React Js Find Square Root of Number
.jpg)
In ReactJS, you can calculate the square root of a number using various methods, including the Math.sqrt() function, the Math.pow() function, and the ES6 exponentiation operator. Additionally, you can also find the square roots of multiple numbers within an array. In this post, we will explore how to achieve these functionalities in a ReactJS application
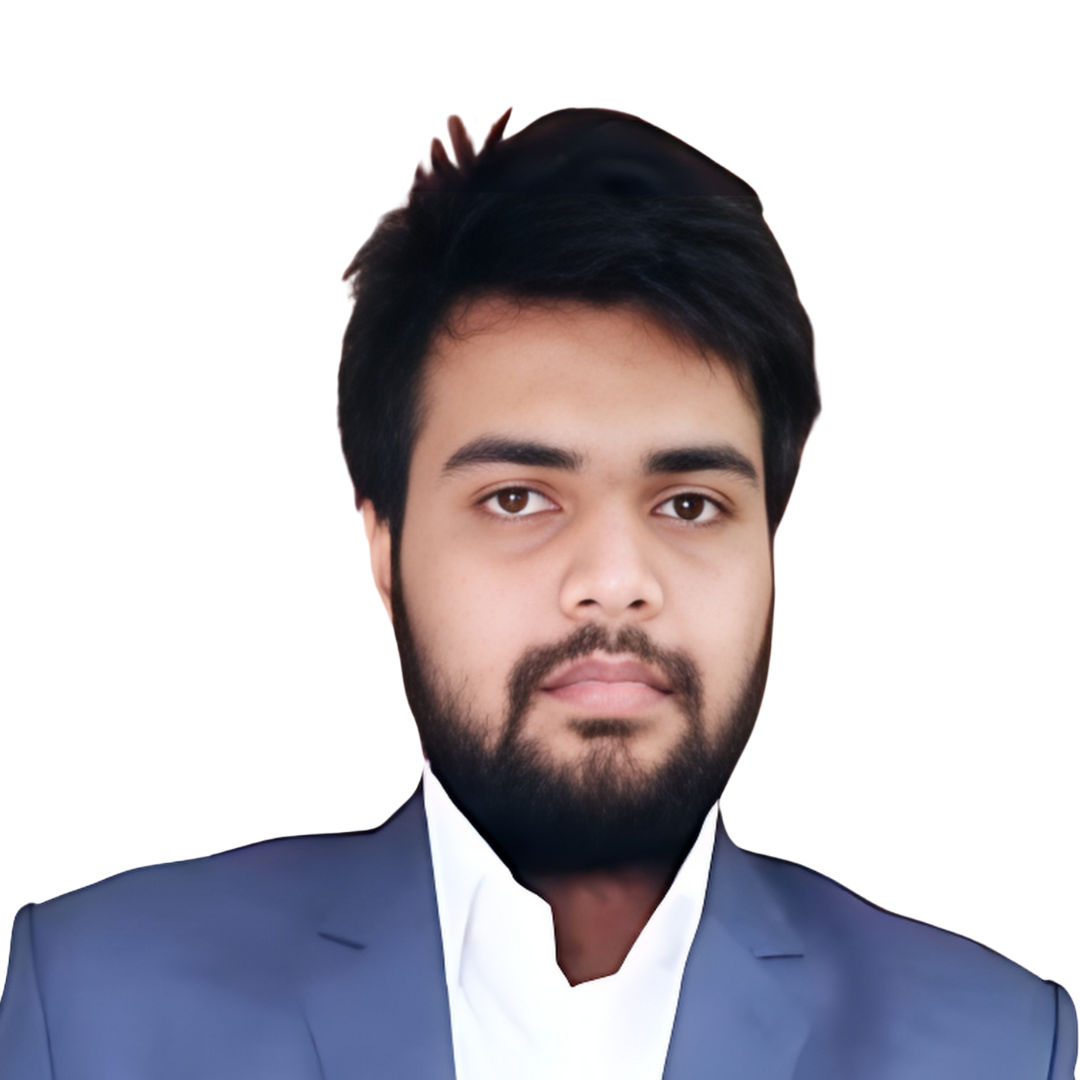
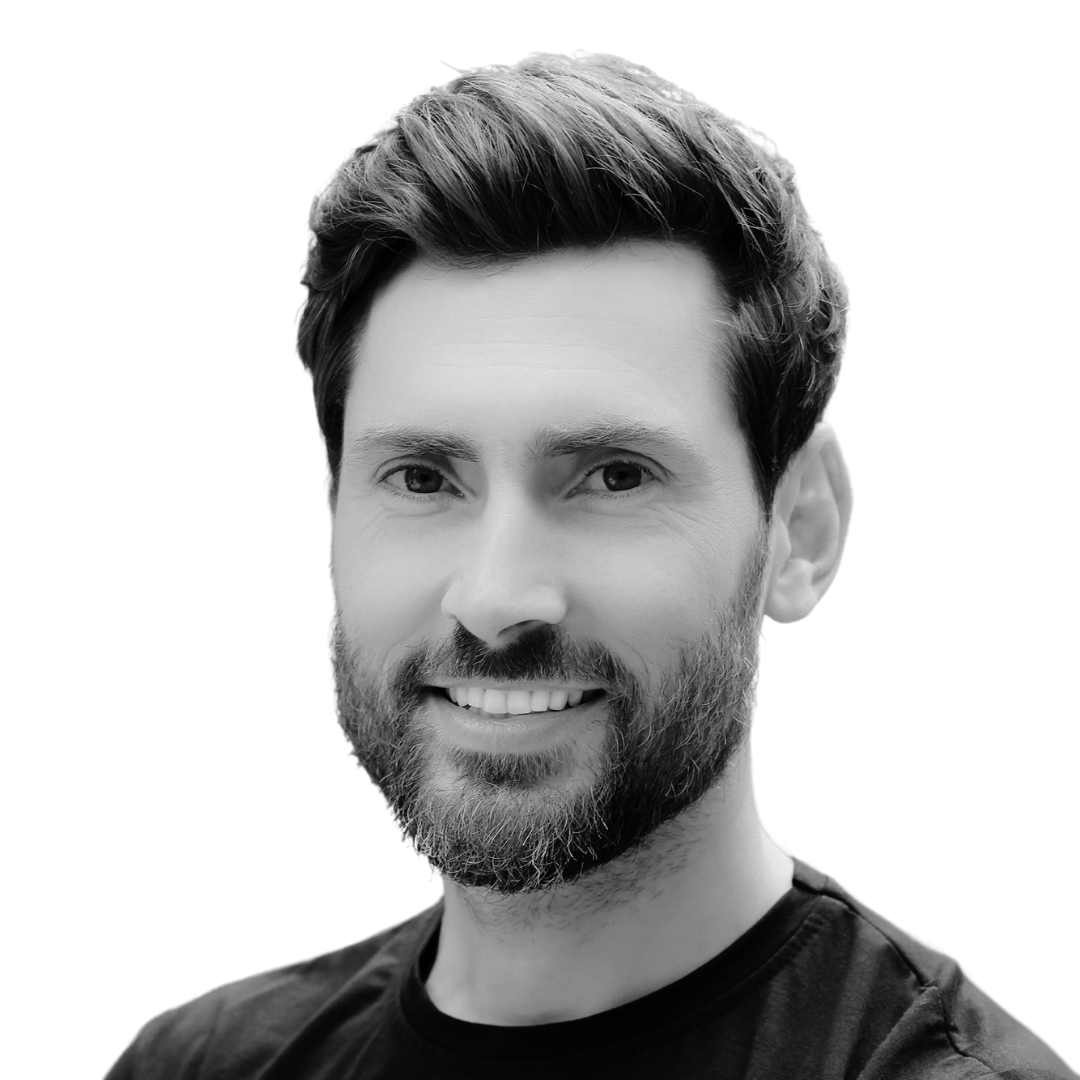
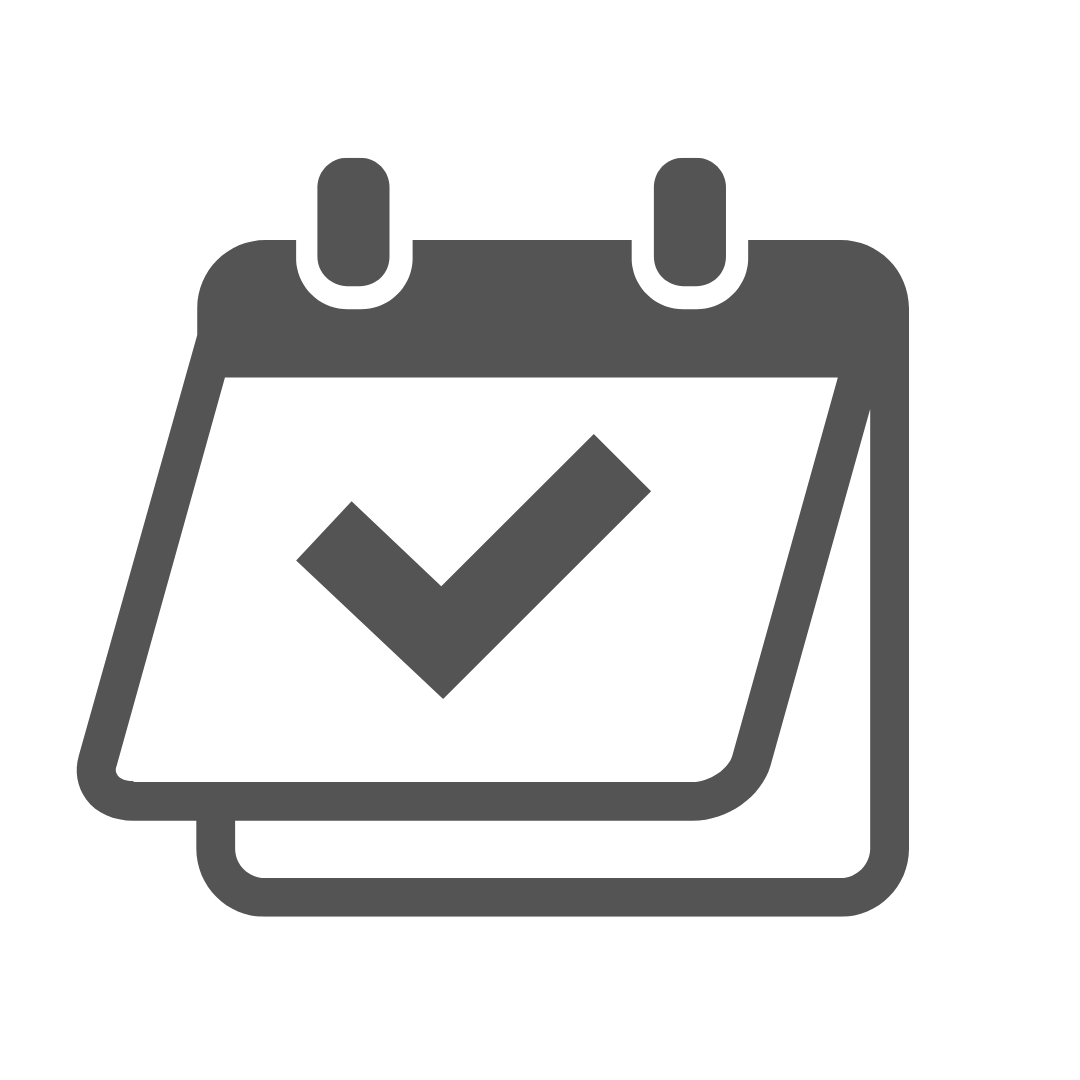
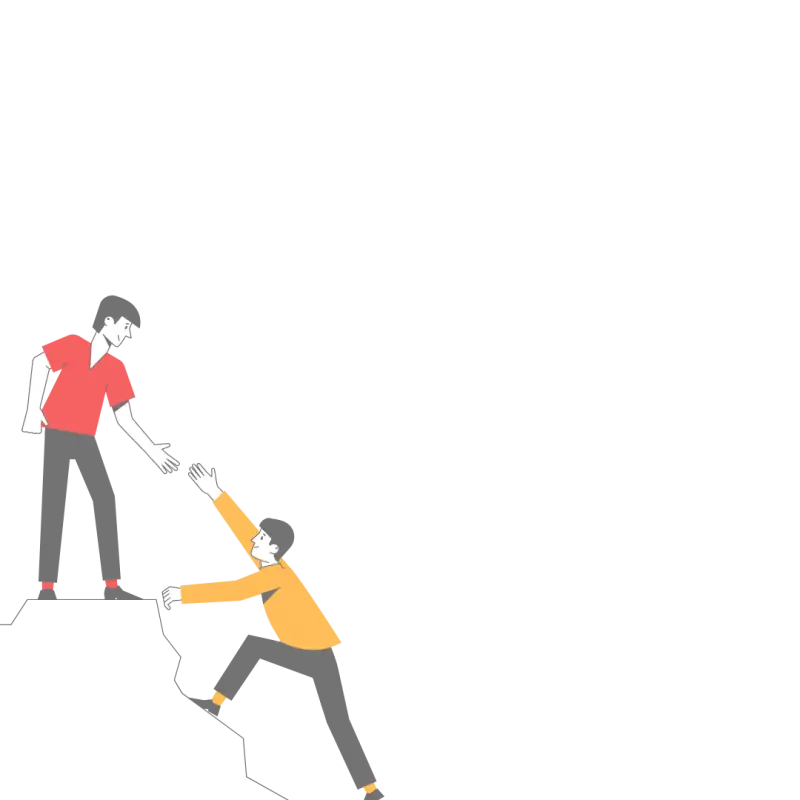
Thanks for your feedback!
Your contributions will help us to improve service.
Calculating Square Root using Math.sqrt()
The first method we will discuss is using the Math.sqrt()
function to find the square root of a single number. Here's a ReactJS component that demonstrates this:
In this example, we use the Math.sqrt()
function to calculate the square root of the number entered by the user. The result is displayed in the UI.
Using Math.pow()
Function
Another way to calculate the square root is by using the Math.pow()
function. Here's a ReactJS component for this approach:
Here, we use the Math.pow()
function with an exponent of 0.5 to find the square root.
Utilizing ES6 Exponentiation Operator
A more modern way to calculate the square root is by using the ES6 exponentiation operator. Here's a ReactJS component that demonstrates this method:
In this example, we use the ES6 exponentiation operator (**
) to find the square root.
So, if you enter a different number in the input field and click the button, it will calculate the square root of that number and display it in the "Square Root" paragraph. Initially, it's set to 400, so the square root will be the square root of 400, which is 20.
Finding Square Roots of an Array
Now, let's explore how to calculate the square roots of multiple numbers within an array. Here's a ReactJS component that does this:
In this component, we start with an array of numbers and use the map
function to calculate the square roots of each number and display the results.
The output of this code
If initialNumbers
contains [4, 9, 12, 56, 67], clicking the button would populate the "Square Roots" section with the calculated square roots of these numbers.
The exact output in the "Square Roots" section would be something like: "Square Roots: 2, 3, 3.464, 7.483, 8.185" (rounded to three decimal places)
These examples showcase various ways to find square roots in a ReactJS application, both for individual numbers and for numbers within an array. You can choose the method that best suits your needs and project requirements.