React Js Get Maximum Value from Array
React Js Get Maximum Value from Array:To obtain the maximum number from an array in ReactJS, you have several options.
One approach is to use the Math.max
function. For example, Math.max(...array)
spreads the elements of the array as arguments to find the maximum value.
Another option is to utilize the reduce
method. array.reduce((max, current) => max > current ? max : current)
iterates through the array and returns the maximum value.
Alternatively, you can employ the sort
method by writing array.sort((a, b) => b - a)[0]
to sort the array in descending order and retrieve the first element, which represents the maximum value.
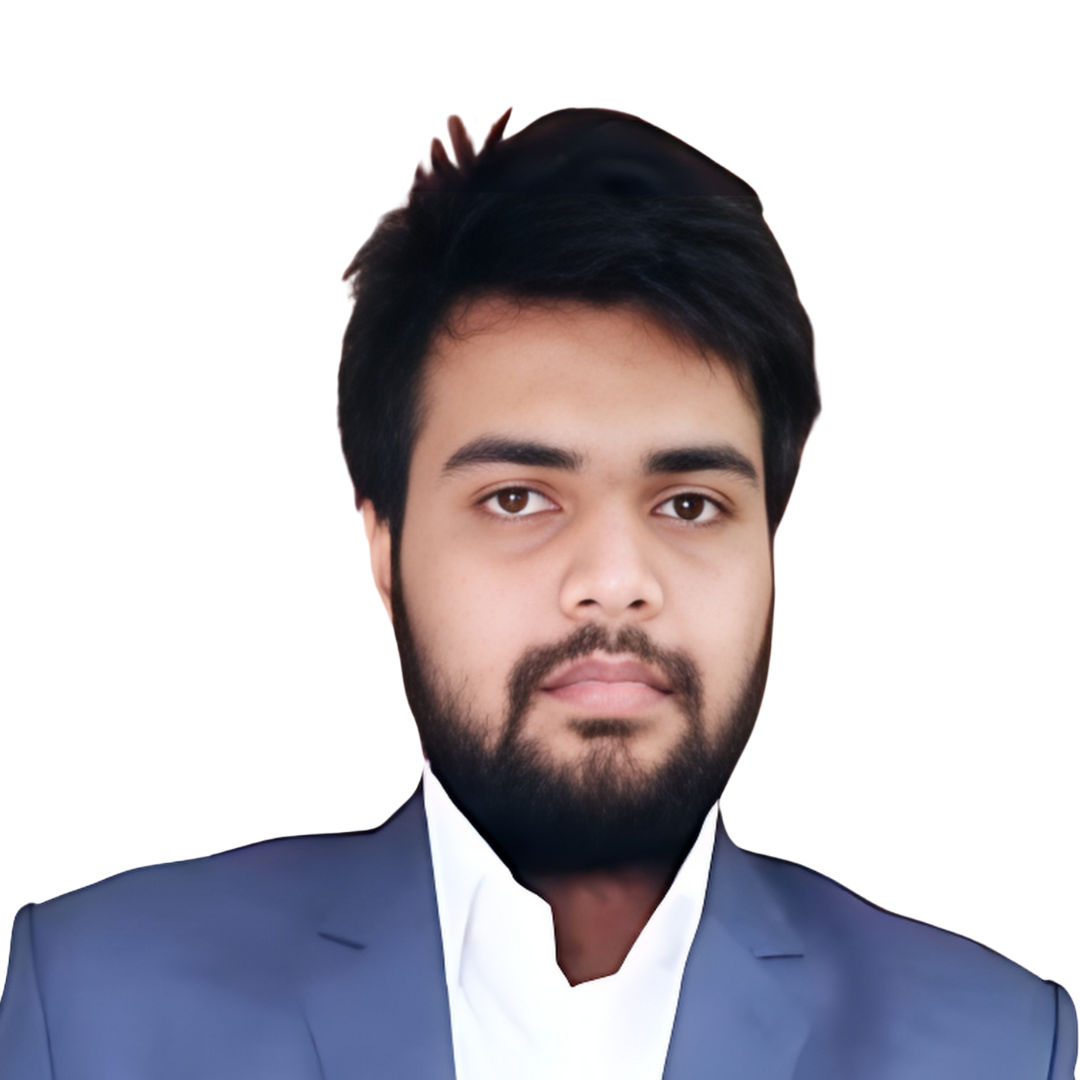
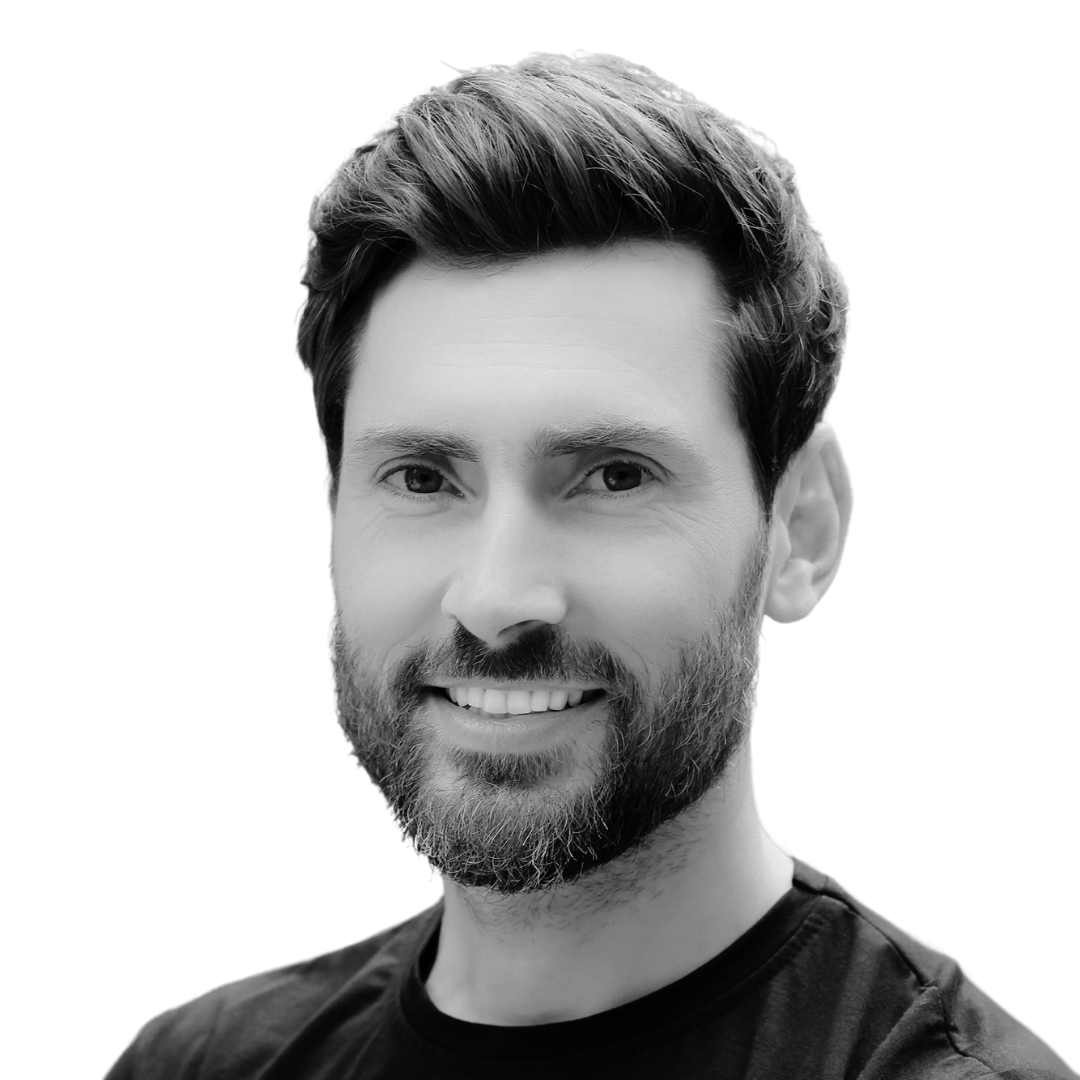
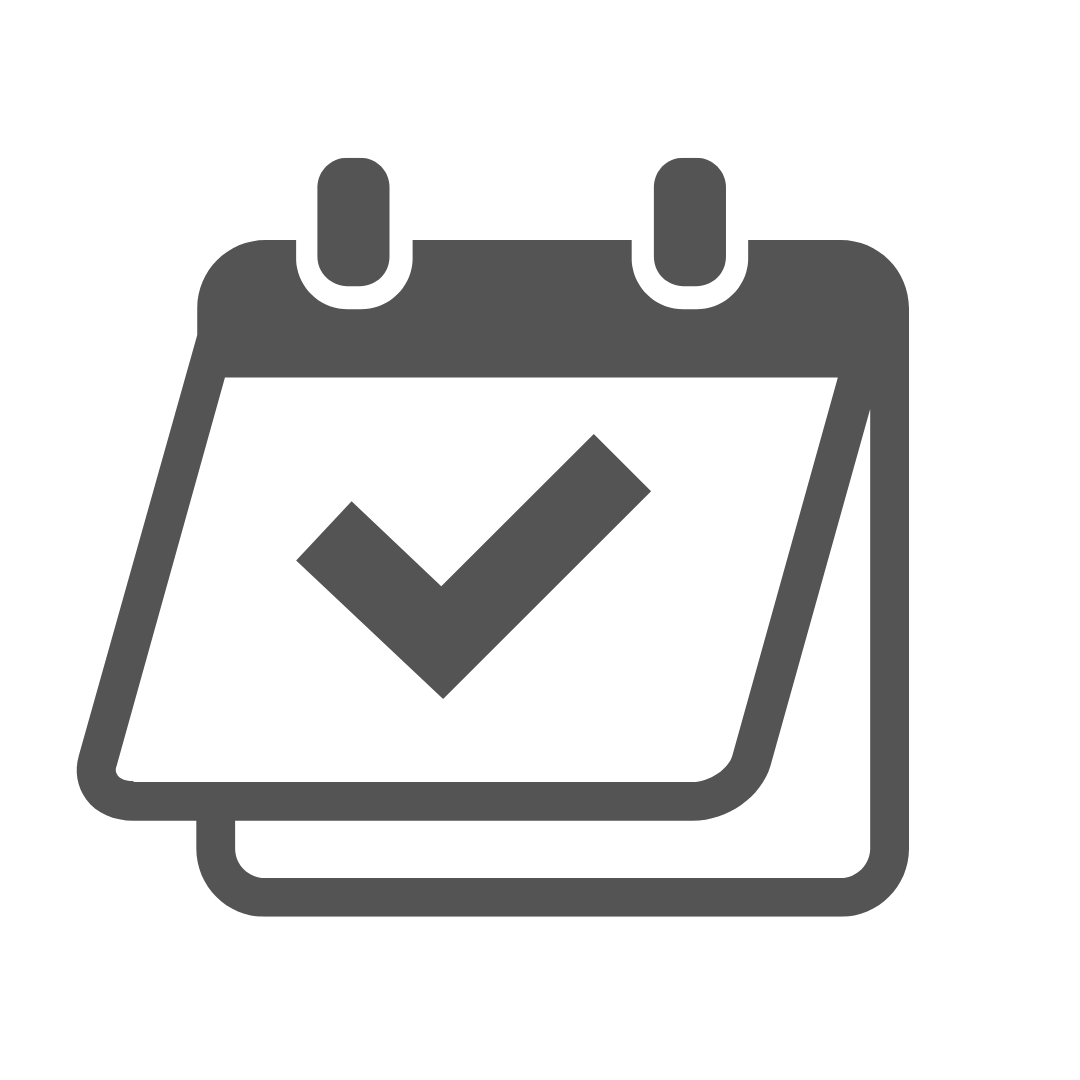
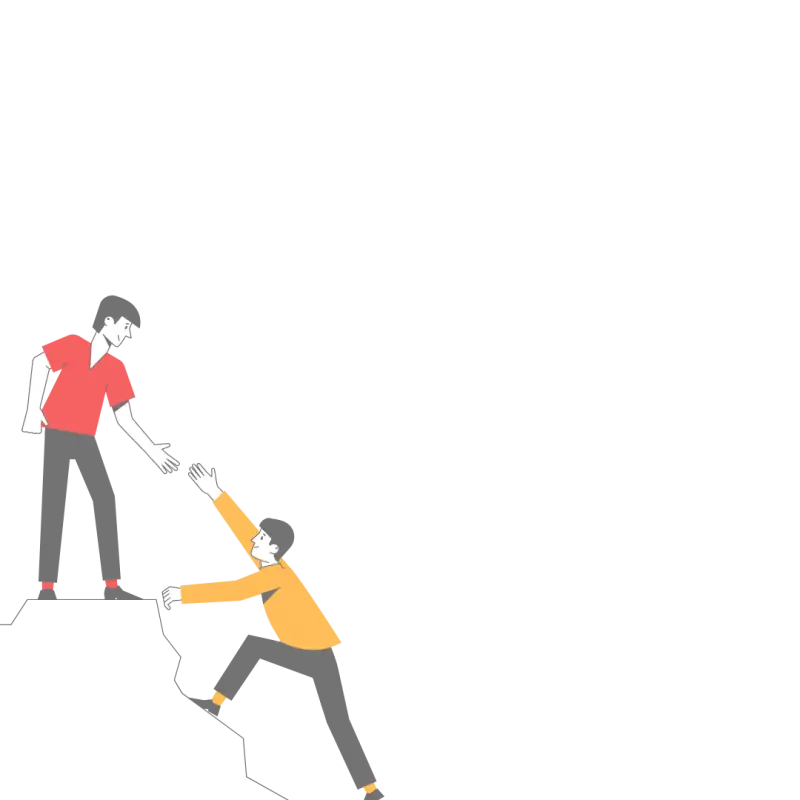
Thanks for your feedback!
Your contributions will help us to improve service.
How can I use the Math.max()
function in React.js to retrieve the maximum value from an array?
In this React.js code snippet, we have a functional component called "App" that uses the useState hook to define an array called "numbers" with some values.
The Math.max() function is then used to find the maximum value in the array by spreading the array elements using the spread operator (...).
The maximum number is displayed in the JSX markup along with the original array.
Output of React Js Get Maximum Value from Array
How can you use the reduce
method in React.js to retrieve the maximum value from an array?
This React.js code snippet uses the reduce
method to find the maximum value from an array of numbers. It initializes the numbers
state with an array [10, 5, 8, 20, 3]
.
The reduce
method compares each element of the array with the accumulator (acc
) and returns the maximum value.
The initial value of the accumulator is set to Number.NEGATIVE_INFINITY
to ensure any number in the array will be greater. The maximum number is displayed on the webpage.
What is the JavaScript code using the sort
method to get the maximum number from an array in React.js
This React.js code snippet sorts an array of numbers in ascending order and retrieves the maximum value from it. It utilizes the useState hook to initialize the array.
By using the sort method with a comparison function, the array is sorted in ascending order.
The maximum value is then obtained by accessing the last index of the sorted array. Finally, the maximum number is displayed in the rendered React component.