React Js show Random Image From array
React Js Show Random Image From Array -: In this ReactJS example, an image generator component is created to display a random image from an array. The component utilizes React's state and lifecycle methods to manage the image selection. Upon rendering, a random image is chosen from the predefined array and displayed. Each time the component re-renders, a different random image is shown, creating a dynamic image generator using React.
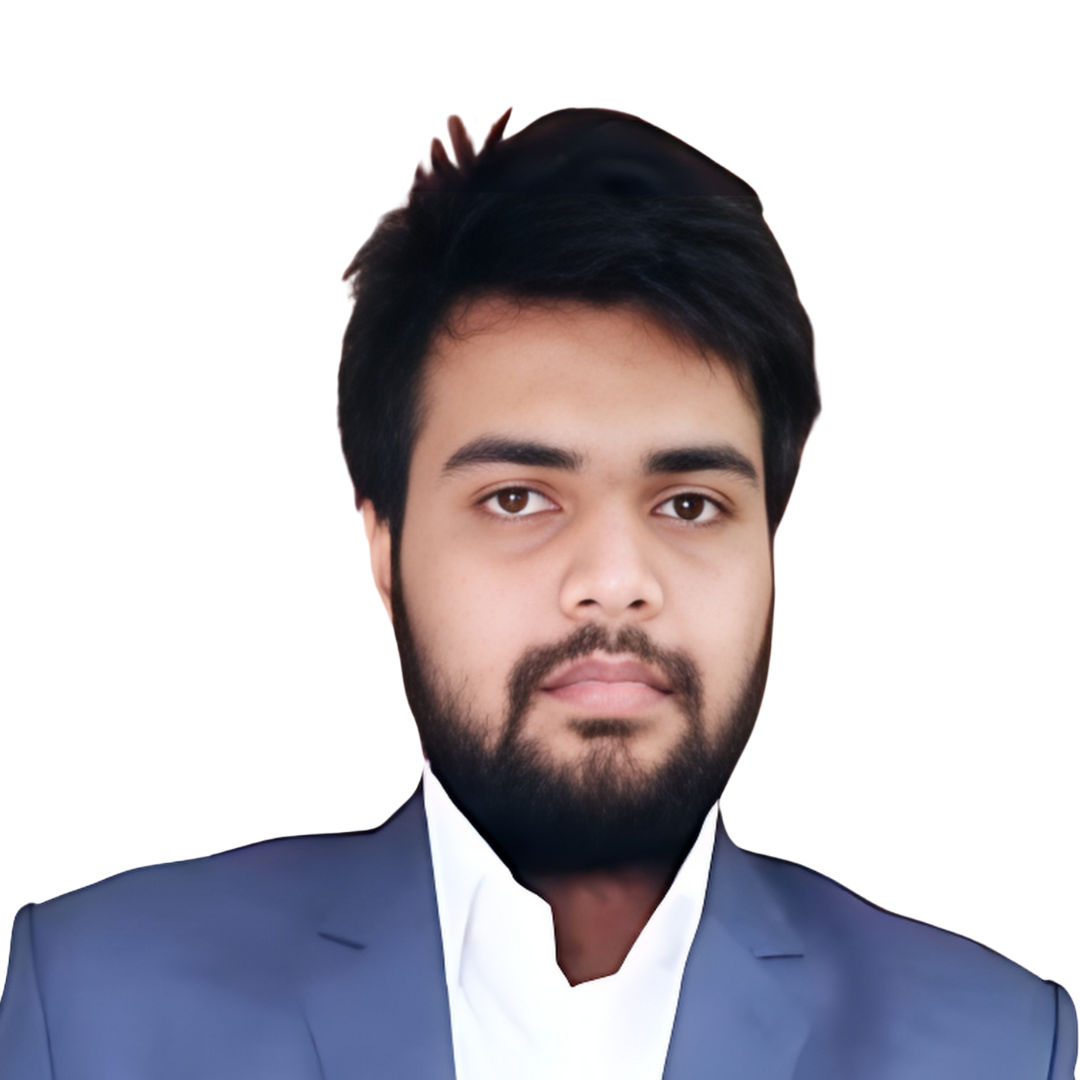
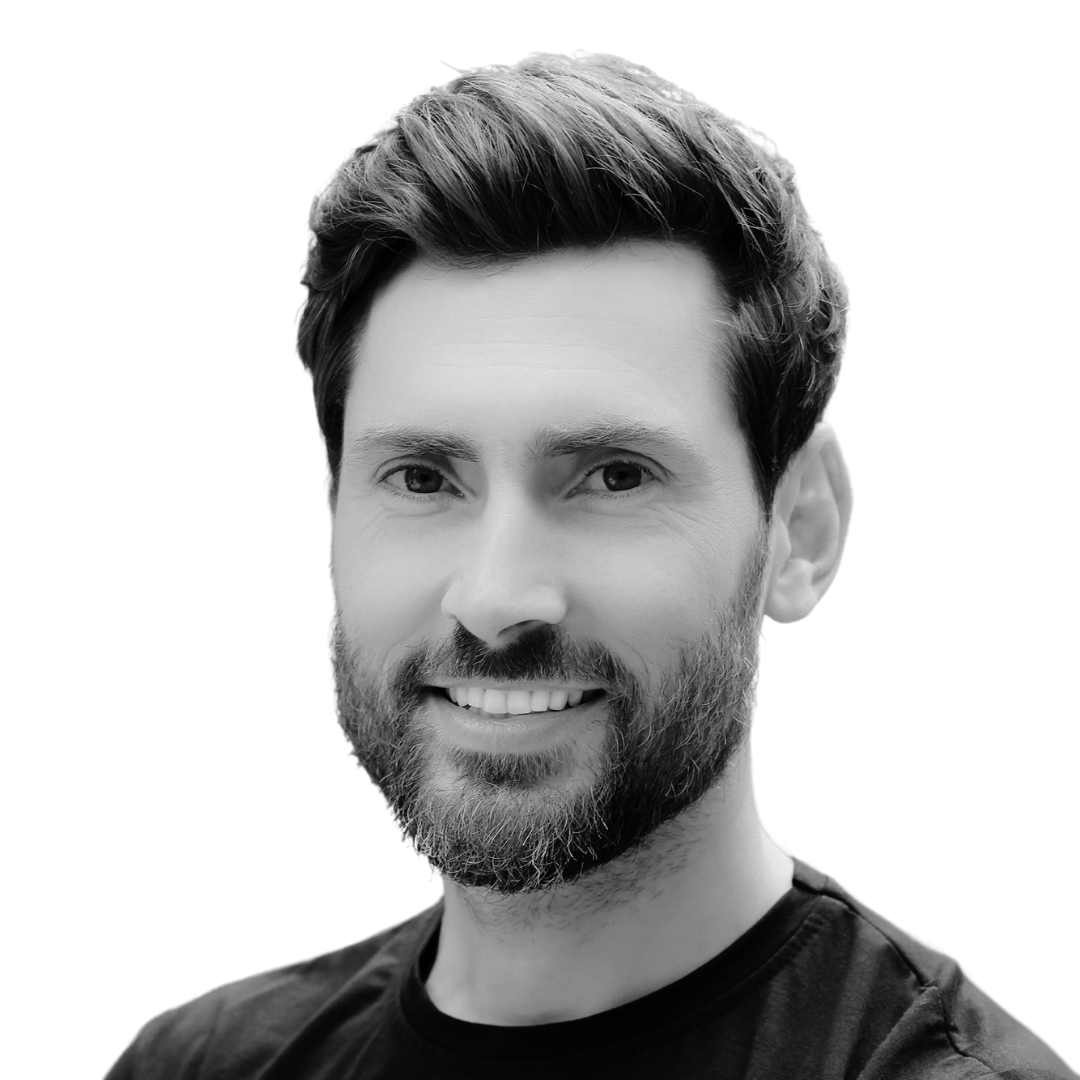
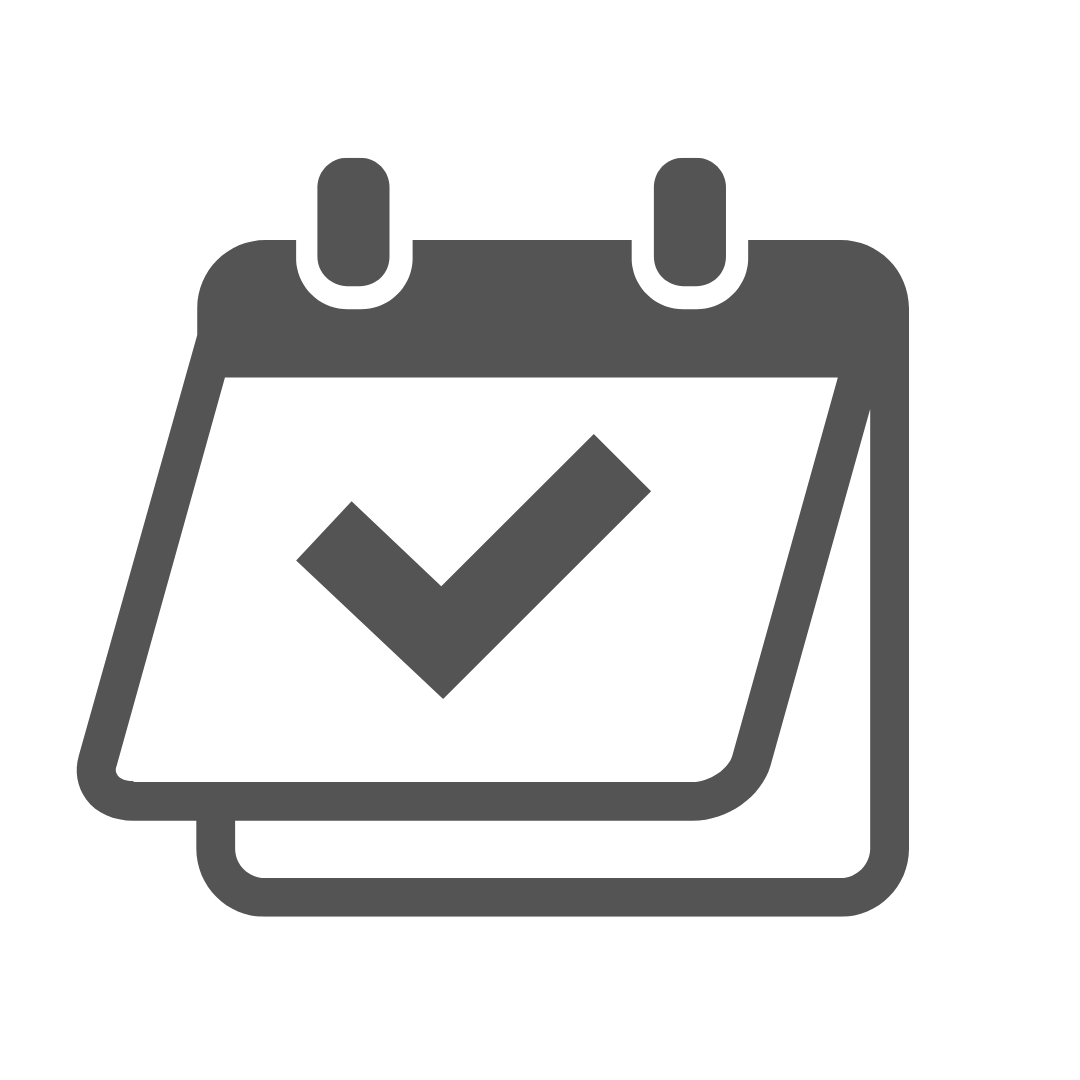
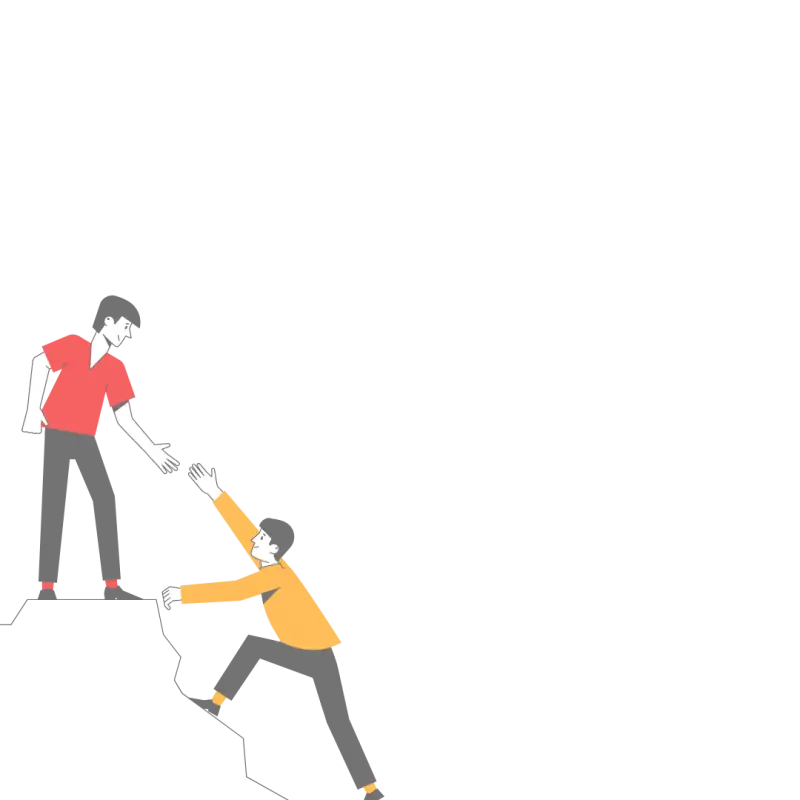
Thanks for your feedback!
Your contributions will help us to improve service.
How to display a random image from an array in Reactjs?
This React JS example displays a random image from an array of image URLs. The component uses React hooks, including useState and useEffect. Upon mounting, it sets a random image as the initial state and renders it. When the "Random Image" button is clicked, a new random image is displayed. The array contains several image URLs, and the getRandomImage function selects a random URL from the array and updates the state with it.