React Format Number with Commas Thousand Separators
In this article, you will learn how to use React Number Format to format numbers with commas and thousand separators in React and JavaScript. You will also learn how to use React Number Format with Material-UI text fields, and how to customize your number formats according to your needs
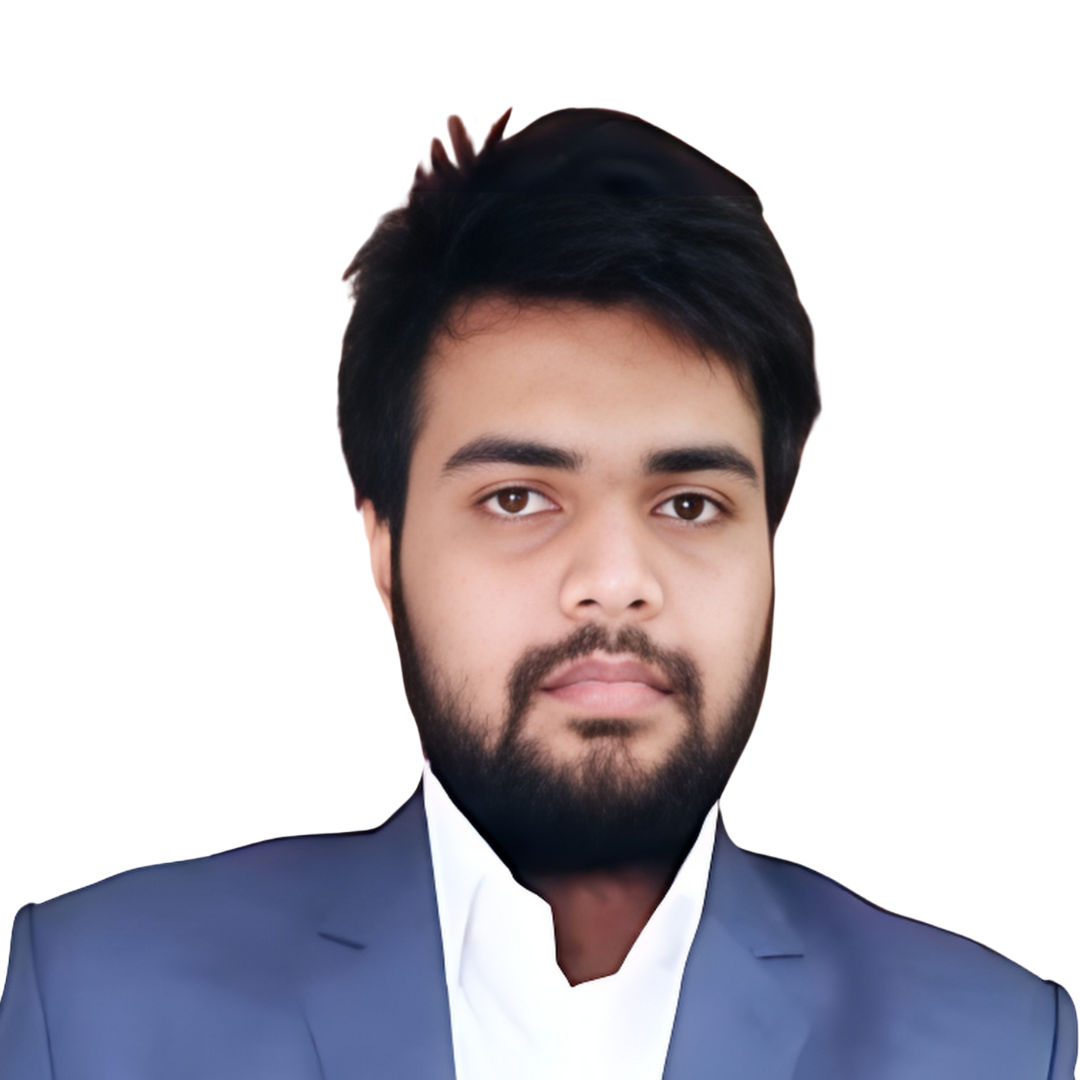
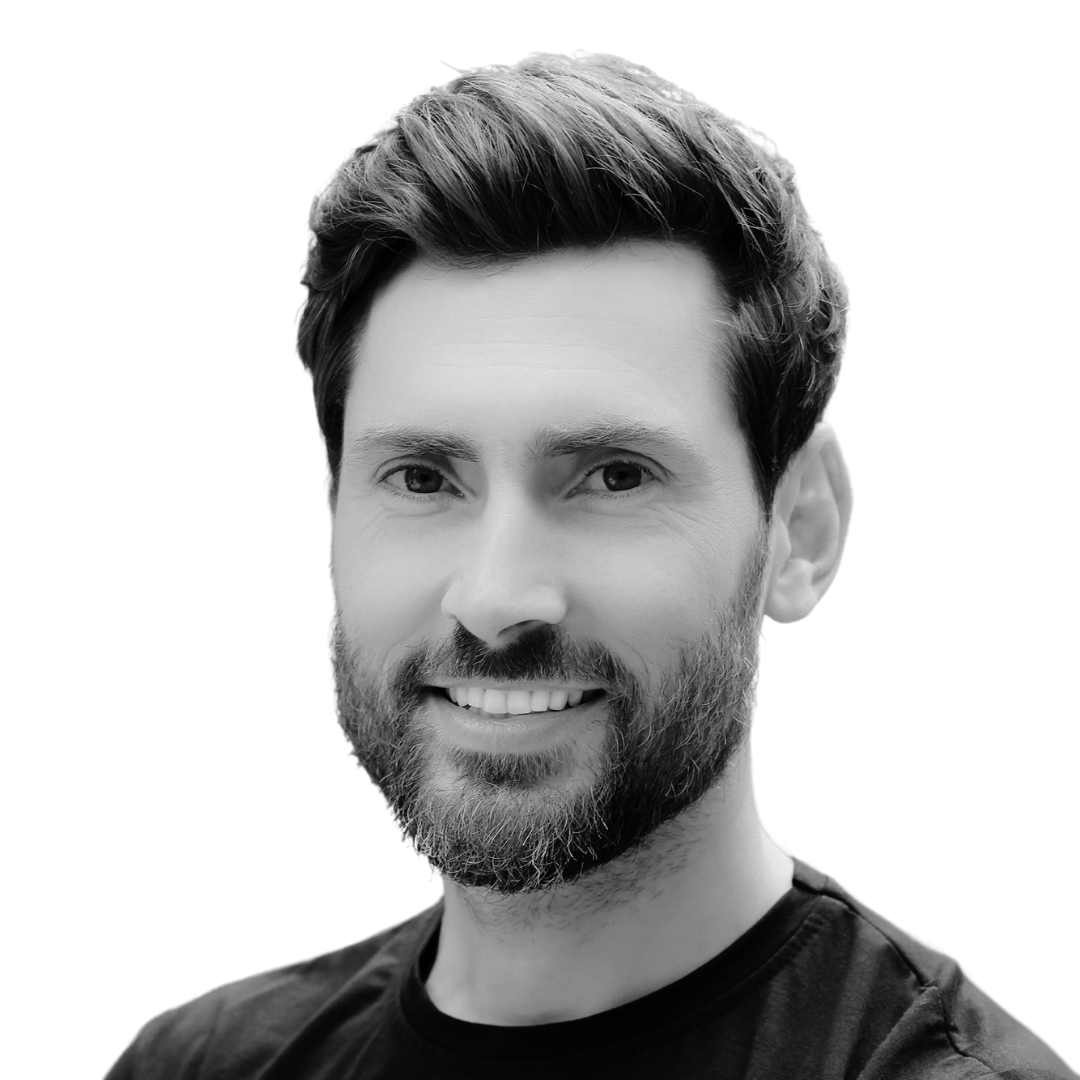
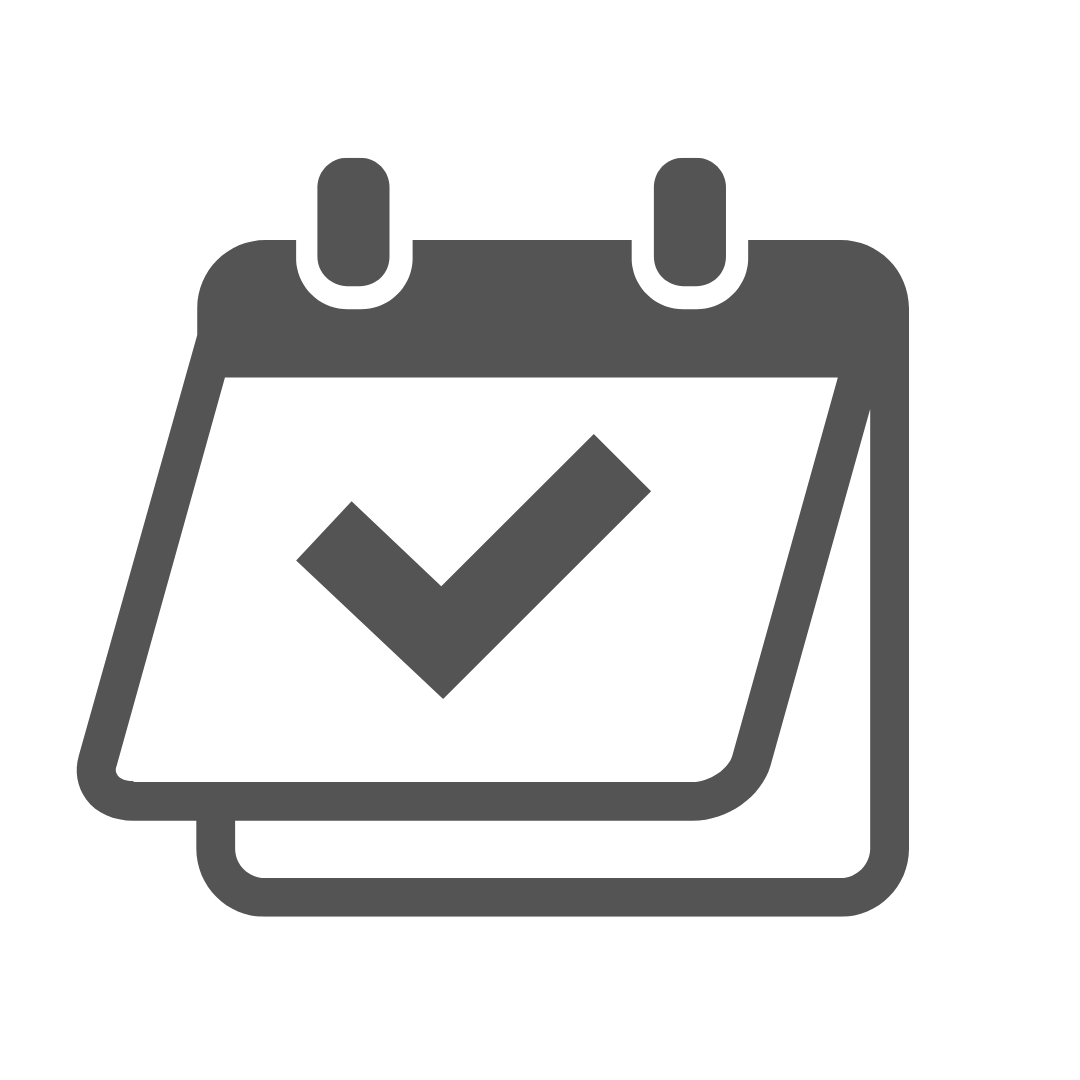
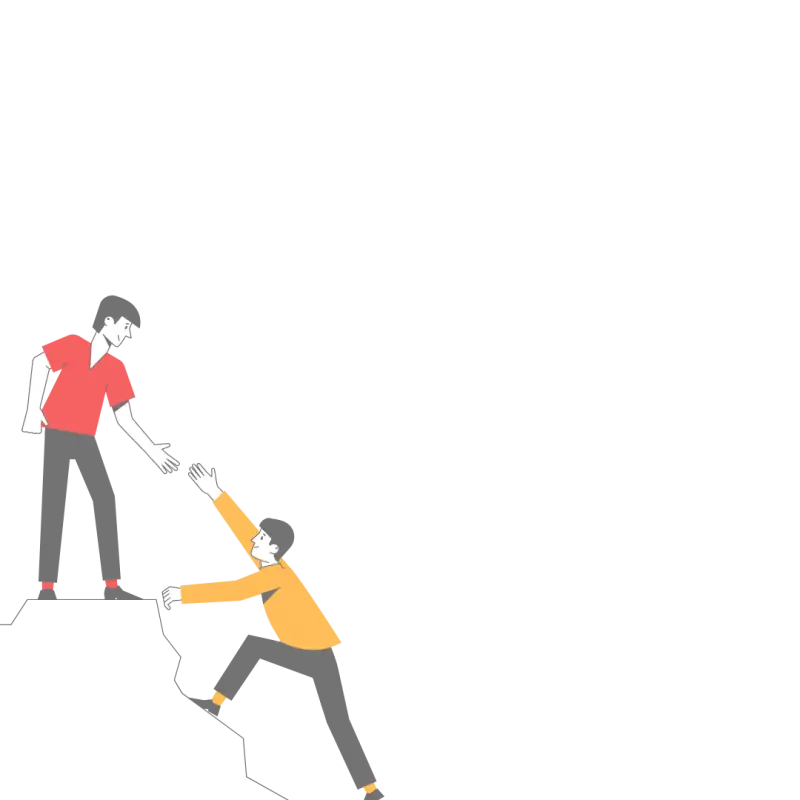
Thanks for your feedback!
Your contributions will help us to improve service.
How can you format a number with commas as the thousands separator in an input field using React.js?
This React.js code snippet demonstrates how to format a number with comma separators in an input field. The code sets up a functional component called "App" that maintains the state of a number using the useState
hook.
The handleChange
function is triggered whenever the input value changes.
Inside the handleChange
function, the input value is sanitized by removing any existing commas using a regular expression. Then, the sanitized value is converted to a number using Number
, and the toLocaleString
method is used to format the number with comma separators
Output of React Js Format Number as Comma Seperator
How can I format a number in Reactjs to a compact and human-readable format?
In this Reactjs code snippet, a component named "App" is created to demonstrate compact number formatting using the Intl.NumberFormat API. The value 12345 is formatted as a compact number using the "en" locale, with the "short" compact display option. The formatted number is then displayed on the webpage.
Output of React Js Compact Format Number
Note: The compact numbers are formatted using abbreviations such as "K" for thousand, "M" for Million, "B" for Billion, and "T" for Trillion. However, if the compactDisplay setting is changed to "long," the abbreviations will be replaced by the full words "thousand," "Million," "Billion," and "Trillion," respectively.
How can I format numbers in Reactjs to display them in US (comma-separated) format?
The provided ReactJS code formats a given number (987,654,321,987) using the US locale with comma separation. It uses the Intl.NumberFormat object to achieve this formatting, which makes the number more readable by adding commas to separate thousands, millions, etc.
Output of React Js Number Format - US (comma separated)
How can I format numbers in Reactjs to use the Indian comma-separated format?
The provided React.js code demonstrates how to format a number in Indian (comma-separated) style using the Intl.NumberFormat
object. The code sets the number
variable to 1,000,000 and then uses the en-IN
locale to create an Indian number formatter. The IndianFormattedNumber
variable stores the formatted number with commas.
Output of React Js Number format - Indian (comma separated)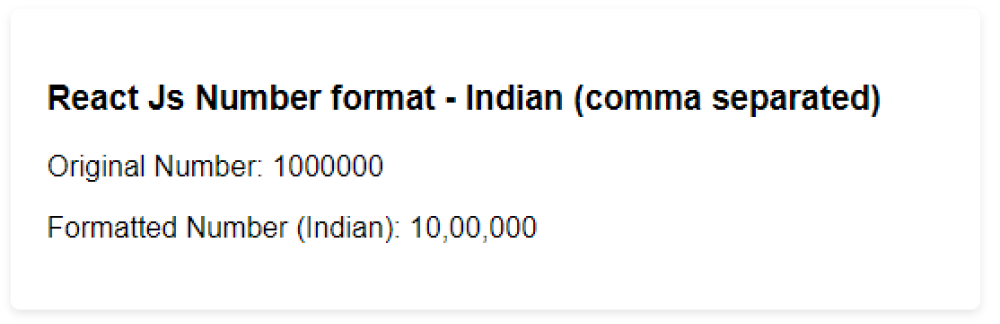
How can I format numbers in React.js to follow the Swiss convention of using apostrophe as a separator (e.g., 1'000'000)?
The provided React JS code formats a large number (98765432198) using the Swiss locale (de-CH) to display it in a more readable format. The formatting separates thousands using an apostrophe. The output will be shown in the web page as:
React Js Number Format - Swiss (apostrophe separated) Original Number: 98765432198 Formatted Number (Swiss): 98'765'432'198
Output of React Js Number Format - Swiss (apostrophe separated)
How can I format numbers in Reactjs to use the Italian convention with dot as a decimal separator?
This React.js code formats a given number in Italian style with dot as the decimal separator. It demonstrates five different methods to achieve this formatting. The first method utilizes Intl.NumberFormat
, the second method uses a custom function with regex, the third method employs toLocaleString
with Italian options, the fourth method uses parseFloat
with fixed decimal places and comma as the separator, and the fifth method swaps the decimal and thousands separators using regex. The formatted numbers are displayed on the webpage using different approaches.
Output of React Js Format Number - Italian (dot separated)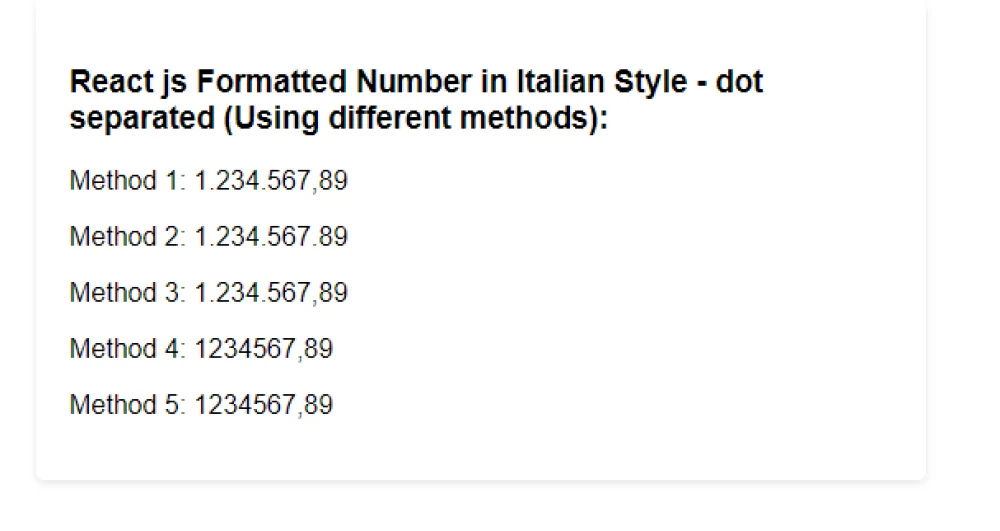
How can I format numbers in Reactjs to be displayed in the Swedish format with space as the thousands separator?
This React JS code snippet formats a number (1234567.89) in the Swedish locale with space as the separator. It uses the Intl.NumberFormat
object to achieve this. The formatted number is then displayed on the webpage using ReactDOM.
Output: React Js format Number - Swedish (space separated) Original Number: 1234567.89 Formatted Number (Swedish): 1 234 567,89
Output of React Js format Number - Swedish (space separated)
How can I format numbers in React.js to use Russian style with space as a thousands separator?
This React.js code snippet formats a number (12345676) using Russian conventions (space-separated thousands) by using the toLocaleString
method with the "ru-RU" locale. The result is displayed on the web page as follows:
React Js format Number - Swedish (space separated) Original Number: 12345676 Formatted Number: 12 345 676
Output of React Js format number - Russian (space separated)
How can I format numbers in Reactjs to be hyphen-separated?
This React.js code formats a number with hyphen-separated groups. The function formatNumberWithHyphen
takes a number as input, removes non-digit characters, and then splits the digits into groups of 3 from right to left. It finally joins these groups with hyphens and returns the formatted string. The FormatNumberApp
component displays the original number (1234567890) and the formatted number (123-456-789-0) in the browser using ReactDOM.