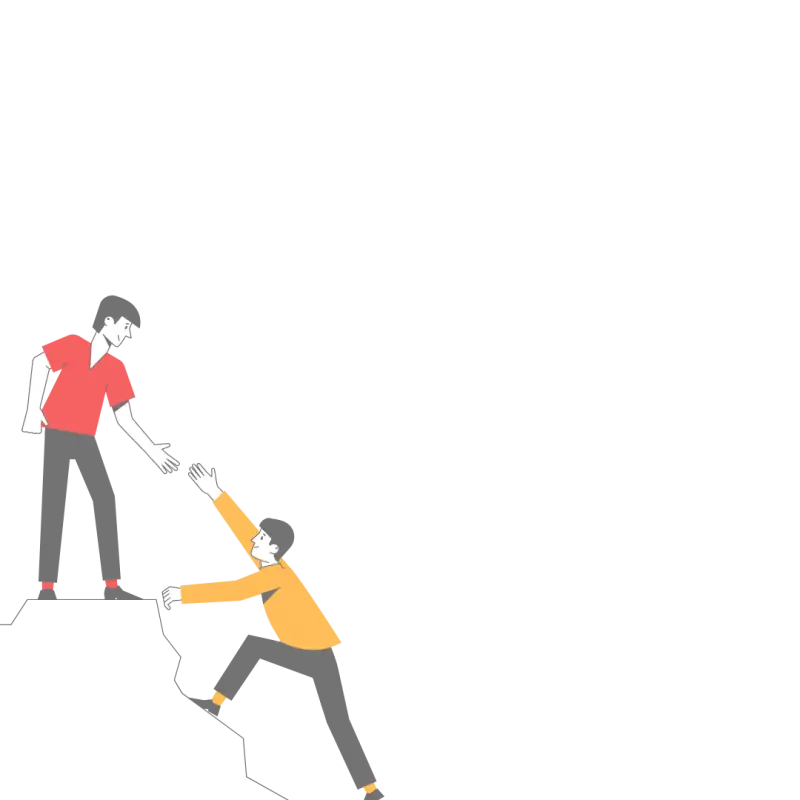
Thanks for your feedback!
Your contributions will help us to improve service.
How can I display/show a JSON array in a table using React.js?
This React.js code displays a JSON array as a table. The code defines a functional component called "App" that uses the "useState" hook to initialize and manage a state variable called "partyData," which is an array of objects. Each object represents a person's data with properties like "id," "name," and "party."
Inside the "return" statement, the code renders a container div with a heading and a table. The table has a header row and a body with rows dynamically generated using the "map" function. Each row displays the ID, name, and party from each object in the "partyData" array.
React Js Display/Show JSON array in table Example
xxxxxxxxxx
<script type="text/babel">
const { useState } = React;
function App() {
const [partyData, setPartyData] = useState([
{
"id": 1,
"name": "John Smith",
"party": "Democratic Party"
},
{
"id": 2,
"name": "Sarah Johnson",
"party": "Republican Party"
},
]);
return (
<div className='container'>
<h1>React JS Display JSON data as table</h1>
<table>
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Party</th>
</tr>
</thead>
<tbody>
{partyData.map((item) => (
<tr key={item.id}>
<td>{item.id}</td>
<td>{item.name}</td>
<td>{item.party}</td>
</tr>
))}
</tbody>
</table>
</div>
);
}
ReactDOM.render(<App />, document.getElementById("app"));
</script>